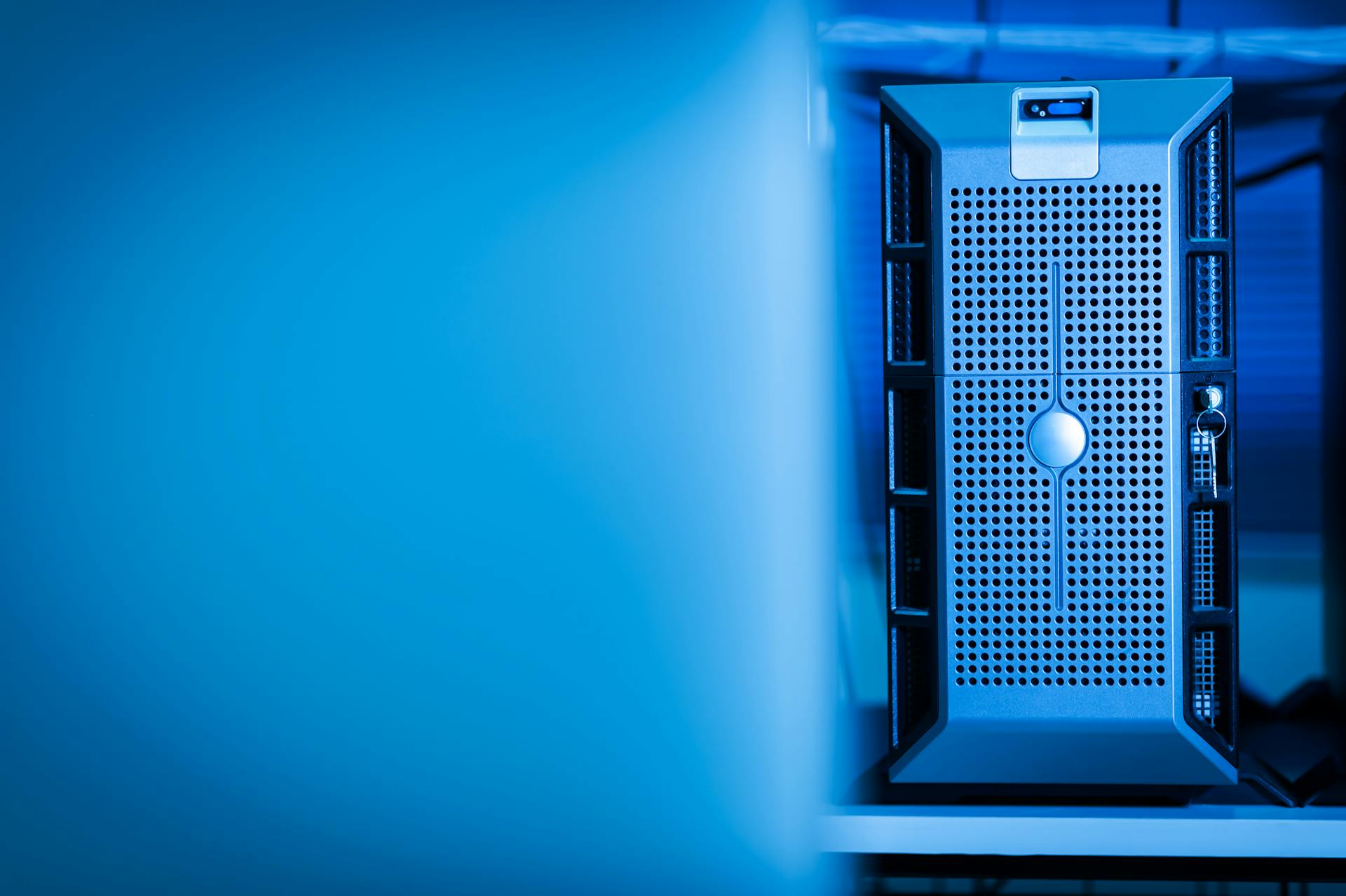
Getting Started with Google Cloud Storage in Python is a breeze, thanks to the `from google.cloud import storage` module. This module allows you to interact with Google Cloud Storage buckets and objects directly from your Python code.
To begin, you'll need to install the Google Cloud Client Library, which can be done using pip: `pip install google-cloud-storage`. This library provides a simple and intuitive API for working with Google Cloud Storage.
You can authenticate with Google Cloud Storage by using the `GOOGLE_APPLICATION_CREDENTIALS` environment variable, which should be set to the path of your JSON key file. Alternatively, you can use the `Client` class to create a client instance and authenticate programmatically.
