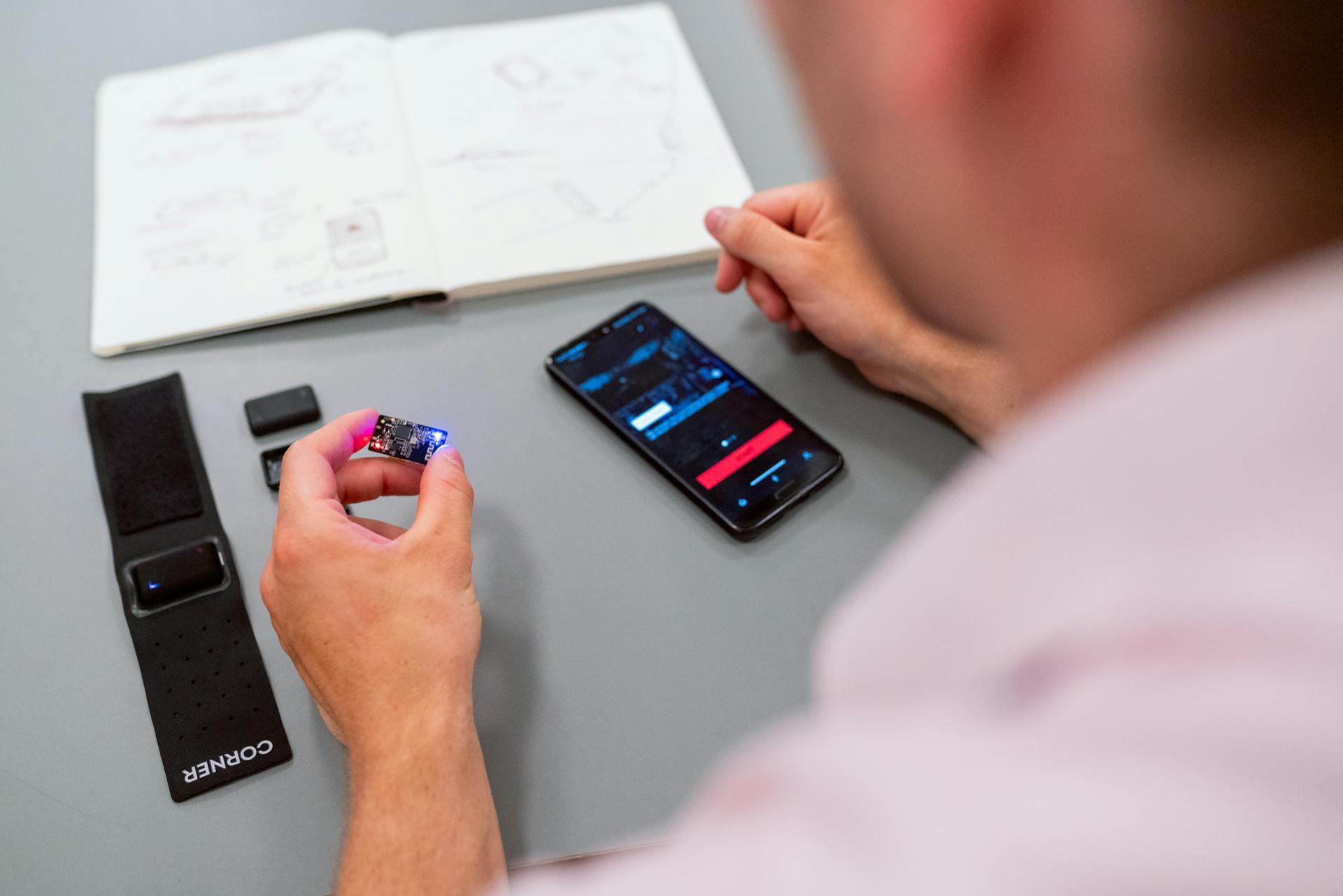
MUI NextJS App Router is a powerful tool that enables you to create fast and scalable web applications. It's built on top of the Material-UI (MUI) library and Next.js framework.
With MUI NextJS App Router, you can create routes for your application using the `app` function. This function takes a component as an argument and returns a new instance of the App component, which is the top-level component of your application.
The App Router also provides a `useRouter` hook that allows you to access the router instance in your components. This hook is useful for navigating between routes and accessing route parameters.
By using MUI NextJS App Router, you can create a robust and maintainable routing system for your Next.js application.
Recommended read: Intercepting Routes Next Js Example
Getting Started
To set up a MUI Next.js app router, you'll need to create a new Next.js project with the MUI library installed.
