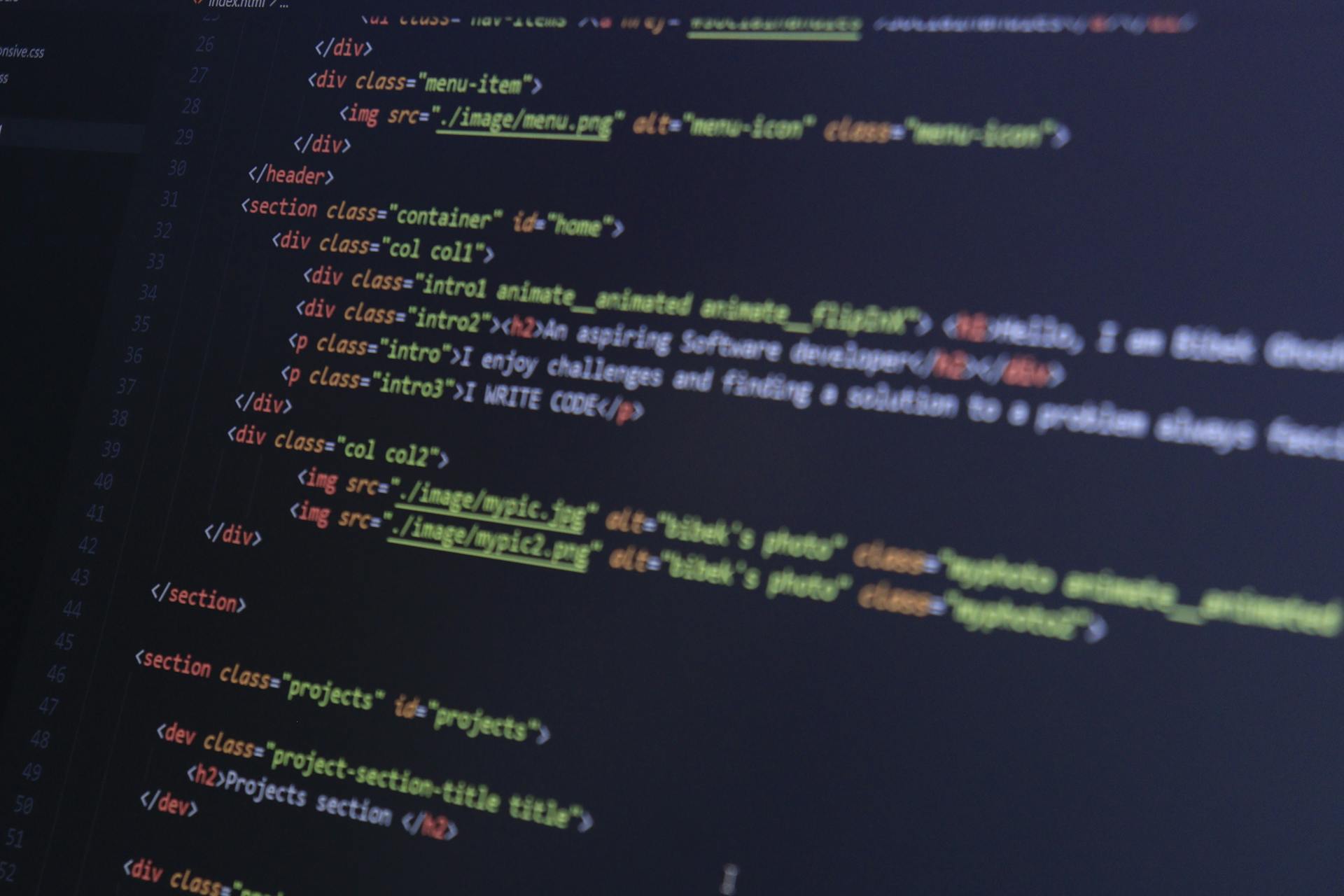
To make OpenAPI Gen Next.js effortless for Next.js applications, you can use the `openapi` package, which provides a simple API for generating client code from OpenAPI definitions.
This package is compatible with Next.js out of the box, thanks to its ability to generate API routes that can be used with Next.js's built-in API routes feature.
By using `openapi`, you can automatically generate client code for your Next.js application, saving you time and effort in the process.
With `openapi`, you can easily create a client that can make requests to your Next.js API routes, making it a great tool for building robust and scalable APIs.
A unique perspective: Debug Nextjs Client Frontend Webstorm
Setting Up
To set up your project, you can use WunderGraph's create-wundergraph-app CLI. Run the command `npx create-wundergraph-app my-project -E nextjs` to create a new project with Next.js enabled.
You'll then need to install the necessary dependencies by running `npm i && npm start`. This will start the development server, and you can view the results on localhost:3000. If everything went well, you should see the WunderGraph + Next.js starter splash page with a sample query.
Check out the `wundergraph.config.ts` file in the `.wundergraph` directory in your root for more information.
Suggestion: Next Js Project
Setting Up WunderGraph
Setting up WunderGraph involves using the create-wundergraph-app CLI to set up both the WunderGraph server and Next.js.
You can do this by running the command npx create-wundergraph-app my-project -E nextjs, which will create a new project directory.
