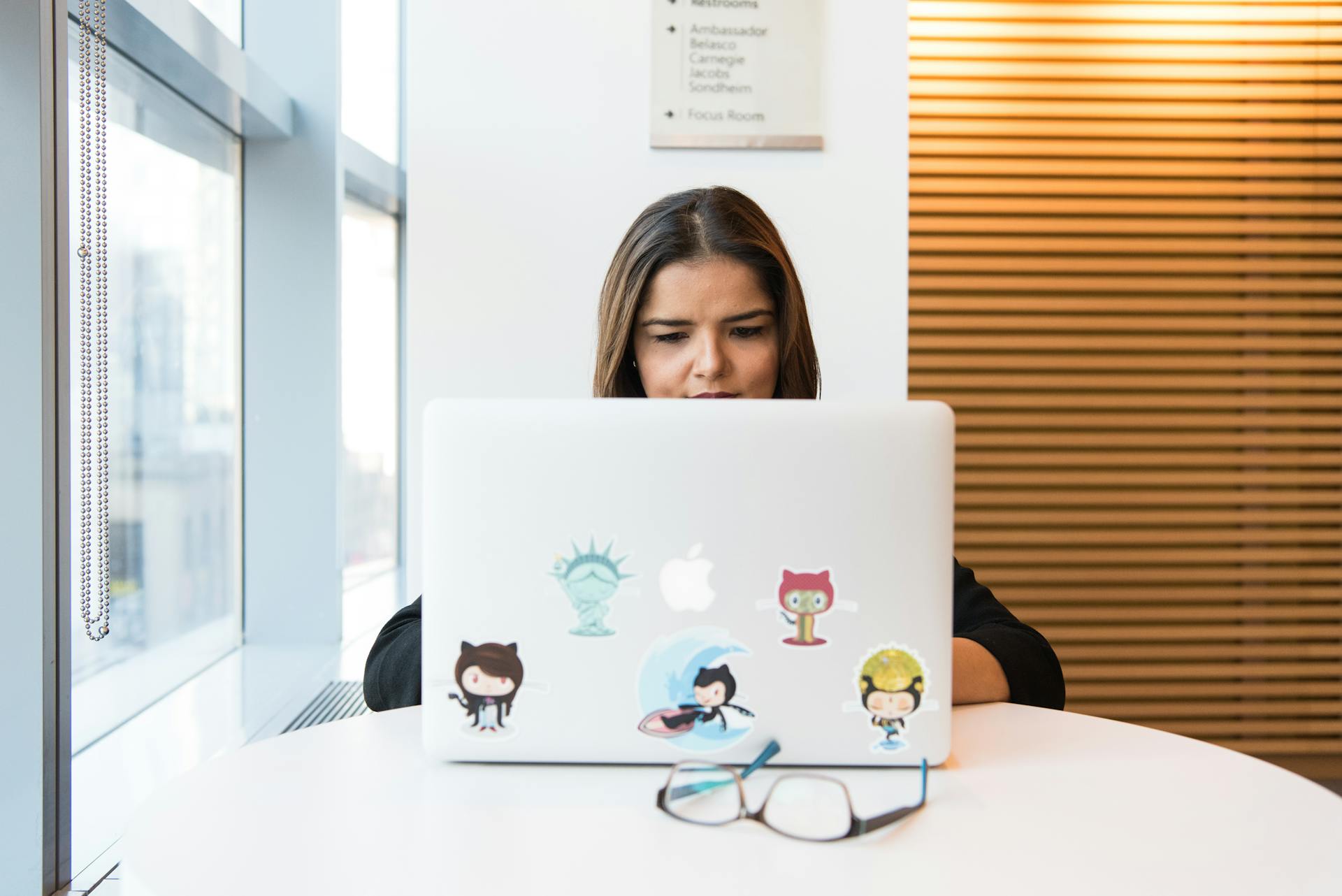
Next.js and SvelteKit are two popular frameworks for building fast, scalable, and maintainable web applications. Next.js boasts a large community and a wide range of features, including built-in support for internationalization and server-side rendering.
SvelteKit, on the other hand, focuses on simplicity and ease of use, with a smaller but still significant community. It's built on top of the Svelte compiler, which allows for efficient and fast rendering of components.
One key difference between the two frameworks is their approach to rendering. Next.js uses server-side rendering (SSR) by default, which can improve SEO and page load times. SvelteKit, by contrast, uses a hybrid approach that combines client-side and server-side rendering.
