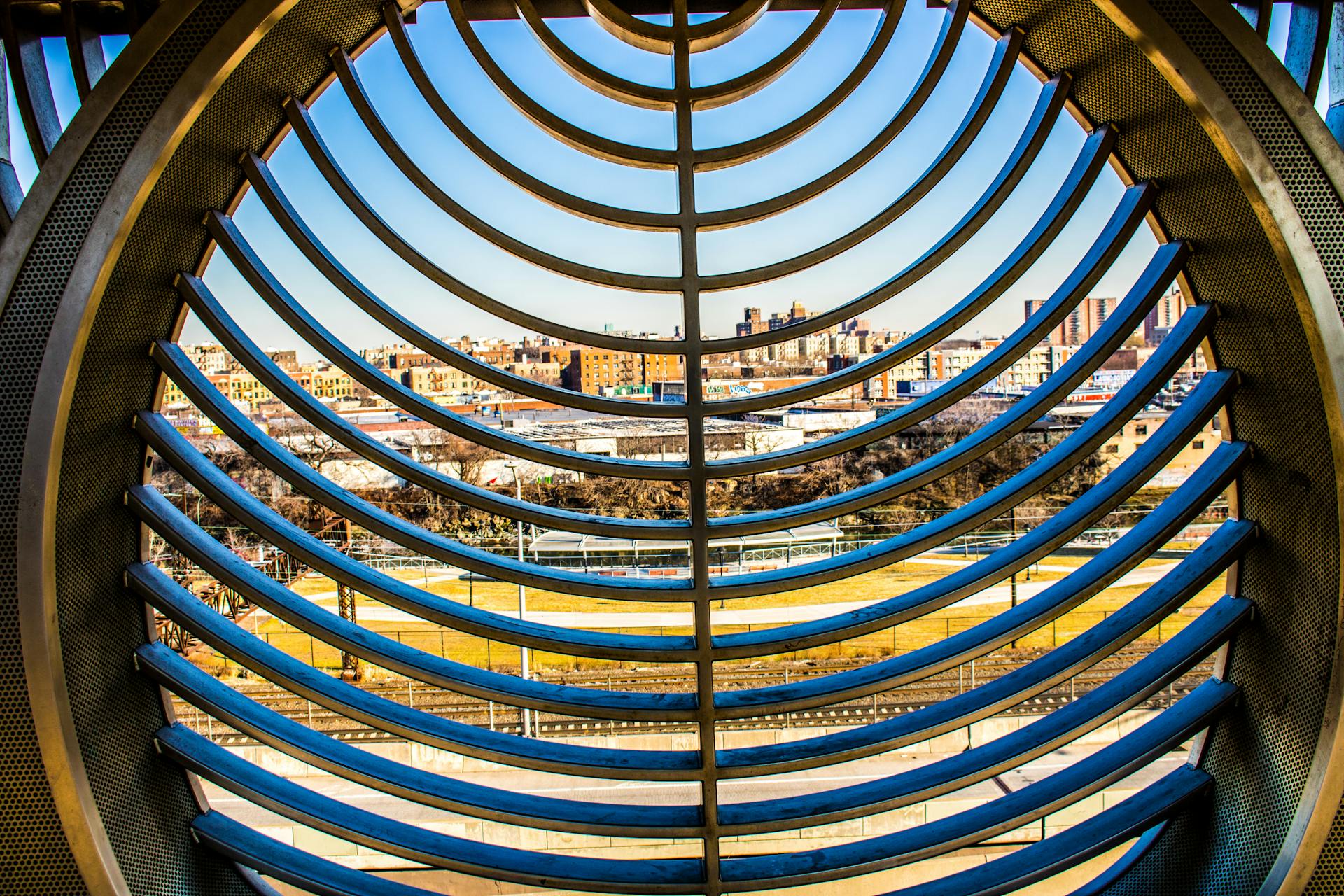
CSS Grid is a powerful layout system that allows you to control the order of items in a grid.
Grid items can be ordered horizontally or vertically using the grid-template-areas property, which defines the areas of the grid.
