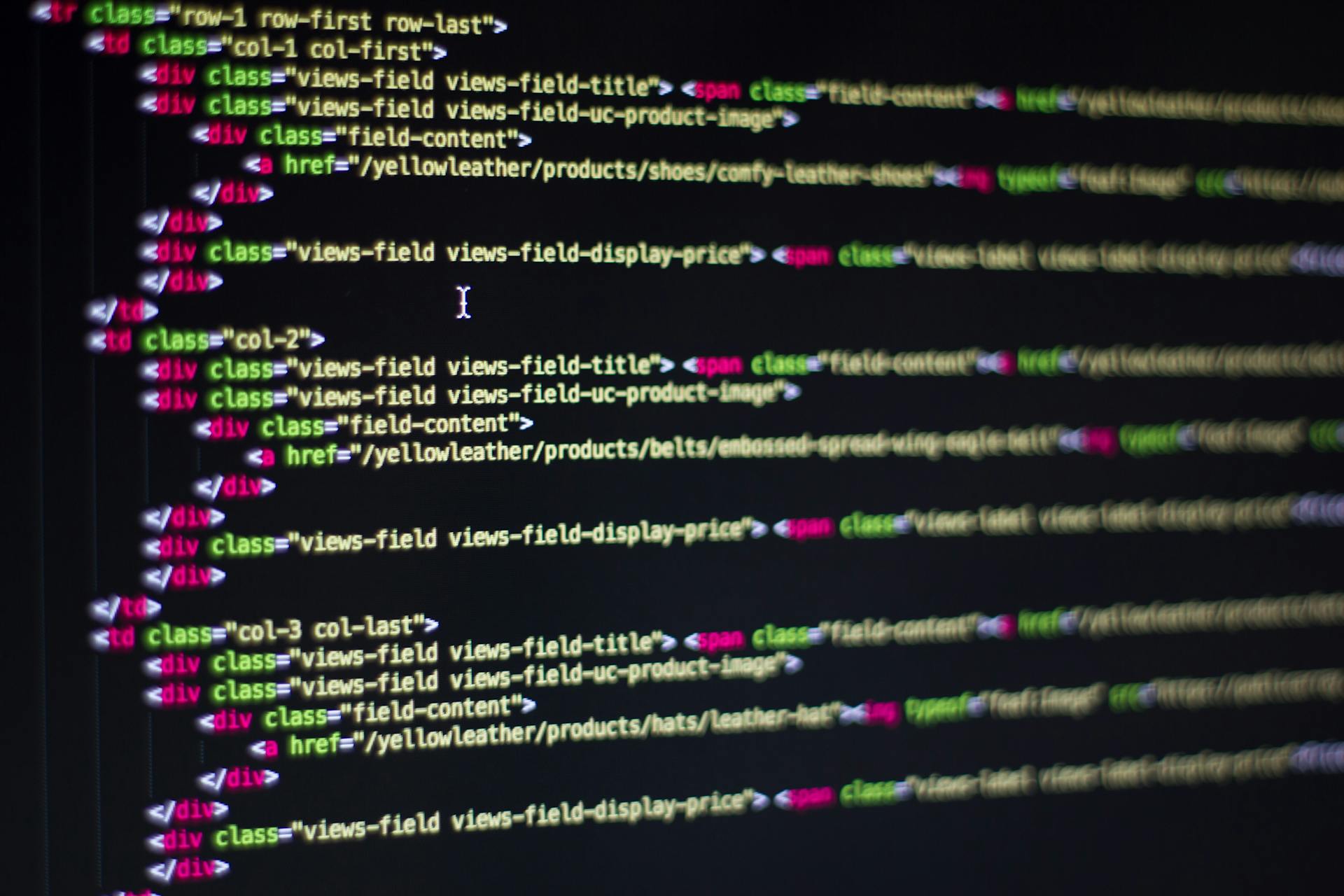
XML is a markup language that provides a way to store and transport data between systems, making it a crucial component in web services. It's used to describe the structure and content of data, allowing different systems to communicate and exchange information.
In web services, XML is often used to format data for exchange between systems, such as between a client and a server. This is done using XML messages, which can be sent over the internet or other networks.
