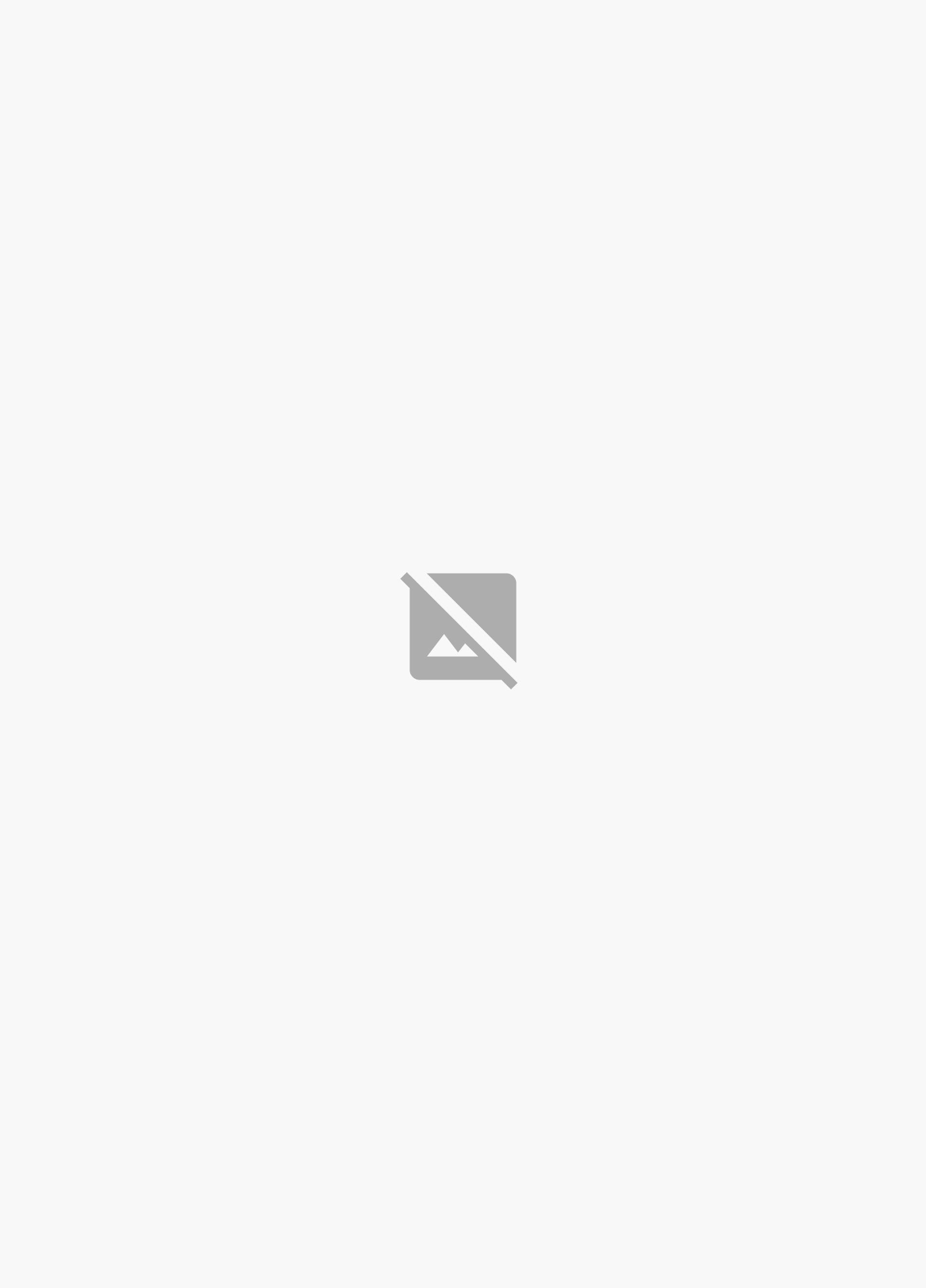
To create a carousel in Twitter Bootstrap, you need to include the carousel JavaScript plugin in your project. This can be done by adding the necessary JavaScript files to your project, including the bootstrap-carousel.js file.
