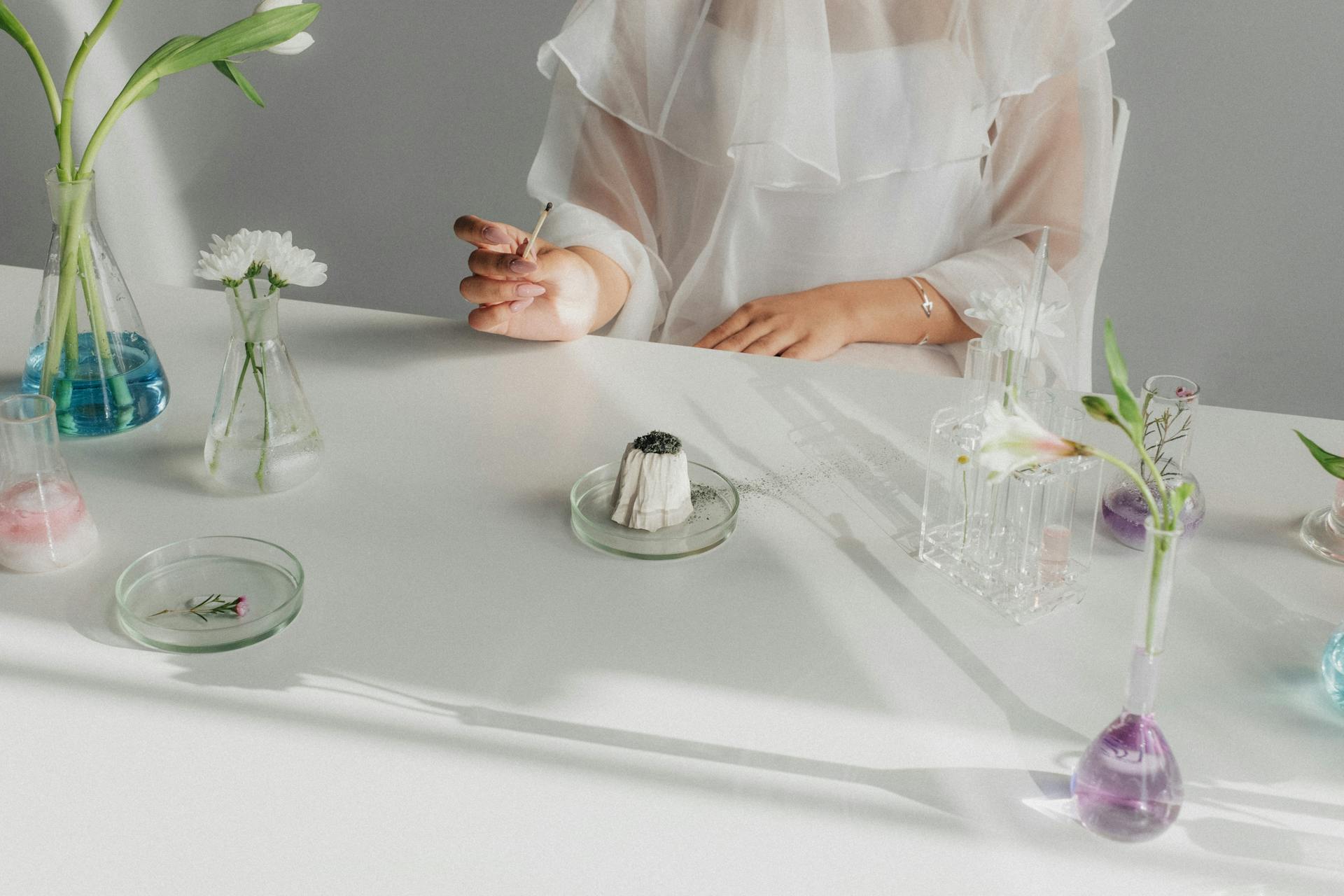
Flask is a lightweight Python web framework that's perfect for building web applications. It's ideal for small to medium-sized projects, and its simplicity makes it easy to learn and use.
Flask's flexibility is one of its greatest strengths, allowing developers to build applications that fit their specific needs. With Flask, you can choose from a variety of libraries and tools to create a robust and scalable web application.
A Flask web application typically consists of a single file, often called the "app file", that contains all the necessary code. This file is usually named `app.py` and serves as the entry point for your application.
To get started with Flask, you'll need to install the framework and a web server, such as Gunicorn or uWSGI. This will allow you to run your application and test its functionality.
You might like: Css in Html File
What is Flask?
Flask is an API of Python that allows us to build web applications. It was developed by Armin Ronacher. Flask's framework is more explicit than Django's framework and is also easier to learn because it has less base code to implement a simple web application.
Additional reading: What Is Django Software
Flask is built on top of the Werkzeug WSGI toolkit, which provides web server functionality needed for handling requests and responses, and the Jinja2 templating engine, which gives Flask the ability to handle HTML templates.
