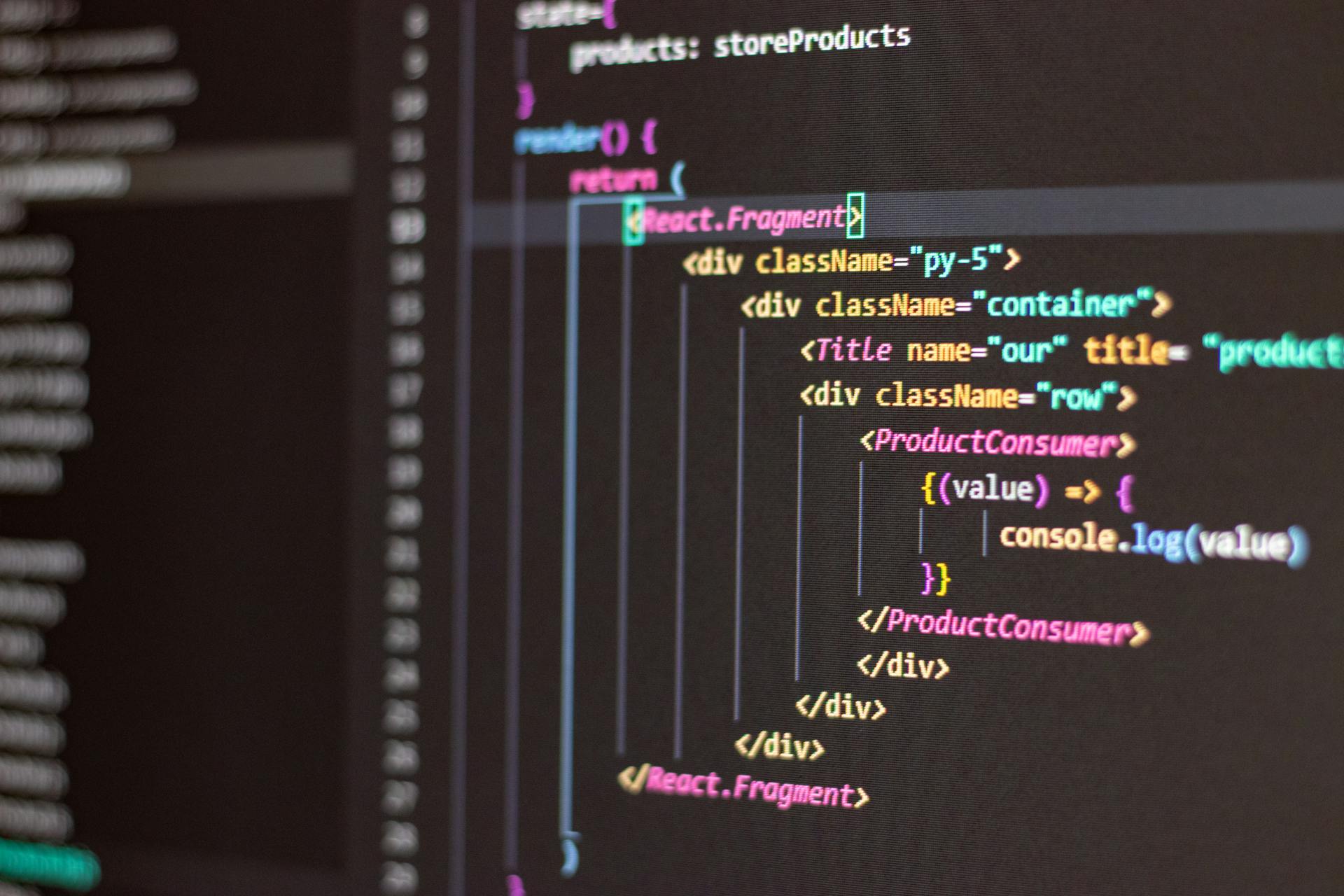
Implementing real-time toast in Next.js projects is a game-changer for user engagement. You can use the `next-toast` library to create customizable toast notifications.
To get started, you'll need to install the library using npm or yarn. In the "Setting up Next Toast" section, we discussed the importance of using the `toast` function to display notifications. This function takes in several parameters, including the message, type, and duration.
The `toast` function is used extensively throughout the project, and it's essential to understand how it works. By default, the toast notification is displayed at the top of the screen, but you can customize its position using the `position` parameter.
Project Setup
To set up the project for real-time toasts in Next.js, install the toast component from shadcn/ui by copying the NPM command from the toast component page in the shadcn/ui docs and running it in your terminal.
You'll see that it installs three separate files: toast.tsx, toaster.tsx, and use-toast.tsx.
Save your changes and start up the development server using npm run dev.
Refresh the app in the browser to load the newest client-side code.
Head over to the Knock dashboard and run a test to send a message inside your in-app workflow.
