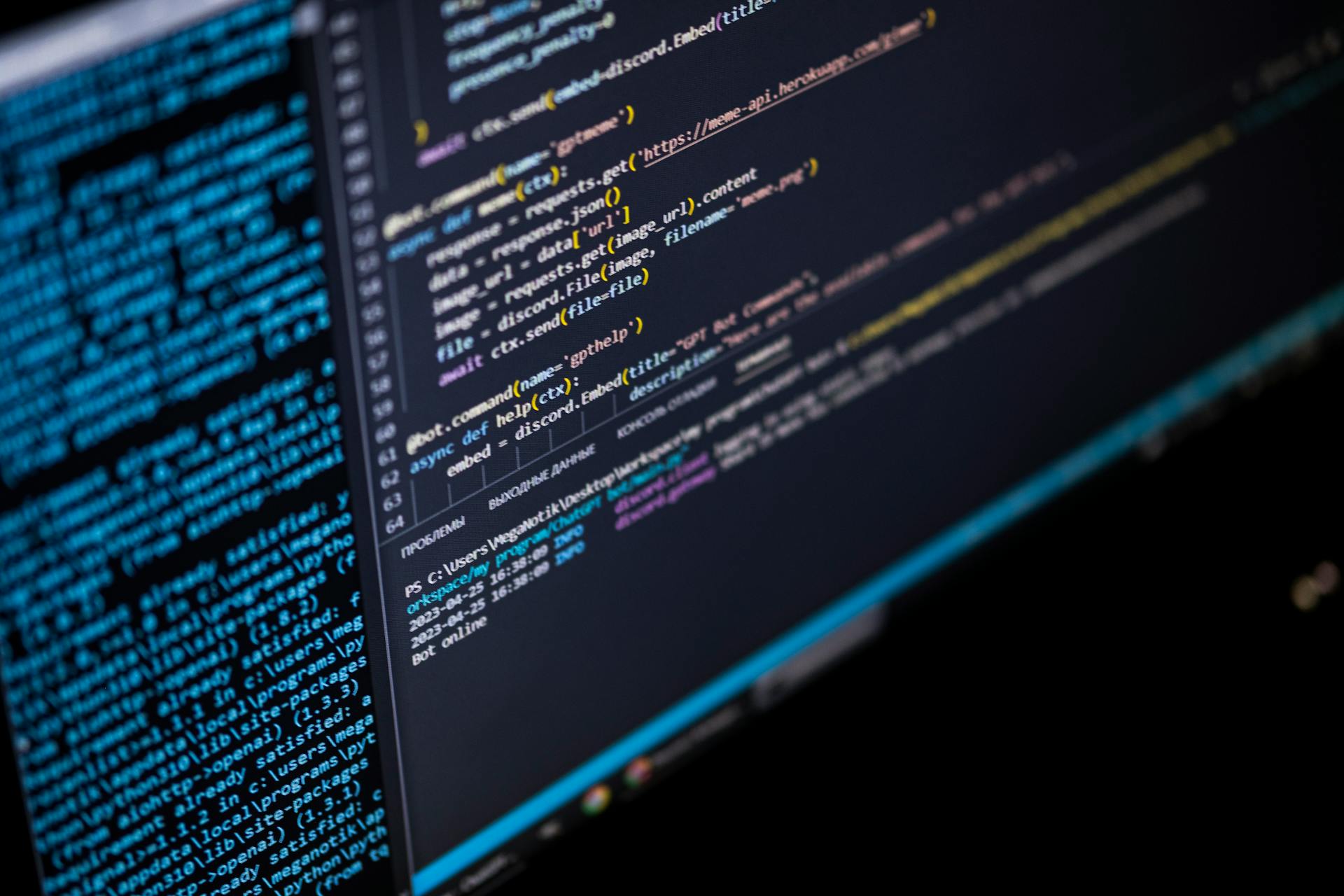
Nextjs SSG is a powerful tool for creating fast and secure websites. It uses a technique called Static Site Generation (SSG) to pre-render pages at build time.
With Nextjs SSG, you can pre-render pages as static HTML files, which can be served directly by a web server without the need for server-side rendering. This approach provides better performance and security compared to traditional server-side rendering.
By using Nextjs SSG, you can take advantage of its built-in support for internationalization and localization, making it easy to create multilingual websites.
For your interest: Nextjs Rendering
Static Site Generation
Static Site Generation is a game-changer for web development, transforming the way we build and deploy websites. It's been around for two decades, with pioneers like Movable Type in 2001 and Jekyll in 2008 leading the way.
Early adopters of SSG were mostly small, fast-loading sites, but it's since evolved into a must-have framework for high-performance applications. The use of SSG surged around 2015 with the advent of the Jamstack architecture, promoting a static-first approach to web development.
You might like: Nextjs Ssg App Router
Next.js has become a leading choice for static site generation since introducing this capability in version 9.3, released in March 2020. The framework combines the speed and SEO benefits of SSG with a powerful ecosystem, enabling developers to create fast, SEO-friendly websites without compromising on user experience.
Here are the key benefits of using SSG:
- Speed: SSG generates pre-rendered HTML, which means faster page loads and improved user experience.
- SEO: Search engines can easily index static HTML files, improving SEO and search rankings.
- Scalability: SSG reduces the load on servers, making it ideal for high-traffic websites.
To get started with SSG in Next.js, you'll need to create a new project and configure it to use static site generation. This involves creating a new Next app, modifying the project configuration, and running the build command to generate static HTML files.
