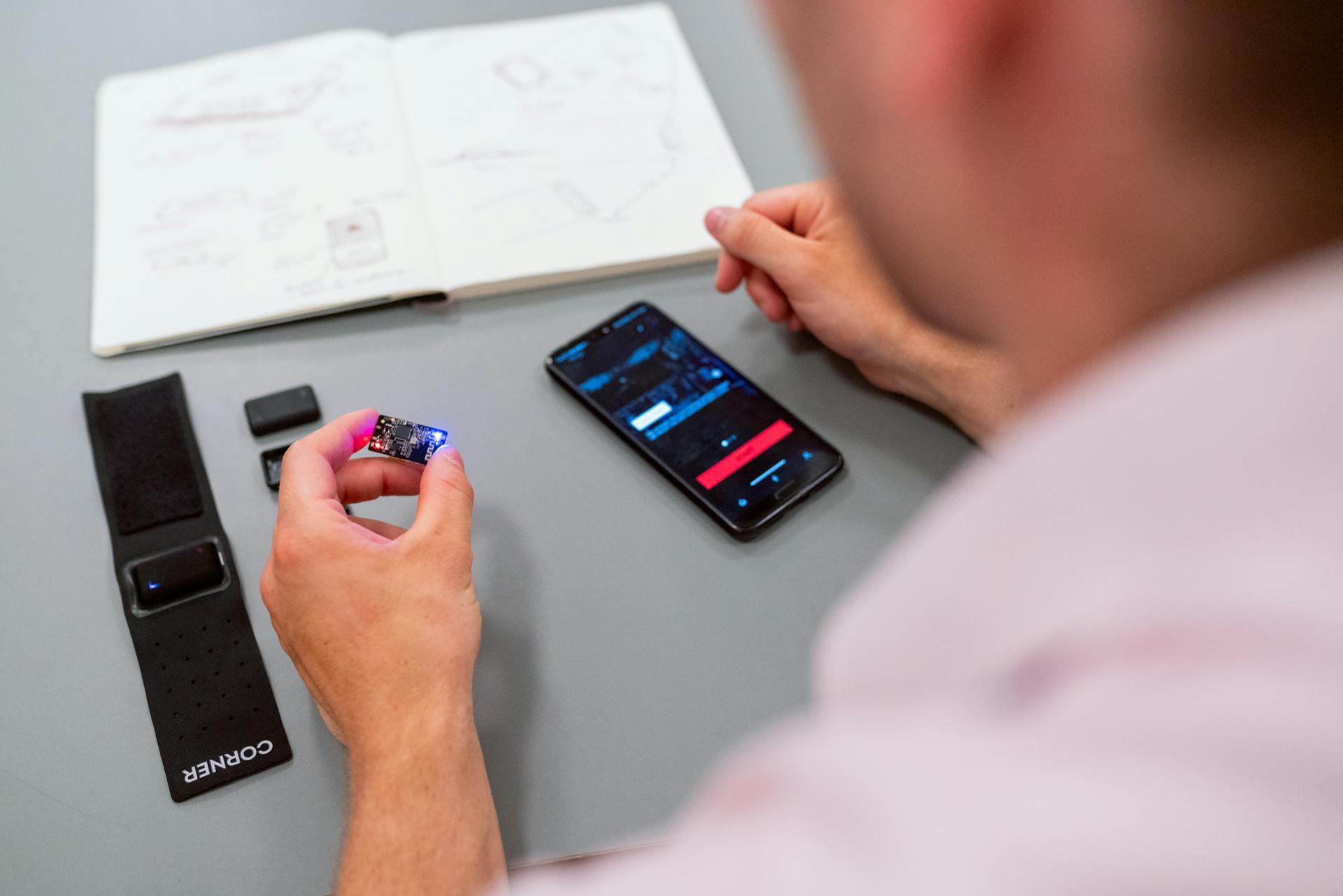
Let's dive into some real-world examples of Next.js projects on GitHub. If you're new to Next.js, you might want to start with the official tutorial on GitHub Authentication, which provides a step-by-step guide on how to add authentication to your Next.js app.
This tutorial uses the Next.js GitHub OAuth package to authenticate users and provides examples of how to use the package in various scenarios. The examples cover topics such as setting up authentication, handling user sessions, and protecting routes with authentication.
One example from the tutorial shows how to set up authentication with GitHub OAuth, which involves installing the Next.js GitHub OAuth package and setting up a GitHub OAuth app. This example is a great starting point for anyone looking to add authentication to their Next.js app.
The tutorial also covers more advanced topics, such as how to handle user sessions and protect routes with authentication. For example, it shows how to use the `getServerSideProps` method to fetch user data from GitHub and store it in a session.
Project Setup
To set up a Next.js project, start by running the command `create-next-app` in your terminal, which will create a new app and prompt you to enter your project name.
This command uses the yarn package manager, but you can also use npm if needed.
Once the installation is completed, move into your folder directory and run the command to start the project.
You'll then need to install the NextAuth package, which is essential for authentication in your app.
If you're using TypeScript, don't worry – NextAuth.js comes with its types definitions within the package.
Deployment
Deployment is the final step in getting your Next.js app up and running. You'll need to deploy to Vercel, which requires setting up environment variables and linking your Vercel postgres database.
First, you'll need to set up your Vercel project name by prepending your first and last name to "blogr-nextjs-prisma". For example, if you're called "Jane Doe", your project name should be "jane-doe-blogr-nextjs-prisma".
