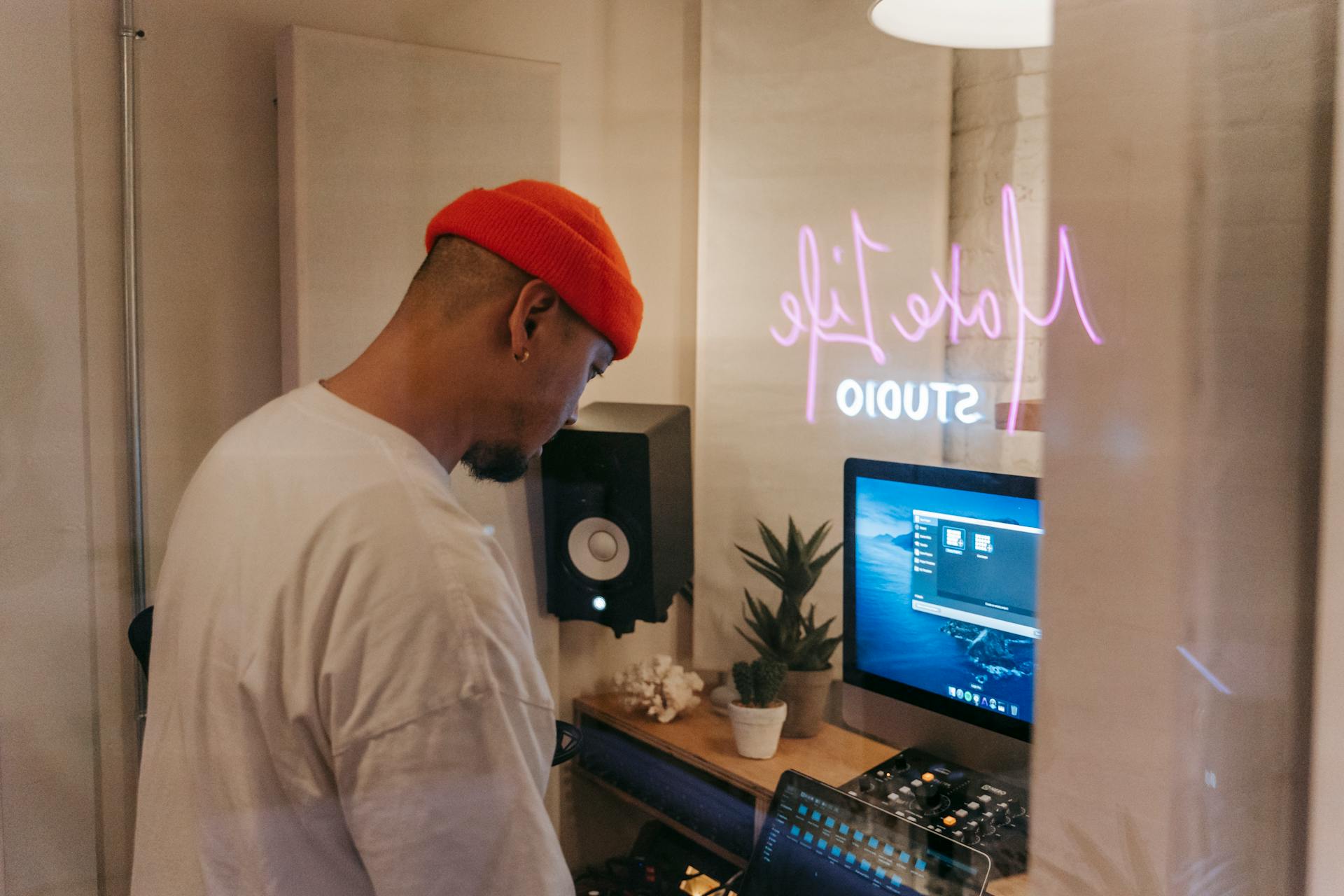
Uploading files to a website is a common task, but it can be a bit tricky to set up from scratch. To start, you need to create an HTML form that allows users to select files from their computer.
The form will have an input type of "file" to specify that it's for file uploads. This input type is supported in most modern browsers and is the standard way to handle file uploads in HTML.
The next step is to create a server-side script to handle the uploaded file. This script will be responsible for storing the file on the server and doing any necessary processing.
To handle file uploads, you'll need to specify the type of file that can be uploaded. This is done using the "accept" attribute on the file input. For example, you can specify that only image files can be uploaded by setting the accept attribute to "image/*".
Related reading: Itext Insert Image in Header File from Html
Security and Best Practices
Using a dedicated file upload area on a non-system drive can help prevent cyberattacks. This makes it easier to impose security restrictions on uploaded files.