Here's a brief overview of the project structure:
- `pages`: contains all our pages and defines routing for our app
- `pages/api`: contains API endpoints (can be removed if not needed)
- `public`: contains static assets
By using SSG in Next.js, you can create high-performance, secure, and scalable web apps that elevate the user experience.
Configuring Next.js
Configuring Next.js is crucial for a smooth deployment experience. Certain Next.js versions require specific Node.js versions, so it's essential to configure a specific Node version by setting the engines property in your package.json file.
To configure routing and middleware, you need to exclude routes starting with .swa in your middleware configuration. This can be achieved by adding a matcher in your middleware.ts (or .js) file to exclude routes starting with .swa.
Here are the steps to configure middleware and routing for a successful deployment to Static Web Apps:
- Exclude routes starting with .swa in your middleware.ts (or .js) file in your middleware configuration.
- Configure your redirects in next.config.js to exclude routes starting with .swa.
- Configure your rewrite rules in next.config.js to exclude routes starting with .swa.
If your application size exceeds 250 MB, you can enable the standalone feature in Next.js to optimize app size and enhance performance. To do this, add the following property to your next.config.js:
Configure Runtime Version
Configuring the runtime version for Next.js is crucial to ensure compatibility with specific Node.js versions. Certain Next.js versions require specific Node.js versions.
You can designate a version by setting the engines property of your package.json file. This ensures that the correct Node.js version is used during the build process.
Next.js versions that require specific Node.js versions will throw errors if the incorrect version is used. It's essential to configure the correct version to avoid these issues.
To configure a specific Node version, you can set the engines property of your package.json file to designate a version. This property is used to specify the required Node.js version for your application.
By following these steps, you can ensure that your Next.js application is built with the correct Node.js version, preventing errors and ensuring smooth deployment.
Set Environment Variables
Setting environment variables is crucial for Next.js, as it uses them at build time and request time to support both static page generation and dynamic page generation with server-side rendering.
You'll want to set environment variables both within the build and deploy task, and in the Environment variables of your Azure Static Web Apps resource.
Next.js relies on environment variables to function properly, so it's essential to get this setup right from the start.
Make sure to set environment variables correctly to avoid any issues with your Next.js application.
Enable Logging
Enabling logging for Next.js is a crucial step in troubleshooting server API errors. This involves adding logging to the API to catch errors that may occur.
If this caught your attention, see: Nextjs Api
To do this, you can use Application Insights, which is a logging tool provided by Azure. You'll need to create a custom startup script to preload the Application Insights SDK.
A preload script for Application Insights + Next.js can be found in the relevant documentation. If you encounter any issues, you can refer to the GitHub issue for troubleshooting.
Preloading with Next.js requires some specific configurations, but following the example provided can help you get started.
For your interest: Next Js Script
Without Data
In Next.js, you can create a page using Static Site Generation without fetching external data.
You can do this by creating a simple React component, like the About component, which renders a static text.
This component is pre-rendered as a static HTML file during the build process.
Running npm run build and then npm start generates a static version of the about.js page, which serves as an HTML file, providing fast and efficient rendering for users accessing the page.
This approach is useful for pages that don't require external data to render.
Enabling Features
You can enable the standalone feature in Next.js to optimize app size and performance. This feature creates a compressed version of the application with necessary package dependencies, built into a folder named .next/standalone.
The standalone feature can help deploy your app on its own without node_modules dependencies, making it a great solution for apps exceeding 250 MB.
To enable the standalone feature, add the following property to your next.config.js:
You'll also need to configure the build command in the package.json file to copy static files to your standalone output.
Here are the steps to enable the standalone feature:
- Add the property to next.config.js
- Configure the build command in package.json
Enable logging for Next.js by adding logging to the API to catch errors. This is especially useful for server API troubleshooting.
To preload the Application Insights SDK, you'll need to create a custom startup script.
Data and Fetching
In Next.js SSG, fetching external data is a crucial step in pre-rendering pages. You can load data however you like, but in this tutorial, we'll be using GraphQL with Apollo Client.
To get started, you need to install some Apollo-related packages by running the command in your terminal. This will set up the foundation for your data fetching.
There are two scenarios for fetching external data: page content fetches only external data, and content fetches from specific page paths from external data. To handle the first scenario, you can use the getStaticProps function to fetch data at build time and pass it as props to the page's component.
The getStaticProps function is used to fetch data at build time and pass it as props to the page's component. It's a crucial function in Next.js SSG.
To handle dynamic routes, you need to export an asynchronous function named getStaticPaths from a dynamic page. This function is executed at build time, giving you the flexibility to specify which paths should be pre-rendered.
Here's an example of creating a dynamic route for showing a single blog post based on its id:
In this example, the filename is [id].js, indicating that it's a dynamic route with the parameter id. The getStaticPaths function fetches external data to determine dynamic paths based on the id parameter.
Frequently Asked Questions
Is NextJS still popular?
Yes, Next.js remains a highly popular choice among web developers, having jumped from 11th to 6th place in the Stack Overflow survey of 2023. Its growing acceptance and effectiveness in the developer community continue to drive its popularity.
Sources
- https://pagepro.co/blog/how-to-use-next-js-static-site-generator/
- https://learn.microsoft.com/en-us/azure/static-web-apps/deploy-nextjs-hybrid
- https://staticmania.com/blog/how-can-we-use-ssg-in-next-js
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://opyjo2.hashnode.dev/how-nextjs-has-enhanced-the-react-ecosystem-ssg-and-ssr
Featured Images: pexels.com