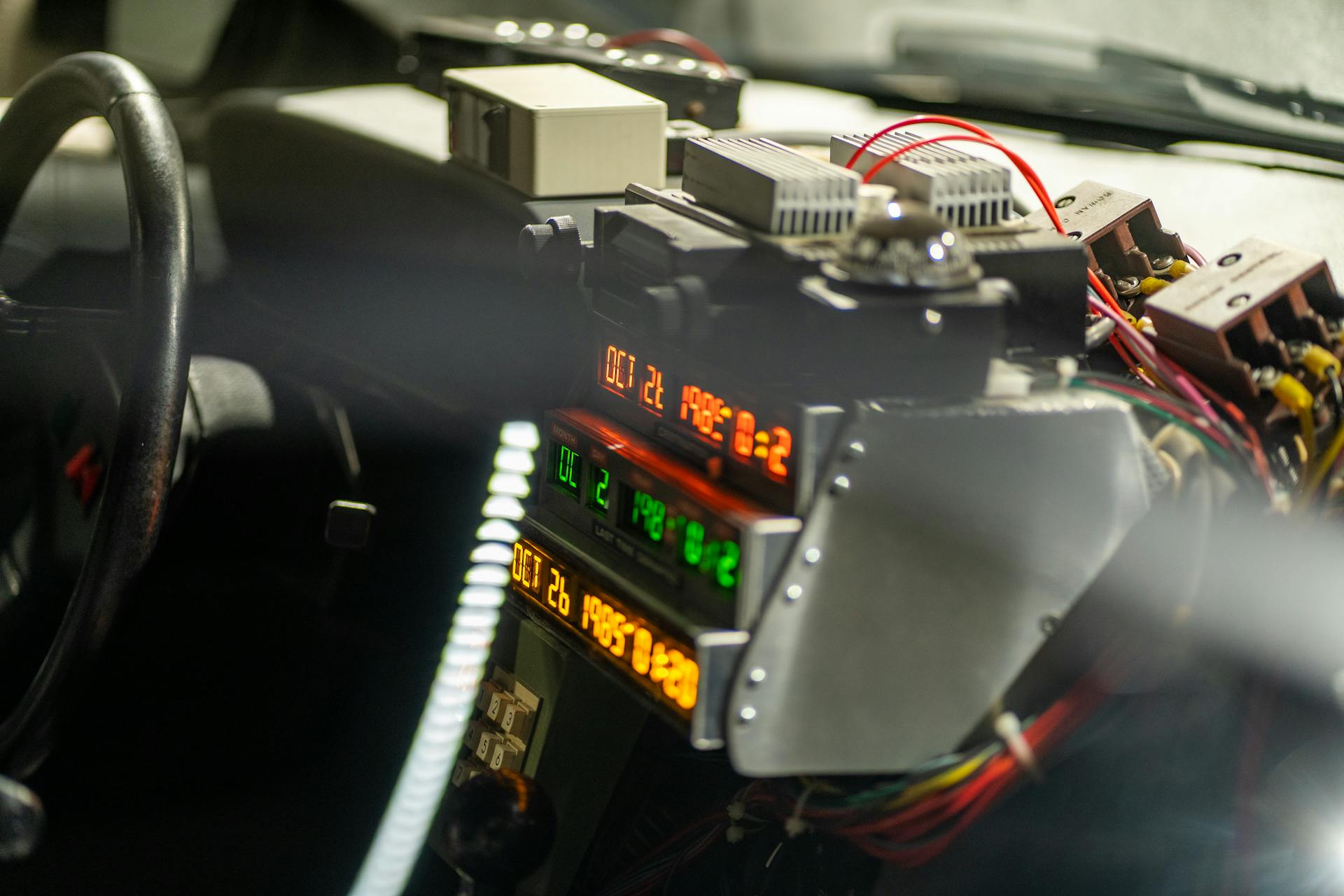
Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications. To create a database-driven application, we'll use Postgres, a powerful and flexible open-source relational database.
Next.js provides a built-in API route feature that allows us to create API endpoints for interacting with our database. This feature is enabled by default in Next.js 10 and later versions. We can create API routes using the `pages/api` directory.
