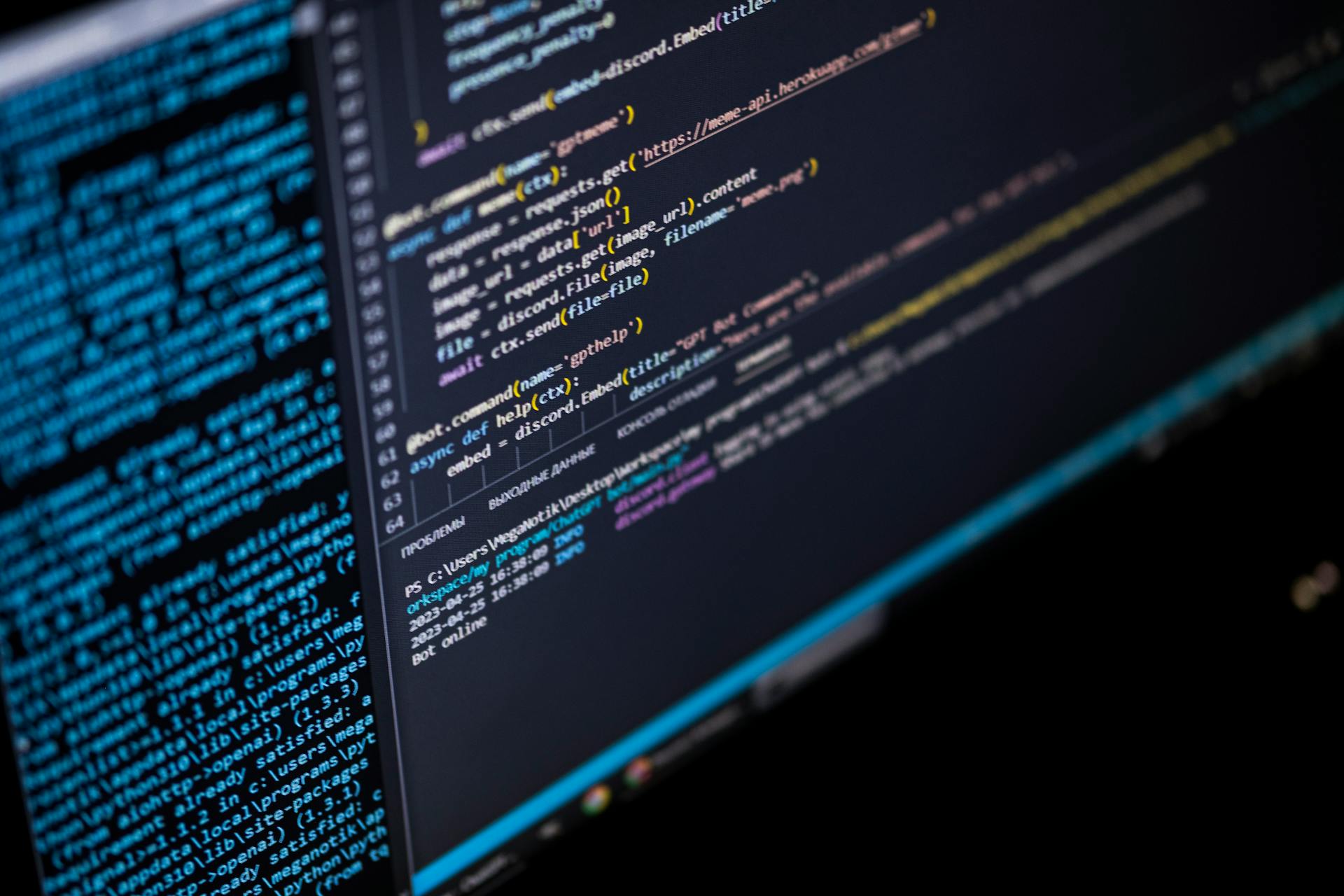
Using Next.js with Promise and Async/Await in React Apps can be a game-changer for handling asynchronous operations.
In Next.js, promises are used to handle asynchronous operations, and async/await syntax is the recommended way to write promise-based code.
Async/await allows you to write asynchronous code that's easier to read and maintain, making it a great choice for Next.js developers.
By using async/await, you can write code that's more readable and maintainable, which is especially important when working with complex asynchronous operations.
On a similar theme: Next Js Using State Context
Async Programming in Next.js
Async programming in Next.js allows for non-blocking I/O operations, improving performance and responsiveness.
This is achieved by using callbacks, promises, and async/await syntax.
In Next.js, you can use the fetch API to make asynchronous requests to an API endpoint. The fetch API returns a promise that resolves to the response data.
Async programming in Next.js is particularly useful when working with APIs, as it enables your application to continue running while waiting for the API response.
Suggestion: Fetch Next Js
Understanding Async/Await in React
Async/Await in React is a game-changer for handling asynchronous code, allowing you to write non-blocking code that's much easier to read and maintain.
