
Nextjs and Supabase are a powerful combination for building fast and scalable applications. Supabase is a PostgreSQL database with a RESTful API and real-time capabilities, while Nextjs is a React-based framework for building server-rendered and statically generated applications.
Supabase provides a simple and intuitive API for interacting with the database, making it easy to integrate with Nextjs. With Supabase, you can easily manage data and perform CRUD (Create, Read, Update, Delete) operations.
To get started with Nextjs Supabase application development, you'll need to install the Supabase client library in your Nextjs project. This will allow you to interact with the Supabase database and perform operations such as creating and reading data.
Explore further: Nextjs Database
Project Setup
To set up your project, start by creating a new project in the Supabase Dashboard. This is as simple as following three steps: create a new project, enter your project details, and wait for the new database to launch.
You can then initiate your project with Next.js by running the command "nextjs-supabase" in the terminal. This will create a new app folder where you'll set up the Next.js app template.
To connect to your Next.js app later, you'll need to install the Supabase client package. You can do this by running one of the following commands: "npm install @supabase/supabase-js" or "yarn add @supabase/supabase-js".
Once the app has finished setting up, you can open the folder in your favorite code editor and remove the basic template in your src/app/page.tsx file. Replace it with an h1 heading saying "Welcome to Workout App."
Here's a quick rundown of the steps to set up your Supabase project:
- Create a new project in the Supabase Dashboard.
- Enter your project details.
- Wait for the new database to launch.
To create a database table, you'll need to sign in to the app dashboard using your GitHub account and create your organization and a new project within it. Then, you can click on the SQL Editor icon on your dashboard and click New Query to enter the SQL query that will create the workout table.
Take a look at this: Query in Nextjs
Database Setup
To start building with Next.js and Supabase, you need to set up your database.
Create a new project in the Supabase Dashboard by following these steps: create a new project, enter your project details, and wait for the new database to launch.
You can use the "User Management Starter" quickstart in the SQL Editor to set up your database schema, or copy and paste the SQL from the Supabase documentation and run it yourself.
To connect Next.js with your Supabase database, you'll need your Project URL and Anon Key, which can be found on your database dashboard.
Create a .env.local file in the root of your project and include your URL and keys in the file. Don't forget to include .env.local in your gitignore file to prevent it from being pushed to the GitHub repo.
Here are the environment variables you'll need to set up:
Now that you have your environment variables set up, you can create a Supabase client file by creating a file called supabase.js at the root of your project. Inside the supabase.js file, import the createClient function from Supabase and create a variable called supabase, passing in your parameters: URL (supabaseUrl) and Anon Key (supabaseKey).
Authentication
Authentication is a breeze with Supabase and Next.js. Supabase enables multiple authentication mechanisms, including username & password, Magic email link, and integration with popular services like Google, Facebook, Apple, GitHub, Twitter, Azure, GitLab, and Bitbucket.
You can choose from a variety of authentication providers, listed below:
- Username & password
- Magic email link
- Apple
- GitHub
- Azure
- GitLab
- Bitbucket
For Next.js, Supabase offers the @supabase/ssr package for Server-Side Auth, which makes it easy to configure your project to use cookies for storing user sessions.
For more insights, see: Next Js Drizzle Supabase
Server-Side Auth
Server-Side Auth is a crucial aspect of authentication, and Supabase makes it easy to integrate with popular frameworks like Next.js. The @supabase/ssr package is specifically designed for Server-Side Auth, providing all the necessary functionalities to configure user sessions using cookies.
To get started, you'll need to install the package for Next.js. Once installed, you can proceed with configuring your Supabase project to use cookies for storing user sessions. For more information, check out the Next.js Server-Side Auth guide.
Broaden your view: Nextjs Server Only
Supabase's Server-Side Auth allows for quick configuration of user sessions, making it a great choice for developers looking to streamline their authentication process. With Supabase, you can easily implement row-level security policies directly from the built-in SQL editor.