To connect our Next.js application to a Postgres database, we'll use the `pg` library, which is a popular and well-maintained Postgres driver for Node.js. We'll also use the `next-auth` library to handle authentication and authorization in our application.
Worth a look: Why Use Nextjs
Project Setup
To set up your Next.js project, you need to verify the connection to your PostgreSQL database. This is done later when setting up Prisma. Ensure your Next.js application can connect to the PostgreSQL database by testing the connection.
You'll also need to create a Next.js application. To do this, ensure you have Node and npm installed in your development environment. Then, create a new directory called prisma-next with the command `npx create-next-app prisma-next`.
Suggestion: Next Js Prisma
Once the directory is created, navigate to it and start the development server using `npm run dev`. This will start a development server at http://localhost:3000.
Here are the steps to create a Next.js application in a concise list:
- Create a new directory called prisma-next with `npx create-next-app prisma-next`.
- Navigate to the prisma-next directory.
- Start the development server using `npm run dev`.
By following these steps, you'll have a basic Next.js application set up and ready to go.
Database Configuration
To start using your PostgreSQL database with Next.js, you'll need to configure Prisma to connect to it. This involves setting up the prisma.schema file and configuring the database connection.
First, open the prisma/schema.prisma file and define your database models and connection settings. You'll need to configure the datasource db block to use the database connection URL from your .env file. This URL tells Prisma how to connect to your PostgreSQL database.
You can find the connection URL format in the documentation, which is to replace the elements in curly brackets with your own database details and save it in the .env file. Then, in schema.prisma, specify the database connection URL.
Expand your knowledge: Nextjs Database
To test the connection, start your Next.js development server and visit http://localhost:3000/todos. You'll get an empty page, which is expected since you need to create the table and fill it in with some data!
Here are the steps to create the table and insert some sample tasks:
1. Go to the Supabase Dashboard and copy the Project URL and API Key into an .env file.
2. Create a table named todo and insert some sample tasks in the SQL Editor.
Once you've created the table, you can use Prisma's basic APIs for create, delete, update, and read to interact with your database efficiently. These APIs are: create, delete, update, and read, which are accessible through the PrismaClient.
Data Management
Data Management in Next.js with Postgres is a breeze. You can create new records in your PostgreSQL database using Prisma and Next.js by defining an API route in your Next.js application to handle POST requests for creating new data.
Expand your knowledge: Next Js Drizzle Supabase
To create an API route, you'll need to use the Prisma client to insert the new record into the database. This involves setting up an API route in your Next.js application to handle POST requests for creating new data, such as creating a new post.
To fetch data from your PostgreSQL database, you can use either server-side rendering (SSR) or static site generation (SSG). Here's a brief overview of how to fetch and display data using both methods:
To get the database data into the view, you'll need to install @prisma/client and run the command npx prisma generate. This will generate the TypeScript or JavaScript code corresponding to the table structure, the functions used to perform database queries, inserts, updates, and deletes, as well as the associated type definitions.
Related reading: Nextjs Prisma Docker
Creating Models
Creating models is a crucial step in defining your database schema with Prisma. You need to open the prisma.schema file and define your models that correspond to the tables in your PostgreSQL database.
To define your models, you can create a User model to represent users in your application. The User model has fields for id, name, and email, with id set as the primary key and email marked as unique.
You can also specify default values and constraints for your fields, such as @default(autoincrement()) for auto-incrementing IDs and @unique for unique fields.
Here's an example of how to define a User model:
```html
model User {
id String @id @default(cuid())
name String
email String @unique
}
```
This code defines a User model with id, name, and email fields, where id is the primary key and email is a unique field.
Similarly, you can define other models, such as a Post model, that includes fields for id, title, content, authorId, and a relation to the User model.
Here's an example of how to define a Post model:
```html
model Post {
id String @id @default(cuid())
title String
content String
authorId String @relation(fields: [authorId], references: [id])
author User
}
```
This code defines a Post model with id, title, content, and authorId fields, where authorId is a relation to the User model.
By defining your models, you can create a clear and organized database schema that reflects your application's data structure.
Deleting Data
Deleting data is an essential part of data management. To do this, you need to create an API route that can handle DELETE requests.
This API route will allow you to delete specific records from your database. To create it, you'll need to follow a few steps, which are outlined below.
