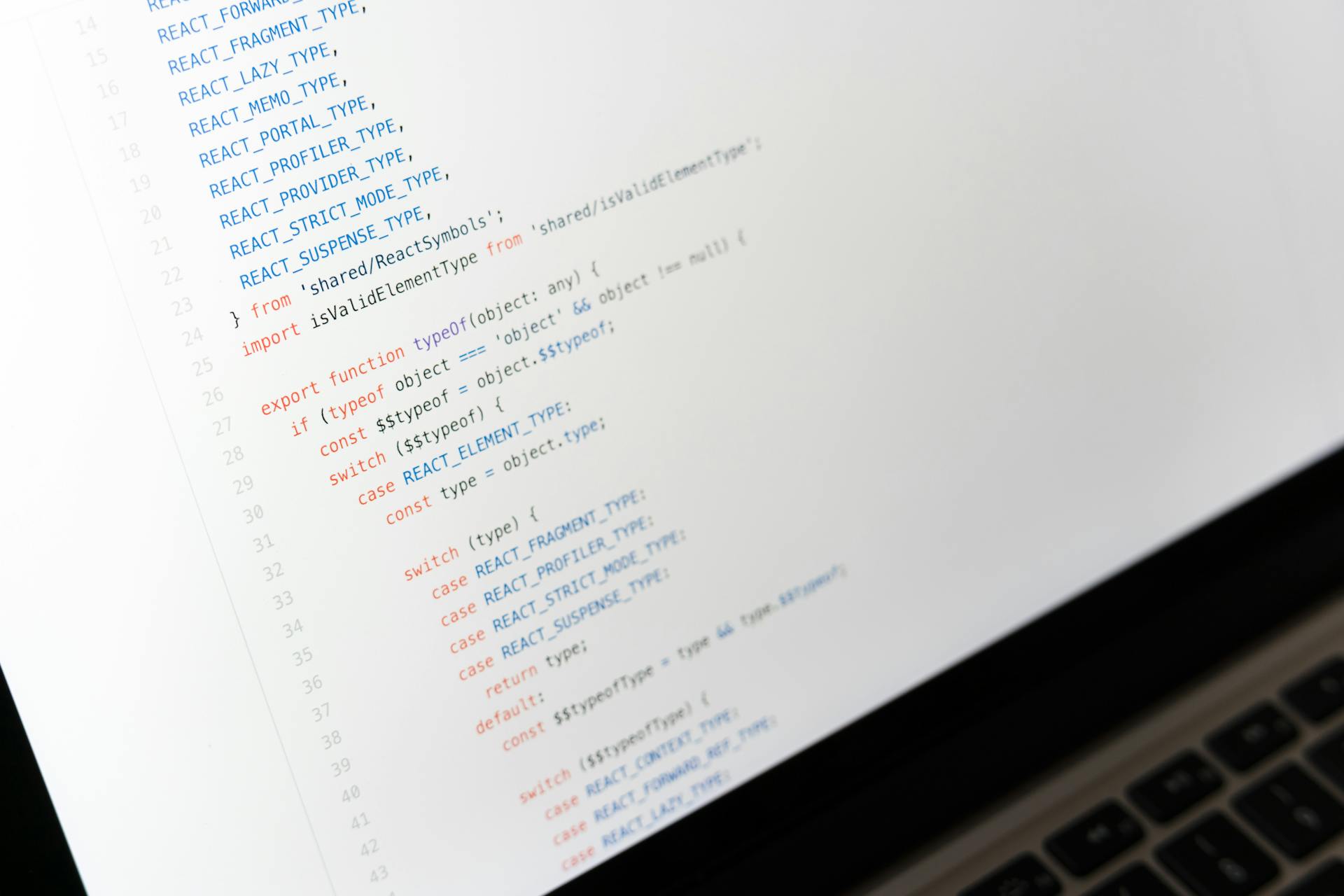
Next.js introduced server components, which allow you to render components on the server. This is a game-changer for large-scale applications, but it also raises questions about the use of hooks.
