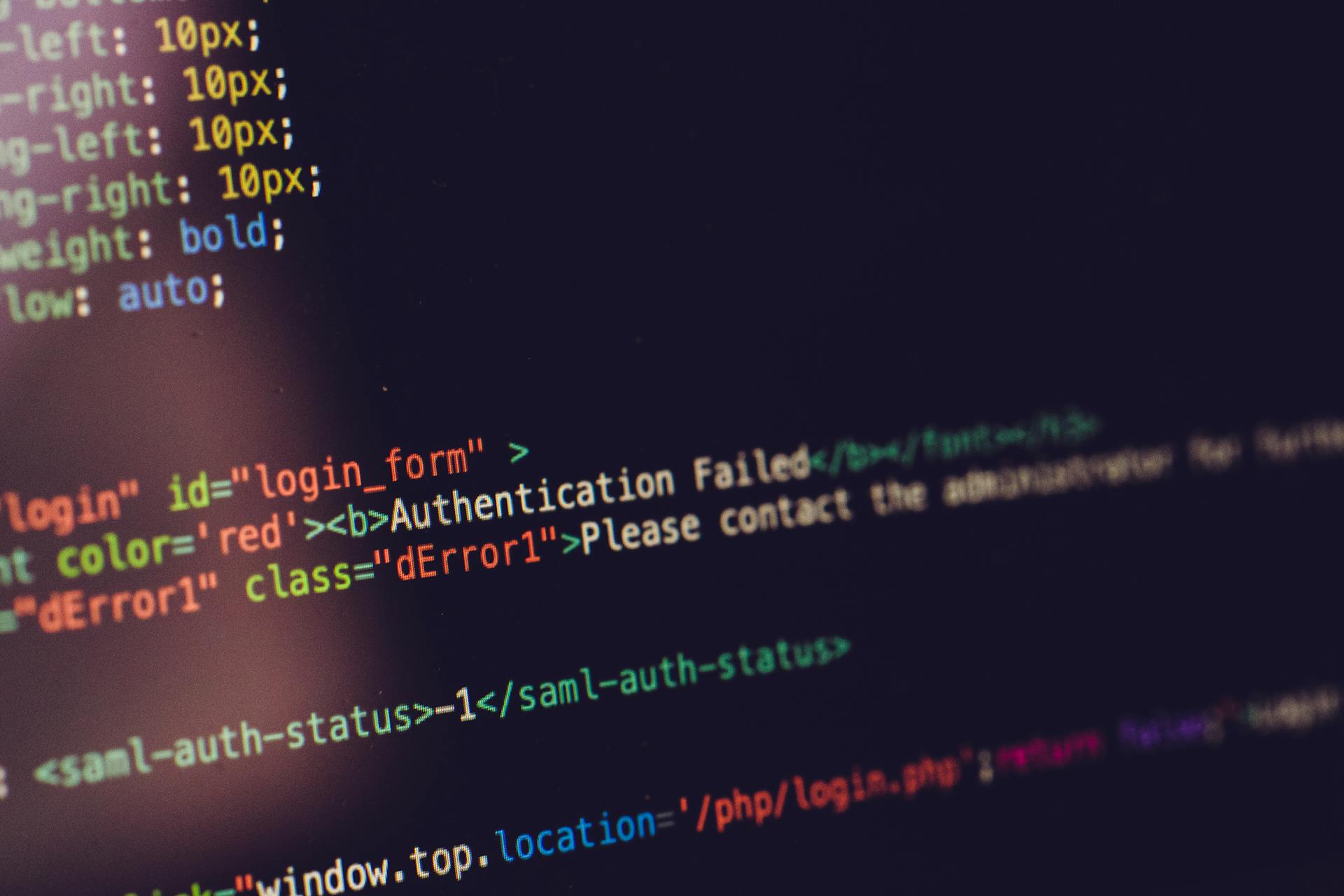
Handling 404 errors in Nextjs is crucial for a seamless user experience. A well-crafted 404 page can make a significant difference in how users perceive your application.
To get started, you can use the built-in `getStaticProps` method to handle 404 errors. This method allows you to pre-render pages at build time, making it ideal for handling 404 errors.
