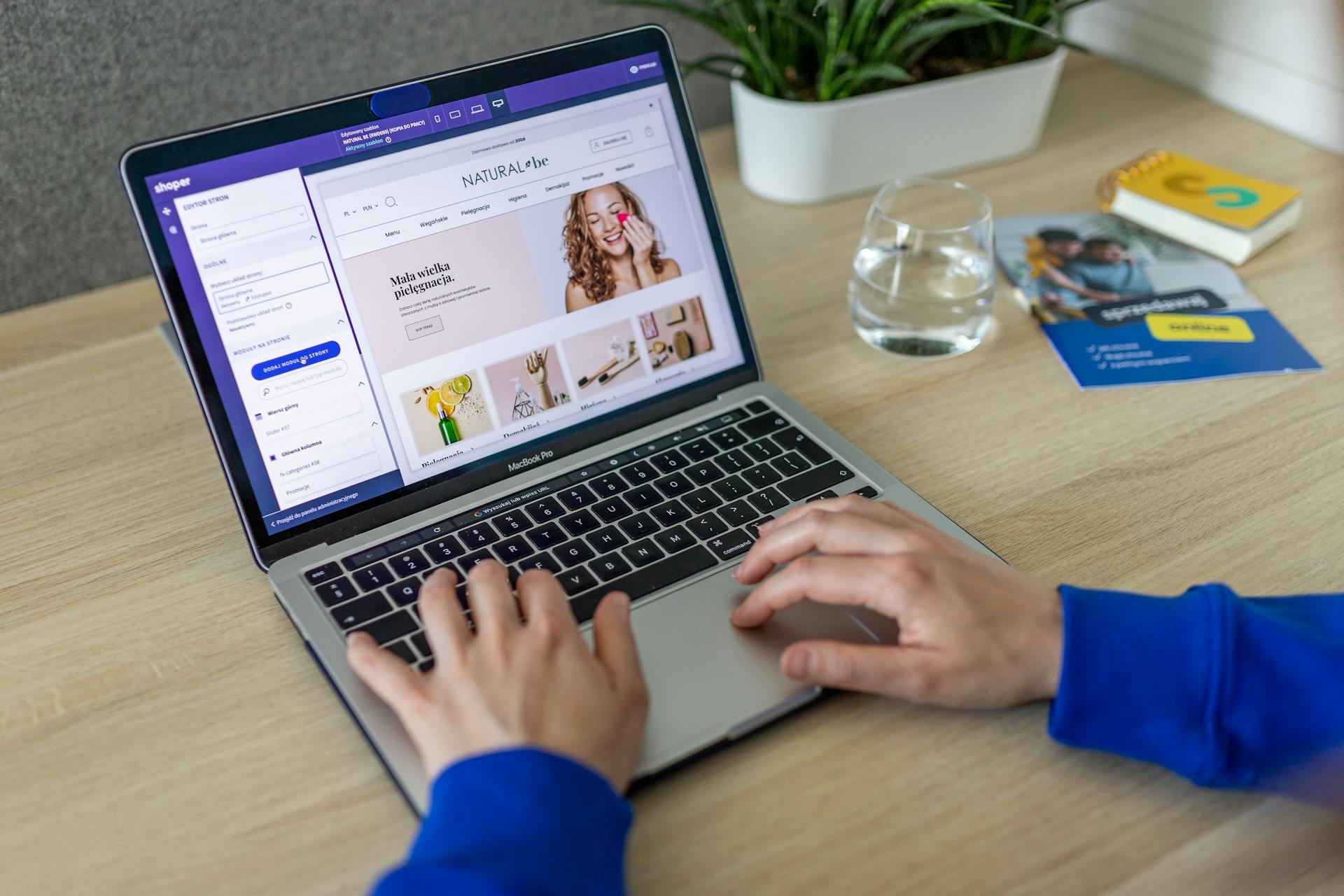
Building a Shopify app with Next.js is an exciting project that can be completed with the help of Next.js Commerce and Visual Editing.
Next.js Commerce is a powerful tool that allows you to create a seamless shopping experience for your customers. It provides pre-built pages and APIs to help you get started quickly.
With Visual Editing, you can create a user-friendly interface for merchants to customize their store's design without needing to write any code. This feature is especially useful for apps that require merchants to input specific settings or preferences.
By using Next.js Commerce and Visual Editing, you can create a Shopify app that is both functional and visually appealing, giving you a solid foundation to build upon.