Here are some of the authentication mechanisms supported by Supabase:
- Username & password
- Magic email link
- Apple
- GitHub
- Azure
- GitLab
- Bitbucket
Supabase's Server-Side Auth also enables you to implement fine-grained access controls, making it easy to configure authorization and data access patterns. With its built-in SQL editor, you can implement row-level security policies to control access to your data.
Auth Confirmation Path Update
To update the Auth confirmation path, you need to change the Confirm signup email template to use a token hash. Specifically, you should replace {{ .ConfirmationURL }} with {{ .SiteURL }}/auth/confirm?token_hash={{ .TokenHash }}&type=signup.
You'll also need to make the same change in the Magic Link tab, but set the type to magiclink. This will allow you to use the token hash for verification.
For more insights, see: Why Use Next Js
To process the GET request for email OTP verification, you'll need to create a route handler in app/auth/confirm/route.ts. This handler extracts the token_hash, type, and next parameters from the request URL.
If both token_hash and type are present, it creates a Supabase client and attempts to verify the OTP. If the verification is successful, it redirects the user to the next URL, removing the token_hash and type parameters.
For another approach, see: Routes in Nextjs
App Development
Building an app with Next.js and Supabase is a breeze, thanks to the powerful combination of back end services and easy-to-use client-side libraries and SDKs provided by Supabase. This end-to-end solution lets you build individual features and services on the back end and integrate them seamlessly on the front end.
Supabase is also open source, giving you the option to self-host or deploy your backend as a managed service, and you can get started with a free tier that doesn't require a credit card. I've had experience building full-stack apps, including leading the Front End Web and Mobile Developer Advocacy team at AWS and writing a book on building these types of apps.
To get started with building your app, you'll need to update the app to implement basic navigation and layout styling, and configure logic to check if the user is signed in. Here are some of the key features you can expect from Supabase, including row-level security, real-time database, Supabase UI, user authentication, and edge functions.
Building an App
Building an app can be a daunting task, but with the right tools and approach, it can be a breeze. Supabase is a serverless, open-source alternative to Firebase built on top of the PostgreSQL database, providing all the backend services needed to create a full-stack application.
To get started, you'll need to update your app to implement basic navigation and layout styling, as well as configure some logic to check if the user is signed in and show a link for creating new posts if they are. This can be done by adding code to your _app.js file.
Next, you'll need to fetch and render a list of posts, which can be achieved by updating your index.js file to include a query for and rendering of the list of posts. This will allow users to view all the posts in your app.
Supabase comes with a range of features that make full-stack application development easier, including row-level security (RLS), a real-time database, and user authentication. These features can be used to restrict rows in your database tables, listen to real-time changes, and authenticate users securely.
You can also use Supabase's edge functions to perform tasks such as integrating with third parties or listening for WebHooks. These functions are TypeScript functions distributed globally at the edge, close to users, making them ideal for tasks that require low latency.
Here are some of the key features of Supabase's user authentication system:
- Email and password authentication
- Magic links for secure sign-in
- Google and GitHub authentication
- Automatic user ID and assignment of user ID
By using Supabase, you can create a full-stack application with a robust backend and easy-to-use client-side libraries and SDKs. This will allow you to focus on building the features and services that matter most to your users, without worrying about the underlying infrastructure.
Creating a User Profile Page
Creating a user profile page is a crucial step in app development. To start, you'll need to create a new file named profile.js in the pages directory.
The profile page uses the Auth component from the Supabase UI library. This component is essential for rendering a "sign up" and "sign in" form for unauthenticated users.
For authenticated users, the profile page will display a basic user profile with a "sign out" button. This feature is enabled by the magic sign in link.
You'll need to add the necessary code to the profile.js file to bring your user profile page to life.
Utilities and Middleware
To create a solid foundation for your Next.js Supabase project, you'll need to set up some essential utilities and middleware.
You should create two files: `client.js` and `server.js`, which will handle client-side and server-side Supabase access, respectively. These files should be organized within the `utils/supabase` directory at the project root.