To delete records, you'll need to create an API route to handle DELETE requests. This is typically done by using a specific HTTP method, such as DELETE.
Here's a basic example of how to create a DELETE API route:
- API Route for Deleting Data:
Note that the specifics of creating a DELETE API route will depend on the framework and programming language you're using.
Environment Setup
To set up your environment for a Next.js application with a PostgreSQL database, you need to configure environment variables. Create an .env file in the root directory of your project to store sensitive information.
This file will store your database connection URL, so replace user, password, localhost, and mydatabase with your actual PostgreSQL credentials and database name. Update next.config.js to load these environment variables.
To do this, add the following configuration to your next.config.js file: This ensures that your application can access the DATABASE_URL during runtime.
You'll also need to install the Prisma Client, which includes the prisma and @prisma/client packages. Install these via npm and initialize Prisma by running the npx prisma init command on the terminal. This will generate a new file called schema.prisma and a .env file to which you’ll add the database connection URL.
Here's a quick rundown of the steps:
Installing the Client
To install the Prisma Client, you'll need to install the prisma and @prisma/client packages via npm.
You'll also need to initialize Prisma by running the npx prisma init command on the terminal.
This command will generate a new file called schema.prisma, which contains the database schema.
A .env file will also be generated, where you'll add the database connection URL.
By following these steps, you'll be ready to start using Prisma and querying your database.
Setting Up Environment Variables
To set up environment variables, you need to create an .env file in the root directory of your project. This file will store your environment variables.
You'll need to create an .env file in the root directory of your project, and replace user, password, localhost, and mydatabase with your actual PostgreSQL credentials and database name.
In your next.config.js file, add the following configuration to ensure that Next.js is configured to load these environment variables. This ensures that your application can access the DATABASE_URL during runtime.
Here's a simple way to do this:
- Create an .env file in the root directory of your project.
- In the .env file, add your environment variables, such as DATABASE_URL.
- In your next.config.js file, add the configuration to load the environment variables.
By following these steps, you'll be able to securely store your database connection URL and make it accessible to your Next.js application.
API Integration
API Integration is a crucial aspect of building a Next.js application with Postgres. You can use API routes to handle CRUD operations for your database.
API routes are essential for handling CRUD operations in your Next.js application. To set up an API route, you can create a new folder in the pages/api directory and add a file with a handler function.
A different take: Nextjs Crud
In a Next.js application, you can set up an API route that uses Prisma to fetch data from the database and return it to the client. Prisma is typically used on the server side, where it can safely interact with your database.
To create an API route with Prisma, you need to create a new file in the pages/api/db directory and add a handler function. This function can use the create method provided by the Prisma Client to create a new user record in the User table.
You can also use the Prisma client instance to query the database in your Next.js application. To do this, you need to generate the Prisma client and create a new file in the lib directory with a prisma.js file.
Here's a step-by-step guide to creating a Prisma client instance:
1. Generate the Prisma client.
2. Create a new folder called lib and add a new file named prisma.js.
3. In the prisma.js file, add the code to create a Prisma client instance.
With the Prisma client instance created, you can now import it in your files and start querying the database. For example, you can create a new folder named profile in the app directory and add a new file named page.js. In this file, you can add a simple form containing two input boxes for the name and email and a submit button.
For another approach, see: Routes in Nextjs
Here's a list of the steps to create an API route with Prisma:
- Create a new folder in the pages/api directory and add a file with a handler function.
- Use the create method provided by the Prisma Client to create a new user record in the User table.
- Generate the Prisma client and create a new file in the lib directory with a prisma.js file.
- Create a Prisma client instance in the prisma.js file.
Sources
- https://fly.io/docs/js/frameworks/nextjs/
- https://www.dhiwise.com/post/building-a-full-stack-app-with-nextjs-postgres-and-prisma
- https://www.makeuseof.com/postgresql-connect-nextjs-application-using-prisma/
- https://v1docs.withcoherence.com/docs/getting-started/create-nextjs-postgres-full-stack-app
- https://blog.stackademic.com/explore-full-stack-magic-next-js-prisma-next-auth-vercel-postgres-951a9511702f
Featured Images: pexels.com