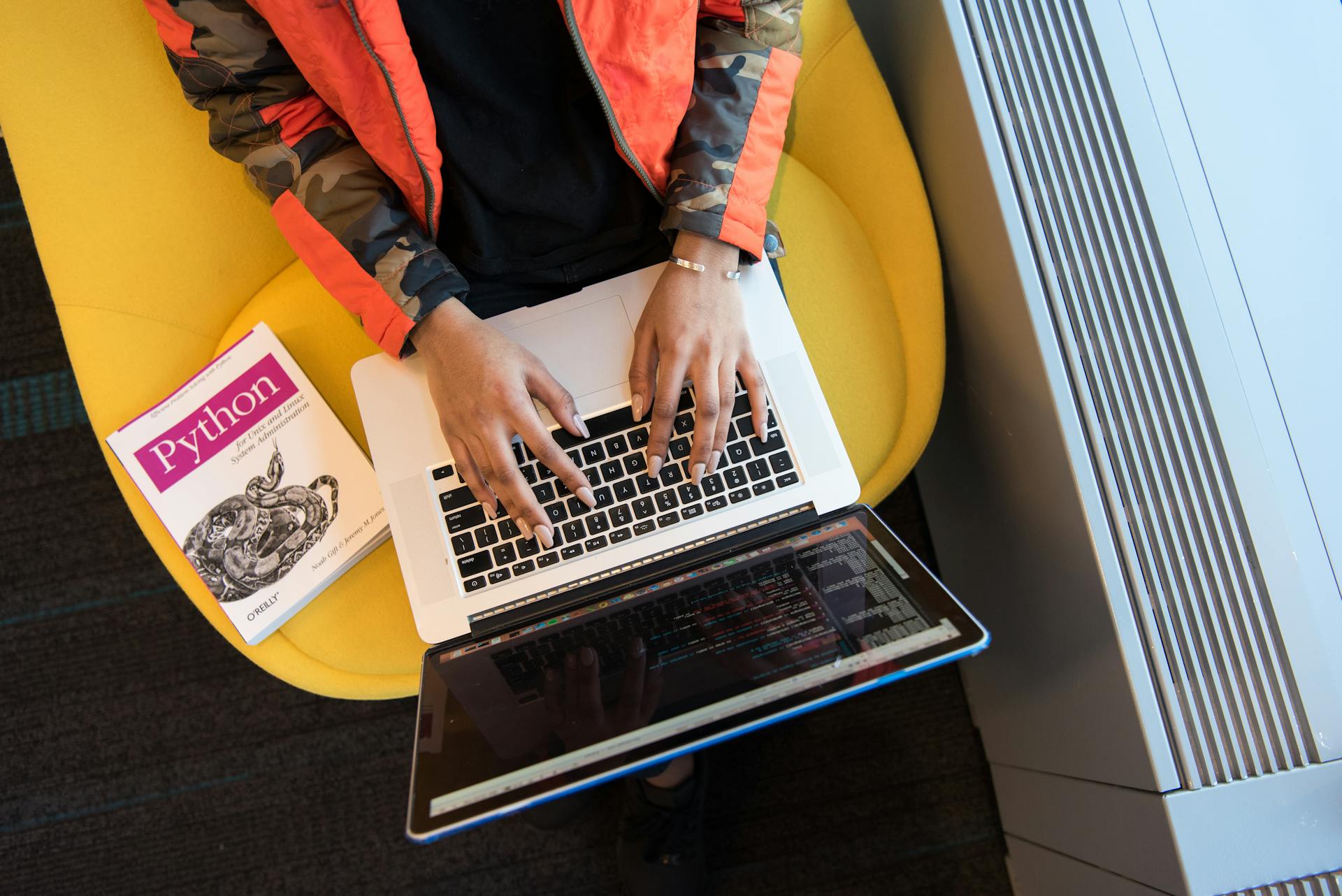
Reading HTML files in Python is a straightforward process that can be accomplished using various libraries, including BeautifulSoup and lxml.
The BeautifulSoup library is particularly useful for parsing HTML and XML documents, allowing you to navigate through the elements and modify the content as needed.
To get started, you'll need to install the BeautifulSoup library using pip, the Python package manager.
With BeautifulSoup installed, you can use its `open` function to read an HTML file and parse its contents.
Setting Up
To get started with reading HTML files in Python, you'll need to set up your environment with the necessary libraries.
First, ensure you have pip installed, as it's the package installer for Python. pip is usually included with Python, but if you're using a different package manager, you'll need to install it separately.
Next, install the BeautifulSoup and lxml libraries using pip. These two libraries will be your primary tools for parsing HTML files.
You can install them using pip commands, specifically pip install beautifulsoup4 and pip install lxml.
Loading and Modifying Files
To load an HTML file into your Python script, you can use the built-in open function to read its contents.
You can open an HTML file by specifying its path and mode, and then read its contents using the read method.
Once the HTML file is loaded, you can modify its contents using string manipulation techniques or more advanced methods provided by libraries like BeautifulSoup.
To remove a specific element from the HTML file, you can use BeautifulSoup's extract method.
Loading and modifying HTML files is a crucial step in web scraping and data analysis, and understanding how to do it can help you extract the information you need.