Expand your knowledge: Next Js Shopify
Setting Up
To set up your Shopify App Next.js project, start by cloning the repo to your codebase and replacing USER/commerce with the name of your repo.
Add the COMMERCE_PROVIDER=shopify line to your .env.local file so it looks like the example. This will help your project connect with the Shopify API.
You might enjoy: Webflow Shopify
You'll also need to create a new file called pages/[[...plasmicPath]].tsx and paste in the code from the example, replacing PROJECTID and APITOKEN with your actual project details.
This will get your project up and running, but to add more features, you'll need to set up the Shopify Storefront API. Some things you can do with the API include adding to cart functionality, displaying products in a cart, going through the checkout process, and implementing user sign in and sign out features.
If this caught your attention, see: Nextjs 13 Api
Set Up Local Dev Env
To set up your local development environment, start by cloning the repo to your codebase, replacing "USER/commerce" with the name of your repo.
You'll also need to add a line to your .env.local file, setting the COMMERCE_PROVIDER to "shopify".
Next, create a new file called pages/[[...plasmicPath]].tsx and paste in the provided code, replacing PROJECTID and APITOKEN with the values from your Plasmic project.
This template can be installed using your preferred package manager, and pnpm is the recommended choice.
GraphiQL Setup
To set up GraphiQL, start by running it and you should see the Shopify GraphiQL App Setup interface.
You'll need to create a ProductsQuery to query for products, so let's start by getting just the product titles.
You must provide an argument to products(), typically either first or last.
Add Shopify's CDN to the list of domains in next.config.js before querying for products.
You might enjoy: Jamstack Shopify
Starter Kit
Setting up a Shopify store can be a daunting task, but having a solid starter kit can make all the difference. You can start with Next.js + Tailwind CSS + Shopify Starter, a fully functional eCommerce store starter that uses Next.js + Tailwind CSS in the front end and leverages the Shopify Storefront API to interact with your Shopify backend.
This starter kit is licensed under the MIT license, so you don't have to worry about any restrictive licensing terms. Another option is the Next.js + Shopify + Builder.io starter kit, which is SEO optimized, themable, and highly customizable.
For another approach, see: Next Js Starters
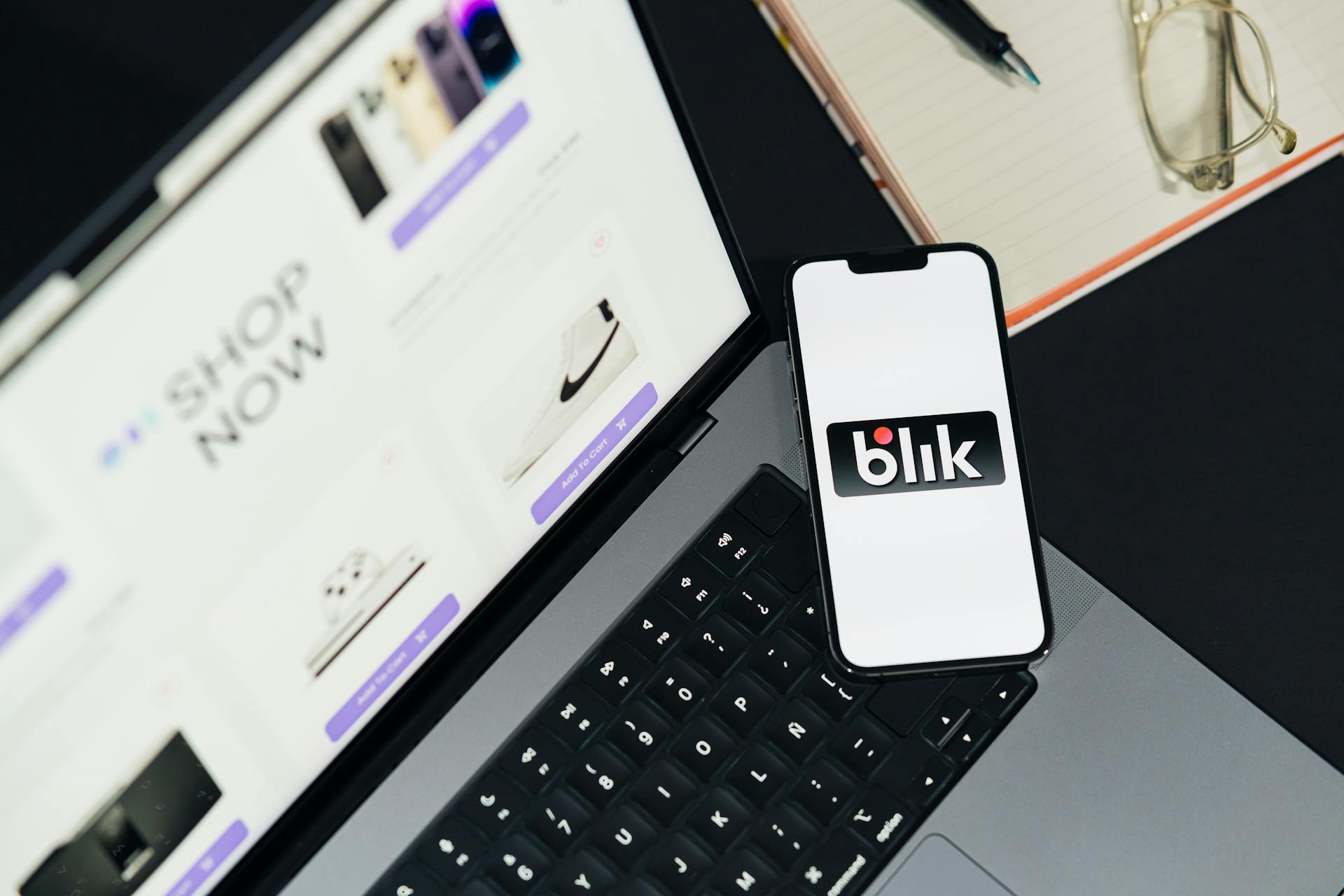
If you're looking to create a headless Shopify store, this starter kit is a great choice. It's also worth considering the Shopify App Template - Next.js App Router, which is a template for building a Shopify app using Next.js and Typescript.