Server components do not support the use of React hooks, except for a few specific cases.
One of the main reasons for this limitation is that server components need to be statically analyzed, which is not possible with hooks that are not static.
However, there are some exceptions to this rule, such as the `useEffect` hook, which can be used in server components, but only for side effects that are safe to run on the server.
Broaden your view: Next Js Typescript Hooks
Why Hooks Can't Be Used in Server Components
Hooks can't be used in Server Components because they don't have a concept of a "state" that persists across re-renders.
Server Components are executed once on the server to produce HTML, but they lack a browser context, which means hooks like useEffect can't function.
You might enjoy: Nextjs Module Not Found Can't Resolve 'fs'
This is because useEffect relies on the DOM, which isn't available in a server environment.
Here are some key reasons why hooks can't be used in Server Components:
- Execute once on the server to produce HTML.
- Don’t have a concept of a “state” that persists across re-renders.
- Lack a browser context, so hooks like useEffect (which relies on the DOM) cannot function.
To add interactivity to Server Components, you can compose them with Client Components using the "use client" directive.
Broaden your view: Use Client Nextjs
When to Choose Client-Side
If you need interactive behavior, wrap your logic in a client component. This is where hooks like useState and useEffect come into play.
You'll want to use client-side rendering when you need to update the UI in response to user interactions. This is exactly what hooks like useState and useEffect are designed for.
Client components are the way to go when you need to access the DOM. This is a key difference between client and server components.
For example, if you need to update the UI based on user input, client-side rendering is the way to go. This is where hooks like useState and useEffect can be super helpful.
For more insights, see: Nextjs Ui
Adding Interactivity to Server Components
Adding interactivity to Server Components is a bit tricky, as they can't use interactive APIs like useState. You can't send Server Components to the browser, so they're not compatible with useState.
To make Server Components interactive, you can combine them with Client Components using the "use client" directive. This allows you to leverage the strengths of both worlds.
Curious to learn more? Check out: Next Js Debugging
Server Components Interactivity
Server Components are not sent to the browser, so they cannot use interactive APIs like useState.
To add interactivity to Server Components, you can compose them with Client Components using the "use client" directive.
This approach allows you to leverage the strengths of both Server and Client Components, creating a powerful combination for building interactive applications.
By using the "use client" directive, you can bring the interactivity of Client Components to Server Components, enabling a more seamless user experience.
Server Components can now respond to user input and dynamic changes, making them a more integral part of your application's architecture.
Suggestion: Next Js Client Side Rendering
Adding Hooks to Server Components
Adding hooks to server components is a crucial step in making them interactive.
In our previous examples, we've seen how to create server components with a simple render method. However, this approach doesn't allow for any interactivity.
To add hooks to server components, we can use the `useEffect` hook, which allows us to run some side-effect code when the component mounts or updates.
We can also use the `useState` hook to store and update state in our server components.
For instance, we can use `useState` to store a counter value and update it whenever the component updates.
By adding hooks to our server components, we can make them more interactive and dynamic.
Recommended read: Nextjs App Router Hooks
Frequently Asked Questions
Can you use custom hooks in server components?
Unfortunately, custom hooks that rely on browser-specific features cannot be used in server components. However, you can still use custom hooks in client-side components, and we have more information on how to adapt your hooks for server-side rendering
Sources
- https://stackoverflow.com/questions/67293649/how-to-use-hook-in-server-side-render-on-next-js
- https://medium.com/@turingvang/can-you-use-hooks-in-server-components-of-nextjs-7630ff70e0e1
- https://react.dev/reference/rsc/server-components
- https://www.dhiwise.com/post/nextjs-useeffect-guide-for-managing-side-effects
- https://brenelz.com/posts/react-server-components-with-next/
Featured Images: pexels.com