To deploy to Vercel, you'll need to set the Authorization Callback URL to https://FIRSTNAME-LASTNAME-blogr-nextjs-prisma.vercel.app/api/auth.
You'll also need to set the following environment variables in your .env file:
- GITHUB_ID: Set this to the Client ID of the GitHub OAuth app you just created
- GITHUB_SECRET: Set this to the Client Secret of the GitHub OAuth app you just created
- NEXTAUTH_URL: Set this to the Authorization Callback URL of the GitHub OAuth app you just created
- SECRET: Set this to your own strong secret
Once you've set all the environment variables, you can deploy your app by clicking the "Deploy" button.
Introduction to NextAuth.js
NextAuth.js is an open source authentication library designed for Next.js and serverless applications. It allows users to sign in with their favorite preexisting logins without needing to create a database or backend.
NextAuth.js supports OAuth 1.0, 1.0A, 2.0, and OpenID Connect, making it compatible with any OAuth service. Many popular sign-in services are already supported, including email and passwordless authentication.
Here are some of the key features of NextAuth.js:
- JSON Web Tokens and database sessions are both supported.
- It can be used without a database (for example, OAuth + JWT).
- MySQL, MariaDB, Postgres, SQL Server, MongoDB, and SQLite are all supported natively.
- Excellent compatibility with popular hosting provider databases.
- It's designed for Serverless but runs anywhere (AWS Lambda, Docker, Heroku, and so on…).
NextAuth.js is an open-source solution that gives you control over your data and is secure by default, making it a reliable choice for authentication needs.
What Is NextAuth?
NextAuth.js is an easy-to-implement open source authentication library originally designed for Nextjs and serverless. It's a game-changer for developers who want to add authentication to their applications without the hassle.
One of the best things about NextAuth is that it doesn't require you to create or use any database or backend for authentication. It takes care of all that for you.
NextAuth supports OAuth 1.0, 1.0A, 2.0, and OpenID Connect, making it a versatile solution for authentication.
Here are some of the key benefits of using NextAuth:
- Supports OAuth 1.0, 1.0A, 2.0, and OpenID Connect
- Many popular sign-in services are already supported
- Email and passwordless authentication are supported
- Allows for stateless authentication with any backend
- JSON Web Tokens and database sessions are both supported
- Designed for Serverless but runs anywhere
- An open-source solution that gives you control over your data
- MySQL, MariaDB, Postgres, SQL Server, MongoDB, and SQLite are all supported natively
- Excellent compatibility with popular hosting provider databases
- It can be used without a database (for example, OAuth + JWT)
- Secure by default
By using NextAuth, you can add authentication to your applications quickly and easily, without the need for complex setup or configuration.
Error Handling
Error Handling is crucial for a smooth user experience. Sentry and LogRocket help track and diagnose errors, allowing you to spot patterns and recurring problems.
To handle custom errors, Next.js provides a pages/_error.js file where you can set up custom error pages. This way, users get guided through troubleshooting steps if something goes wrong.
Using middleware to catch and manage errors at different stages of the authentication process is also a good practice. NextAuth.js's signIn and signOut methods can include error handling logic to manage specific authentication failures.
API and Authentication
To add authentication to your Next.js project, you create a new folder named auth inside the pages/api directory and add a file called [...nextauth].js. This file contains the dynamic route handler for NextAuth.js and all global NextAuth.js configurations.
You can protect your API routes using the unstable_getServerSession() method, which creates a session object from the request, response, and auth options. This object provides details of the logged-in user.
To test authentication, you write unit tests for your authentication logic using Jest and end-to-end integration tests using Cypress. You also mock OAuth providers during tests to avoid hitting real provider APIs.
Create API Routes
To create API routes for authentication, you'll need to create a new folder named auth inside the pages/api directory.
This folder will contain a file called [...nextauth].js, which serves as the dynamic route handler for NextAuth.js and holds all your global NextAuth.js configurations.
Inside [...nextauth].js, you'll configure the global NextAuth configurations for specific providers, such as Google and Github.
To do this, you'll import NextAuth from the next-auth package and also import the GoogleProvider and GithubProvider, which are then passed into the providers array.
The providers array stores all NextAuth providers, and in this case, it will contain configurations for both Google and Github authentication.
Protect API Routes
To protect your API routes, you can use the unstable_getServerSession() method. This method allows you to check if a user is logged in and retrieve their session details.
You'll need to import the unstable_getServerSession method and the authOptions props. Then, you can create a session object from the unstable_getServerSession method, passing in the req, res, and authOptions as arguments.
The session object will provide you with the details of the logged-in user. You can then check if a session exists and send the requested data to the client, or send a 401 (Unauthorized) response if no session exists.
For example, you can update and protect the pages/api/hello.js API route with the code snippet below. This will ensure that only authenticated users can access the API route.
By using the unstable_getServerSession() method, you can easily protect your API routes and ensure that only authorized users can access your application's data.
Sources
- https://medium.com/@vi.nhon.53th/next-js-v13-demo-login-with-github-and-google-31cd56e547de
- https://nextjs.org/docs/app/building-your-application/deploying
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://vercel.com/guides/nextjs-prisma-postgres
- https://refine.dev/blog/nextauth-google-github-authentication-nextjs/
Featured Images: pexels.com