Grid items can also be ordered using the grid-template-columns and grid-template-rows properties, which define the number of columns and rows in the grid.
To change the order of grid items, you can use the grid-column and grid-row properties, which specify the position of an item in the grid.
The grid-template-columns property can also be used to define the width of each column in the grid.
For your interest: Css Grid Align Items
Grid Basics
Most browsers shipped native support for CSS Grid as of March 2017, making it a great time to start using it.
You can define a container element as a grid with the display: grid property.
To set the column and row sizes, you'll need to use grid-template-columns and grid-template-rows.
The source order of the grid items doesn't matter, which means you can place them in any order you like.
You can rearrange your grid with media queries using just a couple lines of CSS.
A fresh viewpoint: Grid Template Areas Css
Grid Properties
Grid properties are used to define the layout of grid items.
Grid container properties, such as grid-template-columns and grid-template-rows, determine the number and size of grid tracks.
Grid item properties, like grid-column and grid-row, specify the position of each item within the grid.
These properties can be used to create complex grid layouts by nesting grids within each other.
Recommended read: Css Grid Properties
Grid-Template-Columns
Grid-template-columns is a crucial property for defining the columns of a grid. It's a space-separated list of values that represent the track size, with the space between them representing the grid line.
You can use a length, percentage, or the fr unit to specify the track size. The fr unit allows you to set the size of a track as a fraction of the free space of the grid container.
For example, using the repeat() notation can help streamline your definition if you have repeating parts. This notation is equivalent to writing out the same values multiple times.
If this caught your attention, see: Css Grid Fr
If multiple lines share the same name, you can reference them by their line name and count. This can be a big time-saver when working with complex grids.
Here are some examples of using the fr unit to set the size of tracks as a fraction of the free space:
- 1fr: one track is one third the width of the grid container
- 2fr 1fr: two tracks are one third and one sixth the width of the grid container, respectively
Column-Gap
Column-gap is the space between columns in a grid container. It's a crucial property to understand when designing layouts.
The column-gap property can take on any unit value, including pixels, ems, and rems. For example, a column-gap of 20px means there will be a 20-pixel gap between each column.
A column-gap of 0 means there will be no gap between columns. This is useful for creating a seamless layout.
Worth a look: 2 Column Grid Css
Row-Gap
Row-gap is a property that controls the gap between rows in a grid container. It can be set using the grid-row-gap or row-gap shorthand property.
Setting a row-gap can help improve the readability of your grid layout by creating a clear distinction between rows. For example, a row-gap of 10px can add a 10px gap between each row.
The row-gap property can be set in terms of length values, such as pixels or percentage.
A unique perspective: Css Grid Spacing
Grid-Column-Gap
Grid-Column-Gap is a property that allows you to add space between grid columns.
This property is useful for creating a more visually appealing grid layout, especially when you have multiple columns of content.
You can set the Grid-Column-Gap property to a specific length, such as 10px or 20px, to add space between each column.
For example, if you set Grid-Column-Gap to 20px, there will be 20px of space between each column.
This means that if you have three columns, there will be 40px of space between them, because 20px x 2 = 40px.
You can also use the Grid-Column-Gap property to add space between columns on the left and right sides of the grid container, by setting the Grid-Column-Gap shorthand property to a value with two values, such as 10px 20px.
Intriguing read: Css Grid Width
Grid Order
Grid order is not exclusive to Grid, it can also be used with flexbox. It allows us to change the order of individual items within the flow.
Items have a default order of 0. Changing an auto-placed item's order to -1 will place it at the start of the grid auto-placed grid items – after explicitly placed items, if there are any placed at the start of the grid.
The order property will only affect auto and semi-auto placed items (those using a single span value).
A fresh viewpoint: Css Grid Auto Fit
Grid Container
The grid container is the foundation of a grid layout. It's the element where you apply the display: grid property.
In this setup, the grid container is the direct parent of all the grid items. This means that all the items inside it are considered grid items.
A grid container can have multiple grid items, but it's essential to note that the grid items are direct children of the grid container. This is a crucial aspect of grid order.
