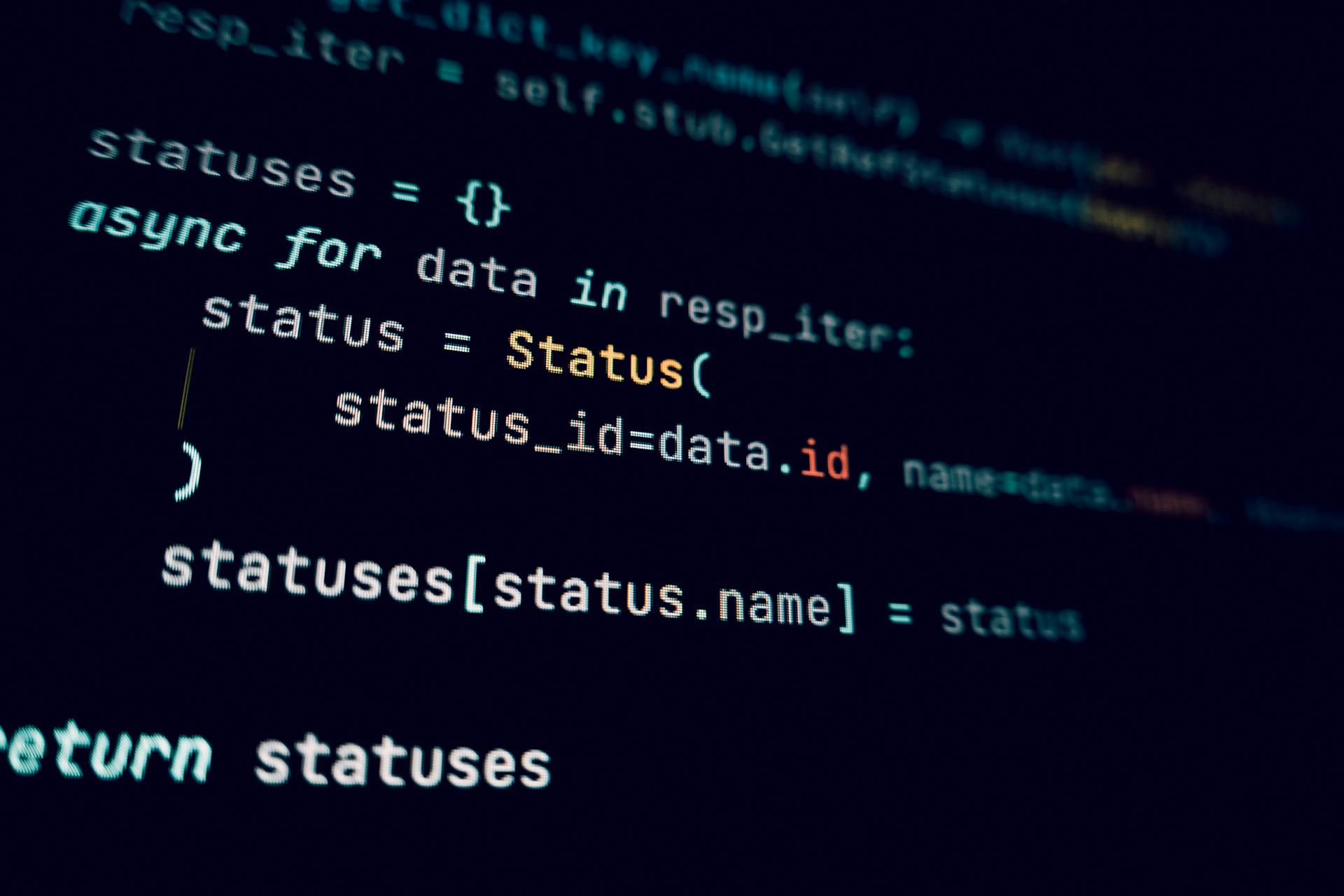
Azure API Apps are a great way to create, deploy, and manage APIs. They provide a scalable and secure way to build APIs that can be consumed by various clients.
One of the key features of Azure API Apps is their ability to handle large volumes of traffic, making them ideal for high-traffic APIs. This is due to their auto-scaling capabilities, which allow them to dynamically adjust to changing loads.
With Azure API Apps, you can easily manage and monitor your APIs using the Azure Portal or Azure CLI. This makes it easy to keep track of your API's performance and make any necessary adjustments.
Azure API Apps also support various authentication and authorization methods, including Azure Active Directory, OAuth, and API keys. This allows you to control access to your API and ensure that only authorized clients can access it.
Services and Features
Azure API Apps are built on top of Azure App Service, which offers a range of features and capabilities to support your API development needs.
App Service is a fully managed platform that allows you to build, deploy, and scale web applications and APIs in the cloud. You can learn more about App Service features and capabilities.