The carousel component is made up of several key elements, including the carousel-inner, carousel-item, and carousel-control elements. These elements work together to create a smooth and seamless carousel experience.
The carousel-inner element is where the carousel images are placed, and it's a required element for the carousel to work properly.
Customization
You can customize the transition duration of the carousel by changing the $carousel-transition-duration Sass variable before compiling or adding custom styles to the compiled CSS.
The transition duration should be defined first, especially if multiple transitions are applied, such as transform and opacity. For example, you can use `transition: transform 2s ease, opacity .5s ease-out`.
You can also customize the carousel by passing options via data attributes or JavaScript. Options include interval, keyboard, pause, ride, and wrap.
Options
Options are a crucial part of customizing your carousel. You can pass options via data attributes or JavaScript.
The interval option specifies the amount of time to delay between automatically cycling an item. If set to false, the carousel will not automatically cycle.
The keyboard option determines whether the carousel should react to keyboard events. By default, it is set to true, allowing users to navigate through slides using the left and right arrow keys.
The pause option can be set to 'hover' to pause the cycling of the carousel when the mouse pointer enters the carousel and resume cycling when the mouse pointer leaves.
The ride option autoplays the carousel after the user manually cycles the first item, or on load if set to 'carousel'.
You can also set the wrap option to false to make the carousel stop at the last slide instead of cycling continuously.
Here is a summary of the options:
Data Attributes
Data Attributes are a powerful tool for customizing carousels in Bootstrap. They allow you to easily control the position of the carousel without writing a single line of JavaScript code.
You can use the data-slide attribute to move the slide position relative to its current position by using the keywords prev or next. Alternatively, you can use data-slide-to to pass a raw slide index to the carousel, which shifts the slide position to a particular index beginning with 0.
Using data-slide-to="2" will shift the slide position to the third slide, starting from 0. This is a convenient way to jump to a specific slide in the carousel.
The data-ride attribute is used to mark a carousel as animating starting at page load. However, if you don't use data-ride="carousel" to initialize your carousel, you have to initialize it yourself.
Data attributes like data-bs-slide and data-bs-slide-to work similarly to data-slide and data-slide-to. They allow you to control the slide position and jump to specific slides using the carousel's index.
The data-bs-ride attribute has the same function as data-ride, marking the carousel as animating starting at page load. But be aware that using data-bs-ride in combination with explicit JavaScript initialization is redundant and unnecessary.
Navigation
To create a navigation system for your Twitter Bootstrap carousel, you'll need to understand the basic markup pattern required by the Bootstrap carousel.
The Bootstrap carousel requires a specific markup pattern, with appropriate classes, as stated in the documentation.
The markup pattern starts with a div container, which wraps the carousel's content.
This div container has a class of "carousel" to indicate that it's a carousel component.
In addition to the "carousel" class, you'll also need to include a "carousel-inner" class within the carousel div to define the inner content of the carousel.
Appearance
The Twitter Bootstrap carousel is a visually appealing feature that can be customized to fit your website's style.
The carousel's appearance can be customized using various classes and options.
You can change the carousel's width by adding the .carousel-inner class to the inner container, which can be set to a fixed width using CSS.
Custom Transition
Custom transitions can be a game-changer for your carousel's appearance.