In terms of performance, both frameworks are capable of producing fast and efficient applications. However, Next.js has a slight edge when it comes to initial page load times, thanks to its built-in support for static site generation (SSG).
What Is Next.js and SvelteKit?
SvelteKit is a framework built on top of Svelte, providing a comprehensive suite of tools and features for building, deploying, and scaling web applications.
It's known for compiling components to highly optimized JavaScript code during the build process, making it faster and more efficient than other frameworks.
SvelteKit takes this approach one step further by providing a complete end-to-end solution for building and deploying web applications, allowing developers to focus on building and refining their web applications without worrying about underlying infrastructure or deployment processes.
Some notable sites made with SvelteKit include Svelte.dev, nytimes.com, and daisyui.com.
What Is Svelte?
Svelte is a JavaScript library created by the same team behind SvelteKit. It's known for its approach to building web applications by compiling components to highly optimized JavaScript code during the build process.
SvelteKit is built on top of Svelte and provides a comprehensive suite of tools and features for building, deploying, and scaling web applications.
SvelteKit takes this one step further by providing a complete end-to-end solution for building and deploying web applications. This means you can focus on building and refining your web applications without having to worry about underlying infrastructure or deployment processes.
Here are some notable sites made with SvelteKit:
- Svelte.dev
- nytimes.com
- daisyui.com
What Is Next.js?
Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
It was created by Guillermo Rauch in 2016 and has since become a widely-used tool in the web development community.
One of its key features is automatic code splitting, which allows developers to split their code into smaller chunks, making it easier to manage and load only the necessary code for each page.
Next.js also provides built-in support for internationalization (i18n) and accessibility features, making it a great choice for building global and inclusive applications.
Developers can also use Next.js to create API routes and server-side rendering, giving them more control over the rendering of their application.
Next.js has a large and active community, with a wide range of resources and plugins available to help developers get started and stay up-to-date with the latest features.
Key Features and Architecture
SvelteKit and Next.js are two popular frameworks for building web applications. SvelteKit excels at swift deployment, internationalization support, and efficient image handling, resulting in straightforward, high-performance code.
Next.js, on the other hand, is tailored for React developers and includes extensive tooling and configurations. It's particularly excellent in client-side routing for dynamic content management.
Here are some key features of Next.js:
- Server-Side Rendering: Generates HTML on the server for each request, improving SEO and initial page load performance.
- Static Site Generation: Creates static HTML files at build time, perfect for content-heavy sites that don't change frequently.
- Incremental Static Regeneration (ISR): Allows you to update static content after the initial build without needing a full rebuild, combining the benefits of static and dynamic sites.
- Automatic Code Splitting: Intelligently splits your code into smaller chunks, loading only what's necessary for each page.
- Built-in Routing: Provides an intuitive file-system based routing, simplifying navigation in your app.
- Internationalization (i18n): Built-in support for internationalized routing and language detection, making it easier to build multilingual applications.
- API Routes: Allows you to build your API endpoints as part of your Next.js app, streamlining back end integration.
Features and Architecture
SvelteKit and Next.js are two popular frameworks for building web applications, each with its unique strengths and features.
SvelteKit focuses on swift deployment, internationalization support, and efficient image handling, resulting in straightforward, high-performance code.
SvelteKit follows a component-based architecture, where each piece of UI is represented as a self-contained component, making it easy to manage and maintain your application.
A key benefit of this architecture is that you can reuse components across your application, streamlining development and reducing code duplication.
Here are some key features of Next.js:
- Server-Side Rendering: Generates HTML on the server for each request, improving SEO and initial page load performance.
- Static Site Generation: Creates static HTML files at build time, perfect for content-heavy sites that don't change frequently.
- Incremental Static Regeneration (ISR): Allows you to update static content after the initial build without needing a full rebuild.
- Automatic Code Splitting: Intelligently splits your code into smaller chunks, loading only what's necessary for each page.
- Built-in Routing: Provides an intuitive file-system based routing, simplifying navigation in your app.
- Internationalization (i18n): Built-in support for internationalized routing and language detection, making it easier to build multilingual applications.
- API Routes: Allows you to build your API endpoints as part of your Next.js app, streamlining back end integration.
In contrast, SvelteKit excels at efficient image handling and internationalization support, making it a great choice for applications that require these features.
Routing
Routing is a crucial aspect of any web application, and both Next.js and SvelteKit offer robust solutions. Next.js stands out with its advanced client-side routing, ideal for dynamically managed content.