With Azure App Service, you can easily deploy and manage your API apps, and take advantage of features like automatic scaling, load balancing, and SSL/TLS support.
Worth a look: Deploy to Azure Container Apps
Services Available
App Service offers various services, including Web App, API App, Mobile App, and Logic App. These services cater to different needs, from web development to mobile applications.
Web App is one of the most used services, offering serverless code that can be run on-demand without provisioning or managing infrastructure. This means you only pay for the compute time your code uses.
API App, another popular service, allows you to create an Azure API app with specific requirements.
To better manage and monitor your Web Apps and API Apps, App Service offers features such as Turbo360.
Intriguing read: Azure Mobile Apps
Automate Discovery with Swashbuckle
Using Swashbuckle, you can automate API discovery by leveraging the Swagger framework, which is a popular, open-source tool for designing, building, and documenting RESTful APIs.
Swashbuckle is especially useful if you're building your API using ASP.NET or ASP.NET Core, as it can automatically generate Swagger metadata for you, saving you a lot of time and effort.
Swashbuckle also includes an embedded version of swagger-ui, which it will automatically serve up once installed, making it easier to test and explore your API endpoints.
To use Swashbuckle in your API App project, follow these steps:
- Go to https://swagger.io/swagger-ui/ and either download swagger or use the live demo.
- Enter the path to your API definition, such as https://wolfgangapi.azurewebsites.net/swagger/docs/v1.
By automating API discovery with Swashbuckle, you can streamline your development process and make it easier to maintain and document your APIs.
Hosting and Deployment
Hosting an Azure API App is a straightforward process. You can host it in Azure by following these steps: In Solution Explorer, right-click the project and select Publish, then select Azure and select the Next button.
To deploy your API App from Visual Studio, right-click on your project in the Solution Explorer and click Publish. In the Publish dialog, select the Create New option on the App Service tab and click Publish.
You can also create a new API App in the Azure Portal by selecting New > Web + Mobile > API App. Name it, choose a plan for hosting, select the required Resource Group, and click CREATE.
Here are the steps to deploy your API App to Azure:
- Right-click on your project in the Solution Explorer and click Publish.
- In the Publish dialog, select the Create New option on the App Service tab and click Publish.
- On the Create App Service blade, provide an App name, subscription, resource group, and hosting plan.
You can also use the Azure portal to deploy your API App. To do this, follow these steps: Go to the Azure Portal and select New > Web + Mobile > API App, name it, choose a plan for hosting, select the required Resource Group, and click CREATE.
To create a new API App, you can use Visual Studio, the Azure portal, Azure CLI, or PowerShell. You can also use the Azure API App template in Visual Studio to create a new API App project and add NuGet packages, such as Newtsonsoft.Json and Swashbuckle.
Take a look at this: Create Sample Azure Function for .net Frame Work
Monitoring and Management
Monitoring and Management is a crucial aspect of Azure API App. You can monitor the state of your Azure API App and Web App against the expected state using a Status Monitor or Threshold Monitor, which will send alerts through configured notification channels if the current state deviates from the expected state.
You can also monitor Azure API App and Web App on their metrics, such as CPU Time, Server Errors, and Memory Working Set, to understand their efficiency, reliability, and consumption. Data Monitoring can be configured for an extensive set of metrics, including Http Server Errors.
App Service provides built-in monitoring capabilities, like resource quotas and metrics, which can be used to set up alerts and automatic scaling. Additionally, Azure provides built-in diagnostics to assist with debugging.
Here's a list of some of the metrics you can monitor:
- CPU Time (Bytes)
- Server Errors (Count)
- Health Check Status (Count)
- Http 101 (Count), Data In (Bytes)
- Data Out (Bytes)
- Average Response Time (seconds)
- Response Time (seconds)
- Memory Working Set (Bytes)
- Average Memory Working Set (Bytes)
- IO other Bytes Per Second
- Gen 0 Garbage Collection (Count)
- Gen 1 Garbage Collection (Count)
- Gen 2 Garbage Collection (Count)
Resource limits of API Apps are defined by the App Service plan associated with the app. Exceeding these limits can cause your app to respond with a 403 HTTP error, application reset, or write operations to fail.
Security and Configuration
Security is a top priority when working with Azure API Apps. We can secure our apps with any standard identity provider like Azure AD.
Using Azure AD allows us to federate user authentication using popular SSO protocols such as OAuth. This provides a secure way for users to access our app.
To configure our Client App to use the Azure API App, we need to add the API URL to the environment files. This includes environment.ts and environment.prod.ts.
We also need to modify the getBaseUrl() method in the main.ts file to read the API URL from the environment file. This ensures our Client App can connect to the Azure API app to fetch data.
