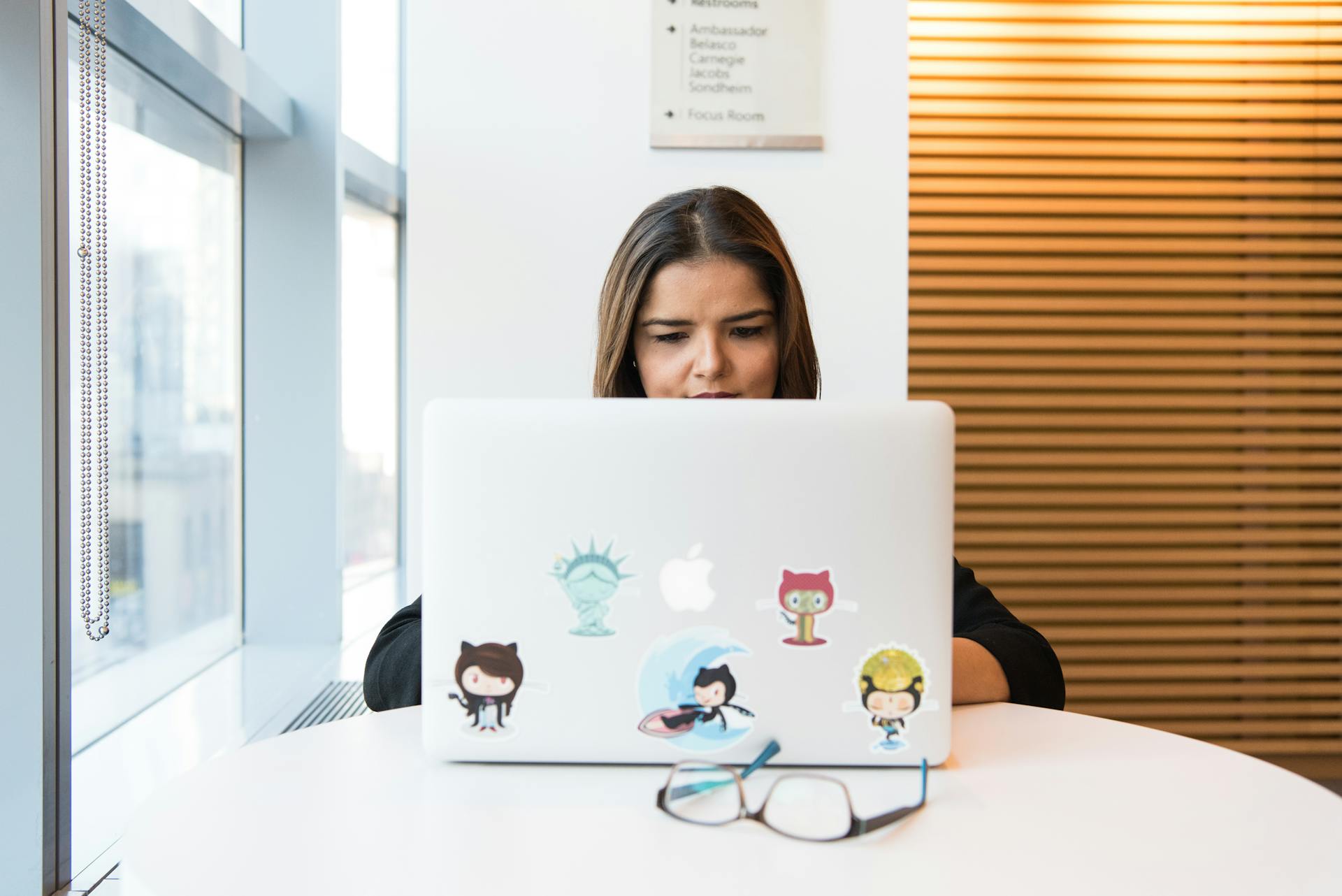
Nextjs umožňuje tvoriť komponenty pomocou súborov JSX, ktoré sú preložené na JavaScript. Týmto spôsobom môžeš tvoriť reusablu kódu.
Pri tvorení komponentov je dôležité riadiť ich stav a interakcie s užívateľom. Nextjs poskytuje rôzne spôsoby, ako to urobiť, vrátane používania štátov a zmienenia ich pomocou funkcií.
Stylizácia komponentov je ďalšou dôležitou črtou Nextjs vývoja. Môžeš použiť CSS-in-JS nástroje ako napríklad styled-components alebo emotion, alebo riešiť stylizáciu pomocou CSS súborov.
What is Next.js?
Next.js is a modern React framework for building web applications. It's a very powerful tool that offers both server-side rendering and static site generation.
Next.js is optimized for maximum speed and security, requiring minimal configuration effort. This makes it an ideal choice for developers who want to build fast and secure web applications.
To use Next.js, you'll need to have Node.js installed on your computer, specifically version 16.8.x or later. Don't worry about the operating system, as Next.js supports Windows, macOS, and Linux, whether you're using a standalone distribution or WSL.
Next.js Server-Side Rendering
Next.js Server-Side Rendering is a powerful feature that allows you to render pages on the server, making it easier to manage dynamic data and improve SEO.
Server-side rendering is particularly useful for pages that require immediate display of dynamic data, such as news websites that need to show the latest articles.
You can use the `getServerSideProps()` function in Next.js to fetch data on the server and pass it to your React components.
For example, you can use the `getServerSideProps()` function to fetch data from the Star Wars API and render it on the page.
Server-side rendering is also beneficial for SEO, as search engines can easily crawl and index fully rendered pages received from the server.
You can create a separate folder for pages in your project, and Next.js will automatically generate new pages from JavaScript files in that folder.