With Next.js, you can create routes that are automatically handled by the framework, making development easier for projects with stable content needs. For example, you can use file-based routing to define routes for your application.
SvelteKit, on the other hand, focuses on simplicity and primarily supports static pre-rendering. However, it also handles dynamic routes effectively, making it a great choice for projects with stable content needs.
Here's a comparison of the routing systems in Next.js and SvelteKit:
In SvelteKit, you can define routes using a routes object that maps URL segments to components. For example, you can use the following code to define routes:
```javascript
routes: {
'/': { component: Home },
'/about': { component: About },
'/[slug]': { component: BlogPost }
}
```
This code defines three routes: the root URL, the about URL, and a dynamic route for blog posts. The `[slug]` syntax indicates that the route should accept a dynamic value, which is passed to the `BlogPost` component as a prop.
State Management Solutions
SvelteKit simplifies state management using Svelte's integrated store system, providing a more unified approach across all components. This approach is in contrast to Next.js, which offers greater flexibility by allowing developers to choose from various state management libraries.
Next.js uses the React framework, giving you access to popular state management tools like Redux and MobX. You can manage state in Next.js using the useState hook, making it a solid choice for projects that require robust state management.
SvelteKit, on the other hand, does not have a built-in state management solution. Instead, you can use the Svelte store to manage state or opt for a third-party library like XState or Recoil.
Here's a comparison of state management solutions in Next.js and SvelteKit:
Both frameworks offer robust solutions, making your choice dependent on the specific needs of your project.
Server-Side Rendering Techniques
Server-side rendering is a powerful technique that allows for faster initial load times and better SEO. Both Next.js and SvelteKit robustly support server-side rendering.
Next.js uses server-side rendering to improve SEO through effective code splitting and lazy loading, beneficial for larger projects. This approach generates HTML on the server and sends it to the client, resulting in a fast and optimized first-load experience.
SvelteKit, on the other hand, opts for client-side JavaScript rendering for speedier interactions. However, it still provides built-in server-side rendering, making it easy to generate HTML on the server and deliver it to the client.
Server-Side Rendering Techniques
Server-side rendering is a powerful technique that can greatly improve the performance and SEO of your application. SvelteKit and Next.js are two popular frameworks that robustly support server-side rendering.
SvelteKit uses client-side JavaScript rendering for speedier interactions, while Next.js uses server-side rendering to improve SEO through effective code splitting and lazy loading. This is beneficial for larger projects that require fast and optimized performance.
Server-side rendering generates HTML on the server and sends it to the client, providing a fast and optimized first-load experience. This is particularly useful for applications that require seamless navigation between pages.
The Link component in Next.js handles navigation between pages in the application, making it easy to create a seamless user experience. This allows users to click on links and be taken to the desired page without any delays.
SvelteKit provides built-in server-side rendering, making it easy to generate HTML on the server and deliver it to the client. This results in faster initial load times and better SEO, which is essential for any application that wants to rank high in search engine results.
By using server-side rendering, you can improve the performance and SEO of your application, making it more appealing to users and search engines alike.
Static Site Generation
Static site generation is a powerful technique that allows you to build fast, lightweight, and scalable websites that can be easily hosted and served from a CDN.
With SvelteKit, you can build your application for production with a single command. This command prepares your site for deployment.
SvelteKit also supports static site generation, which exports your application as a set of static files that can be easily hosted and served from a CDN. This process involves two commands: one to build your application for production, and another to export it as static files.
Tools and Ecosystem
Next.js and SvelteKit both offer robust tools and ecosystems to support complex applications. Next.js provides a rich selection of tools and libraries, ideal for data-heavy applications.
SvelteKit, on the other hand, offers strong foundational support and flexibility for content-driven projects. Notably, it's backed by Vercel and Render, which can be a significant advantage for developers.
Next.js excels in providing a wide range of tools and libraries, making it a top choice for developers building complex, data-heavy applications.
Automatic Code Splitting
Automatic Code Splitting is a feature of Next.js that allows you to split your code into smaller chunks. This helps keep your initial load times fast, even as your application grows in size.
The Head component is used to set the title of the page, which is an important aspect of search engine optimization (SEO). It's used to ensure the page's title is set correctly, even when the page is dynamically generated on the server.
Using Automatic Code Splitting, you only load the parts of your application that are needed. This makes your application more efficient and faster to load.