Always disable execute permissions on the file upload location to prevent malicious code from being executed.
To prevent user-supplied file names from being used, create a safe file name for the file using Path.GetRandomFileName or Path.GetTempFileName. This is especially important when saving files to physical storage.
Razor automatically HTML encodes property values for display, making it safe to use code like this:
Always HtmlEncode file name content from a user's request to prevent cross-site scripting (XSS) attacks.
Here are some security steps to reduce the likelihood of a successful attack:
Form Scenarios and Binding
There are two general approaches for uploading files: buffering and streaming. Buffering involves reading the entire file into an IFormFile, which can be memory-intensive and may cause the site to crash if too many files are uploaded at once.
A different take: Html File Upload Multiple Files
Streaming, on the other hand, involves processing the file as it's being uploaded, reducing the demand on memory and disk space. However, streaming doesn't improve performance significantly and is best suited for small files.
To support file uploads, HTML forms must specify an encoding type (enctype) of multipart/form-data. This is necessary to allow the file to be uploaded successfully.
- Buffering is suitable for small files, while streaming is better suited for larger files.
- Streaming reduces the demand on memory and disk space, but doesn't improve performance significantly.
In Razor Pages forms, the individual files uploaded to the server can be accessed through Model Binding using IFormFile. The sample app demonstrates multiple buffered file uploads for database and physical storage scenarios.
Scenarios
Buffering is a common approach for uploading files, but it can be resource-intensive. If an app attempts to buffer too many uploads, it may run out of memory or disk space.
The entire file is read into an IFormFile, which is a C# representation of the file used to process or save the file. This approach is suitable for small files, but it's not recommended for large files.
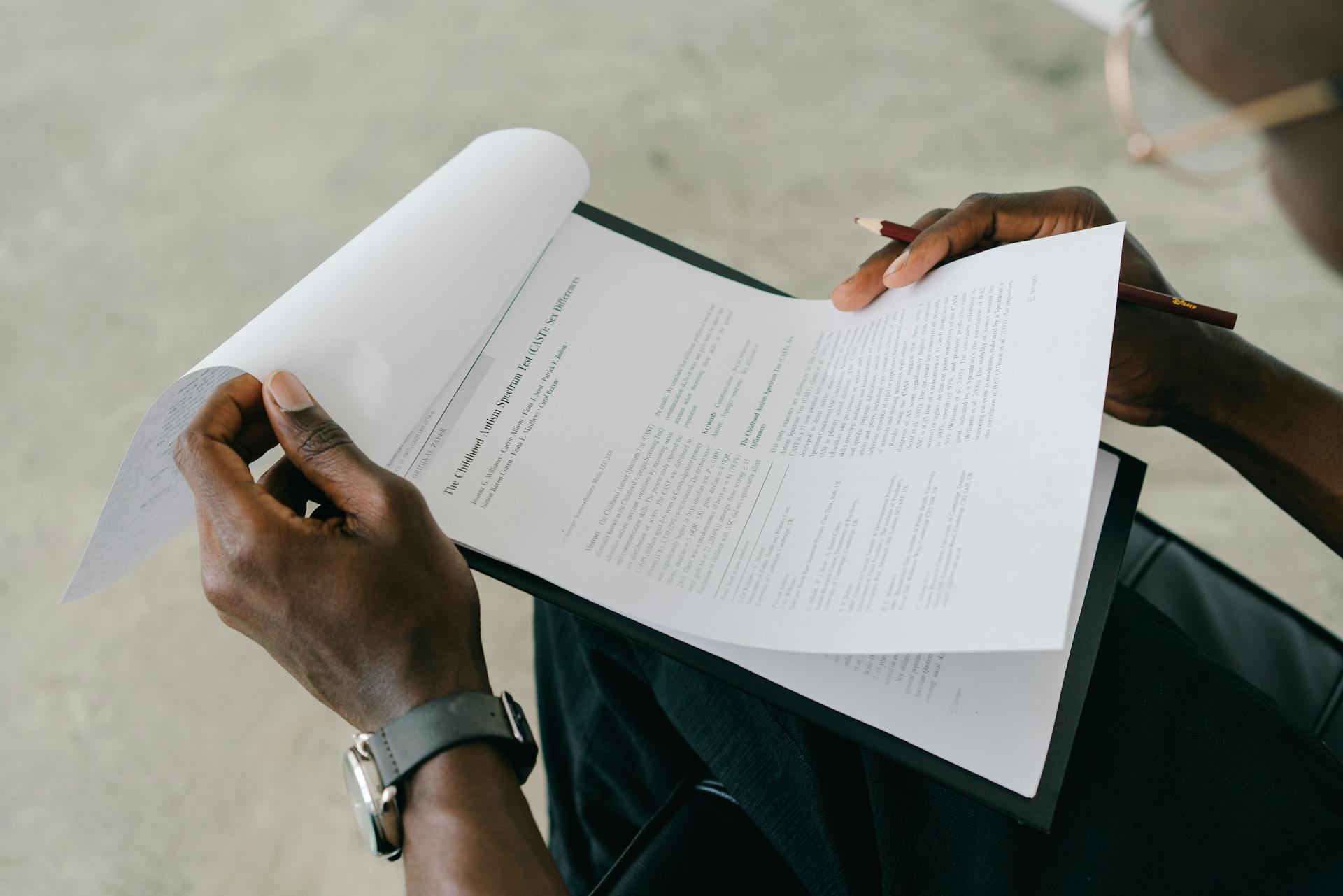
Any single buffered file exceeding 64 KB is moved from memory to a temp file on disk. Temporary files for larger requests are written to the location named in the ASPNETCORE_TEMP environment variable.
