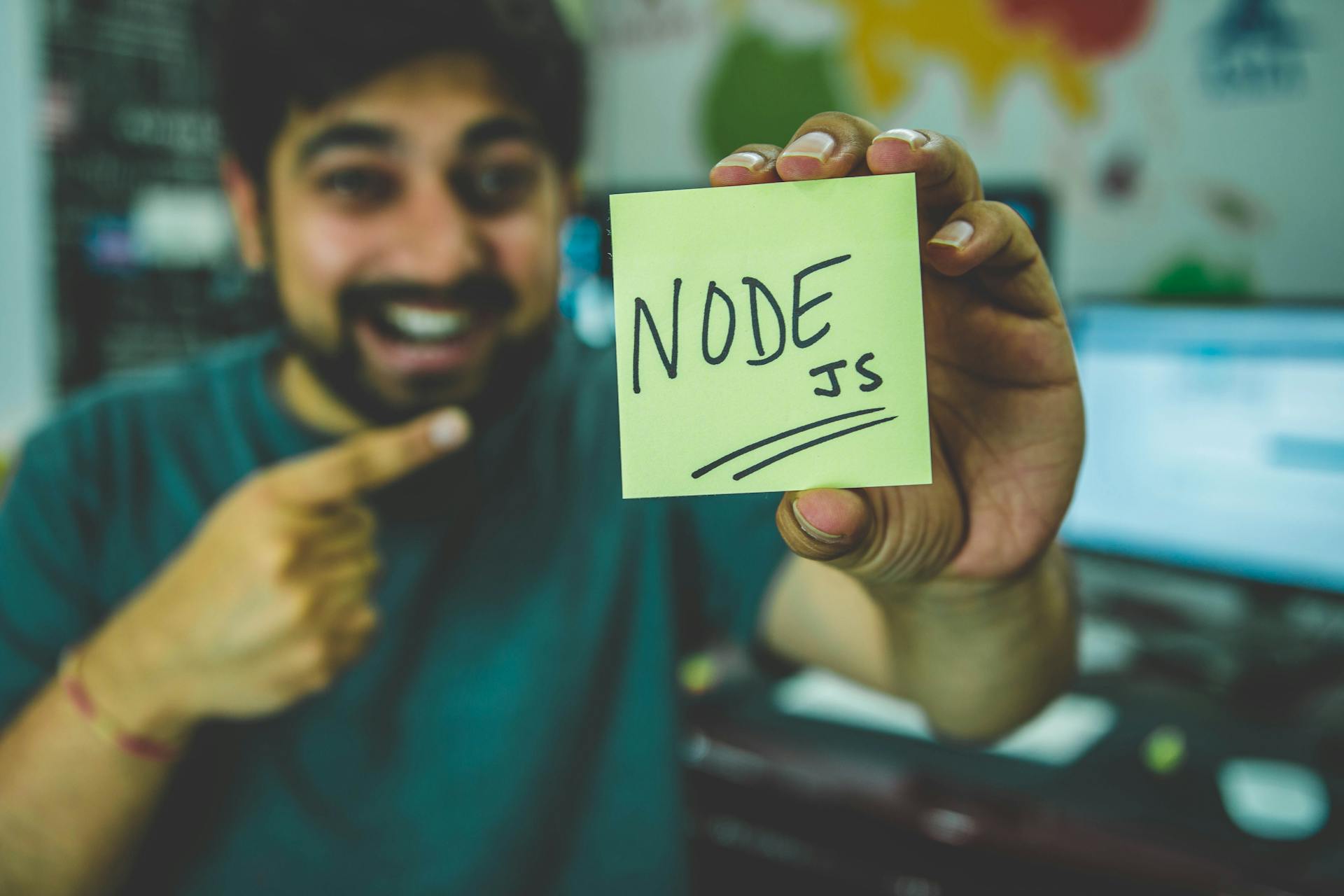
Migrating from React to Next JS can seem daunting, but it's a great opportunity to modernize your application and take advantage of Next JS's powerful features.
To start, you'll need to decide whether to use the Next JS upgrade tool or do a manual migration. The upgrade tool is a great option if you're just starting out, but manual migration provides more control over the process.