Tools and Ecosystem
Next.js provides developers a rich selection of tools and libraries ideal for complex, data-heavy applications.
Within the React ecosystem, Next.js stands out for its robust support of complex applications, leveraging the strengths of React to deliver high-performance results.
SvelteKit, on the other hand, offers strong foundational support and flexibility, making it a top choice for content-driven projects.
Vercel and Render have notably backed SvelteKit, providing a solid foundation for developers to build and deploy content-driven applications.
The combination of Next.js and SvelteKit offers developers a versatile and powerful toolset, allowing them to choose the best solution for their project's specific needs.
Performance and Deployment
SvelteKit typically offers better raw performance, especially for smaller to medium-sized applications, due to its compile-time approach resulting in smaller bundle sizes and generally faster initial load times and runtime performance.
SvelteKit's philosophy of compilation over interpretation results in smaller and faster applications compared to those built with Next.js, which can load only the needed code, leading to faster load times and smaller app sizes.
Next.js, on the other hand, offers features that drastically improve load times and overall user experience, such as automatic code-splitting, which only loads the code necessary for each page, and the integration of Rust-based transpilation, which improves build times and makes it particularly beneficial for large projects.
The adoption of Rust for these tasks reflects a broader industry trend towards leveraging more performant languages in development toolchains to enhance developer experience and application performance.
Performance Analysis
SvelteKit's compile-time approach results in smaller bundle sizes, making it a great choice for smaller to medium-sized applications.
SvelteKit's philosophy of compilation over interpretation leads to faster load times and smaller app sizes compared to Next.js.
Next.js offers automatic code-splitting, which only loads the code necessary for each page, significantly improving load times and user experience.
The adoption of Rust for building and bundling applications in Next.js version 12 has improved build times, making it particularly beneficial for large projects with complex dependencies.
Rust-based transpilation, part of the Turbopack bundler, replaces traditional JavaScript-based tooling and enhances developer experience and application performance.
SvelteKit typically offers better raw performance, especially for smaller to medium-sized applications, thanks to its excellent performance optimizations out of the box.
Image optimization and automatic code splitting are also key features of SvelteKit that contribute to its performance advantages.
Next.js's Rust-based transpiler improves build times compared to older JavaScript bundlers like Webpack, making it a significant advancement in application performance.
Deployment and Scalability
Next.js offers seamless deployment with Vercel, a platform created by the same team, making it a convenient option for developers.
Having a well-established pattern for building and scaling large applications is crucial, and Next.js has this covered.
Next.js can be deployed to various platforms, thanks to its adapter-based system for different deployment targets.
The wide range of deployment options available with Next.js is a significant advantage over other frameworks.
Less established patterns for large-scale applications are a drawback for some frameworks, but not Next.js.
Real-World Applications and Support
SvelteKit and Next.js are both popular JavaScript frameworks, but they have different strengths and weaknesses. SvelteKit has a more tailored fit for projects requiring a specific setup, as seen in real-world applications developed using this technology.
Next.js, on the other hand, has the advantage of being backed by Vercel, which offers tailored support plans for enterprises requiring guaranteed uptime and dedicated service. These plans are particularly advantageous for businesses that need a high level of support.
Both frameworks showcase regular updates and consistent communication from the core team, indicating a strong commitment to their development and support.
Real-World Applications
SvelteKit and Next.js are two leading JavaScript frameworks that have been used in real-world applications to deliver impressive performance and results.
SvelteKit has been used in projects that require a tailored fit for different project requirements, such as the one mentioned in the article section, where it was used to develop a specific application.