The use of XML in web services enables interoperability between different systems, allowing them to communicate and exchange data in a standardized way. This is achieved through the use of XML schema, which defines the structure and format of the data being exchanged.
By using XML in web services, developers can create systems that are more flexible and adaptable, able to handle different types of data and systems.
On a similar theme: Historical Website Traffic
Creating a Client
Creating a client for your web service is a crucial step in making it accessible to users. To create a client, you'll need to code the implementation class.
You can use either an application client or a web client, both of which can be created using the same basic steps. The application client is a stand-alone application that accesses the service, while the web client is a servlet that makes the call through a port.
The starting point for developing a client is to code the client class. You'll also need to use the wsimport Maven goal to generate and compile the web service artifacts needed to connect to the service.
Here are the basic steps for creating a client:
- Code the client class.
- Use the wsimport Maven goal to generate and compile the web service artifacts needed to connect to the service.
- Compile the client class.
- Run the client.
If you're using NetBeans IDE, the IDE will perform the wsimport task for you.
Endpoint Requirements and Testing
To create a web service endpoint, you must follow some specific requirements. The implementing class must be annotated with either the jakarta.jws.WebService or the jakarta.jws.WebServiceProvider annotation.
Here are the key requirements for a web service endpoint:
- The implementing class must be annotated with either the jakarta.jws.WebService or the jakarta.jws.WebServiceProvider annotation.
- The business methods must be public and must not be declared static or final.
- The implementing class must not be declared final and must not be abstract.
- The implementing class must have a default public constructor.
- The implementing class must not define the finalize method.
Testing the methods of a web service endpoint is also crucial. You can test the methods using GlassFish Server, which allows you to test the methods of a web service endpoint.
Suggestion: Azure Service Endpoints
Endpoint Requirements
An XML Web Services endpoint must be annotated with either the jakarta.jws.WebService or the jakarta.jws.WebServiceProvider annotation.
The implementing class can explicitly reference an SEI through the endpointInterface element of the @WebService annotation, but it's not required to do so. If no endpointInterface is specified in @WebService, an SEI is implicitly defined for the implementing class.
Business methods of the implementing class must be public and cannot be declared static or final. They must also be annotated with jakarta.jws.WebMethod.
The implementing class must have Jakarta XML Binding-compatible parameters and return types for business methods exposed to web service clients.
Here's a quick rundown of the requirements for business methods:
- Must be public
- Cannot be static or final
- Must be annotated with jakarta.jws.WebMethod
- Must have Jakarta XML Binding-compatible parameters and return types
The implementing class must not be declared final and must not be abstract. It must also have a default public constructor.
Endpoint Testing
Testing a web service endpoint is a crucial step in ensuring its functionality and reliability. GlassFish Server allows you to test the methods of a web service endpoint.
To test a SOAP web service, you'll need to run and test it. Simply right-click on the project, select Run As > Run on Server, and the web container will create a WSDL file that describes the service's remote API.
Note the location of the WSDL file, as you'll need it to access the web service. The WSDL file location can be found in the console output of the server.
With the WSDL file location in hand, you can use Eclipse's Web Services Explorer to browse to the file and invoke the public methods of the web service. This web-based SOAP client makes it easy to test the web service's functionality.
Take a look at this: Google Cloud Bucket How to Store Index File
Interoperability and Platform Independence
Jakarta XML Web Services supports the Web Services Interoperability (WS-I) Basic Profile Version 1.1. This profile clarifies the SOAP 1.1 and WSDL 1.1 specifications to promote SOAP interoperability.
The WS-I Basic Profile Version 1.1 is a document that promotes SOAP interoperability by clarifying the SOAP 1.1 and WSDL 1.1 specifications. Jakarta XML Web Services supports this profile to ensure seamless communication between different systems.
Expand your knowledge: Microsoft Xml Core Services
To support WS-I Basic Profile Version 1.1, the JAX-WS runtime supports doc/literal and rpc/literal encodings for services, static ports, dynamic proxies, and the Dynamic Invocation Interface (DII). This means that developers can create services that can be consumed by different systems, regardless of the underlying technology.
XML Web services help create platform-neutral systems by using the same set of standards as the Internet. This allows applications built on one platform to call services built on a completely unrelated platform.
