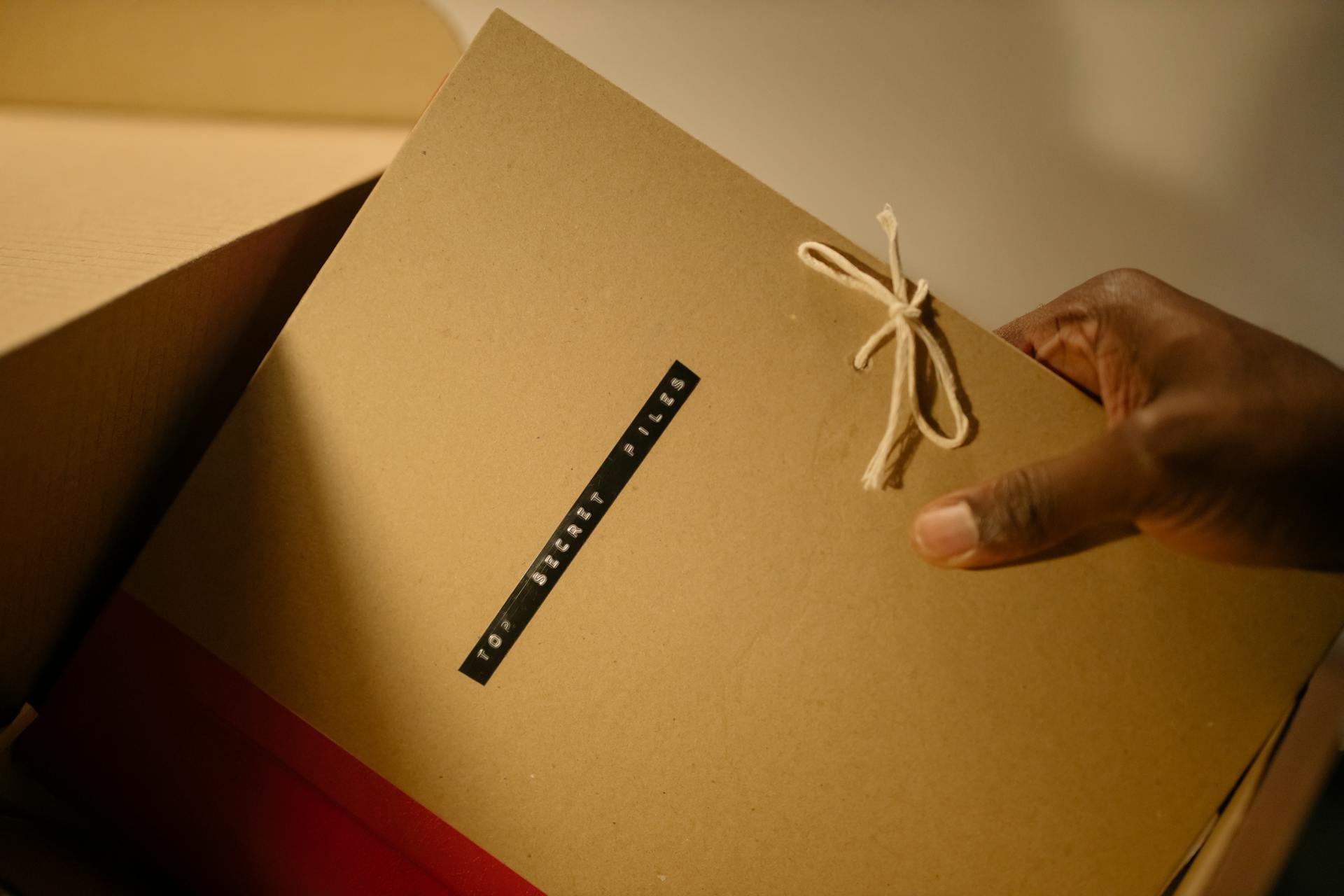
Uploading files to OneDrive using the API can be a bit tricky, but with the Microsoft Graph and CSOM, it becomes a breeze.