Streaming is a better approach for uploading large files, as it reduces the demands for memory or disk space. However, it doesn't improve performance significantly for small files.
To upload small files, use a multipart form or construct a POST request using JavaScript. This approach is useful for small files, but it's not suitable for large files.
Here are some options for uploading small files:
- Use a Fetch Polyfill (for example, window.fetch polyfill (github/fetch)).
- Use XMLHttpRequest. For example, the AJAXSubmit function uses XMLHttpRequest to send a POST request with a FormData object.
The FileUpload is used in the Razor Pages form, and it's essential to use a matching name for the parameter of the C# method. This ensures that the file is received correctly and processed or saved accordingly.
Files can be stored in various locations, including a database, physical storage (file system or network share), or a data storage service (for example, Azure Blob Storage).
A different take: How to Upload File to Google Cloud Storage Using Reactjs
Small Buffered Model Binding to Storage
To upload small files using buffered model binding, specify an encoding type of multipart/form-data in the HTML form. This allows the form to support file uploads.
You can use a Razor Pages form to upload a single file, and the individual files uploaded to the server can be accessed through model binding using IFormFile. The sample app demonstrates multiple buffered file uploads for database and physical storage scenarios.
Any single buffered file exceeding 64 KB is moved from memory to a temp file on disk. Temporary files for larger requests are written to the location named in the ASPNETCORE_TEMP environment variable.
Use a Fetch Polyfill or XMLHttpRequest to upload small files. For example, you can use the window.fetch polyfill or the XMLHttpRequest object.
Here are some security considerations to keep in mind:
- Remove the path from the user-supplied filename.
- Save the HTML-encoded, path-removed filename for UI or logging.
- Generate a new random filename for storage.
The action method can accept a single IFormFile or any of the following collections that represent several files:
- A collection of IFormFile objects.
- A collection of IFormFile objects with a specific name.
When uploading files using model binding and IFormFile, the action method can also accept a bound model property. This property can be used to store the uploaded file in a database or on the file system.
Advanced Form Customization
You can specify the encoding type for a form element using the enctype attribute, which can be set to "multipart/form-data" or "application/x-www-form. multipart/form-data is necessary if your users are required to upload a file.
There are two types of methods available for the method attribute: POST and GET.
To validate the data and format it according to specified formats, you can use the type attribute, specifically type="file" for uploading a file.
The INPUT NAME attribute is used to specify a name for an INPUT element, which is used to reference the form data after submitting the form or reference the JavaScript or CSS element.
The accept attribute's value is a semicolon-separated list of one or more file types or file type designations, specifying which file types to accept.
Here's a list of the available HTTP methods:
- POST
- GET
The enctype attribute can be set to one of the following values:
- multipart/form-data
- application/x-www-form
Frequently Asked Questions
How do I attach a file to HTML?
To attach a file to HTML, use the `` element, which allows users to select files from their device storage. This file can then be uploaded or manipulated using JavaScript and the File API.
Sources
- https://www.filestack.com/fileschool/html/html-file-upload-tutorial-example/
- https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file
- https://learn.microsoft.com/en-us/aspnet/core/mvc/models/file-uploads
- https://dev.to/faddalibrahim/how-to-create-a-custom-file-upload-button-using-html-css-and-javascript-1c03
- https://developer.mozilla.org/en-US/docs/Web/API/File_API/Using_files_from_web_applications
Featured Images: pexels.com