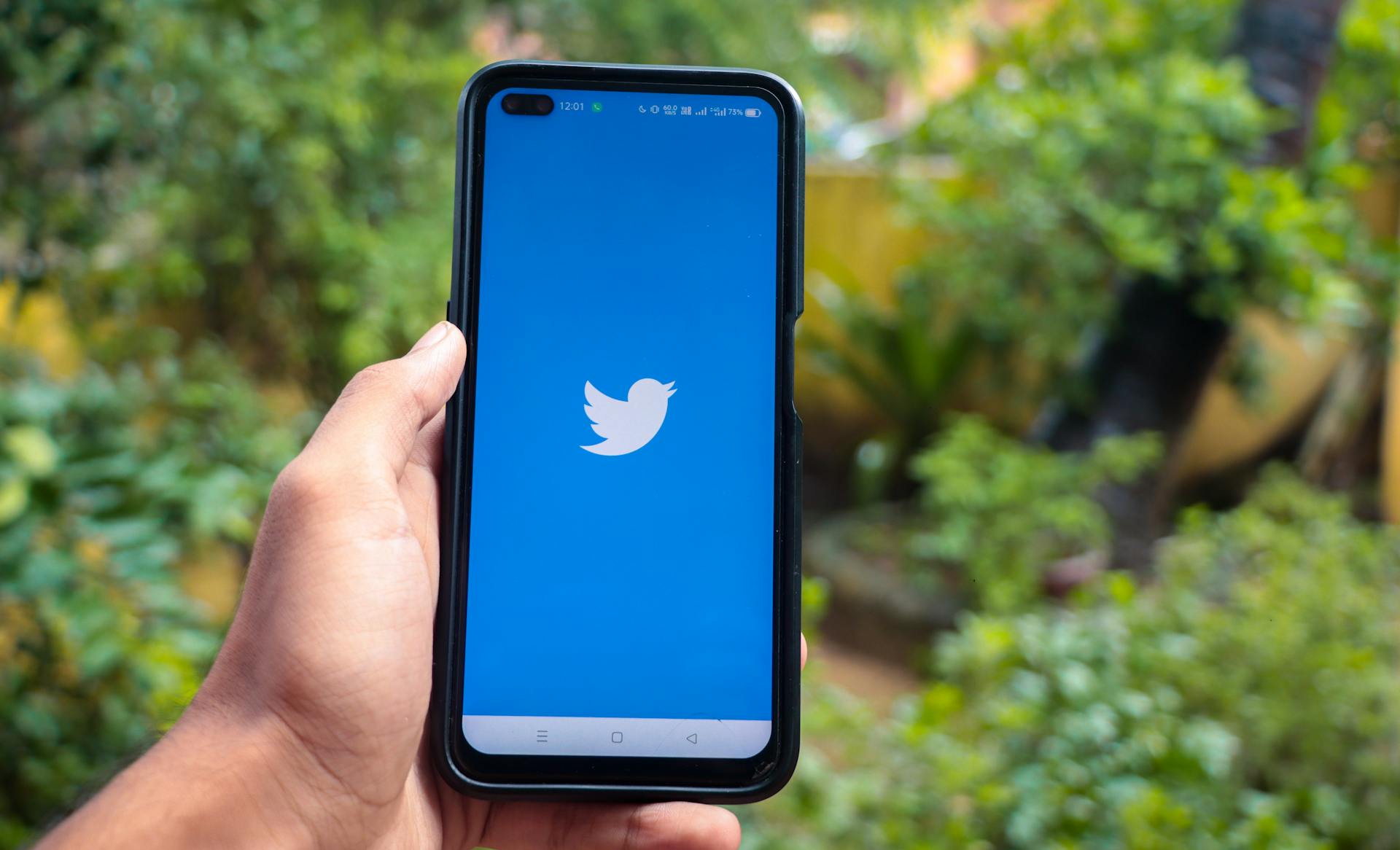
To change the transition duration of .carousel-item, you can use the $carousel-transition-duration Sass variable before compiling or custom styles if you're using the compiled CSS.
If multiple transitions are applied, make sure the transform transition is defined first, such as transition: transform 2s ease, opacity .5s ease-out.
This is crucial for a smooth and seamless animation experience.
Dark Variant Creation
To create a dark variant of the carousel, add .carousel-dark to the .carousel for darker controls, indicators, and captions.
Controls are inverted compared to their default white fill with the filter CSS property, giving them a distinct look.
Captions and controls have additional Sass variables that customize the color and background-color, allowing for further customization.
However, it's worth noting that dark variants for components were deprecated in v5.3.0, so you may need to consider alternative methods for achieving the desired effect.
You can achieve a similar effect by setting data-bs-theme="dark" on the root element, a parent wrapper, or the component itself, as recommended in the documentation.
Behavior
The Twitter Bootstrap carousel is designed to cycle through a series of content, built with CSS 3D transforms and a bit of JavaScript.