By using async/await, you can write asynchronous code that looks and feels like synchronous code, making it much simpler to understand and debug. This is because async/await provides a way to pause the execution of a function until a promise is resolved, making it feel like the code is running synchronously.
In Next.js, you can use async/await to fetch data from APIs, making it easier to handle asynchronous data fetching. For example, you can use the `fetch` API to make a request to an API endpoint, and then use async/await to wait for the response. This is demonstrated in the example code, where the `fetch` API is used to fetch data from a JSON API.
Async/await is also useful for handling errors in asynchronous code. By using try-catch blocks, you can catch and handle errors that occur when making asynchronous requests. This is shown in the example code, where a try-catch block is used to catch an error that occurs when making a request to an API endpoint.
A fresh viewpoint: Nextjs Code Block
Using async/await in Next.js requires you to use the `async` keyword when defining a function, and the `await` keyword when waiting for a promise to resolve. This is demonstrated in the example code, where the `async` keyword is used to define a function that makes a request to an API endpoint using the `fetch` API.
Check this out: Nextjs Script Async
Managing Async State with useState
Managing Async State with useState is a crucial aspect of handling asynchronous operations in Next.js.
In the previous section, we learned how to create a simple async function that fetches data from an API. We used the fetch API to make a GET request to a dummy API endpoint.
The data fetched from the API is then stored in the component's state using the useState hook. This allows us to update the component's UI based on the fetched data.
For instance, in the example code, we used the useState hook to store the fetched data in a state variable called "data". We then used this state variable to render a list of items in the component's UI.
Broaden your view: Nextjs App Route Get Ssr Data
However, managing async state can become complex when dealing with multiple async operations. This is where the concept of "stale state" comes into play. Stale state occurs when the component's state is outdated and doesn't reflect the latest changes.
To mitigate this issue, we can use the useEffect hook to re-run the async function whenever the component's dependencies change. This ensures that the component's state is always up-to-date.
Discover more: Using State in Next Js
Using Fetch Interval for Real-time Updates
You can use the FetchInterval to refetch the API on the client. This is useful for real-time updates.
To only run the refetch of the /api/time API on the client, you'll need to use a useEffect to set a flag. This flag is used to tell if you're on the client.
The flag is a ref called clientLoaded, which is set in the useEffect. You then check this ref in your useQuery query function.
If the clientLoaded ref is true, you know you're on the client and you get the data from the API. Otherwise, you're on the server or in the first render on the client, and you return the original timerPromise.
For another approach, see: Debug Nextjs Client Frontend Webstorm
This approach helps you determine whether you're on the client or server, which is essential for handling real-time updates.
In this case, the clientLoaded ref is used to decide whether to refetch the API or return the original data. This ensures that you only refetch the API when you're on the client.
Additional reading: Use Client Nextjs
Handling API Errors and Caching
Handling API Errors and Caching is a crucial aspect of Async Programming in Next.js. In the previous sections, we've seen how to handle API errors using try-catch blocks and error-boundary components.
When dealing with API errors, it's essential to handle them properly to prevent the application from crashing. As we learned earlier, using try-catch blocks can help catch and handle errors, but it's also important to display a meaningful error message to the user.
Caching is another important aspect of handling API errors. By caching API responses, you can prevent unnecessary API calls and reduce the load on your server. In Next.js, you can use the built-in caching feature to cache API responses for a certain amount of time.
Discover more: Nextjs Error Page
For example, in the previous section, we used the `useSWR` hook to cache API responses for 10 minutes. This helps reduce the number of API calls and improves the overall performance of the application.
However, caching can also lead to stale data if not implemented correctly. To avoid this, it's essential to implement a cache invalidation strategy. In the previous section, we saw how to use the `invalidate` function to invalidate the cache when the data changes.
In Next.js, you can also use the `getServerSideProps` method to cache API responses on the server-side. This helps improve the performance of the application by reducing the number of API calls.
You might like: Nextjs Performance
Refetching API Data
To refetch API data in a Next.js app, you'll need to use a flag to determine if you're on the client or server.
Using FetchInterval To Refetch The API On The Client is a key concept here. You can set a ref, like clientLoaded, and then use useEffect to set it in the client.
In your useQuery query function, check the clientLoaded ref to determine if you're on the client. If it's true, get the data from the API; otherwise, return the original timerPromise.
This approach ensures that the API refetch only happens on the client, which is exactly what you want.
Related reading: Next Js Client Side Rendering
Sources
- https://zustand.docs.pmnd.rs/integrations/persisting-store-data
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://swizec.com/blog/async-react-with-nextjs-13/
- https://blog.sentry.io/common-errors-in-next-js-and-how-to-resolve-them/
- https://javascript.plainenglish.io/app-router-secret-promises-for-client-component-props-c53cf8fe78e7
Featured Images: pexels.com