Take a look at this: Css Grid Container
Grid-Column
Grid-column is a powerful tool in CSS Grid Order. grid-column-end disables the default behavior of the last column in a row aligning to the opposite edge. This is especially useful when you want to control the alignment of your grid items.
You can use grid-column-end in combination with grid-column to create a more complex layout. For example, you can use grid-column-end to specify that a grid item should end at a specific column, while also using grid-column to specify the start column.
grid-column and grid-row are shorthand properties that combine the longhand versions of grid-column-start and grid-column-end, and grid-row-start and grid-row-end, respectively. They use the same syntax as the longhand versions, with the same values accepted, including span.
If you don't specify an end line value, grid-column will span 1 track by default. This means that if you only specify a start line value, the grid item will take up one column.
Curious to learn more? Check out: Css Grid Row Span
Grid-Row-Gap
Grid-row-gap is a property that allows you to set the size of the grid lines between rows. You can think of it like setting the width of the gutters between the rows.
The size of the grid lines can be specified with a length value, such as 10px or 1em. This will create a gutter between the rows, but not on the outer edges.
The unprefixed property row-gap is already supported in some modern browsers, including Chrome 68+, Safari 11.2 Release 50+, and Opera 54+.
A fresh viewpoint: Css Grid Rows Auto
Grid-Auto-Flow
Grid-auto-flow is a property that controls how the auto-placement algorithm works, especially when you don't explicitly place grid items on the grid. It determines the order in which auto-placed items are arranged.
There are three values for grid-auto-flow: row, column, and dense. The default value is row, which tells the auto-placement algorithm to fill in each row in turn, adding new rows as necessary. This means that if you have a grid with five columns and two rows, and you only specify spots for two items, the remaining items will be placed across the available rows.
If you set grid-auto-flow to column, the auto-placement algorithm will fill in each column in turn, adding new columns as necessary. This can result in a different visual order of your items, which might cause them to appear out of order, affecting accessibility.
Here's a summary of the grid-auto-flow values:
- row: fills in each row in turn, adding new rows as necessary (default)
- column: fills in each column in turn, adding new columns as necessary
- dense: attempts to fill in holes earlier in the grid if smaller items come up later
Note that dense only changes the visual order of your items and might cause them to appear out of order, which is bad for accessibility.
Grid Template
Grid Template is a powerful feature of CSS Grid that allows you to define areas on the grid and reference them to position items.
You can name these areas in the grid container, kind of like drawing them out, and space them out for readability. For example, we'll need to name four areas: header, main content, sidebar, and footer.
These areas can be defined in the grid container, and they can be referenced later to position items. The fr units used for the container need to be adjusted for the header, so let's set grid-template-columns to 1fr and 1fr to create two equally sized columns.
Here are the four areas we'll need to name, listed out for reference:
- header
- main content
- sidebar
- footer
The full-width footer does not need different columns set since the content is in the middle, so we can leave it as is.
Grid Units and Functions
Fractional units in CSS Grid, like 1fr, mean "portion of the remaining space". This makes them super flexible and friendly in combination with other units.
You can use fractional units to define columns, and they're especially useful when you need to distribute space evenly.
For example, a declaration like 1fr 3fr means 25% 75% of the remaining space, but unlike percentage values, fractional units are much more forgiving.
You might like: Css Grid Extra Space
Fr Units
Fr units are a type of unit in CSS Grid that represent a portion of the remaining space.
They essentially mean "portion of the remaining space" and are much more flexible than fixed percentage values.
Fractional units are friendly in combination with other units, making them a great choice for layouts where you need to adjust the size of elements based on their content.
You'll likely end up using a lot of fractional units in CSS Grid, like 1fr.
Check this out: Css Grid Space between
Repeat() Function
The repeat() function is a game-changer when it comes to saving typing. It can be used to repeat a value or a string a specified number of times.
One of the most useful features of the repeat() function is its ability to work in conjunction with keywords like auto-fill and auto-fit.
Auto-fill allows you to fit as many possible columns as possible on a row, even if they are empty. This is especially useful when you have a lot of data to display.
Curious to learn more? Check out: Css Grid Automatic Columns
Auto-fit, on the other hand, fits whatever columns there are into the space, preferring to expand columns that already have content rather than empty ones.