To ensure seamless authentication, you'll need to implement middleware that refreshes expired Auth tokens and stores them securely. This involves refreshing the Auth token with `supabase.auth.getUser()`, passing the refreshed token to Server Components through `request.cookies.set`, and passing it to the browser with `response.cookies.set`. Be sure to only run this middleware on routes that access Supabase.
Here's a brief overview of the middleware setup:
- Refresh the Auth token with `supabase.auth.getUser()`
- Pass the refreshed token to Server Components with `request.cookies.set`
- Pass the refreshed token to the browser with `response.cookies.set`
Obtain API Keys
To obtain API keys, you first need to access the API settings page in your dashboard.
Go to the API Settings page in the dashboard to find your Project URL and anon key.
You'll find your Project URL, anon, and service_role keys on this page, so take note of them.
Create a .env.local file at the root of your project to save your API URL and anon key.
Paste the API URL and the anon key you copied from the API settings page into this file.
Here's a quick rundown of what you need to copy from the API settings page:
- Project URL
- anon key
- service_role key
Utilities
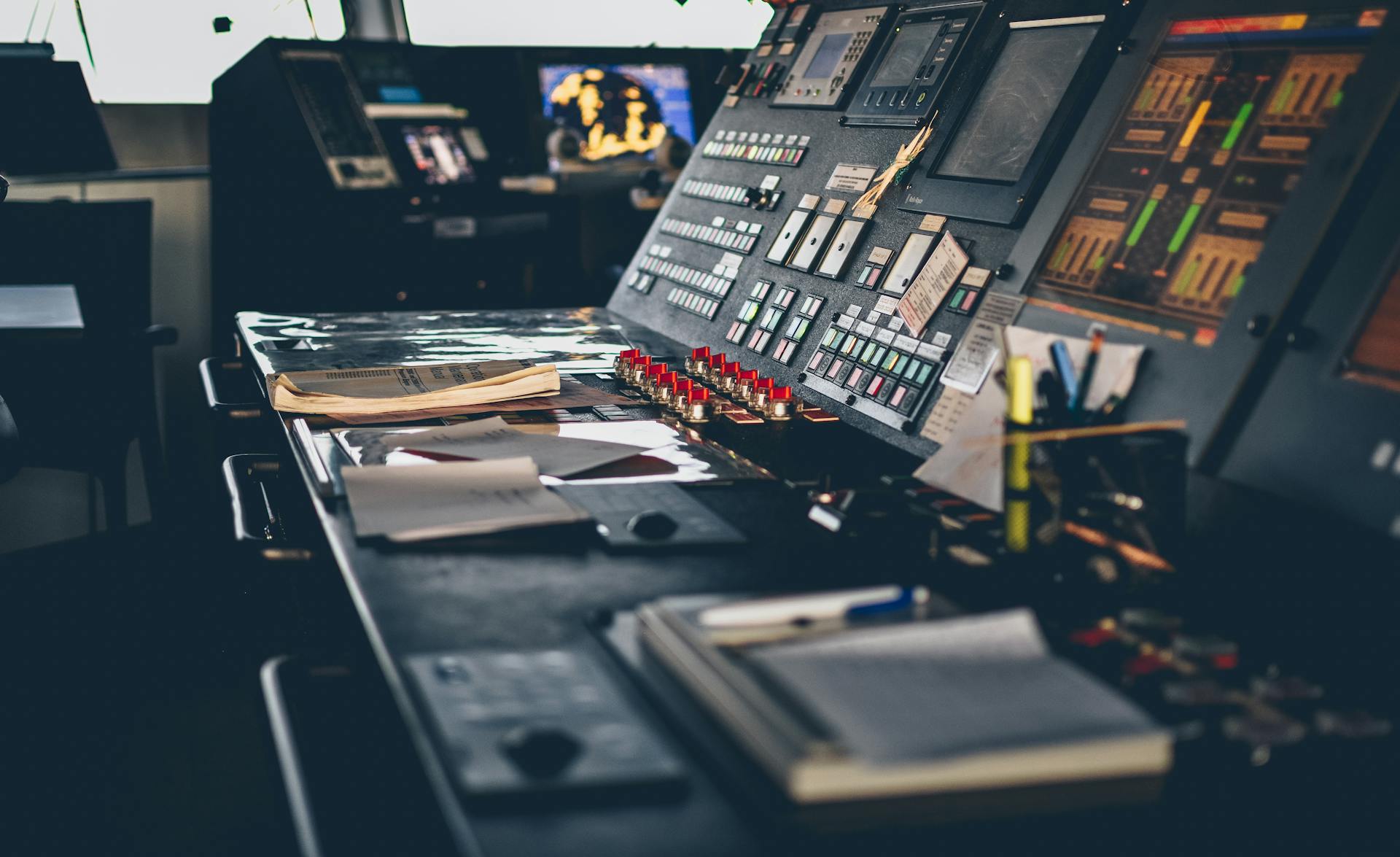
Utilities are an essential part of any application, providing a way to reuse code and make your life as a developer easier.
