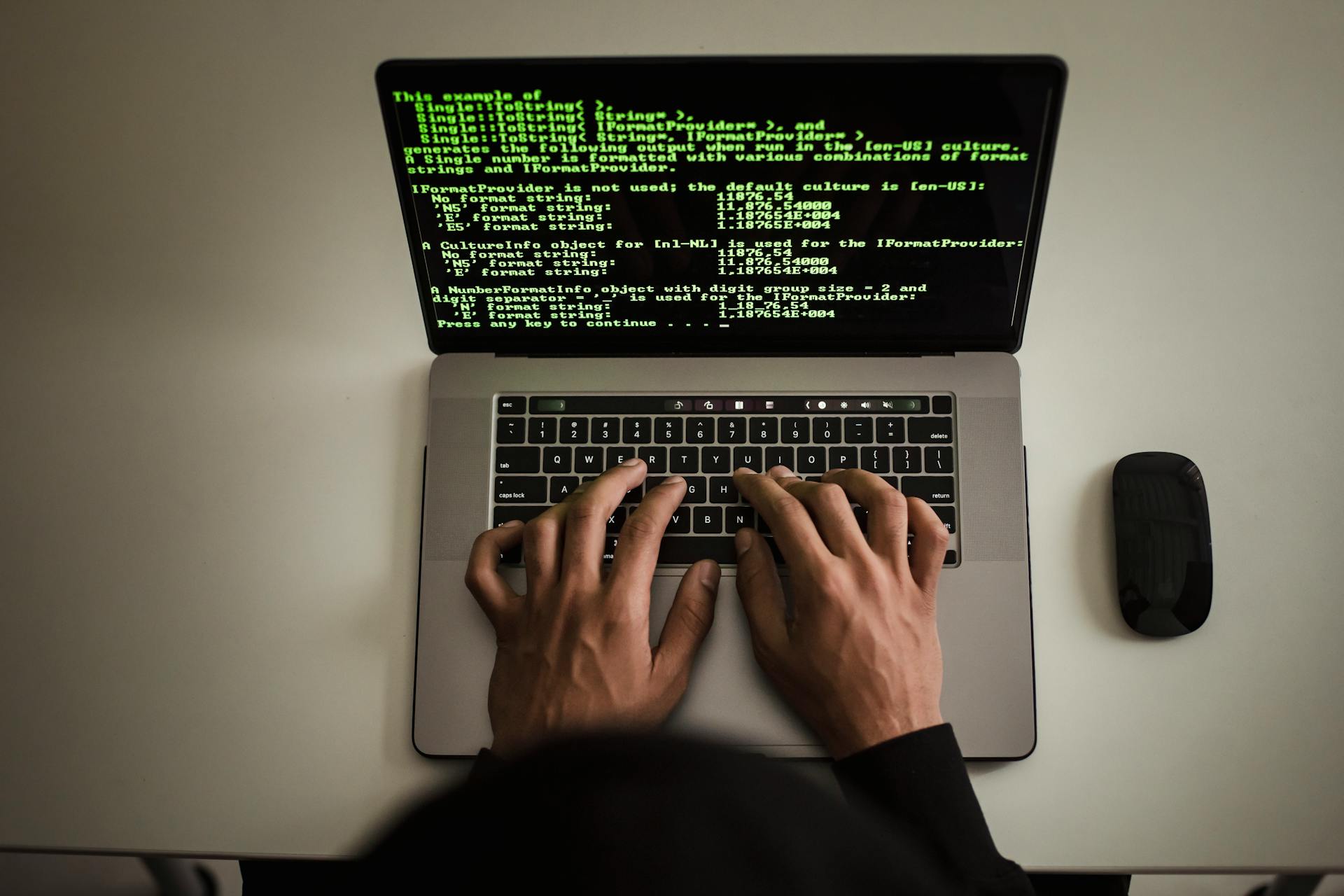
Next.js micro frontend development is a game-changer for building scalable and maintainable applications.
With Next.js, you can create a single-page application that acts as a container for multiple micro frontends, each with its own routing and lifecycle.
This approach allows for greater flexibility and efficiency in development, as each micro frontend can be built and deployed independently.
Next.js provides built-in support for micro frontend architecture through its use of modules and isolated components.
On a similar theme: Front End Web Programming
Module Federation
Module Federation is a feature introduced in Webpack 5 that enables developers to dynamically share and consume JavaScript modules across multiple applications at runtime. This approach to code sharing allows for better collaboration between development teams working on separate parts of an application.
By using Module Federation with Next.js, the application can be divided into a Host app and one or more Remote apps. The Host app is responsible for rendering the application shell and can consume code from the Remote apps.