Here's a quick rundown of the differences between auto-fill and auto-fit:
- auto-fill: Fit as many possible columns as possible on a row, even if they are empty.
- auto-fit: Fit whatever columns there are into the space. Prefer expanding columns to fill space rather than empty columns.
Grid Alignment and Flow
Grid alignment and flow are crucial aspects of CSS grid order. A good way to think about grid flow is to imagine it as a flowing river, where explicitly placed items are boats anchored in the river, and auto-placed items flow around them from left to right.
Items placed using a span value alone will still flow like others, but they'll be restricted by their own explicit size. This is because they're semi-auto placed.
The grid placement algorithm resolves item placement in a specific order, which affects how items are placed. For example, if we only place an item on the column axis using span, successive items will be placed after it. This can create an implicit track if there are enough items to fill the grid exactly.
Expand your knowledge: Css Grid Flow
Here are the alignment options for align-content:
- start – aligns the grid to be flush with the start edge of the grid container
- end – aligns the grid to be flush with the end edge of the grid container
- center – aligns the grid in the center of the grid container
- stretch – resizes the grid items to allow the grid to fill the full height of the grid container
- space-around – places an even amount of space between each grid item, with half-sized spaces on the far ends
- space-between – places an even amount of space between each grid item, with no space at the far ends
- space-evenly – places an even amount of space between each grid item, including the far ends
Understanding Flow
Think of a grid as a flowing river, where explicitly placed items are boats anchored in the river, with auto-placed items flowing around them from left to right.
Any explicitly placed items will have a significant impact on the grid's flow, and items that are only explicitly placed on one axis will participate in the grid flow on the remaining axis.
An item with a span of 2 will flow onto the next row if there are less than 2 grid columns available, making it behave like a semi-auto placed item.
Items placed using a span value alone will still flow like the others, but they'll be restricted by their own explicit size.
If we place an item on the column axis using span, successive items will be placed after it, and if we have enough items to fill the grid exactly, they'll create an implicit track.
This is because of the order in which item placement is resolved in the grid placement algorithm.
Suggestion: Flexbox Css 2 Columns
Align-Content
Aligning the grid within its container can be tricky, especially when the total size of the grid is less than the size of its container. This is because the grid items are sized with non-flexible units like pixels (px), which means they won't automatically resize to fit the container.
To fix this, you can use the align-content property, which aligns the grid along the block (column) axis. This is different from justify-content, which aligns the grid along the inline (row) axis.
You can choose from a few options to align the grid: start, end, center, stretch, space-around, space-between, or space-evenly. Each of these options has a specific effect on the grid's alignment.
Here are the details of each option:
Grid with Caution
Grid properties that affect the visual order of grid items should be used with caution, as they don't change the source order and can adversely affect accessibility.
Rachel Andrew has written extensively about the importance of accessibility in grid layout, specifically regarding content re-ordering.
In my experience, explicit item placement is often the reason for visual re-ordering, not the default behavior of grid layout.
Properties like grid-row and grid-column can be used to reorder grid items visually, but be aware of the potential accessibility issues.
Accessibility
Accessibility is crucial when working with CSS Grid. Re-ordering content can cause issues, especially in two dimensions.
Grid, Flexbox, and absolute positioning can all cause problems if not used carefully. The order of items in the source document is followed by screen readers and keyboard-only navigators.
Be particularly careful when using flex-direction to reverse the order, the order property, or placement of Grid items that moves them out of logical order. Dense packing mode of grid-auto-flow can also cause issues.
For more information, check out these resources:
- Grid Layout and Accessibility - MDN
- Flexbox and the keyboard navigation disconnect
Sources
- https://css-irl.info/debugging-css-grid-part-3-auto-flow-order-and-item-placement/
- https://wpengine.com/resources/combine-flexbox-and-css-grids-for-layouts-how-to/
- https://get.foundation/sites/docs/grid.html
- https://css-tricks.com/snippets/css/complete-guide-grid/
- https://www.smashingmagazine.com/2018/04/best-practices-grid-layout/
Featured Images: pexels.com