By default, Nextjs uses the `getStaticProps` method to handle 404 errors, but you can also use the `useRouter` hook to catch and handle 404 errors. The `useRouter` hook provides a way to access the current route, allowing you to detect and handle 404 errors.
In Nextjs, you can use the `next/config` module to configure access control for your application. This module provides a way to specify access control rules for your application, ensuring that sensitive data is protected.
Check this out: Why Use Nextjs
Error Handling and Access Control
In NextJS, you can use the "notFound" function to hide certain pages from certain users, like hiding the admin page from a normal user. This is done by creating a "not-found.js" file inside the route segment where the page you want to hide is located.
Worth a look: Nextjs App Loading Page Not Working
The "not-found.js" file defines the UI shown to the user when they try to access a page that doesn't exist. Next.js will return a 200 HTTP status code for streamed responses, and a 404 HTTP status code for non-streamed responses.
Components defined in "not-found.js" cannot receive or utilize any external data passed as props, which means they are static in nature. This is because "not-found.js" components do not accept any props.
To implement the "not-found" file convention in Next JS, start the development server by running a command in your project directory. This will allow you to test your "not-found" page in action.
When a user goes to an unmatched URL that is not defined by the application, the root "not-found.js" file gets rendered, showing the user the "NotFoundPage" UI. This can be seen in action when you try to access a URL like "http://localhost:3000/abcd" that is not defined by your application.
For more insights, see: Next Js Referenceerror Window Is Not Defined
Implementing Not-Found Page
To implement a not-found page in Next.js, you can create a "not-found.js" file inside the app directory, which will serve as a default page for unmatched URLs.
This file will provide users with a link to go back to the home page.
When a user visits an unmatched route, such as "http://localhost:3000/abcd", they will be shown the "NotFoundPage" UI.
You can also use the "global" class to style the not-found page with a specific design, like the red color used in the "app/not-found.tsx" file.
In addition to creating a not-found page for the root URL, you can also implement a global 404 error page that applies to all routes, including child routes.
For example, accessing the "/about/test" URL will trigger the red-colored not-found page.
To implement a global 404 page, create a "not-found.tsx" file in the "app" folder and add a link to a non-existing route in the "layout.tsx" file.
This will display the global not-found page when routes are not found, providing a consistent user experience.
See what others are reading: Link Nextjs
Dynamic Routes
Dynamic routes are a powerful feature in Next.js that allow you to create flexible and scalable routes. You can create a dynamic route by wrapping a folder's name in square brackets: [folderName]. For example, you can access dynamic user profiles like '/user/1', '/user/2', etc.
To validate the user ID, you can implement a rule that restricts the ID to a range between 0 and 10. If an invalid ID is provided, you can use the 'notFound' function from "next/navigation" to throw a 'NEXT_NOT_FOUND' error.
You can also provide a unique 'not-found' page for incorrect dynamic route IDs by adding a 'not-found.tsx' file within the '[id]' folder. By modifying the className to 'unique', the content will now render in green.
To create a global 404 page, you can add a 'not-found.tsx' file in the 'app' folder and style it with a red color to indicate its global usage. This global 404 page will be displayed when routes are not found and also applies to child routes.
Broaden your view: Next Js Localstorage Is Not Defined
Module Error Handling
Module not found errors in Next.js often occur when you're importing a module that's not accessible on the client side, like the fs module.
To resolve this, keep all Node.js/server-related code inside Next.js data fetching APIs like getServerSideProps, getStaticPaths, or getStaticProps.
You can also modify your next.config.js file to include an entry for webpack, which will determine if the build is for the server-side or client-side and add the fs module to the fallback list if it's not for the server-side.
If you're not using Node.js modules and still get the error, ensure the module has been installed and imported properly, and double-check for any casing errors.
In cases where the error is caused by a package you imported, modifying the next.config.js file can resolve the issue.
Worth a look: Next Js Document Is Not Defined
Sources
- https://www.geeksforgeeks.org/next-js-file-conventions-not-found-js/
- https://medium.com/@a.pirus/custom-loading-and-404-pages-in-next-js-13-tutorial-f864dd0f8801
- https://blog.sentry.io/common-errors-in-next-js-and-how-to-resolve-them/
- https://next-auth.js.org/errors
- https://clerk.com/docs/references/nextjs/clerk-middleware
Featured Images: pexels.com