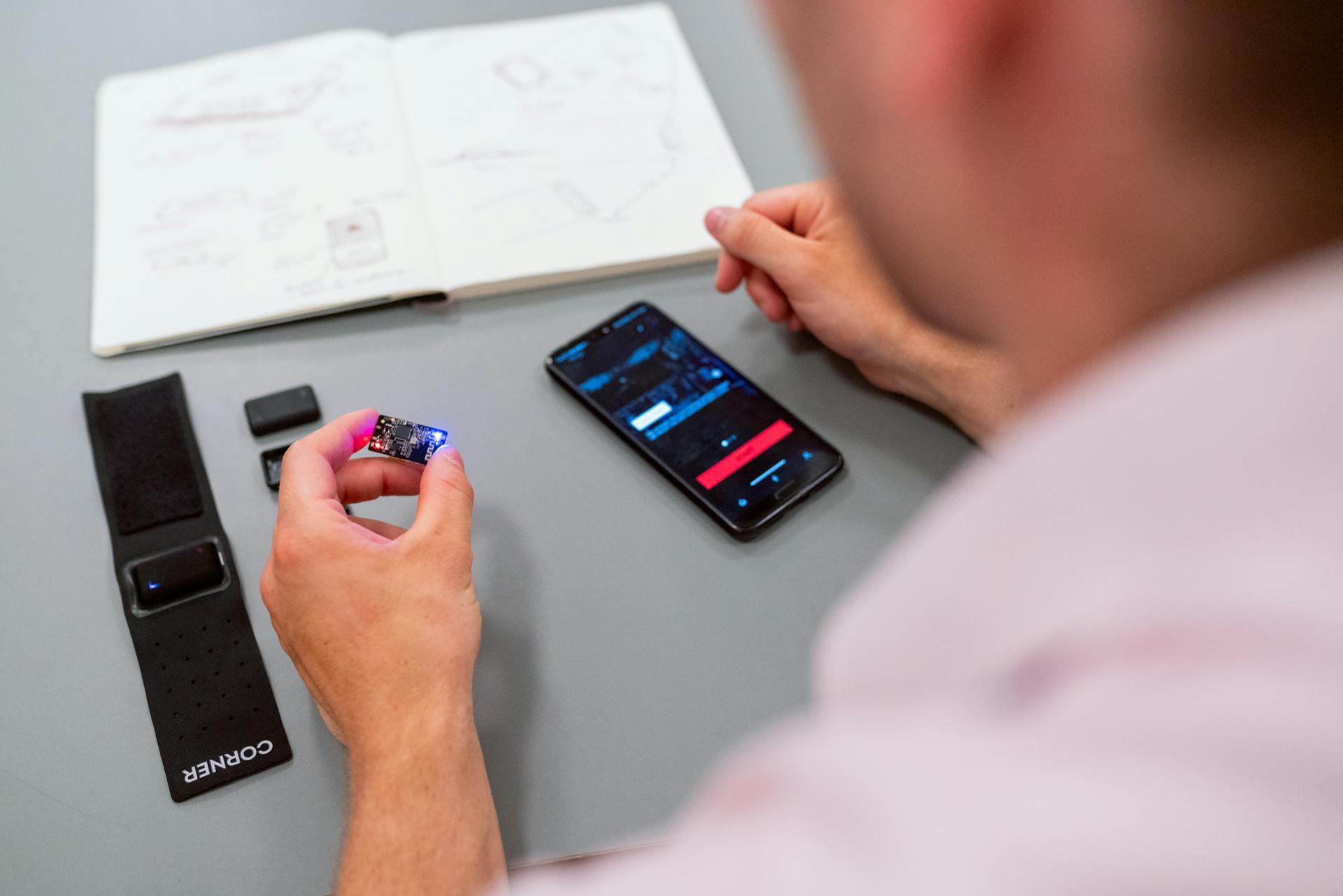
The practical use of SvelteKit and Next.js can be understood through real-world applications and case studies, which provide valuable insights into the strengths and weaknesses of each framework.
One of the key benefits of using SvelteKit is its ability to deliver fast and efficient results, as seen in the example mentioned in the article section.
Next.js, on the other hand, has been used in projects that require a high level of performance and scalability, such as the ones powered by Next.js mentioned in the article section.
SvelteKit and Next.js are both popular choices among developers due to their ease of use and flexibility, making them a great fit for a wide range of projects and applications.
Developers can learn from real-world applications and case studies of SvelteKit and Next.js to gain a deeper understanding of how to use these frameworks to achieve their goals.
Commercial Support and Official Team Assistance
Commercial support can be a game-changer for businesses, and Next.js has a clear advantage here with its backing by Vercel.