The Microsoft Graph is a RESTful API that provides a unified programmability model for accessing the entire Microsoft cloud ecosystem, including OneDrive.
To use the Microsoft Graph, you'll need to register your application and obtain a client ID and client secret, which you'll use to authenticate your API requests.
Authentication is a crucial step in uploading files to OneDrive, as it ensures that your API requests are authorized and secure.
If this caught your attention, see: Google Drive Files Stuck Uploading
Prerequisites
To get started with the OneDrive API upload file process, you'll need to meet some basic prerequisites.
First, you'll need to have a Microsoft account with OneDrive. This is the foundation of the process, and without it, you won't be able to access the API.
To access the OneDrive API, you'll need to register an application in the Azure Portal. This will give you the necessary credentials to make API calls.
If this caught your attention, see: How Do I Access Amazon Cloud Drive
You'll also need Node.js installed on your system, as it's required for making API calls to Microsoft's Graph API.
To make API calls, you'll need to install axios, a library that simplifies the process of making HTTP requests.
Here's a quick rundown of the prerequisites:
- A Microsoft account with OneDrive
- An application registered in Azure Portal for OneDrive API access
- Node.js installed on your system
- Install axios to make API calls to Microsoft’s Graph API
Creating a Session
To create an upload session, your app must first request a new upload session, which creates a temporary storage location for the file's bytes until the upload is complete. This is essential for large file uploads.
To initiate the process, specify the ID or path of the parent folder if you're uploading a new file, or the ID or path of the file to update if you're modifying an existing one. I've found that being clear about the file's origin helps avoid confusion later on.
The upload session is completed once the last byte of the file has been uploaded, at which point the final file appears in the destination folder.
Take a look at this: New Google Drive Shortcuts
OAuth 2.0 Authentication
OAuth 2.0 Authentication is a crucial step in creating a session, allowing you to securely access user data.
To start, you'll need to register your app in Azure and generate access and refresh tokens using the OAuth 2.0 flow. This involves using the Authorization URL to generate the first access and refresh tokens.
The Authorization URL is: https://login.microsoftonline.com/common/oauth2/v2.0/authorize
You'll need to replace YOUR_CLIENT_ID and YOUR_REDIRECT_URI with your actual values. Once the user signs in, the system will provide an authorization code.
To exchange the authorization code for access and refresh tokens, use the following POST request: POST https://login.microsoftonline.com/common/oauth2/v2.0/token
The request body should include client_id, client_secret, code, redirect_uri, grant_type, and scope.
Here's a summary of the required parameters:
- client_id: YOUR_CLIENT_ID
- client_secret: YOUR_CLIENT_SECRET
- code: AUTHORIZATION_CODE
- redirect_uri: YOUR_REDIRECT_URI
- grant_type: authorization_code
- scope: Files.ReadWrite.All offline_access
Store the access and refresh tokens in a secure location, such as environment variables like REACT_APP_ONEDRIVE_ACCESS_TOKEN and REACT_APP_ONEDRIVE_REFRESH_TOKEN.