Using the HTTP protocol, requests are sent from client applications to XML Web services for processing in an XML standard format. The request is then processed, and an XML document containing the requested data is sent back to the client application.
The WS-I Basic Profile Version 1.1 is a key factor in achieving platform independence with XML Web services. By supporting this profile, developers can create services that can be consumed by different systems, without worrying about the underlying technology.
The various web services with web platforms are XML plus HTTP, XML plus Soap, XML plus UDDL, XAML, XMS, XLANG, and the XKMS.
Check this out: Xml Conversion Services
SOAP and JAX-WS
SOAP is used as the medium by which XML Web services accept and transmit information to the rest of the world. It's an XML subset called Simple Object Access Protocol that marks up calls to XML Web service methods and functions, including required arguments.
A simple SOAP document can be sent to the URL of an XML Web service and, if formatted properly, will cause the service to run a method and send back its results in another SOAP document. This helps maintain type safety even across differing platforms.
The Java API for XML Web Services, or JAX-WS, is used by JBossWS, which leverages the JBoss Application Server as its target container. It's a programming model that focuses on web service deployments that use EJB3 service implementations.
Java Schema Mapping
Java Schema Mapping is a crucial step in SOAP and JAX-WS development. It involves mapping Java classes to XML data types.
The Java language provides a richer set of data types than XML schema, which means you'll need to map your Java classes to the corresponding XML data types. This process is called schema-to-Java mapping.
See what others are reading: Java Web Dev
Here's a list of common Java classes and their corresponding XML data types:
In addition to mapping Java classes to XML data types, you'll also need to consider the mapping of XML data types to Java data types. This is especially important when working with primitive types such as integers and strings.
Here's a list of common XML data types and their corresponding Java data types:
By understanding these mappings, you'll be able to effectively integrate your Java classes with XML data types, making it easier to develop and deploy SOAP and JAX-WS applications.
SOAP
SOAP is a standard format used for sending and receiving data between systems. It's an XML subset that marks up method calls and returned results so that clients can understand what's being sent back to them.
SOAP documents are used to transmit information to and from XML Web services. They're essentially a way of marking up a call to an XML Web service method or function, including all of its required arguments.
A simple SOAP document can be sent to the URL of an XML Web service, causing the service to run a method and send back its results in another SOAP document. This is a powerful way for systems to communicate with each other.
Data in SOAP documents is marked up with XML tags denoting the type of data being sent, such as string or long, and the variable name to which the data was sent. This helps maintain type safety even across differing platforms.
Recommended read: Google Cloud Platform Data Centers
Java API for JAX-WS
Java API for JAX-WS is built on top of the Java API for XML Web Services, or JAX-WS for short. JBossWS uses the JBoss Application Server as its target container.
JAX-WS supports EJB3 service implementations, which is a key feature of this API. The JBoss Application Server is a popular choice for deploying web services.
For web service deployments that leverage EJB3 service implementations, JAX-WS is the way to go. JBossWS is designed to work seamlessly with JAX-WS.
Additional reading: Web Server Programming
If you're looking to deploy a web service using JAX-WS, you'll need to understand the JAX-WS programming models. JAXB documentation is also a valuable resource for more complex mapping problems.
JAX-WS is a powerful tool for building web services, and with the right guidance, you can create robust and scalable applications.
What About the Payload?
When working with SOAP and JAX-WS, one thing to consider is the payload. The method parameters and return values represent our XML payload, which needs to be compatible with JAXB2.
JAXB2 relies on meaningful defaults, so you may not need any JAXB annotations, but it's a good idea to include them for documentation purposes.
In some cases, JAXB annotations are necessary to ensure compatibility. For example, JAXB relies on default values for certain elements, but adding annotations can provide more clarity and control over the payload.
Consuming Web Services
Consuming web services is a fundamental aspect of working with XML in web services.
You can start by creating web service clients from the WSDL, which JBossWS ships with a set of tools to generate the required JAX-WS artefacts to build client implementations.
In the most basic usage patterns, you would usually start from the WSDL, but for a more detailed introduction to web service clients, consult the user guide.
To create a web service client, you'll need to follow these basic steps:
- Code the implementation class.
- Compile the implementation class.
- Package the files into a WAR file.
- Deploy the WAR file.
- Code the client class.
- Use the wsimport Maven goal to generate and compile the web service artifacts needed to connect to the service.
