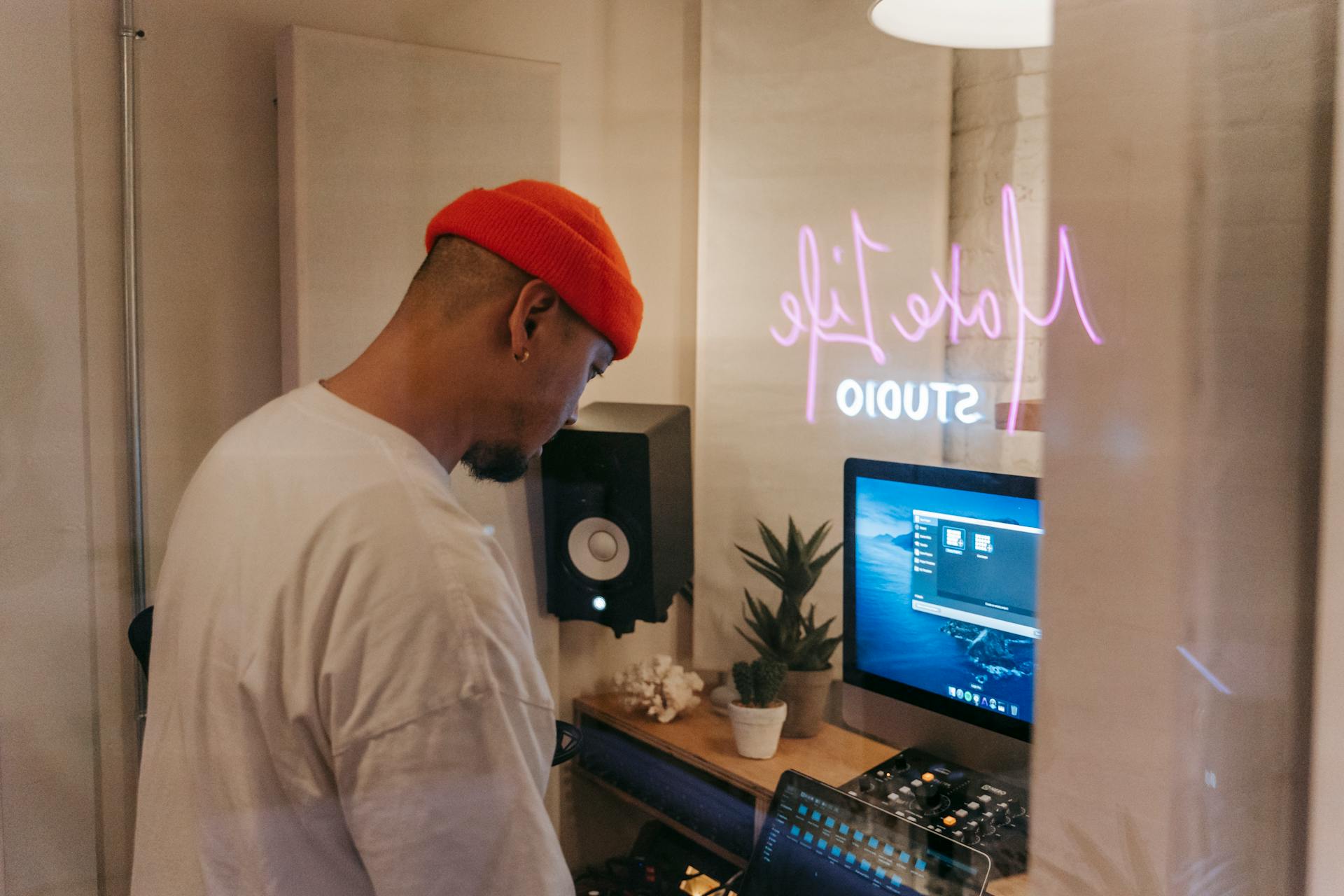
Next.js upload 组件 makes it easy to add file upload functionality to your Next.js applications.
To use Next.js upload 组件, you need to install the 'next-upload' package.
With Next.js upload 组件, you can upload files of various types, including images, videos, and documents.
A different take: Next Js Draggable
Getting Started
To get started with nextjs upload 组件, you'll need to create a NextUploadConfig that defines how to connect to a storage service, like AWS S3.
First, create a file called config.ts in your src/app/upload directory and define your storage service settings there.
You can use any S3 compatible service, not just AWS S3.