Since OneDrive access tokens expire after 1 hour, you must refresh tokens to maintain long-term access. You can use the refresh token to get a new access token by sending a POST request to https://login.microsoftonline.com/common/oauth2/v2.0/token with the following parameters:
- client_id: YOUR_CLIENT_ID
- client_secret: YOUR_CLIENT_SECRET
- refresh_token: YOUR_REFRESH_TOKEN
- redirect_uri: YOUR_REDIRECT_URI
- grant_type: refresh_token
- scope: Files.ReadWrite.All offline_access
Create a Session
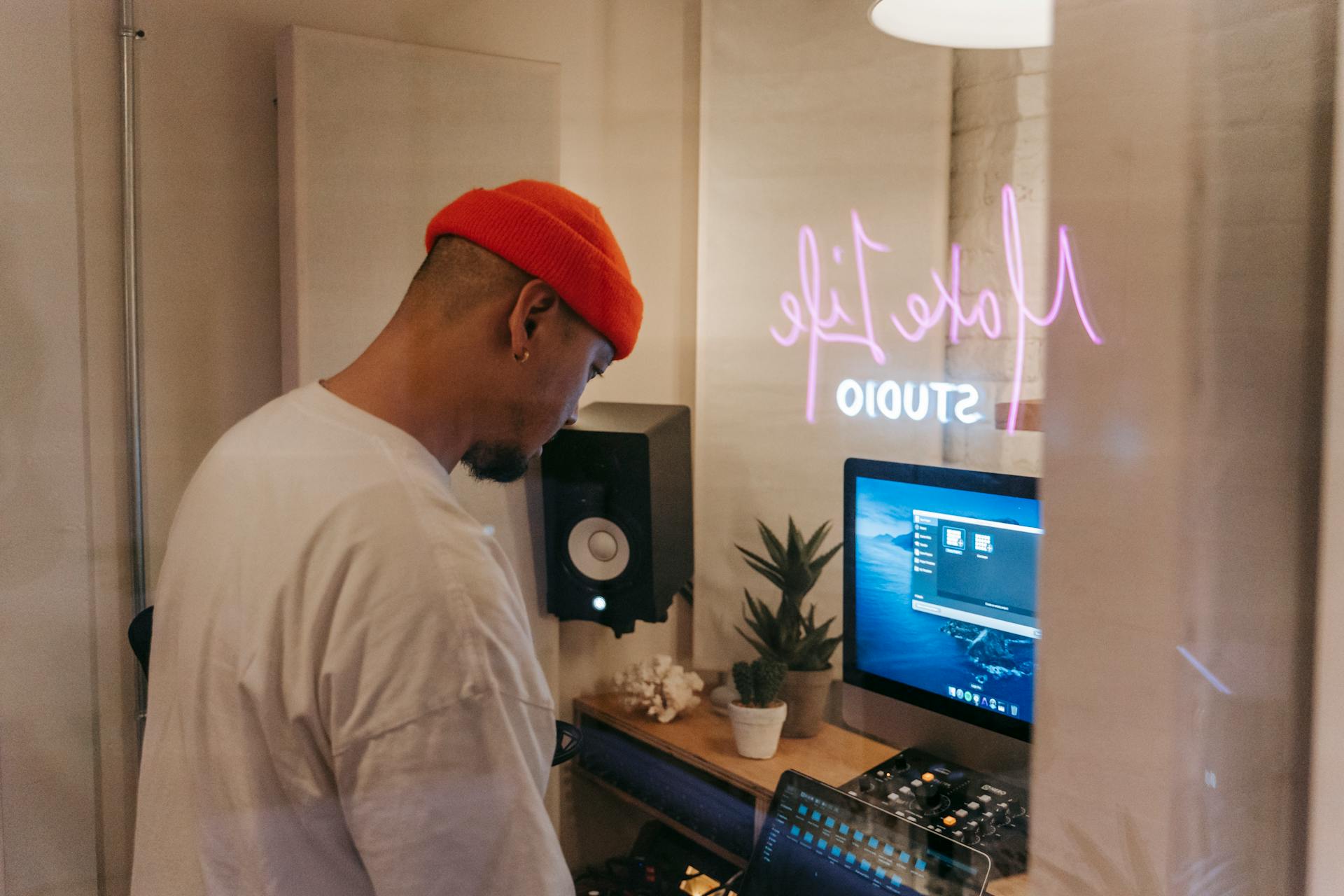
To create a session for file upload, your app must first request a new upload session. This creates a temporary storage location where the bytes of the file will be saved until the complete file is uploaded.
To initiate the process, specify the ID or path of the parent folder if you're uploading a new file. If you're updating an existing file, specify the ID or path of the file to update.
A new upload session can be created by making a request to the Graph API, which will provide the details of the newly created uploadSession, including the URL used for uploading the parts of the file.
Here's a summary of the required information for creating a new upload session:
Once you've created the upload session, you can start uploading the file or its parts.
Uploading a File
Uploading a file to OneDrive using the Graph API involves a few key steps.