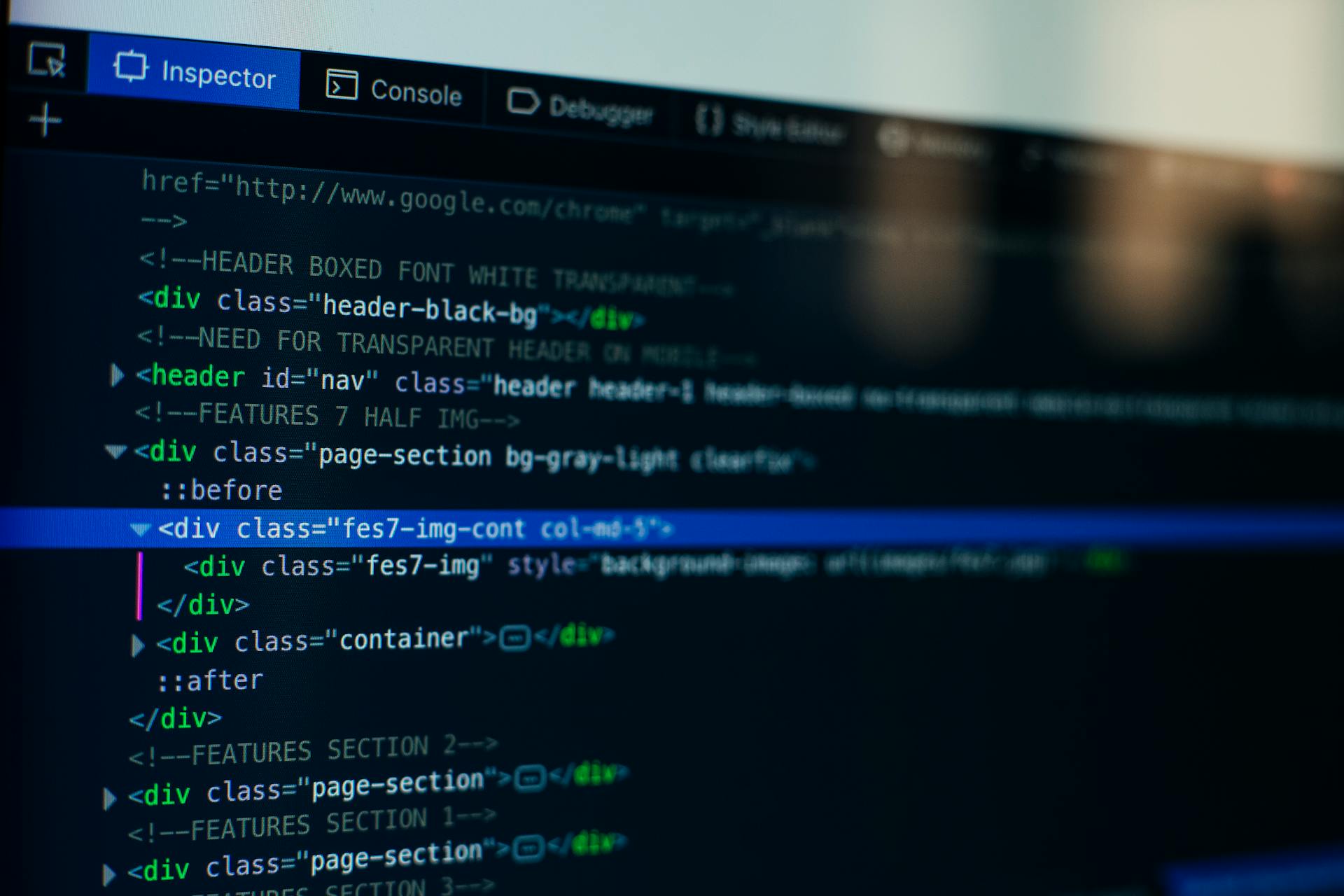
Vercel offers tailored support plans that are particularly beneficial for enterprises requiring guaranteed uptime and dedicated service.
SvelteKit, on the other hand, doesn't offer structured commercial support plans, but its creators and core developers are very active in community forums.
This means SvelteKit users can get hands-on assistance and be part of a collaborative environment that's fostered by the core team.
Both Next.js and SvelteKit have regular updates and consistent communication from their core teams, which is a big plus for developers who want to stay up-to-date with the latest developments.
As the communities around these frameworks grow and evolve, the support and resources available are also shifting, making it an exciting time to be part of these ecosystems.
Frequently Asked Questions
Is SvelteKit worth it?
SvelteKit is worth it if your team is already familiar with Svelte, offering a seamless developer experience with minimal code. It's also a great choice for those seeking a similar feature set to Next.js with a more streamlined approach
Why use SvelteKit over Svelte?
SvelteKit offers enhanced SEO and initial load performance for complex applications, building on Svelte's efficient JavaScript output. This makes SvelteKit a great choice for larger, more demanding projects
What are the cons of SvelteKit?
SvelteKit has a relatively short history and evolving tooling, which may impact its stability and compatibility. Additionally, it may require some adjustment for developers familiar with other frameworks due to its unique learning curve.
Featured Images: pexels.com