First, install the required packages, including `@mui/material` and `@emotion/react`. This will provide you with the necessary components and utilities to get started.
For another approach, see: Mui Nextjs React Type Mismatch
MUI provides a wide range of pre-built components, including buttons, inputs, and cards, which can be easily integrated into your Next.js app.
Create a new file called `pages/_app.js` and add the MUI theme configuration to it. This will enable the MUI theme in your app.
To use the app router, you'll need to create a new file called `pages/_app.tsx` and add the `AppRouter` component to it.
The `AppRouter` component will serve as the main entry point for your app's routing.
For another approach, see: App Routing vs Page Routing Nextjs
Next.js Routing
In Next.js, the Link component handles all navigation, but it's not the only one you'll need.
The MUI Link component is necessary for styling, and it accepts a prop called component, which allows it to be tied to a third-party framework like Next.js.
To combine both, use the component prop inside your page.js to tie your MUI Link with a Next.js Link.
For external or deep links, you don't need Next's Link component because you're leaving your app's router.
Discover more: Next Js Link Component
Advanced Routing Features
The App Router in Next.js offers several advanced routing features that make it a compelling choice over the classic Pages Router. One of these features is support for Nested Routes & Layouts, which allows you to create shared layouts between multiple pages.
App Router also includes Route Groups, which enable you to organize routes without affecting the URL, and opt-in specific routes into a layout. You can also use Loading UI to create meaningful loading states.
Here are some of the key features included in App Router:
- Layouts that can be shared between multiple pages.
- Route Groups that allow you to organize routes without affecting the URL.
- Loading UI that helps you create meaningful loading states.
- Intercepting Routes that allow you to load a route in different ways depending on the context.
Server Actions (Alpha)
Server Actions (Alpha) is a game-changer for handling mutations on the server that can be called directly from the client.
Next.js 13.4 introduced Server Actions, which integrate with the rest of the data lifecycle and can mutate data, revalidate any existing cache of that data, and then re-render the page in a single network roundtrip.
Using a Server Action is incredibly simple, as shown in the example code, which notates the action as a Server Action with the use server directive.
Take a look at this: Nextjs App Route Get Ssr Data
The action is then set as the form's action, and when the form is submitted, it invokes the Server Action, which runs on the server, allowing you to make use of secrets or make calls directly to a database.
This approach eliminates the need for multiple API calls, as Next.js is able to deduplicate requests, so the API is called only once, as seen in the example where getProduct is called multiple times.
Broaden your view: Nextjs Api Router
Nested Routes & Layouts
Nested Routes & Layouts are a game-changer in Next.js, and App Router takes it to the next level. It allows you to share layouts between multiple pages, making it easier to maintain a consistent design throughout your application.
Route Groups are another powerful feature that lets you organize routes without affecting the URL, giving you more flexibility in how you structure your app. You can also opt-in specific routes into a layout, which is super handy when you want to reuse code.
A different take: Next Js Nested Routes
Loading UI is a feature that helps you create meaningful loading states, making your app feel more responsive and polished. It's a small detail, but it can make a big difference in user experience.
Intercepting Routes is another feature that allows you to load a route in different ways depending on the context. This is useful when you need to handle different scenarios, like loading data from an API or showing a fallback page.
Here are some of the key features of App Router's Nested Routes & Layouts:
- Shared layouts between multiple pages
- Route Groups for organizing routes without affecting the URL
- Loading UI for creating meaningful loading states
- Intercepting Routes for loading routes in different ways depending on the context
Conclusion
The conclusion of our discussion on Advanced Routing Features leaves us with a clear understanding of the capabilities and limitations of Next.js App Router.
Next.js App Router may not be suitable for large-scale production applications, as evidenced by Udacity's decision to stick with MSW and Chakra.
Being an early adopter of Next.js App Router carries inherent risks, making it more suitable for smaller-scale applications.
If you're comfortable taking on the risk and the problems mentioned don't pose significant obstacles for your application, then Next.js App Router is definitely worth using.
Sources
- https://nextjs.org/docs/app/building-your-application/routing/loading-ui-and-streaming
- https://engineering.udacity.com/what-is-next-js-app-router-is-it-ready-for-the-main-stage-bed07ef9519f
- https://javascript.plainenglish.io/mastering-next-js-4cfff5a89882
- https://blog.logrocket.com/getting-started-mui-next-js/
- https://blog.devgenius.io/mastering-next-js-mui-box-component-497d76a01528
Featured Images: pexels.com