See what others are reading: Nextjs Module Federation
Each remote component can have its own getServerSideProps, meaning that remote components can be responsible for both data fetching and rendering their data. This enables development teams to work autonomously on their respective micro frontends, leading to more efficient collaboration and faster development cycles.
In a typical micro frontend architecture utilizing Module Federation, each page may import multiple modules from remote applications. However, it is quite common for a page to import only one primary module that represents the complete page or a significant portion of it from a remote application.
This approach has several advantages, including:
- Simplified Integration: Importing a single module per page simplifies the integration process, as there are fewer dependencies to manage and coordinate.
- Clear Responsibility: Each remote application is responsible for a specific page or functionality, resulting in a clear separation of concerns and making it easier to understand and maintain the overall application.
- Faster Load Times: Loading a single module per page can potentially reduce the amount of data fetched and processed by the browser, leading to improved performance and faster load times.
- Enhanced Autonomy: Development teams can work independently on their respective pages or micro frontends without affecting other parts of the application, fostering better collaboration and efficient development processes.
- Easier Updates: With a single module per page, updates or changes can be made in isolation without impacting other pages, streamlining the deployment process.
Setting Up
Setting up a Next.js micro frontend involves creating two empty projects, one for the Host and one for the Remote, and installing the necessary module federation package.
To start, you'll need to add the NextFederationPlugin to the Remote app's next.config.js file. This is where you define the modules that need to be shared between the Host and Remote apps.
Expand your knowledge: Can Vercel Host Nextjs Servers
The NextFederationPlugin is imported from @module-federation/nextjs-mf and added to the config object. You'll also need to specify the exposed pages and components that should be shared.
Here's an example of what the Remote app's next.config.js file might look like:
```javascript
const { NextFederationPlugin } = require('@module-federation/nextjs-mf');
const nextConfig = {
reactStrictMode: true,
webpack(config, { isServer }) {
config.plugins.push(
new NextFederationPlugin({
name: 'remote',
filename: 'static/chunks/remoteEntry.js',
exposes: {
'./SomePage': './pages/somePage.js',
'./SomeComponent': './components/someComponent.js'
},
shared: {
// specify shared dependencies
},
})
);
return config;
},
};
```
Next, you'll need to add the NextFederationPlugin to the Host app's next.config.js file. This is where you specify the remotes that should be consumed by the Host app.
The remotes are defined using a function that returns an object with the remote's name and location. In this case, the remote is located at http://localhost:3001/_next/static/chunks/remoteEntry.js.
Here's an example of what the Host app's next.config.js file might look like:
```javascript
const { NextFederationPlugin } = require('@module-federation/nextjs-mf');
const remotes = (isServer) => {
const location = isServer ? 'ssr' : 'chunks';
return {
remote: `remote@http://localhost:3001/_next/static/${location}/remoteEntry.js`,
On a similar theme: Next Js Chat App
};
};
const nextConfig = {
reactStrictMode: true,
webpack(config, { isServer }) {
config.plugins.push(
new NextFederationPlugin({
name: 'host',
filename: 'static/chunks/remoteEntry.js',
remotes: remotes(isServer),
exposes: {
// Host app also can expose modules
}
})
);
return config;
},
};
```
With the NextFederationPlugin configured in both the Host and Remote apps, you can now consume the shared modules in the Host app using the next/dynamic function.
Related reading: Stripe Nextjs
Demo Project
A demo project is available for Module Federation with Next.js and Chakra UI.
You can find the demo project, which showcases how to use Module Federation with Next.js and Chakra UI, by following the link provided.
The demo project is a great way to get hands-on experience with Module Federation and see how it can be used in a real-world application.
You might like: Next Js Movie Streaming Ui
Micro Frontends
Micro frontends are a modern approach to developing web applications by breaking down large, complex frontends into smaller, more manageable pieces.
This allows for the division of a large application into smaller, self-contained services that can be developed, deployed, and maintained independently.
Each micro frontend is responsible for a specific part of the application's functionality, such as authentication, product listings, or shopping carts.
This approach enables different teams to work on different parts of the application simultaneously without interfering with each other.
Each team has the autonomy to choose its own technology stack, allowing them to use the best tools for their specific needs.
This approach also enables independent testing and deployment of each module, speeding up development and making it easier to manage.
Micro frontends can be built with different frameworks or technologies, allowing developers to select the best tools for their specific requirements.
By breaking down the frontend into smaller units, micro frontends make it easier to maintain the life cycle of the development process.