Discover more: How to Run Next Js App
Creating Toast
To create a toast notification in Next.js, you'll want to start by installing a popular library as a helper. This will save you time and focus on the fundamentals of your form state and server actions.
You'll need to create a new component that will be used as a provider for your toast messages. This component will be responsible for rendering the toast messages and should be used in the root layout of your application.
Add the new Provider to the RootLayout in app/layout.tsx to make the setup complete. This will ensure that the toast messages are displayed correctly throughout your application.
You may be wondering why you can't use the Toaster directly in the RootLayout. The reason is that the Toaster is a third-party component that uses React's useContext Hook, which requires it to be declared as a client component.
To show the general message in your form component, you can use the imported toast function from the library. Since your form component is already a client component, you can use React's useEffect Hook to show the toast message.
Expand your knowledge: Next Js Provider
To make the toast message show up for a form submission, you'll need to extract the previous useEffect Hook into its own custom hook and add the timestamp to the dependency array. This will ensure that the toast message is updated correctly when the form is submitted.
You can then use the new custom hook in your form component and provide it with the form state, the general message, the status for the style, and the timestamp for the dependency array. This will display the toast message correctly in your application.
To create a context for your toast notifications, you can create a new file named ToastContext.tsx in the src/hooks folder. Inside this file, you'll use the createContext function provided by React to create a new context.
You'll also need to define an interface for the context and ensure its usage within your codebase. This will provide a clear understanding of the context and its properties.
Next, you'll create a ToastProvider component that will encapsulate your entire application and furnish the ToastContext to all its descendants or children.
Inside the ToastProvider component, you'll create a function called toastMessage that accepts message and type as arguments. You'll also create individual functions for each type of toast notification, such as success, warning, info, and error.
If this caught your attention, see: Nextjs Form Component
These functions will internally call the toastMessage function with the appropriate arguments for their respective types and data. You'll then create a value object that encapsulates all the functions and pass this object to the value prop of the ToastContext.Provider component.
This will enable you to access the functions later from other pages and pass data to display toast messages. You'll then render the ToastMessage component inside the ToastContext component, enabling you to use it globally by passing arguments to the function from other components.
Finally, you can export useToastContext as a custom hook, allowing other components in your application to access the toast context and its functions.
To utilize the context, you'll need to import it into your App component and call it to gain access to the functions within the value object. You can then pass the necessary arguments to display the desired toast message.
To call the toast function in your component, you'll need to extract it from your useToast hook. This will allow you to call the toast function and pass in an object to trigger your toasts.
Discover more: Next Js Component
Conditional
Conditional toasts can be a game-changer for a seamless user experience. By adding a showToast property to the message payload in Knock and setting it to false by default, you can conditionally display toasts based on certain criteria.
This allows you to pass in different properties and use them to determine how your UI should respond to different events. You can then use an if statement in your code to only call the toast function if the item.data.showToast is true.
With this approach, you can trigger a test event with showToast set to false and see the feed update without any toast displayed.
Progressive Enhancement
Progressive Enhancement is built-in with server components/actions and forms in Next.js, meaning the form works without JavaScript in the browser.
You can disable JavaScript in your browser and the form will still work if you build and start the application with npm run build and npm run start.
Suggestion: Npm Next Js
The form will not show loading feedback and toast messages without JavaScript, but the useToastMessage custom hook returns a noscript element that acts as a fallback feedback for users without JavaScript.
This noscript element renders a general message, showing what's possible in a web native world where no JavaScript is needed.
However, building with these barebone building blocks requires a lot of manual work to get to a desired result, highlighting the potential for open source to provide a better developer experience.
Notification Context
To create a notification context, we need to define a new file named ToastContext.tsx in the src/hooks folder. Inside this file, we'll use the createContext function provided by React to create a new context.
We've successfully created the ToastContext using the createContext function. We've also defined an interface for the context and ensured its usage within our codebase.
Now, let's create a ToastProvider component that will encapsulate our entire application and furnish the ToastContext to all its descendants or children. This component will be responsible for providing the context to all the components in our app.
Broaden your view: Next Js Fetch Data save in Context and Next Route
Inside the ToastProvider component, we'll create a function called toastMessage that accepts message and type as arguments, and then proceeds with the necessary actions. This function will be used to display toast notifications.
We'll create individual functions for each type of toast notification, such as success, warning, info, and error. These functions will internally call the toastMessage function with the appropriate arguments for their respective types and data. This will make it easier to display different types of toast notifications.
Sources
- https://nextjs.org/blog/next-15
- https://www.robinwieruch.de/next-forms/
- https://knock.app/blog/adding-realtime-toast-notifications-in-nextjs
- https://stackoverflow.com/questions/76393897/how-to-call-a-notification-toast-after-a-server-action-in-nextjs13
- https://sandeepbansod.medium.com/toast-notification-component-with-nextjs-context-api-using-reactprime-library-80c3801d8068
Featured Images: pexels.com