It works with a variety of content types, including images, text, and custom markup. This makes it a versatile tool for showcasing different types of information on your website.
One key feature is that it includes support for previous/next controls and indicators, allowing users to easily navigate through the content.
How It Works
The carousel is a slideshow that cycles through a series of content using CSS 3D transforms and JavaScript.
It works with a variety of content types, including images, text, and custom markup, and includes support for previous/next controls and indicators.
The carousel will avoid sliding when the webpage is not visible to the user, such as when the browser tab is inactive or the browser window is minimized.
This is achieved through the use of the Page Visibility API, which is supported in certain browsers.
Nested carousels are not supported, and carousels may not be compliant with accessibility standards.
Individual Interval
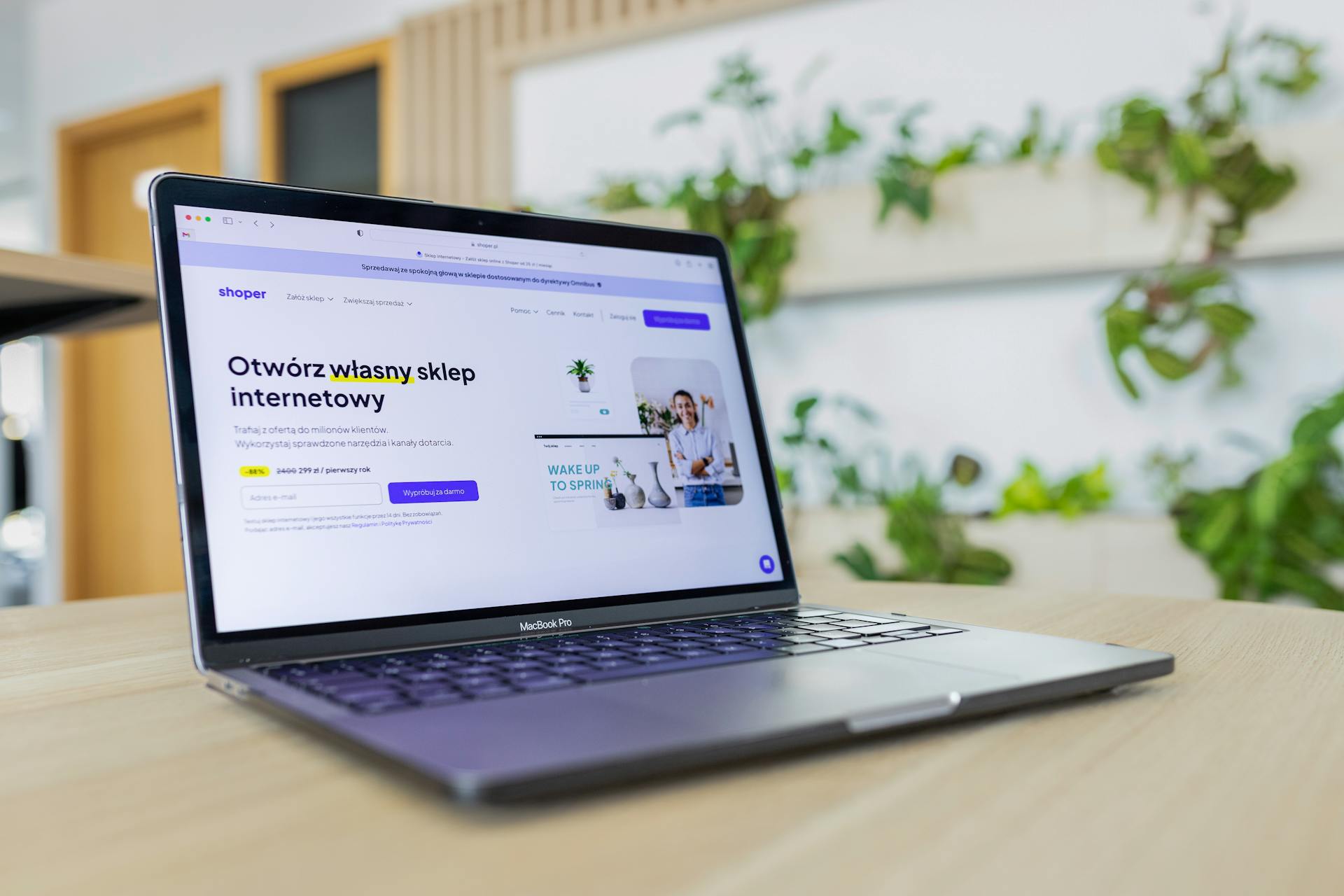
Individual Interval is a powerful feature that lets you customize the timing of your carousel.