Take a look at this: How to Upload File to Google Cloud Storage Using Reactjs
Bucket Management
To work with a bucket, you need to make a bucket handle, which is a reference to a bucket. This handle can exist even if the bucket doesn't exist yet.
You can create a bucket in Google Cloud Storage by calling BucketHandle.Create, and this allows you to set the initial BucketAttrs of a bucket. The third argument to BucketHandle.Create is used for this purpose.
Additional reading: Google Cloud Storage Bucket
Each bucket has associated metadata, represented in this package by BucketAttrs. This metadata can be retrieved using BucketHandle.Attrs.
To list the buckets in a project, you can use the Buckets function, which returns an iterator over the buckets. You can optionally set the iterator's Prefix field to restrict the list to buckets whose names begin with the prefix.
A bucket handle can be obtained using the Bucket function of the Client, which returns a BucketHandle. This handle provides operations on the named bucket.
The name of a bucket must contain only lowercase letters, numbers, dashes, underscores, and dots. The full specification for valid bucket names can be found at a specific URL.
You might enjoy: Google Cloud Bucket How to Store Index File
Object Management
Object Management with Google Cloud Storage is a breeze. You can retrieve an ObjectHandle, which provides operations on a named object, using the Object method of a BucketHandle.
To get a handle on an object, you must provide a valid UTF-8-encoded name. The full specification for valid object names can be found at the Google Cloud Storage documentation.
You can also manage the lifecycle of your objects by setting up an object lifecycle policy. This allows you to automatically archive or delete stale files after a certain amount of time.
Related reading: Object Storage Google
Uploading Objects
You can upload objects to Google Cloud Storage (GCS) using the command `gsutil cp -n object.txt gs://my-bucket/`, but a more straightforward way is to use the command `gsutil cp object.txt gs://my-bucket/`.
To upload an object, you need to use the `gsutil cp` command, which copies a file from a local directory to a GCS bucket.
To upload an object to gcs, use the following command: `gsutil cp object.txt gs://my-bucket/`.
GCS supports pay-per-use services, and apart from Cloud Storage, GCP offers many other services like hosting your websites in the cloud and running your own Operation System from the Cloud.
Here are the steps to upload an object to gcs:
- Use the gsutil cp command to upload the object to gcs.
You can also use the `gsutil` command to upload objects to GCS, but it's essential to note that GCP offers many other services like hosting your websites in the cloud and running your own Operation System from the Cloud.
When you upload an object to gcs, you can specify the bucket name, and the object will be stored in that bucket.
Bucket Website
Bucket Website is a crucial aspect of object management, allowing you to control how your bucket behaves when accessed as a website.
The BucketWebsite configuration holds the bucket's website settings, which determine the service's behavior when displaying bucket contents online.
You can access more information about BucketWebsite and how to configure it by visiting https://cloud.google.com/storage/docs/static-website.
This configuration is especially useful for hosting static websites, making it easy to serve your content directly from your bucket without needing a separate web server.
By controlling how your bucket behaves as a website, you can create a seamless user experience and improve the overall performance of your online presence.
Objects
Objects are a fundamental part of a Google Cloud Storage bucket.
To work with objects in a bucket, you can use the Object method of a BucketHandle, which returns an ObjectHandle that provides operations on the named object. This call does not perform any network operations, so you'll need to use methods on the ObjectHandle to actually fetch or verify the object's existence.
For more insights, see: Activar Vpn Google One
The name of the object must consist entirely of valid UTF-8-encoded runes, and you can find the full specification for valid object names in the documentation.
If you need to retrieve a list of objects in a bucket, you can use the Objects method of a BucketHandle, which returns an iterator over the objects that match a given Query. If the Query is nil, no filtering is done, and the objects will be iterated over lexicographically by name.
Object Versioning
Object Versioning is a lifesaver for accidental deletions - it automatically backs up your data so you can restore it if needed.
You can enable Object Versioning using the gsutil versioning set command.
If you've deleted something by accident, don't panic - Cloud Storage always picks up the most recent version, so you can restore an object using one of the other APIs or tools.
To make any existing App Engine objects available in Firebase, you'll need to set the default access control on your objects to allow Firebase to access them by running the following command using gsutil.
Related reading: Google Cloud Storage vs Firebase Storage
Object Lifecycle Management
Object Lifecycle Management is a game-changer for applications that deal with temporary files.
Google Cloud Storage offers a feature called Object Lifecycle Management, which allows you to automatically delete or archive objects after a certain amount of time.
This feature is super useful for applications that don't need to store files long-term, like photo sharing apps.
For example, you can set up an object lifecycle policy to delete all photos within one day.