Some of the key features of Flask include a simple API for creating web routes and handling requests, support for HTML templates through the Jinja templating engine, and the ability to be highly extendable through third-party extensions.
See what others are reading: Azure Flask
What is?
Flask is a Python API that allows us to build web applications.
It was developed by Armin Ronacher, and its framework is more explicit than Django's, making it easier to learn and implement a simple web application.
Flask is a micro web framework written in Python that's used for developing web applications. It's built on a simple philosophy of keeping things simple and lightweight.
Flask is built on top of the Werkzeug WSGI toolkit, which provides web server functionality needed for handling requests and responses.
For more insights, see: Simple Personal Githubn Tool Web Dev
Here are some of the key features of Flask:
- It has a simple API for creating web routes and handling requests.
- The Jinja templating engine offers support for HTML templates, allowing developers to create web pages easily.
- It's highly extendable, due to its support for third-party extensions.
- It comes bundled with a development server that makes it easy to test and debug apps.
Flask provides a powerful, flexible, yet simple framework for building web applications.
Features
Flask is a micro web framework that offers a range of features to make development easier and more efficient.
It comes with a development server and debugger, which allows you to quickly test and iterate on your code.
Integrated support for unit testing is also included, making it easier to catch and fix bugs early on.
Flask uses Jinja templating, which is a powerful templating engine that makes it easy to separate presentation logic from application logic.
The framework supports secure cookies, also known as client-side sessions, to store and manage user data.
It's 100% WSGI 1.0 compliant, which means it can be easily integrated with other WSGI-compatible applications.
Flask is also Unicode-based, making it suitable for applications that require support for multiple languages.
Here are some of the key features of Flask:
- Development server and debugger
- Integrated support for unit testing
- RESTful request dispatching
- Uses Jinja templating
- Support for secure cookies (client side sessions)
- 100% WSGI 1.0 compliant
- Unicode-based
- Complete documentation
- Google App Engine compatibility
- Extensions available to extend functionality
Getting Started
To start with Flask, Python 3 is required for installation. You can import Flask from the Flask Python package on any Python IDE. To test if the installation is working, check out the code given below.
The '/ URL is bound with the hello() function. When the home page of the webserver is opened in the browser, the output of this function will be rendered accordingly.
To start a Flask application, you can use the run() function. However, this method should be restarted manually for any change in the code. To overcome this, the debug support is enabled so as to track any error.
You can also explore the Flask Quick Start guide, which equips you with the skills needed to excel in Flask development. The guide covers essential topics such as creating the first simple application, running a Flask application, and using Flask routes.
Here are some key topics covered in the Flask Quick Start guide:
- Creating the first simple application
- Running a Flask Application
- Flask Routes
- Flask Models
- Flask – HTTP Method
- Flask – Variable Rules
- Redirects and URL
- Redirect & Errors
- Change Port in Flask app
- Changing Host IP Address in Flask
These topics provide a solid foundation for building your first Flask application. Remember to set up your environment correctly, including setting the FLASK_APP environment variable, to ensure smooth development.
Building Routes
Building routes in Flask is a crucial part of web development, allowing users to access specific web pages directly without navigating from the Home page.
The route() decorator is used to bind a URL to a function, so when a user visits that URL, the associated view function is triggered to handle the request. This is useful for accessing web pages directly.
You can also use the add_url_rule() function of an application object to bind a URL with a function, as shown in the example of the hello_world() function being rendered in the browser when visiting http://localhost:5000/hello.
The url_for() function allows for dynamic building of URLs for specific functions, accepting the name of the function as the first argument and one or more keyword arguments.
Building Routes in Python
Building Routes in Python is a crucial step in creating web applications. It allows users to access web pages directly without navigating from the Home page.
The routing technique is provided by web frameworks so that users can remember URLs. It's done through the route() decorator, which binds the URL to a function.
To bind a URL to a function, you can use the route() decorator or the add_url_rule() function of an application object. For example, visiting http://localhost:5000/hello will render the output of the hello_world() function in the browser.
Most web applications have multiple URLs, so we need to have a way of knowing which function handles which URL. In Flask, this mapping is known as routing.
The route() decorator binds a URL to a view function, so when a user visits a URL that exists on our application, Flask triggers the associated view function to handle the request. For instance, when the application receives a request for the about URL (/about), Flask calls the about() function.
You can specify the HTTP method as an optional argument to the route() decorator to handle different types of requests. For example, we can define a route that handles a PUT request to update user details given their user_id.
A fresh viewpoint: Cloudfront Aws Webflow Example
Sending Form Data to Server
A form in HTML is used to collect information from users, which is then forwarded and stored on the server. The Python with Flask provides this facility by using the URL rule.
To send form data to the server, you can use the URL rule to render a web page with a form, and then post the data to another URL that triggers a function to collect the form data. The function can collect form data present in request.form in a dictionary object.