The command will create a new directory for your project, so be sure to cd into it and run npm i && npm start to get started.
Head on over to localhost:3000 to see the WunderGraph + Next.js starter splash page, which should display the results of a sample query.
Check out the wundergraph.config.ts file in the .wundergraph directory in your root to see the configuration settings for your WunderGraph setup.
You might enjoy: New Nextjs Project Typescript
Strapi Schema Creation
You can create the OpenAPI schema in Strapi using the official plugin @strapi/plugin-documentation.
This plugin gives you two important things: It automatically generates the OpenAPI specifications for your Strapi service as a JSON file, and it provides you with a Swagger UI as a visual documentation to explore and try the REST API.
Worth a look: Strapi Nextjs
The Swagger UI shows the endpoints for the Page collection type created inside Strapi, including endpoints to create, read, update or delete pages.
The OpenAPI specifications can be found in your project under the path generated by the plugin.
Within each path, you can find a reference to its response schema, which is very important for later use.
The response schema for a path can eventually lead you to the actual schema for a Page collection type.
The Page collection type schema is of type object, with properties like path and blocks, which has items that can be different types, such as ContentHeroComponent or ContentImageTextComponent.
The generated OpenAPI specifications match what you defined in Strapi, making it easy to generate TypeScript type definitions.
Note that the @ts-ignore annotations are necessary due to how the OpenAPI documentation is generated by the Strapi documentation plugin.
For your interest: Nextjs Response Json
Creating API
Creating an API is a crucial step in building a Next.js application that can interact with external services. This is where OpenAPI comes into play.
To create an API, you need to define the endpoints, methods, and parameters that your API will support. This is done by writing an OpenAPI specification in YAML or JSON format.
Next.js provides a built-in API route feature that allows you to create API endpoints using the OpenAPI specification. This makes it easy to create a robust and well-documented API.
The OpenAPI specification should include information about the API's endpoints, such as the HTTP method, path, and response types. This information is used by Next.js to generate the API routes.
With Next.js, you can create API endpoints using the `pages/api` directory. This directory is where you can place your API route files, which will be automatically registered by Next.js.
The `pages/api` directory is a special directory in Next.js that allows you to create API routes. Any file placed in this directory will be automatically registered as an API route.
For more insights, see: Using State in Next Js
Generating the Code
Generating the code for your Next.js application is a crucial step in leveraging the power of OpenAPI. To do so, you need to install the openapi-typescript library, which takes your OpenAPI specs and outputs TypeScript type definitions.
Here's an interesting read: Azure Openapi
You'll want to install this library in your frontend application, where you'll use the generated type definitions. Once installed, add a script to your package.json that provides the path to the generated OpenAPI specs or URL endpoint and the location where to output the type definitions.
Running the script is as simple as calling `npx openapi-typescript --input ./openapi.yaml --output ./strapi.d.ts`. This command creates a strapi.d.ts file containing the TypeScript type definitions from your OpenAPI specifications file.
The generated file will contain familiar things, including the interface for all available paths, which is critical down the line. The API routes will exist to create, read, update, or delete the /pages route, and the interface components will have typed types for your Page collection type.
You can use the generated types for the Page content type you created in your Strapi backend. Even the blocks in the dynamic zone from Strapi are typed, as are the types of components that were generated.
Here's a list of the generated types:
- Page
- PageCollection
- ContentImageTextComponent
- text
- textAlign
These types are valuable when developing the graphical representation of your backend Strapi component as a React component in the frontend.
API & Swagger UI
Integrating Swagger UI with your Next.js application can be a game-changer for API documentation and visualization.
You can integrate Swagger UI with your Next.js application by defining an OpenAPI Specification when you create an API route. This will allow you to generate visual displays of your API service.
An OpenAPI Specification can be useful for generating client- and server-side code to interact with your API, or integrating testing tools.
Here are some benefits of documenting your Next.js API routes with OpenAPI Specifications:
- OAS is a standard, language-agnostic interface that humans and computers can use to understand your API service with minimal implementation context.
- An OpenAPI Specification can be useful for generating visual displays of your API service, generating client- and server-side code to interact with your API, or integrating testing tools.
To integrate your API routes with Swagger, you can define an OpenAPI Specification when you create an API route, and then use Swagger to generate visual displays of your API service.
You can also integrate Swagger UI with your Next.js build process by extending the Webpack configuration with your code. This will allow you to run your code to generate the fetchers after each development build.
For example, you can add a code similar to this to your next.config.js file to integrate Swagger UI with your Next.js build process:
In your next.config.js you’ll need a code similar to this.
Discover more: Shadcn Ui Nextjs
Sources
- https://medium.com/strapi/type-safe-fetch-with-next-js-strapi-and-openapi-4375fb924655
- https://docs.pingcap.com/tidbcloud/data-service-oas-with-nextjs/
- https://plainenglish.io/blog/automating-the-generation-of-code-from-openapi-in-your-next-js-application
- https://wundergraph.com/blog/data_fetching_with_next13
- https://blogs.perficient.com/2022/09/13/nextjs-swagger-openapi/
Featured Images: pexels.com