To get started with this template, you'll need to clone the repo and replace the placeholder text with your own project details. This will give you a basic setup for building a Shopify app on Next.js using the app router and server components.
You can install the template using your preferred package manager, such as pnpm. This will clone the template and install the required dependencies, including some providers that are necessary for the app to run.
Here are some of the starter kits you can consider:
These starter kits can save you a lot of time and effort when setting up your Shopify store. By choosing the right one, you can get started with a solid foundation and focus on building a successful online business.
Deploying and Integrating
To deploy a Shopify app with Next.js, you can immediately deploy a new Next.js Commerce project straight to production by clicking the "Clone & Deploy" button on the Next.js Commerce homepage.
You can also manually deploy the app by forking the repo from GitHub and creating a storefront API token by following the instructions in your Vercel project > Settings > Environment Variables.
Once you've deployed your app, you can add Shopify integration by pressing the big blue plus button and selecting "Add component packages." Then, plug in the NEXT_PUBLIC_SHOPIFY_STOREFRONT_ACCESS_TOKEN and NEXT_PUBLIC_SHOPIFY_STORE_DOMAIN from your Vercel project.
Here are the steps to deploy to Vercel:
- Create your Shopify App in the Shopify Partners Dashboard
- Create your project on Vercel and add the environment variables from your Shopify App
- Setup your Shopify App to have the same /api/auth/callback and /api/auth routes as your Vercel deployment (with your hostname)
Deployment
Deployment is a crucial step in getting your Shopify Next.js app up and running. You can deploy this app to a hosting service of your choice, and Vercel is a popular option.
To deploy to Vercel, create your Shopify App in the Shopify Partners Dashboard. This will give you the necessary environment variables to set up on Vercel.
Create your project on Vercel and add the environment variables from your Shopify App. Remember to plug in the NEXT_PUBLIC_SHOPIFY_STOREFRONT_ACCESS_TOKEN and NEXT_PUBLIC_SHOPIFY_STORE_DOMAIN.
Setup your Shopify App to have the same /api/auth/callback and /api/auth routes as your Vercel deployment (with your hostname). This ensures seamless integration between your Shopify App and Vercel deployment.
Vercel should be setup to build using the default Next.js build settings. This will ensure that your app is built correctly and efficiently.
You should also be using a managed Shopify deployment. This will handle scope changes on your app, ensuring that it remains stable and secure.