To initiate the upload process, you'll need to send a PUT request to the Graph API endpoint.
The request body should contain the file data as binary content, which is sent in the body of the request.
You'll also need to pass the access token in the header, which is obtained through OneDrive authentication.
Here's an example of how to do this using the axios library:
```python
const uploadFileToOneDrive = async (path, fileContent) => {
const response = await axios.put(
`https://graph.microsoft.com/v1.0/me/drive/root:${path}:/content`,
fileContent,
{
headers: {
Authorization: `Bearer ${process.env.REACT_APP_ONEDRIVE_ACCESS_TOKEN}`,
'Content-Type': 'application/octet-stream',
},
}
);
return response.data;
};
```
If the file already exists on OneDrive, you may encounter a conflict. To replace the existing file, you can specify the conflict behavior property in the request body.
Here are some possible conflict behaviors you can use:
```markdown
- `replace`: Replaces the existing file with the new one.
- `fail`: Fails the upload process if the file already exists.
- `merge`: Merges the new file with the existing one.
```
Handling File Conflicts
Handling file conflicts is a crucial aspect of uploading files to OneDrive using the API. You can handle upload conflicts by using the @microsoft.graph.conflictBehavior header.
To recover from an upload error, your app can make a PUT request with a new driveItem resource that will be used when committing the upload session. This new request should correct the source of error that generated the original upload error.
See what others are reading: How to Upload to Google Drive from Android
You can indicate that your app is committing an existing upload session by including the @microsoft.graph.sourceUrl property with the value of your upload session URL in the PUT request. This allows OneDrive to preserve the upload session until the expiration time.
To replace files, use the PUT request with conflict behavior handling, as shown in the following example: Conflict Behavior: To replace a file, use the following request with conflict behavior handling: PUT https://graph.microsoft.com/v1.0/me/drive/root:/YOUR_PATH:/content?conflictBehavior=replace
Discover more: How to Use Google One Vpn
Resuming an In-Progress
Resuming an In-Progress Upload is a crucial aspect of Handling File Conflicts. If an upload request is disconnected or fails before the request is completed, all bytes in that request are ignored.