To deploy this policy, you'll use the gsutil lifecycle set command.
Be aware that this policy applies to all files in the bucket, so if you're storing important user backups alongside temporary files, you might want to use two separate buckets or perform deletions manually.
Additional reading: Dropbox Camera Upload Delete from Phone
Manifest Json
The manifest JSON is a crucial component of our object management system. It's a compact representation of the data exposed, located in each data request bucket.
The manifest JSON file contains URLs to all CRAMs, their indexes, and RNASeq fastq in the request. It also grants the account read access on each object Access Control List (ACL).
You can easily parse the manifest JSON using a script or program, or even read it in an editor. The manifest gives you the following information:
- The unique ID of the data request
- The accounts which have access to the data in the manifest
- The Google Cloud Storage (GCS) urls of the aforementioned TAR files
- For each sample in the data request
JSON has good support in most programming languages, including Python. You can load the manifest straight from GCS into a dictionary in just a few lines.
The intent of the manifest is to enable the use of GCP to scale analysis horizontally across virtual machines and avoid large downloads. This is especially useful for Hartwig Medical Foundation, which often follows a pattern of creating a VM with a predefined startup script.
Within the startup script, you can download the data you need, run your analysis, upload the results to your own bucket, and then terminate the VM.
Authentication and Access Control
ACLs (Access Control Lists) are a powerful tool for fine-grained control over access to your Google Cloud Storage objects and buckets. They allow you to specify the role of a user, group, or project for each ACLRule.
A unique perspective: How Do I Access My Google Cloud Storage
To manage ACLs, you can obtain an ACLHandle and call ACLHandle.List to list the ACLs of a bucket or object. You can also set and delete ACLs using the same handle.
IAM (Identity and Access Management) provides an alternative way to control access at the project level, which might be more suitable for your needs.
Bucket Logging
Bucket Logging plays a crucial role in tracking and monitoring activities within a bucket. BucketLogging holds the bucket's logging configuration, which defines the destination bucket and optional name prefix for the current bucket's logs.
Logging configuration is a vital aspect of bucket management, allowing you to store and manage logs in a separate bucket. The destination bucket is specified in the logging configuration.
The logging configuration also includes an optional name prefix, which can be used to identify the logs of the current bucket. This prefix can be useful for organization and filtering purposes.
ACLs
ACLs are a powerful tool for fine-grained control, allowing you to specify the role of a user, group, or project for each object or bucket.
Both objects and buckets have ACLs, which are lists of ACRLRules. You can list the ACLs of a bucket or object by obtaining an ACLHandle and calling ACLHandle.List.
ACLs are suitable for situations where you need more control than what IAM provides at the project level. However, you may prefer using IAM to control access at the project level.
You can also set and delete ACLs, giving you the flexibility to adjust access as needed.
For more insights, see: Block Level Storage
Iam
IAM provides access to IAM access control for the bucket. This means you can manage who has access to your bucket and what actions they can perform.
IAM access control is a powerful tool that lets you grant or deny access to your bucket based on user identity, permissions, and policies. You can use IAM to control access to your bucket in a fine-grained way.
For example, you can use IAM to grant a user read-only access to your bucket, while denying them the ability to delete or modify its contents. This way, you can ensure that sensitive data is protected from unauthorized access.
IAM access control is an essential part of maintaining the security and integrity of your bucket.
Hmac Key
Hmac Key is a crucial component of authentication and access control in Google Cloud Storage.
HMAC keys are used to authenticate signed access to objects.
To enable HMAC key authentication, you should visit https://cloud.google.com/storage/docs/migrating.
HMAC keys can be created using the CreateHMACKey function, which invokes an RPC for Google Cloud Storage.
Note that gRPC is not supported for this function.
UserProject
UserProject is a crucial concept in authentication and access control. It allows you to specify a project ID that will be billed for all subsequent calls.
A user project is required for all operations on Requester Pays buckets. This is because calls with a user project will be billed to that project rather than to the bucket's owning project.
To use a user project, you can call the UserProject method on a BucketHandle. This will return a new BucketHandle that passes the project ID as the user project for all subsequent calls.
This is useful when you need to perform operations on a Requester Pays bucket and you want to be billed to a specific project.