First, you'll need to update your package.json file to include the Next JS package. This will ensure you're using the latest version of Next JS. The package.json file is where you'll also need to update your scripts to use Next JS's built-in development server.
You'll also need to update your Webpack configuration to use Next JS's optimized Webpack configuration. This will help improve your application's performance and make it easier to manage your codebase.
Recommended read: How to Update Nextjs
Benefits of Migration
Migrating from React to Next.js can bring numerous benefits to your web development project. Next.js is a deep modification of React library, offering server-side rendering, improved SEO efficiency, and more.
One of the main reasons developers mention the possibility to convert React to Next.js is due to reusable React components. This means you can adopt existing React components or even entire projects.
Server-side rendering is a great feature that improves the response rate, making it faster for the user. It renders HTTP files on the server and sends them to the user, providing all the needed data in a single file.
Next.js also supports the TypeScript programming language, giving developers more opportunities and untieing their hands in specific cases.
Simple API integration and implementation are also key benefits of Next.js.
Simple code-splitting allows you to save space and decrease the size of your app.
Here are some key benefits of migrating to Next.js:
- Improved SEO efficiency
- Server-side rendering for faster user experience
- Reusable React components
- TypeScript support
- Simple API integration and implementation
- Simple code-splitting
Project Setup
To set up a Next.js project, you'll need to have Node.js and npm installed on your computer. You can check if you have them installed by running `node -v` and `npm -v` in your terminal.
Recommended read: Next Js vs Node Js
Create a new directory for your Next.js project and navigate to it in your terminal. Run `npm init -y` to create a new `package.json` file for your project.
To install the Next.js and React dependencies, run `npm install next react react-dom` in your terminal. This will add the latest versions of Next.js, React, and ReactDOM to your project.
Here are the steps to create a new Next.js project:
- Create a new directory for your project.
- Navigate to the directory in your terminal.
- Run `npm init -y` to create a new `package.json` file.
- Run `npm install next react react-dom` to install the dependencies.
Once you've installed the dependencies, you can create a new file called `pages/index.js` to contain the entry point for your app.
Create App Migration
To create an app migration, start by installing Next.js using npm or yarn by running npm install next or yarn add next. This will install the Next.js package and get you ready to migrate your existing React components.
Next.js is a framework that makes it easy to build server-rendered React apps, with automatic code splitting, optimized performance, and a simple file-system based routing approach. This means you can easily migrate your existing React components to Next.js.
Readers also liked: Npm Next Js
You'll need to set up your project by creating a pages directory and adding your routes as individual files within it. Each route file should contain a default export, which is a function that returns the JSX for that route.
To convert your existing React components to Next.js components, simply import the next/component module and extend the NextComponent class. This will give you access to additional features provided by Next.js.
Here's a step-by-step guide to migrating your React components to Next.js:
- Install Next.js using npm or yarn
- Set up your project by creating a pages directory and adding your routes
- Convert your existing React components to Next.js components
- Update your routes to reflect the file-system based routing approach
- Test your components to ensure they're working as expected
- Migrate your data fetching logic to work with Next.js
By following these steps, you'll be able to migrate your React components to Next.js and take advantage of its many benefits, including automatic code splitting and optimized performance.
Setting Up a Project
To set up a new project, you'll want to make sure you have Node.js and npm installed on your computer. This will allow you to create and manage your project's dependencies.
First, check if you have Node.js and npm installed by running `node -v` and `npm -v` in your terminal. If you don't have them installed, you can download them from the official Node.js website.
Next, create a new directory for your project and navigate to it in your terminal. You can do this by running `mkdir project-name` and then `cd project-name`.
Now, let's create a new package.json file for your project. Run `npm init -y` to generate the file, which will contain all the dependencies and scripts needed to run your app.
Here's a list of the steps to set up a new Next.js project:
- Create a new directory for your project and navigate to it in your terminal.
- Run `npm init -y` to create a new package.json file for your project.
- Install the Next.js and React dependencies by running `npm install next react react-dom`.
Once you've installed the dependencies, you can create a new file called `pages/index.js` in your project directory. This file will contain the entry point for your app.
To start the development server, add a script to your package.json file by running `npm run dev`. This will compile and start the app, and you should see the "Hello, World!" message displayed in your browser.