This can occur if the connection between your app and the service is dropped. If this happens, your app can still resume the file transfer from the previously completed fragment.
To find out which byte ranges have been received previously, your app can request the status of an upload session. This is done by sending a GET request to the uploadUrl.
The server will respond with a list of missing byte ranges that need to be uploaded and the expiration time for the upload session. This information allows your app to recover the upload session and resume the file transfer from the correct point.
Curious to learn more? Check out: How to Transfer Files from Onedrive to My Harddrive
Handling Conflicts
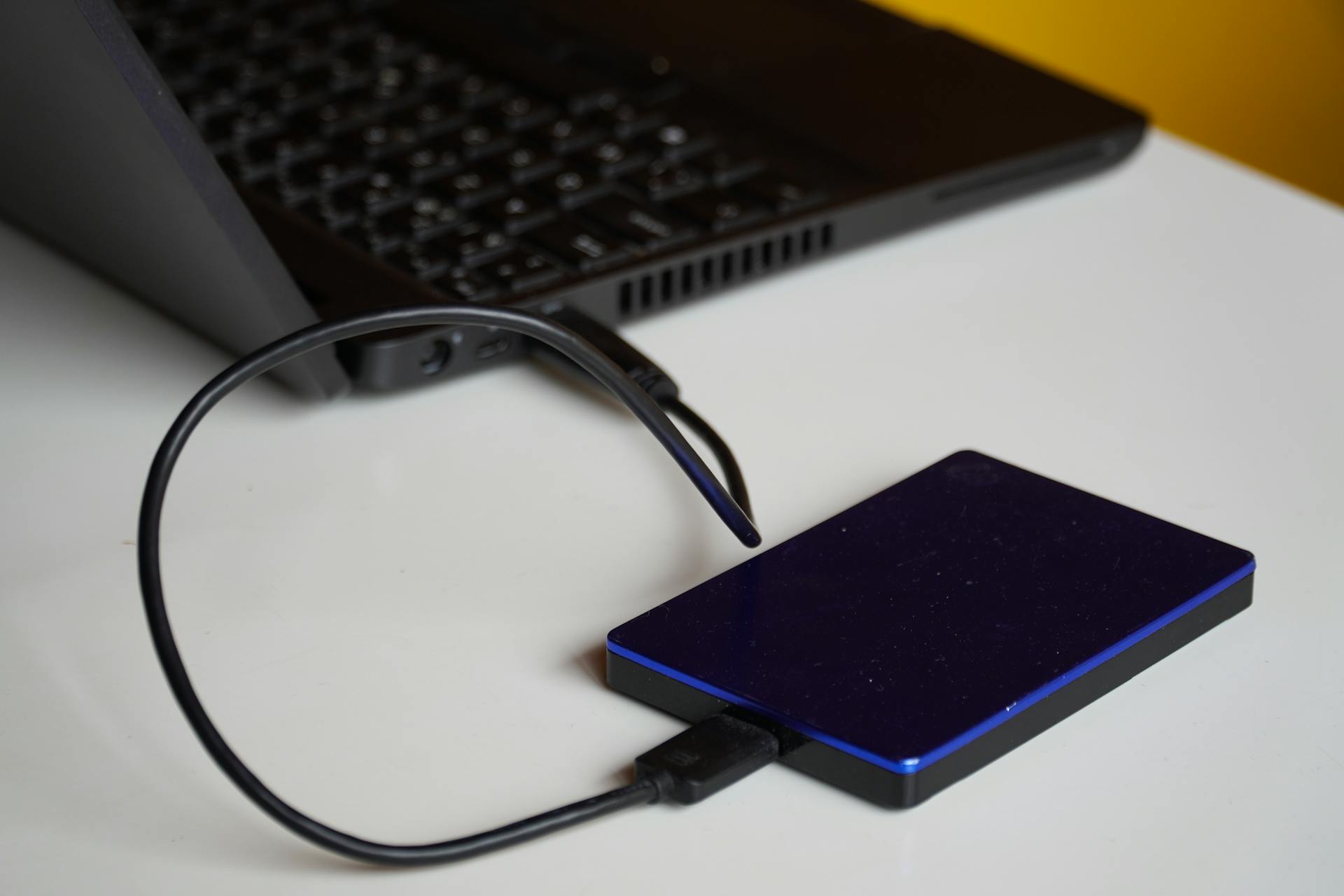
A conflict occurs when you try to upload a file with the same name as an existing item, or when the last byte range of a file upload fails due to a name conflict or quota limitation.
You can handle conflicts by using the @microsoft.graph.conflictBehavior header as expected in the upload call. This allows you to specify how you want to handle the conflict.
If a conflict occurs after the file is uploaded, an error is returned when the last byte range is uploaded. The upload session will be preserved until the expiration time, allowing your app to recover the upload by explicitly committing the upload session.
To explicitly commit the upload session, you must make a PUT request with a new driveItem resource that will correct the source of the error. This new request should include the @microsoft.graph.sourceUrl property with the value of your upload session URL.
You can replace files with the same ETag by using the following request with conflict behavior handling: PUT https://graph.microsoft.com/v1.0/me/drive/root:/YOUR_PATH:/content?conflictBehavior=replace
Here are some steps to handle ETag conflicts:
- Use the conflict behavior handling request to replace the file.
Sources
- https://medium.com/how-tos/how-to-upload-files-to-onedrive-or-sharepoint-using-microsoft-graph-api-and-csom-1d052b21e0a8
- https://learn.microsoft.com/en-us/onedrive/developer/rest-api/api/driveitem_createuploadsession
- https://www.office365clinic.com/2022/08/05/resumable-upload-of-large-files-to-onedrive/
- https://docs-snaplogic.atlassian.net/wiki/spaces/SD/pages/1356464309/OneDrive+-+Upload+File
- https://www.webdevstory.com/onedrive-integration-react/
Featured Images: pexels.com