Micro frontends are an extension of the microservices architectural style to the frontend world, applying the same principles of breaking down complex systems into smaller, manageable pieces.
Additional reading: Can Nextjs Be Used on Traditional Web Application
Exploring Concepts
Breaking down large, complex front ends into smaller, manageable pieces is a modern approach to developing web applications. This is the core idea behind micro front ends.
Traditional practices often have a single team handling the entire user interface, which can make it difficult for applications to grow. This problem is solved by dividing the front end into discrete modules or components.
Each set of modules is responsible for specific parts of the application's functionality, such as authentication, product listings, or shopping carts. This approach allows for independent development, deployment, and maintenance of each module.
Different teams can work on different parts of the application simultaneously, without stepping on each other's toes. This is because each team has autonomy to choose its own technology stack.
Independent testing and deployment of each module can speed up development and make it easier to manage. This is a key benefit of the micro front end approach.
A unique perspective: Nextjs Team
Benefits and Features
Next.js micro frontend offers numerous benefits that boost the development process along with the user experience. Scalability is one of the primary advantages, achieved by breaking down large and complex applications into smaller, independently deployable modules.
This modular approach aligns well with Next.js's capabilities, supporting server-side rendering (SSR) and static site generation (SSG). These features allow each micro front end to leverage improved performance and SEO.
The flexibility of choosing different technologies or frameworks within different modules is another added benefit. Developers can select the best tools for their specific needs without being constrained by a single technology stack.
Each set of micro front end can be updated and deployed independently, reducing the risk of deploying errors across the entire application. This also allows for quicker iterations and bug fixes.
Dynamic imports and code-splitting in Next.js optimize load times by ensuring only the necessary code is loaded for each micro front end.
WunderGraph Integration
WunderGraph is an open-source framework that enables the creation of micro frontends in Next.js applications. It simplifies the process of integrating multiple frontends into a single application.
WunderGraph uses GraphQL to manage data and APIs, allowing for a more efficient and scalable architecture. This is particularly useful in Next.js micro frontend architecture.
Discover more: Next Js Aws Amplify Gen2 Architecture Diagram
WunderGraph's integration with Next.js can be achieved through the use of a WunderGraph client, which handles API requests and caching. This reduces the load on the server and improves application performance.
By using WunderGraph, developers can create a more modular and maintainable codebase, which is essential in Next.js micro frontend architecture.
Backend and API
In a Next.js micro frontend, the backend and API play a crucial role. WunderGraph serves as the Backend-for-Frontend server, acting as an API gateway that coordinates calls between the frontend and backend.
This setup eliminates the need for the frontend to interact directly with backend APIs, simplifying the development process. WunderGraph consolidates data from various datasources into a unified API layer.
With WunderGraph, you can use GraphQL queries or TypeScript Operations to retrieve data from the unified API layer. This allows for efficient data fetching and manipulation.
The unified API layer can be configured to include auth and middleware, further streamlining the development process.
For more insights, see: Is Next Js Frontend or Backend
Dependencies and Setup
In a Next.js micro frontend setup, dependencies play a crucial role. Shared dependencies can be used across different apps or components, avoiding duplicating resources and keeping things running smoothly.
The Module Federation Plugin configuration has a shared option that lets developers mark certain dependencies as shared resources. This means that if a host app has a dependency, the remote app will depend on it, and if the host doesn't have it, the remote will download its own version.
Using the shared option, you can mark dependencies like CSS-in-JS, state management, or data-fetching libraries as shared resources. For example, a shared Chakra UI library can be set up using the singleton option to ensure only one instance is used across all federated applications.
To add microservices as data dependencies, you'll need to add them to the wundergraph.config.ts file, pointing them at the OpenAPI spec JSONs, and including them in the configureWunderGraphApplication dependency array. This will make your data dependencies available to all federated applications.
If this caught your attention, see: Next Js Google Fonts
Frequently Asked Questions
Does Netflix use micro frontend?
Yes, Netflix has publicly shared its implementation of micro-frontends for its applications. This approach allows for more flexibility and scalability in their user interface.
Are micro frontends a good idea?
Yes, micro frontends are a good idea for large and complex web applications, offering flexibility and faster iterations. They enable independent development of application modules, boosting agility and scalability.
Sources
- https://single-spa.js.org/docs/ssr-overview/
- https://alibek.dev/micro-frontends-with-nextjs-and-module-federation
- https://thekevinwang.com/2021/03/26/micro-frontends-nextjs
- https://pattemdigital.com/insight/next-js-micro-frontend-web-development/
- https://wundergraph.com/blog/the-backend-for-frontend-pattern-using-nextjs
Featured Images: pexels.com