- Compile the client class.
- Run the client.
The wsimport Maven goal generates the web service artifacts, which are used to communicate with clients, during deployment.
If you're using NetBeans IDE, it performs the wsimport task for you, making it easier to create a service and client.
A simple XML web services application client, like the HelloAppClient class, accesses the sayHello method of HelloService through a port, which is created at development time by the wsimport Maven goal.
A servlet like HelloServlet also calls the sayHello method of the web service, making the call through a port, similar to the application client.
To consume a WSDL and produce annotated Java classes, use the wsconsume tool, which is used to generate the abstract contract (WSDL) and produce annotated Java classes.
When using wsconsume, you can specify the package name of the generated sources with the -p switch.
Consider reading: Java Google Cloud Storage
XML Schema and Validation
XML schema provides a framework for validation and core processing of Extensible Markup Language.
DSDL or Document Schema Definition Languages offers a framework for validation and core processing of XML, containing individual specifications that can be used separately or collectively for XML validation.
RELAX NG is a grammar-based schema language used to describe, define, and provide limitations for XML language, limiting and defining terms in language.
A fresh viewpoint: Python Framework Django
Java to Schema Mapping
Java-to-Schema Mapping is a crucial step in ensuring data consistency and integrity when working with XML.
Java classes are mapped to XML data types, and this mapping is not always straightforward.
The default mapping of Java classes to XML data types is as follows: Java ClassXML Data Typejava.lang.Stringxs:stringjava.math.BigIntegerxs:integerjava.math.BigDecimalxs:decimaljava.util.Calendarxs:dateTimejava.util.Datexs:dateTimejavax.xml.namespace.QNamexs:QNamejava.net.URIxs:stringjavax.xml.datatype.XMLGregorianCalendarxs:anySimpleTypejavax.xml.datatype.Durationxs:durationjava.lang.Objectxs:anyTypejava.awt.Imagexs:base64Binaryjakarta.activation.DataHandlerxs:base64Binaryjavax.xml.transform.Sourcexs:base64Binaryjava.util.UUIDxs:string
This mapping can be useful for understanding how Java classes are represented in XML.
XML Schema Definition Languages
XML Schema Definition Languages offer a framework for validating and processing XML. They contain individual specifications that are well-defined and can be used separately or collectively.
DSDL, or Document Schema Definition Languages, is one such framework that provides a set of specifications for XML validation. RELAX NG is another language editor that describes, defines, and limits XML language through grammar-based schemas.
Schematron is a rules-based language that creates rules to define and limit XML language. It does not impose limitations, but rather enforces rules to ensure XML compliance.
These schema definition languages play a crucial role in ensuring the structure and syntax of XML documents are correct. They help prevent errors and inconsistencies in XML data.
Add Annotations
Adding annotations to your classes is a crucial step in preparing them for XML schema and validation.
The @XMLType annotation is required for classes that will be sent to SOAP web services clients in XML format.
You'll also need to add the @XmlAccessorType annotation to indicate field-based access, as the XML engine will need to look directly at the properties of your class.
This is especially important if your class has no getter methods, as the XML engine will need to access the properties directly.
XPath
XPath is considered the most successful of all the XML technologies today.
It forms a syntax or a data model to identify different parts of an XML document. This makes it a crucial tool for navigating and extracting specific information from XML documents.
XPath is a key part of XML, allowing developers to pinpoint exactly where they need to look within a document to find the data they're interested in.
Frequently Asked Questions
What is the difference between WSDL and XML?
WSDL (Web Service Description Language) defines web service locations and operations, while XSD (XML Schema Definition) validates XML documents and defines their structure. Understanding the difference between these two is crucial for building and consuming web services effectively.
Sources
- https://jakarta.ee/learn/docs/jakartaee-tutorial/9.1/websvcs/jaxws/jaxws.html
- https://www.informit.com/articles/article.aspx
- https://docs.jboss.org/author/display/WFLY10/Java%20API%20for%20XML%20Web%20Services%20(JAX-WS).html
- https://www.theserverside.com/video/Step-by-step-SOAP-web-services-example-in-Java-using-Eclipse
- https://www.exforsys.com/tutorials/xml/xml-web-services.html
Featured Images: pexels.com