For more insights, see: Next Js vs Create React App
Routing and Navigation
In Next.js, routing is handled through a filesystem-based approach, where the file structure determines the routing.
To get started, create a pages directory within your project. This directory will contain your routes as individual files. For example, if you want to create a page with the URL path /about, you would create a file named about.js inside the pages directory.
Next.js uses a file-based routing system, where the file name determines the URL path. For example, if you create a file called about.js, the route for that page will be /about.
To navigate between routes within your app, you can use the Link component provided by Next.js. This component works similar to the a element in HTML, but it uses client-side navigation to avoid full-page reloads.
Here's a summary of the key steps to update your routes in Next.js:
- Create a new pages directory and add your routes as individual files within it.
- Update your routes to reflect the new file-based routing system.
- Use the Link component to navigate between routes.
By following these steps, you can easily migrate your React components to Next.js and take advantage of its powerful routing features.
Data Fetching
Data fetching is a crucial aspect of building a Next.js app, and it's different from React.js. Usually, React.js apps fetch data in the "useEffect" hook, but this makes requests on the client-side.
Check this out: Next Js Fetch Data save in Context and Next Route
In Next.js, you can use the "getStaticProps" and "getServerSideProps" functions for server-side data fetching. These functions run on the server during build time or server-side rendering, depending on whether you're using Static Site Generation (SSG) or Server-Side Rendering (SSR) respectively.
Both "getStaticProps" and "getServerSideProps" functions allow you to perform fetch requests and return the results. The returned values are available to the default export function as props. You can directly use the Fetch API – fetch() – without putting it inside special functions like "getStaticProps" or "getServerSideProps".
Here are some key differences between "getStaticProps" and "getServerSideProps":
- "getStaticProps" is better when you're aware of the user's request during build time.
- "getServerSideProps" is executed every time a user makes a new request to the page.
- "getStaticProps" runs when static pages are built.
You can use "getStaticProps" to fetch data from a JSON file, and "getServerSideProps" to fetch data from an API.
Here are some examples of using these functions:
* Example of using "getStaticProps" to fetch data from a JSON file:
```javascript
export async function getStaticProps() {
const data = await fetch('https://example.com/data.json');
return {
props: {
Discover more: Next Js Getstaticprops
data: await data.json(),
},
};
}
```
* Example of using "getServerSideProps" to fetch data from an API:
```javascript
export async function getServerSideProps({ params }) {
const id = params.id;
const data = await fetch(`https://example.com/data/${id}`);
return {
props: {
data: await data.json(),
},
};
}
```
You can also use the Fetch API – fetch() – directly in your components, and it will happen on the server, not on the client.
A fresh viewpoint: Next Js Get Server Side Props
Components and Server-Side Rendering
Next.js already renders React components on the server, but Server Components take it a step further by omitting the hydration step. This means the JavaScript components are not sent to the client browser, reducing the JavaScript bundle size sent to the browser.
Server Components have some limitations, such as being non-interactive, but they offer benefits like reduced JavaScript bundle size and secure usage of secret keys and API keys.
To make things interactive, you can use Client Components, which render on the server and hydrate on the client as usual. The use client directive at the top of a file marks all the components in that file as Client Components, and imported components will also function as client components.
Suggestion: Next Js Component
Fetching Data with GetServerSideProps and GetStaticProps
Fetching data with GetServerSideProps and GetStaticProps is a crucial aspect of building efficient and scalable Next.js applications.
These two functions, GetServerSideProps and GetStaticProps, are used to fetch data on the server for each request, ensuring that the data is up-to-date.
In Next.js, GetServerSideProps is a function that is called on the server for every request to a specific page. This is useful for cases where the data is dynamic or cannot be retrieved at build time, such as fetching data from an API.
GetStaticProps, on the other hand, is a function that is called at build time to retrieve data for a specific page. This is useful for cases where the data is static or can be retrieved at build time, such as fetching data from a file or a headless CMS.
To choose between these two methods, consider the nature of your data and when it is available. If the data is static or can be retrieved at build time, use GetStaticProps. If the data is dynamic or cannot be retrieved at build time, use GetServerSideProps.