If this caught your attention, see: Creating Simple Html to Extract Information from Xml File
Parsing Libraries
BeautifulSoup is the most popular HTML parsing library, known for its ease of use and powerful tools for searching, navigating, and modifying HTML content.
It's a third-party package that needs to be installed using pip, and can be used to create parsing trees and extract useful data from HTML documents.
BeautifulSoup provides a simple and intuitive API that makes it easy to extract data from HTML documents, supporting a wide range of parsing strategies and handling malformed HTML documents with ease.
lxml is a high-performance library that provides a fast and efficient parsing engine, making it ideal for parsing large or complex XML and HTML documents.
It supports a wide range of parsing strategies, including XPath and CSS selectors, and is highly compatible with various XML and HTML standards.
Here are the key features of popular HTML parsing libraries:
pyquery is a Python library that provides a jQuery-like syntax for parsing HTML documents, making it easy for developers familiar with jQuery to get started with HTML parsing in Python.
Pandas.read
Pandas.read is a powerful function that allows you to read data from various file formats into a DataFrame.
It supports reading data from CSV, Excel, JSON, and other file types.
You can specify the file path and optional parameters to customize the reading process.
You might like: Basic Html How to Read Json of Data
For example, you can use the 'header' parameter to specify whether the first row of the file contains column names.
The 'na_values' parameter allows you to specify custom missing value indicators.
Pandas.read can also read data from URLs, making it easy to fetch data from online sources.
Remember to check the documentation for specific file formats and parameters.
Parser Overview
Parsing libraries are a crucial part of any web scraping or data extraction task, and there are several options to choose from.
BeautifulSoup is a widely used Python library for web scraping and parsing HTML and XML documents. It's easy to use and provides a lot of powerful tools for searching, navigating, and modifying HTML and XML content.
lxml is a high-performance library that provides a fast and easy way to parse HTML and XML documents. It's built on top of libxml2 and libxslt libraries, which make it one of the fastest and most memory-efficient HTML parsing libraries available in Python.
Consider reading: File for Important Documents
html5lib is a pure-Python library that provides a simple and easy-to-use API for parsing and manipulating HTML and XML documents. It's designed to parse HTML5 documents, which can be more complex than earlier versions of HTML.
requests-html is a Python library that combines the power of the requests library with the flexibility of HTML parsing using a browser-like interface. It provides a simple and intuitive way to extract data from HTML documents, and can even render JavaScript and CSS.
pyquery is a Python library that provides a jQuery-like syntax for parsing HTML documents. It's built on top of lxml and supports a wide range of parsing strategies, including CSS selectors and XPath.
Here's a quick comparison of these popular parsing libraries:
Note that this is not an exhaustive list, and there are many other parsing libraries available for Python. However, these five libraries are some of the most popular and widely used options.
Example and Overview
Let's take a look at some examples of how to read and parse HTML files in Python. We can start by using the BeautifulSoup library, which is a powerful tool for searching, navigating, and modifying HTML content.
You can load an HTML file using BeautifulSoup by creating a BeautifulSoup object and passing in the file path. For example, if we have an HTML file called 'myhtml.html', we can load it into BeautifulSoup like this: `soup = BeautifulSoup('myhtml.html', 'html.parser')`.
To remove an element from the HTML structure, you can use the `find` method to locate the element and then use the `decompose` method to remove it. The modified HTML can be printed using the `prettify` method to visualize the changes.
We can also use the HTMLParser class to print out start tags, end tags, and data as they are encountered. This is useful for debugging and understanding the structure of the HTML document.
Additional reading: How to Use Notepadd for Html Coding
Let's take a look at some of the most popular Python HTML parsers. Here are a few of the most widely used ones:
Extracting and Traversing
Extracting specific data from an HTML file involves navigating its structure using BeautifulSoup.
To extract data, you often need to find the desired element or elements using their tags, classes, or attributes.
You can use the .children attribute to iterate over the children of a tag, making it easier to traverse the HTML structure.
Recursive child generators are a powerful technique to extract the desired information by recursively traversing the entire structure.
You can load an HTML file, create a BeautifulSoup object, and then extract all li elements within a ul element to get the article titles.
The .children attribute allows you to iterate over the children of a tag, making it easier to traverse the HTML structure.
Traversing the HTML structure with recursive child generators can be achieved by defining a recursive function that prints the tag name and its content and then recursively calls itself for each child element.
By using recursive child generators, you can extract the desired information by traversing the entire HTML structure.
Take a look at this: Self Closing Tag Html
Frequently Asked Questions
Can pandas read HTML?
Yes, pandas can read HTML, thanks to the read_html() function that extracts tables from web pages and converts them into DataFrames. This makes web scraping tabular data a breeze, even for beginners.
Sources
- https://pandas.pydata.org/docs/reference/api/pandas.read_html.html
- https://www.tutorialspoint.com/how-to-parse-local-html-file-in-python
- https://docs.python.org/3/library/html.parser.html
- https://www.scrapingdog.com/blog/best-python-html-parsing-libraries/
- https://scrapeops.io/python-web-scraping-playbook/best-python-html-parsing-libraries/
Featured Images: pexels.com