By adding data-bs-interval="" to a .carousel-item, you can change the amount of time to delay between automatically cycling to the next item.
You can use this feature to create a unique experience for each item in your carousel, making it more engaging and interactive.
To set an individual interval, simply add the data-bs-interval attribute to the specific .carousel-item you want to modify.
This feature is super useful when you want to highlight a particular item or create a sense of anticipation before moving on to the next one.
Disable Touch Swiping
Disable touch swiping can be a bit tricky, but it's actually quite simple. You can disable it by setting the touch option to false.
Carousels support swiping left/right on touchscreen devices to move between slides. This is a pretty standard feature, but if you don't want it, you can disable it using the data-bs-touch attribute.
Next When Visible
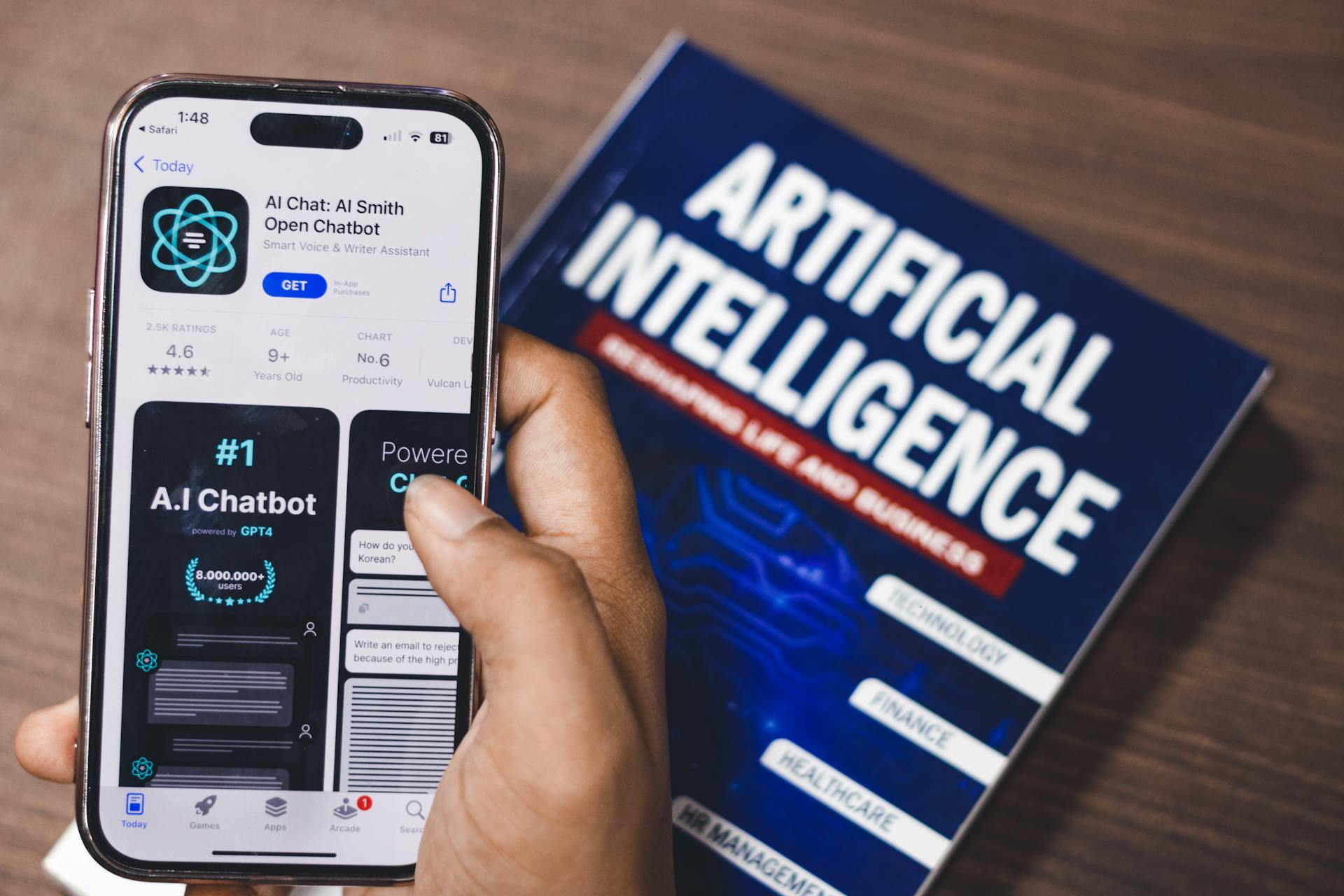
Next When Visible is a crucial aspect of carousel behavior. It prevents the carousel from cycling to the next item when the page isn't visible or the carousel or its parent isn't visible.