Here are some scenarios where server-side rendering is preferred:
- SEO-sensitive pages
- Pages that require immediate display of dynamic data
- Low-performance devices or slow networks
- High-interactivity pages
On the other hand, client-side rendering is better suited for pages that don't require frequent data updates or are not SEO-sensitive.
By choosing the right rendering approach, you can optimize your Next.js application for better performance and user experience.
Tvorba Nového Komponentu
To create a new component in Next.js, start by making a new folder in the src directory called components. This is where you'll store all your custom components. Inside the components folder, create a new file called Characters.js.
In this file, import the React library and create a new component called Characters as a function. This function will receive an object called characters as a prop and return JSX code that displays information about each character from Star Wars.
Use the map function to iterate over the characters array and create a new div element for each character, displaying their name as a heading and description as a paragraph. Don't forget to use curly brackets to access the individual values in the characters object.
Finally, export the Characters component as a default export, making it reusable throughout your application.
By following these steps, you'll have a fully functional and reusable component that can be imported into other pages, such as the CharactersPage component. This is a fundamental building block of Next.js development, and understanding how to create custom components is essential for creating dynamic and engaging web applications.
Implementacia Stranky
To implement a page in Next.js, you create a new function called `CharactersPage()`. This function generates a new page for a character.
The `CharactersPage()` function uses a component called `Characters` and passes it a parameter called `characters`, which is an object containing character data from Star Wars.
We then export the `CharactersPage()` function as the default export, making it a page in Next.js. This is done by exporting it in the file.
The `CharactersPage()` function is used to generate a page for a specific character, and it's implemented in the `pages/` directory, which is a special directory in Next.js that automatically generates pages for each file inside it.
By exporting the `CharactersPage()` function as the default export, we've created a page that displays a list of characters, thanks to the `Characters` component.
Dynamic Routing
Dynamic Routing is a powerful feature in Next.js, especially when combined with App Directory and TypeScript. It allows you to create flexible and scalable web applications.
One of the key benefits of dynamic routing is that it enables you to create pages that are generated on the fly based on dynamic parameters in the URL. For example, you can have a page for each product, with a URL like '/product/123', where '123' is the product ID.
To work with dynamic routing, you'll need to use the useRouter hook, which allows you to access parameters in the URL and dynamically render content on the page. This hook is a crucial tool for working with dynamic routing in Next.js.
You can also work with multiple parameters in the URL, which is useful for complex pages. For instance, you can have a URL like '/product/category/123', where 'category' is the product category and '123' is the product ID.
To handle unknown routes, you can set up a fallback for dynamically generated pages. This allows you to load content dynamically if a static page is not available. This approach is perfect for pages where data is not known beforehand or changes frequently.
To optimize dynamic routing for performance, consider using Incremental Static Regeneration (ISR) and caching with a CDN. These techniques can significantly speed up the delivery of static content and ensure your application is fast and scalable.
Here are some key tools and technologies to keep in mind when working with dynamic routing:
- Next.js
- Routing
- TypeScript
- App Directory
- Web Development
Vytvoření Next.js Projekt
To create a Next.js project, you can use the following commands. Using the @latest tag ensures you get the latest version, and the commands will also install React and necessary dependencies.
You'll be prompted to answer several questions during project creation, such as whether to use JavaScript or TypeScript, and the original local src directory. For a small project, JavaScript is sufficient. Tailwind is a good choice for styling, and ESLint will help with formatting.
Next.js' native routing is a great feature to use, and importing will remain unchanged. You can configure your project to use these features by running the commands and answering the prompts.
The project name is "moje-aplikace", and you can access it by visiting http://localhost:3000/. The welcoming page will look similar to the image shown in the example.
Development
Next.js development involves building server-rendered React applications with a focus on performance and scalability.
Next.js provides a built-in CSS-in-JS solution called styled-jsx, which allows for server-side rendering of CSS.
The framework also supports internationalization (i18n) out of the box, making it easy to build applications that cater to multiple languages and regions.
Next.js allows developers to use server-side rendering, static site generation, and client-side rendering to optimize performance and improve user experience.
Developers can use Next.js to create pages that are optimized for SEO, with features like automatic XML sitemap generation and support for server-side rendering of pages.
Styling and Features
Next.js supports styling with CSS as well as precompiled Scss and Sass, CSS-in-JS, and styled JSX. This gives developers a lot of flexibility when it comes to designing their applications.
It also comes with TypeScript support and smart bundling, making it easier to manage complex projects.
The open-source transpiler SWC is used to transform and compile code into JavaScript, and Webpack is used to bundle the modules afterward.
Next.js is built with server-side rendering, which reduces the burden on web browsers and provides enhanced security.
This can be done for any part of the application or the entire system, allowing for content-rich pages to be singled out for server-side rendering.
Next.js also features hot reloading, which detects changes as they are made and re-renders the appropriate pages.
This allows changes made to the application code to be immediately reflected in the web browser, though some browsers will require the page to be refreshed.
Next.js uses page-based routing for developer convenience and includes support for dynamic routing.
The software also features hot-module replacement, automatic code splitting, and page prefetching to reduce load time.
Next.js supports Incremental Static Regeneration and static site generation, which can make load times very fast.
Is Server-Side Rendering Worth It?
Server-side rendering can be a game-changer for certain situations.
In the case of Next.js, we've already explored how it works and how to use it to load data on the server-side with the getServerSideProps() function.
Server-side rendering is ideal for situations where you need to pre-render pages or generate static HTML for SEO purposes.
However, in other cases, client-side rendering might be a better choice, especially when dealing with complex or dynamic content that requires user interaction.
Featured Images: pexels.com