Configuring CORS in the Azure API App is also important for security and functionality. We can do this by navigating to the CORS menu under the API section of the Azure API Apps.
Worth a look: Azure Active Directory App Registration
Security
Security is a top priority for any system, and ours is no exception. We can secure our system with any standard identity provider like Azure AD.
Azure AD is a powerful tool for user authentication, and we can use it to federate user authentication using popular SSO protocols such as OAuth.
Take a look at this: Azure Active Directory Graph Api
Configuring the Client
To configure the client app to use the Azure API app, you'll need to add the API URL to the environment files, specifically environment.ts and environment.prod.ts.
The API URL should be added to these files so that the client app knows where to connect to. This is a crucial step in getting your client app up and running with the Azure API app.
In the main.ts file, you'll need to modify the getBaseUrl() method to read the API URL from the environment file. This ensures that the client app can dynamically retrieve the API URL, making it easier to switch between different environments.
Here's a simple step-by-step guide to configuring the client app:
- Add the API URL to environment.ts and environment.prod.ts files.
- Modify the getBaseUrl() method in main.ts to read the API URL from the environment file.
By following these steps, your client app will be configured to connect to the Azure API app, allowing you to fetch data and get started with your project.
Configuring CORS
Configuring CORS is a straightforward process that allows clients to access your API and fetch data. To do this, navigate to the CORS menu under the API section of the Azure API Apps.
In the CORS menu, add the URL of the Client App to the list of allowed origins. This will enable the client app to access the API and fetch data.
Here are the steps to enable CORS:
- In the Azure portal on your API App service, click on CORS under the API menu.
- On the CORS blade, enter the allowed origins or enter an asterisk to allow all origins.
- Click Save.
Once you've made this change, the client app will be able to access the API and fetch data. You've successfully configured the CORS policy for your Azure API App.
Service Level Agreement
A Service Level Agreement (SLA) is a contract between you and Azure that outlines the expected service availability and performance.
Reviewing the SLA for App Service is crucial to understand the uptime and maintenance schedules. The SLA is a promise that Azure will keep your App Service running and available to users.
The SLA for App Service guarantees a minimum of 99.95% uptime, excluding scheduled maintenance. This means that your App Service will be available to users at least 99.95% of the time.
Regularly reviewing the SLA can help you plan for downtime and make necessary adjustments to your application. It's also essential to understand the terms and conditions of the SLA to avoid any unexpected surprises.
Customization and Integration
Customization and Integration is a crucial aspect of Azure API Apps. You can customize the API gateway to meet your specific needs by adding custom policies and actions.
Azure API Apps allows for seamless integration with other Azure services, such as Azure Functions, Azure Logic Apps, and Azure Storage. This enables you to build complex workflows and scalable architectures.
With Azure API Apps, you can also integrate with on-premises systems and services using APIs, such as SAP, Oracle, and Salesforce. This opens up new possibilities for hybrid cloud integration.
Custom policies can be added to the API gateway to enforce security, rate limiting, and caching. For example, you can add a policy to limit the number of requests from a specific IP address.
Azure API Apps provides a visual designer for creating custom policies, making it easy to get started with customization. This designer allows you to drag and drop policy elements to create a custom policy.
Integration with other Azure services is also made easy through a visual designer, enabling you to create complex workflows without writing code. This designer provides a user-friendly interface for connecting services and creating workflows.
Check this out: Windows Azure Integration
Pricing and Plans
Azure API App offers a range of pricing plans to suit different needs and budgets.
The Free Plan is a great option for small projects or testing, with 1 GB of storage and 1 GB of RAM.
You can also opt for the D1 Shared Plan, which provides 1 GB of storage and 1 GB of RAM, with a shared core allocation of 240 CPU minutes per day.
One thing to note is that the Free Plan and D1 Shared Plan have no cost associated with them, as they are both free.
Broaden your view: Azure Logic App Blob Storage Trigger
Frequently Asked Questions
Does Azure have rest API?
Yes, Azure has a REST API that allows you to deploy resources directly. You can also use it to automate and manage your Azure resources programmatically.
What is the difference between Azure API Management and Azure App Service?
Azure API Management focuses on API creation and management, while Azure App Service is a platform for building, deploying, and scaling web apps and APIs with built-in infrastructure management. In short, API Management is for APIs, App Service is for web apps and APIs.
Sources
- https://turbo360.com/blog/azure-api-app-vs-web-app
- https://www.scholarhat.com/tutorial/azure/creating-api-apps-tutorial
- https://code-maze.com/deploying-aspnet-core-web-api-azure-api-apps/
- https://programmingwithwolfgang.com/design-azure-app-service-api-apps/
- https://azure.microsoft.com/en-us/pricing/details/app-service/windows/
Featured Images: pexels.com