Don't cycle carousel to next when the page isn't visible or the carousel or its parent isn't visible, as mentioned in the example. This ensures a seamless user experience.
The "nextWhenVisible" rule is designed to prevent unnecessary cycling, which can be frustrating for users. It's a simple yet effective way to improve user engagement.
By following this rule, you can ensure that your carousel only cycles to the next item when it's actually visible to the user. This makes a big difference in how users interact with your content.
Pause
The pause feature is a great way to control the flow of your carousel. You can stop the carousel from cycling through items by using the command ".carousel('pause')".
Number
The carousel behavior is quite fascinating, and one of the key aspects is how it cycles through frames. You can cycle the carousel to a particular frame using the ".carousel(number)" method.
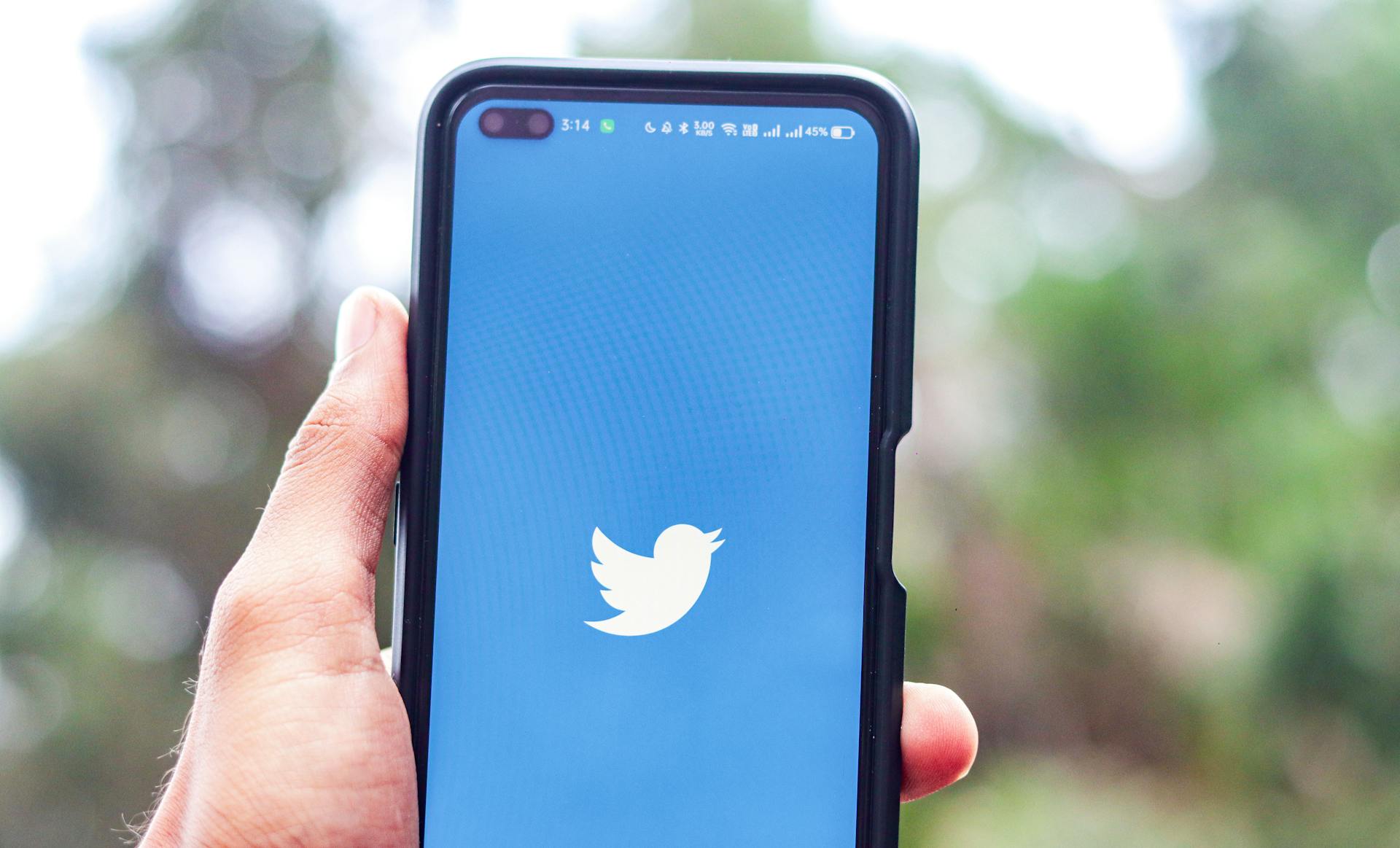
This method is zero-based, meaning the first frame is numbered 0, just like an array. You can use it to target a specific frame and return to the caller before the target item has been shown.
The ".carousel(number)" method returns to the caller before the slid.bs.carousel event occurs, which is an important detail to keep in mind when working with carousels.
Refresh and Check
Refreshing your browser is a crucial step to see the results of your markup. Each image rotation takes five seconds, so be patient.