Next, create an HTTP route that will handle next-upload related requests, such as generating signed URLs.
In the example, a POST route at /upload is used with the Next.js App router.
If you're using the Pages router or a different framework, you can use the NextUpload.pagesApiHandler or NextUpload.rawHandler functions directly.
Once you've set up your NextUploadConfig and HTTP route, you can import helper functions from next-upload/client to send files to your storage service with just one line of code.
You can use these helper functions to send files to your storage service, but how your application handles file-uploads in the browser is up to you.
The example uses react-dropzone to send files to storage via the upload function.
See what others are reading: Nextjs File Upload Api
Upload Methods
Next.js upload components offer several upload methods, including the `useForm` hook from `react-hook-form`. This hook allows for easy form management and validation.
You can also use the `input` element with the `multiple` attribute to allow users to select multiple files for upload. For example, you can create a file input element with the `multiple` attribute set to `true`.
By using these methods, you can create a seamless and efficient file upload experience for your users.
Check this out: Nextjs Server Actions File Upload
Upload to Cloudinary
To upload a video to Cloudinary, you'll need to use their Node.js SDK. This means you'll need to receive the video file, which can be done by taking three steps in your serverless function.
First, you'll need to overwrite the Vercel serverless function behavior, which by default uses bodyParser. This will allow you to access the uploaded files.
Next, you'll need to add formidable to your serverless function, which will enable you to parse form data and access the uploaded files.
Finally, you'll need to upload the file to Cloudinary using cloudinary.v2.uploader.upload(). This can be done in your serverless function using the following code: const file = data?.files?.inputFile.path;
To test the serverless function, you can use a tool like Postman or Insomnia. When adding a video via these tools, make sure to use the inputFile key, as this is what your serverless function is referencing.
Here are the steps to upload to Cloudinary in a concise list:
- Overwrite the Vercel serverless function behavior
- Add formidable to parse form data
- Upload the file to Cloudinary using cloudinary.v2.uploader.upload()
Upload
Uploading files to a serverless function is a bit more involved than you'd think, but don't worry, I've got the lowdown.
To upload a video to Cloudinary, you'll need to use their Node.js SDK, which can be used in a Vercel serverless function. However, you'll need to overwrite the default Vercel serverless function behavior and add formidable to access the uploaded files.
You'll also need to add an event handler to the form to handle the file upload, which will utilise the FormData WebAPI. This will allow you to send the file to the serverless function created earlier.
Here are the steps to upload a file to Cloudinary:
- Overwrite the Vercel serverless function behavior by setting bodyParser: false
- Add formidable to parse the form data
- Use the Cloudinary SDK to upload the file
In the code, you'll see that the file is uploaded via `cloudinary.v2.uploader.upload()`. To test the serverless function, you can use a tool like Postman or Insomnia and add the inputFile key to the request.
The next-upload package is a turn-key solution for integrating Next.js with signed & secure file-uploads to an S3 compliant storage service. It generates signed URLs for uploading files directly to your storage service and optionally integrates with your database to store additional metadata about your files.
File Handling
When you need to handle file uploads in Next.js, you can use Server Actions to simplify the process. You can let users select a photo and upload it, and the server will store it locally in the uploads directory.
The Server Action uploadFile receives the user-submitted form data, retrieves the uploaded file details, and saves the file to the uploads directory. This is a straightforward way to handle file uploads.
Worth a look: Use Theme in Server Components Nextjs
To enable Server Actions, you need to modify next.config.js as shown in the example. This allows the server to handle file uploads and store them locally.
By using Server Actions, you can easily upload files and store them on the server. This is especially useful when you need to handle large files or files that require server-side processing.
To upload a file, simply click on Choose File to select the photo, and then click Upload file. This will trigger the server action and create the file in the uploads directory.
Verification and Policy
You can mark an upload as verified once it has been processed by your application. To enable verification, set the verifyAssets config to true.
This will set the verified property of any uploaded file to false by default. Once you've processed the file, you can mark it as verified by calling NextUpload.verifyAsset(id).
Verifying
Verifying an upload is a straightforward process. To enable verification, set the verifyAssets config to true. This will allow you to mark an upload as verified once it has been processed by your application.