The data filled in the form is posted to the ‘/result’ URL which triggers the result() function. This function can then send the form data for rendering to result.html.
Flask provides built-in support for processing HTML forms and handling user input. To use forms in Flask, you need to define a form class that will inherit from flask_wtf.FlaskForm.
See what others are reading: How to Use Inspect Element to Find Answers
Variables and Data
Variables in Flask allow you to build URLs dynamically by adding variable parts to the rule parameter.
You can mark variable parts with a slash and they'll be passed as keyword arguments to your function. This is done by attaching the variable part to the URL in the route() decorator, like in the example where the URL '/hello/GeeksforGeeks' passes 'GeeksforGeeks' to the hello() function as an argument.
Flask supports various data types for variable parts, including int, float, and path, which can handle directory separator channels and take slashes.
Variables
Variables in web development can be a powerful tool. Flask, a popular Python web framework, allows you to build URLs dynamically by adding variable parts to the rule parameter.
You can mark variable parts in the URL rule with a parameter, which is passed as a keyword argument to your function. For instance, in the example code, the URL http://localhost:5000/hello/GeeksforGeeks would pass 'GeeksforGeeks' to the hello() function as an argument.
Flask supports various data types for variable parts, including string, int, float, and path. The path data type is useful for directory separators, allowing you to use a slash in your URL rule.
The URL rules in Flask are based on Werkzeug's routing module, ensuring that the generated URLs are unique and follow established precedents. This is evident in the example code, where the URL rule is formed based on the parameter attached to the '/hello' path.
You might enjoy: Data Text Html Charset Utf 8 Base64
Forms and Validation
Flask Forms are based on the WTForms library, which provides a flexible and powerful way to handle form data and perform validations.
To use forms in Flask, we need to define a form class that inherits from flask_wtf.FlaskForm. This class will contain the fields that are going to be on the form and any validation rules that should be applied to them.
The WTForms library isn't a part of the standard Flask installation, so we need to install it using a specific command.
We define a form class with fields like email and password, and a submit button. We also have a validators argument to specify validation rules for each field.
For example, we can require the email field to contain a valid email address and the password field to contain a password of no fewer than six characters.
Once we've defined the form class, we can use it in the view function to render the form and process the form data submitted by the user.
For more insights, see: Webflow Password Protect
The form can be rendered as an HTML form using the render_template method, and when a user submits the form we check if the form is valid using the validate_on_submit method.
If the form is valid, we access the email and password fields. The form also includes a csrf_token field to prevent cross-site request forgery (CSRF) attacks.
HTTP and URLs
HTTP and URLs are essential components of a Flask web application. In Flask, you can build URLs dynamically using the `url_for()` function, which accepts the name of a function as its first argument and one or more keyword arguments.
To send data to a server without encryption, you can use the GET HTTP method. This method is useful for retrieving data from a server without modifying it.
Flask also supports other HTTP methods, including POST, PUT, and DELETE. These methods allow you to send data to a server and perform actions such as creating, updating, and deleting resources.
Related reading: Web Server Programming
Here's a quick rundown of the HTTP methods supported by Flask:
Remember, each HTTP method has its own use case, and choosing the right one is crucial for building a robust and scalable web application.
Build a URL
Building a URL is an essential part of creating a web application, and Flask provides a convenient way to do it.
In Flask, you can use the `url_for()` function to build a URL for a specific function. This function accepts the name of the function as its first argument, along with one or more keyword arguments.
To make a URL more memorable and accessible, you can use the `route()` decorator to bind a URL to a function. This way, when a user visits a specific URL, the corresponding function will be executed.
The `add_url_rule()` function of an application object can also be used to bind a URL with a function. This is demonstrated by binding the URL http://localhost:5000/hello to the `hello_world()` function.
HTTP Methods
HTTP methods are the foundation of how your web application interacts with the server.
The Flask web framework supports various HTTP methods, including GET, HEAD, POST, PUT, and DELETE.
GET is used to send data in a form to the server without encryption.
HEAD provides a response body to the form, similar to GET.
POST sends form data to the server, and the data received is not cached by the server.
PUT replaces the current representation of a target resource with a URL.
DELETE deletes the target resource of a given URL.
Here's a summary of the HTTP methods supported by Flask:
Frequently Asked Questions
Is Flask a frontend or backend?
Flask is a Python framework used for backend development, ideal for building scalable and robust web applications. It's a key tool for developers working on the server-side of web development.
Is Flask better than Django?
Flask is a more flexible choice for web app development, ideal for experienced programmers seeking control over app design. It's particularly suited for those who value adaptability over a more structured approach.
Is Flask good for making websites?
Yes, Flask is a great choice for building websites, offering flexibility and scalability for projects of any size. From simple sites to complex enterprise-level systems, Flask has got you covered.
Featured Images: pexels.com