To get started with Supabase utilities, you'll want to create a client.js and a server.js file, which should be organized within the utils/supabase directory at the root of your project. This will allow you to access Supabase from Client Components, which run in the browser, and Server Components, Server Actions, and Route Handlers, which run only on the server.
These utilities will be used to create clients for client-side and server-side Supabase functionality.
You'll need to install the core Supabase and Supabase Server Side Rendering (in beta) packages to create the util function for obtaining the Supabase client in your server components.
Here are the essential utilities files you'll need to create:
- client.js: To access Supabase from Client Components.
- server.js: To access Supabase from Server Components, Server Actions, and Route Handlers.
These files will be used to initialize a Supabase client for server-side rendering in a Next.js application, using environment variables for the Supabase URL and anonymous key, and managing cookies for authentication and session handling.
Middleware
Middleware is a crucial part of any Next.js application, and it's essential to understand how it works. You need middleware to refresh expired Auth tokens and store them, especially since Server Components can't write cookies.
To accomplish this, you'll need to create a middleware.js file at the project root, which will contain the logic for updating the session. This file should be created in addition to another one within the utils/supabase folder.
The middleware.js file will use the supabase.auth.getUser() function to refresh the Auth token and then pass it to Server Components through request.cookies.set. This ensures that the token is updated and can be accessed by the browser.
You can use a matcher to run the middleware only on routes that access Supabase. This is a good practice to avoid unnecessary computations. For more information on matchers, check out the documentation.
Here are the steps to create the middleware:
1. Create a middleware.js file at the project root.
2. Create another middleware.js file within the utils/supabase folder.
3. Use the supabase.auth.getUser() function to refresh the Auth token.
4. Pass the refreshed token to Server Components through request.cookies.set.
5. Use a matcher to run the middleware only on routes that access Supabase.
By following these steps, you'll ensure that your application is properly configured to handle Auth tokens and protect user data. Always use supabase.auth.getUser() to protect pages and user data, and never trust supabase.auth.getSession() inside server code.
Configuring SMTP
Configuring SMTP is a crucial step in setting up your email services. You'll need to paste your API key into the Password field.
The Username for SMTP settings should be set to "apikey". This is a specific detail that's worth noting.
Set the Host to smtp.sendgrid.net, and the Port number to 587. This combination is required for a successful setup.
For Sender details, use the same email address you used to configure your Single Sender identity. This ensures consistency and prevents any issues.
Once you've entered all the required information, click Save to complete the configuration.
Sources
- https://supabase.com/docs/guides/getting-started/tutorials/with-nextjs
- https://blog.logrocket.com/build-full-stack-app-next-js-supabase/
- https://egghead.io/lessons/supabase-query-data-from-supabase-using-next-js
- https://www.freecodecamp.org/news/the-complete-guide-to-full-stack-development-with-supabas/
- https://akoskm.com/how-to-build-a-simple-magic-link-sign-in-with-nextjs-supabase-and-sendgrid/
Featured Images: pexels.com