Here are the basic setup steps for deploying to Vercel:
- Create your Shopify App in the Shopify Partners Dashboard
- Create your project on Vercel and add the environment variables from your Shopify App
- Setup your Shopify App to have the same /api/auth/callback and /api/auth routes as your Vercel deployment (with your hostname)
Integration Features
Integration Features are a key part of deploying and integrating your project. One of the most powerful features is Shopify integration, which allows you to automatically sync products from Shopify into Sanity.
To enable Shopify integration, you'll need to press the big blue plus button and select "Add component packages". From there, you'll need to configure the package as prompted, plugging in your NEXT_PUBLIC_SHOPIFY_STOREFRONT_ACCESS_TOKEN and NEXT_PUBLIC_SHOPIFY_STORE_DOMAIN.
Here are some of the key features you can expect from Shopify integration:
- Automatically syncs products from Shopify into Sanity
- Custom action to sync product cart thumbnails back to Shopify from Sanity
- Tracks product status (draft/published) from Shopify to help control visibility while editing
- Deleted products and variants are preserved and flagged in Sanity
- Updates the URL on variant changes while keeping a clean history stack
- Vanity shop URL masking
- Global Cart with access to all variant data for line items
- Supports Single Variant products out of the box
- Product photo galleries with variant granularity
- Dynamic /shop collection page
- Custom collection pages
- Ability to surface a variant option on product cards
In addition to Shopify integration, you can also take advantage of other integration features like Builder.io Visual CMS, which provides ultra high performance, SEO optimized, themable, and personalizable features.
Next.js Features
Next.js is a powerful framework for building fast, scalable, and secure web applications. It's a great choice for building Shopify apps.
One of the key features of Next.js is its ultra high performance. This is achieved through its ability to pre-render pages and use server-side rendering.
Next.js is also SEO optimized, which is crucial for Shopify apps to rank well in search engines.
Here are some of the key features of Next.js:
- Ultra high performance
- SEO optimized
- Themable
- Personalizable (internationalization, a/b testing, etc)
- Builder.io Visual CMS integrated
These features make Next.js an ideal choice for building Shopify apps that are fast, secure, and easy to maintain.
Storefront and UI
Storefront and UI is where the magic happens. You can build a complete Shopping Cart starter with TypeScript, Tailwind CSS, Headless UI, Next.js, React.js, and Shopify Hydrogen React, all connected to the Shopify Storefront GraphQL API.
The Shopify Storefront API is a powerful tool that allows you to interact with your Shopify backend using Next.js and Tailwind CSS. This is the foundation for a fully functional eCommerce store starter that leverages the Shopify Storefront API to connect your frontend and backend.
A unique perspective: Api Route Nextjs
Storefront
Storefront is a crucial component of any e-commerce platform, and when it comes to Shopify, there are several options to choose from. Next Shopify Storefront is a complete Shopping Cart starter built with TypeScript, Tailwind CSS, and Next.js.
You can connect Shopify with TakeShape to open up new possibilities for e-commerce on the Jamstack. This connection allows you to get data for your products and content from a single GraphQL API.
Next Shopify is an open-source starter that includes a context, a hook, and an API route handler to manage a Shopify Storefront in your Next.js app. It's easy to use and Next.js friendly, with features like storing cart id in localStorage and global app cache with react-query.
Here are some key features of Next Shopify:
- Easy to use, Next.js friendly implementation of the Shopify Storefront API.
- Store your cart id in localStorage.
- Global app cache with react-query.
- API route handler with catch-all API routes.
This starter template is licensed under the MIT license, making it a great option for developers who want to build a Shopify store with Next.js and Tailwind CSS.
Headings
Headings are crucial in organizing your content, and in the context of Storefront and UI, they play a significant role in Next.js and Shopify Embedded Apps.
The goal of using headings is to provide a clear structure and hierarchy to your content, which is especially important when building a Shopify Embedded App with Next.js.
In the example of migrating legacy code from pages router to app router, headings can help to separate and organize different sections of code, making it easier to understand and maintain.
Headings can also improve accessibility by providing a clear outline of the content for screen readers and other assistive technologies.
Using consistent heading levels and styles can enhance the user experience and make your content more readable.
Sources
- https://www.plasmic.app/blog/nextjs-commerce-tutorial
- https://github.com/ozzyonfire/shopify-next-app
- https://medium.com/@andrewshearerdev/use-next-js-13-with-typescript-to-create-a-headless-shopify-app-f6146f6db991
- https://www.ppspy.com/shopify/building-and-deploying-a-shopify-app-with-nextjs-4730
- https://medevel.com/15-shopify-starter/
Featured Images: pexels.com