On a similar theme: If I Delete Onedrive Will It Erase Everything
Notifications
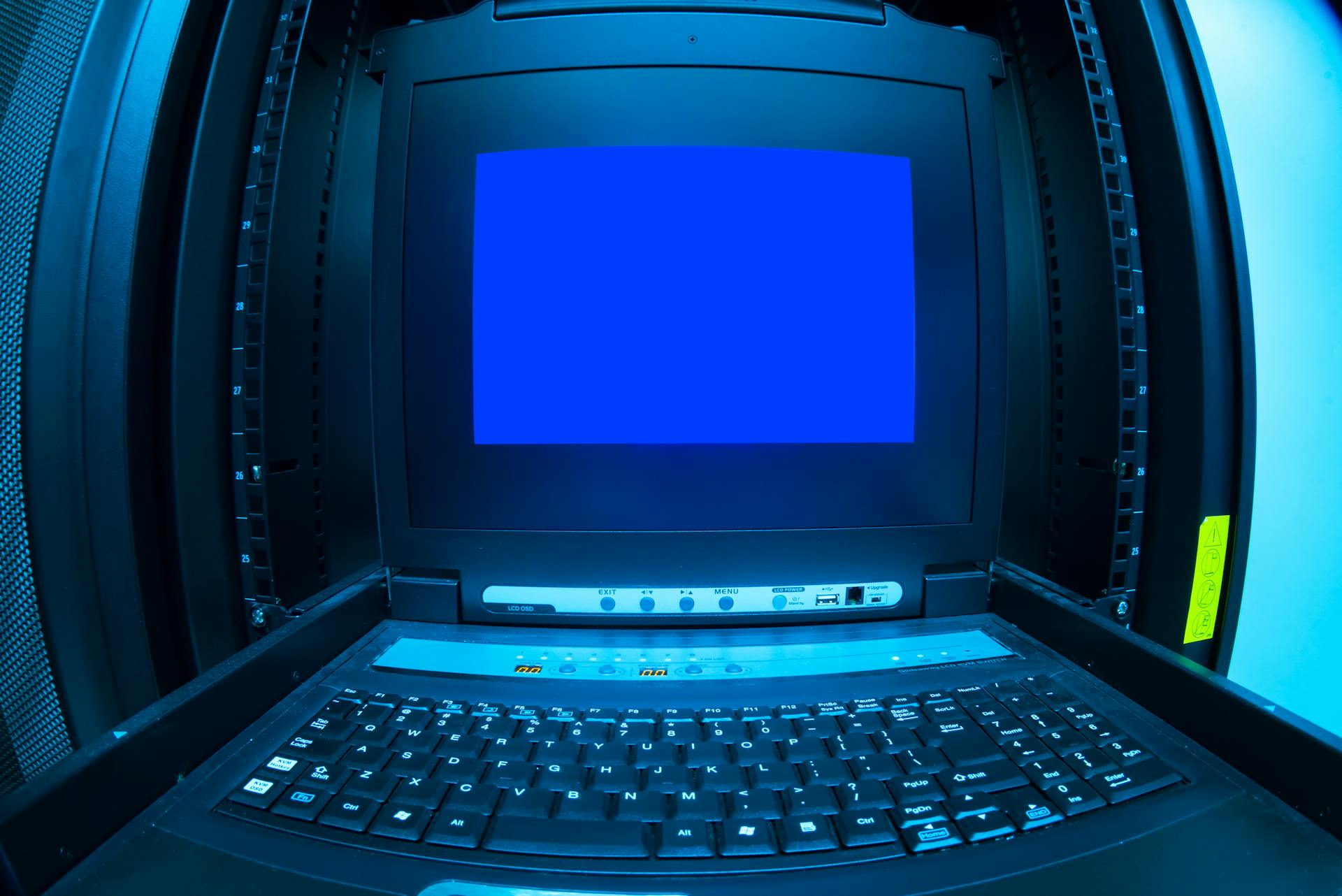
Notifications play a crucial role in ensuring data integrity and security. They allow you to be notified when specific events occur in your bucket, such as data updates or deletions.
In the context of Authentication and Access Control, notifications are configured for a bucket, which returns all notifications as a map indexed by notification ID. This is particularly useful for keeping track of changes to your data.
Note that gRPC is not supported for notifications, so you'll need to use another protocol for this feature. This is something to keep in mind when setting up notifications for your bucket.
Notifications can be a powerful tool for maintaining data security and integrity, but it's essential to understand the limitations and requirements of this feature.
Expand your knowledge: Why Cloud Security Is Important
Sources
- https://pkg.go.dev/cloud.google.com/go/storage
- https://firebase.google.com/docs/storage/gcp-integration
- https://hartwigmedical.github.io/documentation/accessing-hartwig-data-through-gcp.html
- https://doc.dataiku.com/dss/latest/connecting/gcs.html
- https://www.geeksforgeeks.org/uploading-and-downloading-objects-in-google-cloud-storage-command-line-and-api/
Featured Images: pexels.com