To ensure the slideshow works properly, make sure your mouse doesn't hover over it, as this will pause the timer. I've noticed this can be a common mistake, so double-check your mouse movements.
To refresh and check your work, select your desired images and edit them to your desired dimensions, keeping them consistent. Consistent dimensions are key to a smooth slideshow experience.
Here are the steps to follow:
- Select your desired images.
- Edit them to your desired dimensions.
- Wrap the images in links if you'd like.
- Supply your desired captions.
By following these steps and being mindful of the slideshow's timer, you'll be able to see your results and make any necessary adjustments.
Activate
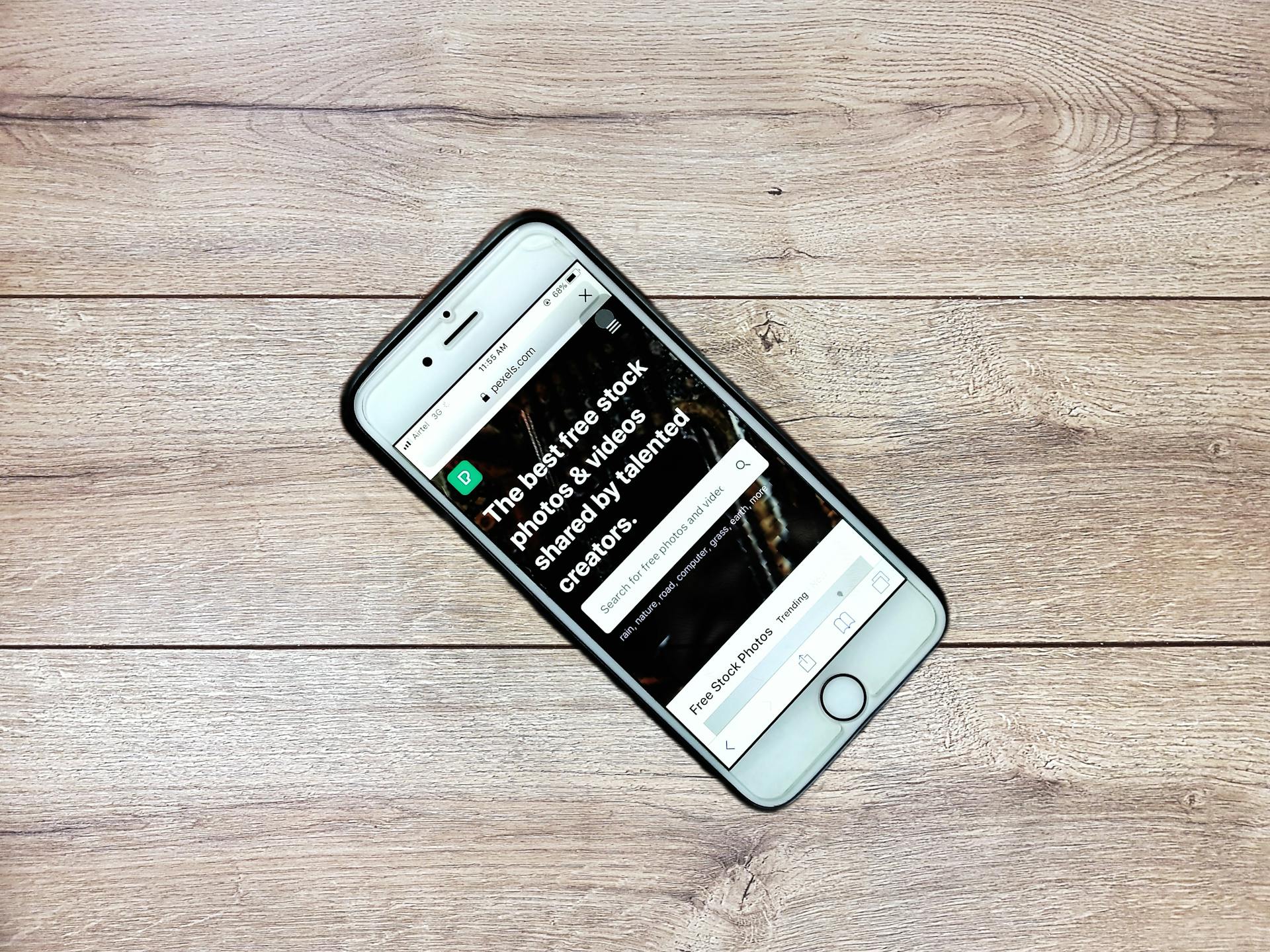
Activating a carousel is a breeze with Bootstrap. You can create carousels easily via data attributes without writing any JavaScript code.
With Bootstrap, you can create carousels using data attributes, which is a great time-saver. This method eliminates the need for JavaScript code altogether.
To activate a carousel manually, you can use the carousel() method in your JavaScript code. This method is particularly helpful when you don't want your carousel to start sliding or animating at page load.
Manually activating a carousel via JavaScript can be very useful in situations where you want more control over the carousel's behavior.
Activate Data Attributes
You can create carousels easily via data attributes without writing a single line of JavaScript code. This is a game-changer for anyone who wants to get started with carousels quickly.
The data-slide attribute accepts the keywords prev or next, which alters the slide position relative to its current position. This means you can easily move the carousel forward or backward with just a few lines of code.
Use data-slide-to to pass a raw slide index to the carousel, which shifts the slide position to a particular index beginning with 0. For example, data-slide-to="2" shifts the slide position to the third index.
The data-ride="carousel" attribute is used to mark a carousel as animating starting at page load. This is a convenient way to get your carousel up and running with minimal code.
Alternatively, you can use data-bs-slide or data-bs-slide-to in Bootstrap versions, which work in a similar way to the original data-slide and data-slide-to attributes.
Sources
- https://getbootstrap.com/docs/4.0/components/carousel/
- https://getbootstrap.com/docs/5.3/components/carousel/
- https://getbootstrap.com/docs/5.0/components/carousel/
- https://webdesign.tutsplus.com/twitter-bootstrap-101-the-carousel--webdesign-7442a
- https://www.tutorialrepublic.com/twitter-bootstrap-tutorial/bootstrap-carousel.php
Featured Images: pexels.com