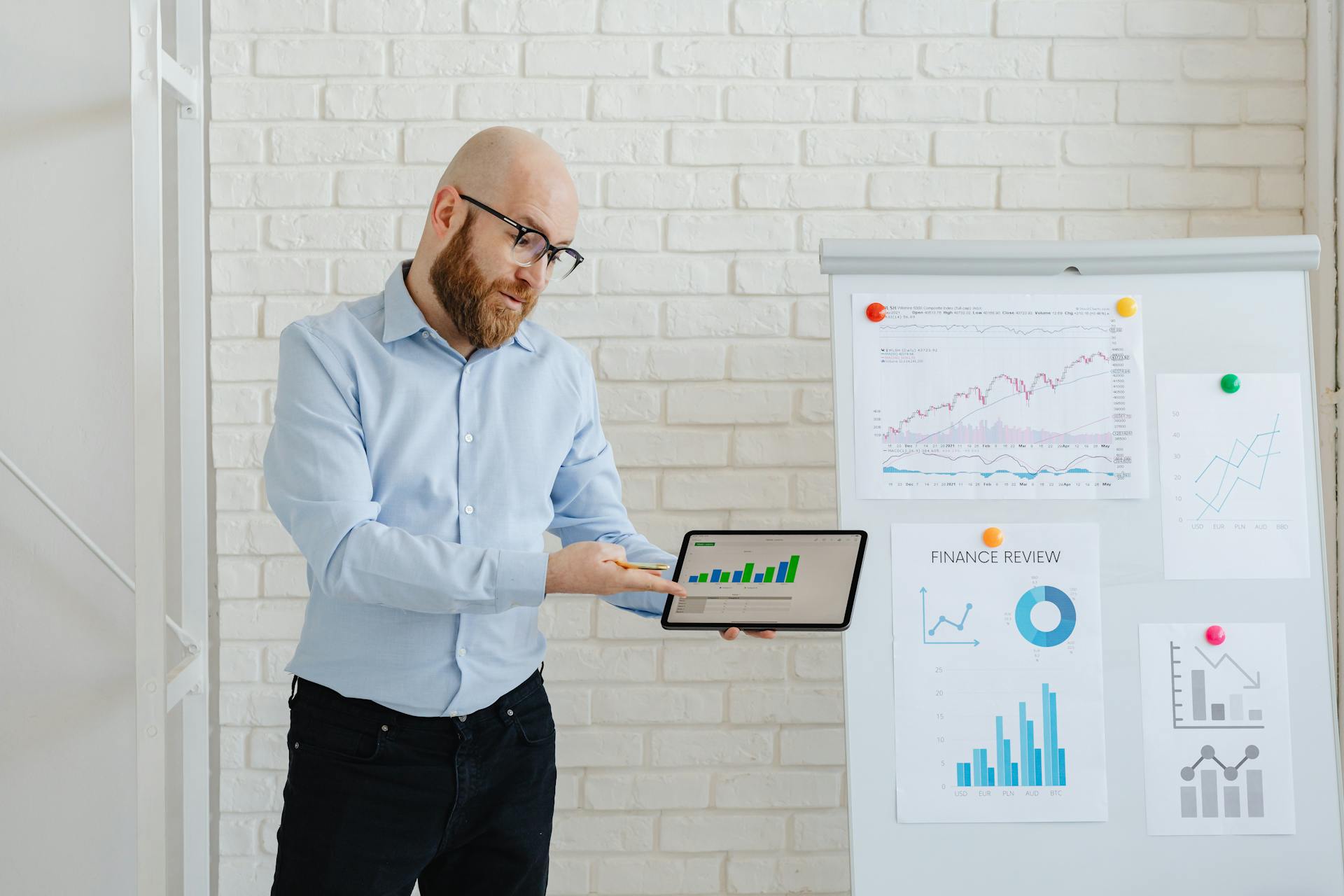
You'll need to instantiate NextUpload with an Store instance to get started. This will enable the verified property on any file that is uploaded, which will be set to false by default.
Once you've processed the file, you can mark it as verified by calling NextUpload.verifyAsset(id). This will update the verified property to true.
Generate Post Policy
Generating a post policy is a crucial step in the verification and policy process. This process involves using a specific method to create a signed post policy.
The method used to generate a post policy is called generatePresignedPostPolicy, which is a type of function that returns a promise. This promise resolves to an object containing a signed post policy.
The generatePresignedPostPolicy method can be called with two different sets of arguments: any and NextToolRequest, or GeneratePresignedPostPolicyArgs and NextUploadRequest.
See what others are reading: Nextjs Promise
Featured Images: pexels.com