For another approach, see: Next Js Build
Here is a summary of the key differences between GetStaticProps and GetServerSideProps:
Both GetStaticProps and GetServerSideProps can be used together to create efficient and scalable applications. By understanding the differences between these two functions, you can make informed decisions about how to fetch data in your Next.js application.
Server Components & Client Components
Server Components are a new feature in React that allows you to render components on the server. This means the server sends the rendered HTML to the client browser, reducing the amount of JavaScript sent to the browser.
With Server Components, tasks like data fetching and API requests are performed fully on the server, improving performance and security. This also allows secret keys and API keys to be safely used inside server components without getting exposed to clients.
Server Components are not interactive, so things like useState, useEffect, and onClick won't work. To make things interactive, you can use Client Components, which render on the server and hydrate on the client as usual.
Client Components can be created by adding the use client directive at the top of a file, which marks all components in that file as Client Components. If you import components into a client component, they will also function as client components.
However, you can't import a server component into a client component. Some features, like SEO metadata, can only be used inside a server component and can't be imported within a client component.
App.js Gone
The _app.js file is gone in the App Router.
You can use layout files – layout.js (.jsx or .tsx) – to define custom app structures.
Layout files can be placed alongside page.js files inside route files.
Multiple levels of nested layouts are allowed, with a parent layout file in the root directory and sub-layouts inside route folders.
The root layout file is a must-have and takes the inner layouts and pages as its children.
You can import global stylesheets within the root layout.
Deployment and Testing
To deploy your Next.js app, you'll need to build it for production using the next build command. This will optimize your code and assets for better performance.
To ensure your app is optimized for production, set the NODE_ENV environment variable to production. This will enable Next.js to use production mode, which includes optimizations like minification and code splitting.
You can use a hosting service like Vercel, Heroku, or AWS to handle the deployment and scaling of your app. These platforms will take care of the technical details, so you can focus on other aspects of your project.
Here are some hosting options for Next.js apps:
Testing your app is also crucial, and you can continue using your existing React tests in the Next.js project. Jest and other popular testing libraries are compatible with Next.js.
Deploying to Production
To deploy your Next.js app to a production environment, you'll want to run the next build command to optimize your code and assets for better performance.
This will generate a build folder containing the optimized assets and HTML files, which you can then use to deploy your app. Next.js uses the production mode by default when the NODE_ENV environment variable is set to production.
Using a hosting service like Vercel, Heroku, or AWS can handle the deployment and scaling of your app, so you don't have to worry about it. These platforms provide a seamless integration with Next.js, making it easy to create an optimized production build.
Here are the steps to follow:
- Set the NODE_ENV environment variable to production
- Run the next build command to build your app for production
- Use a hosting service like Vercel, Heroku, or AWS
- Use a CDN to improve the performance of your app
A content delivery network (CDN) can help improve the performance of your app by serving your assets from a global network of servers, reducing the load on your server and improving loading times for users.
Step 7: Testing
Testing is an essential part of ensuring your Next JS project is stable and reliable. You can continue using your existing React component tests in the Next JS project.
Jest and other popular testing libraries are fully compatible with Next JS, allowing you to use your existing test suites without any issues. This makes it easier to maintain a consistent testing workflow throughout your project.
Styling and Files
Migrating your React project to Next JS is a big step, but don't worry, you can keep your existing styling solution.
Next JS supports various CSS modules and preprocessors, but you can also stick with what you know.
If you were using CSS modules in your previous React project, you can simply copy this file into the Next JS project.
You can then import and use it in your components as before, without any issues.
In Next JS, you can also handle assets and static files, like images and fonts, in a built-in way.
Just place these files in the public directory, and they'll be served at the root URL.
For example, if you have an image logo.png, move it to the public directory and reference it in your components using the root path.
Sources
- https://incora.software/insights/convert-react-to-nextjs
- https://www.coralnodes.com/migrate-nextjs-app-router-pages-router/
- https://nextjs-ja-translation-docs.vercel.app/docs/migrating/from-create-react-app
- https://stefaniq.com/migrating-your-react-app-to-next-js-a-comprehensive-guide/
- https://www.educative.io/answers/steps-for-migrating-an-existing-react-project-to-next-js
Featured Images: pexels.com