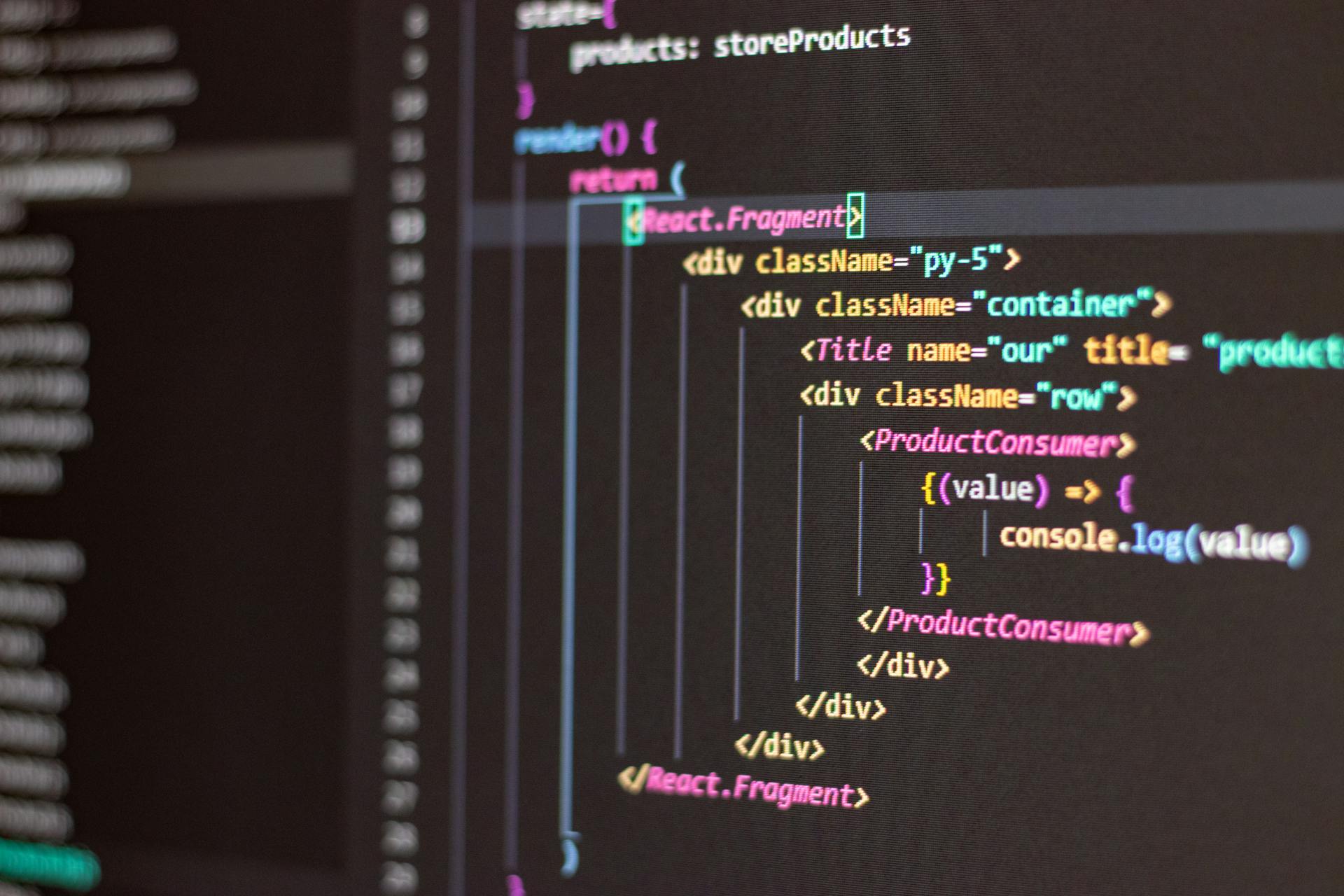
Building an e-commerce site with Next.js and Shopify integration is a great way to create a fast and scalable online store. This approach allows you to leverage the strengths of both technologies, resulting in a seamless user experience.
With Next.js, you can create a server-side rendered (SSR) application that provides instant page loading and improved SEO. By integrating Shopify, you can tap into its robust e-commerce features and payment processing capabilities.
Shopify's API provides a robust way to integrate your store with Next.js, allowing you to fetch products, customers, and orders with ease. This integration also enables you to use Shopify's payment gateways, such as PayPal and Apple Pay.
To get started, you'll need to install the Shopify API plugin for Next.js, which provides a simple and intuitive way to connect your store to your application.
Broaden your view: Webflow and Shopify
Setting Up
Setting up a Next.js Shopify integration requires some initial setup. You'll need to create a Shopify partner account if you don't already have one.
Related reading: Jamstack Shopify
To start, log in to your partner account and create a Shopify development store to test your implementation. This is where you'll be making requests to the Storefront API. You'll need to generate your API credentials to make authenticated requests.
Create products and product variants in your store, such as dummy products like meats and cheeses. This will help you test your implementation. You'll also need to create a private app on your Shopify admin dashboard to represent your client application.
Here's a step-by-step guide to setting up your Shopify development store:
- Create a Shopify partner account if you don't already have one.
- Log in to your partner account and create a Shopify development store.
- Generate your API credentials.
- Create products and product variants in your store.
- Create a private app on your Shopify admin dashboard.
Once you have your Shopify development store set up, you'll need to set up your Next.js project. You can use the full demo as your starting point or start a Next.js project from scratch using the starter project.
Suggestion: Clone Next Js Project
Project Creation
To create a Next.js project, you'll start by running the command `npx create-next-app@latest` in your terminal. This will prompt you to name your project, which you'll name "shopify-store" and select "No" for TypeScript and "Yes" for EsLint.
You'll then create a new folder called utils in the base folder of your app. Inside this folder, you'll add a new file named shopify.js.
Next, you'll create a new folder called components in the base folder. Inside this folder, you'll add the ProductCard/ProductCard.js file.
Recommended read: Shopify App Nextjs
API Integration
API Integration is a crucial step in building a seamless shopping experience for your customers. You can use the Shopify Storefront API to fetch products, manage the cart, and retrieve checkout URLs.
To start, you'll need to activate the Storefront API by enabling private app development in your Shopify admin. This will give you access to the API and allow you to create a private app.
Once you've activated the API, you'll need to retrieve a Storefront API token. This token will be used to authenticate your API requests. You can do this by clicking on the "Create private app" link and following the prompts.
To make GraphQL requests, you'll need to install the graphql-request library using npm. You can then use the library to send GraphQL requests to the Shopify Storefront API.
Here are the basic queries you'll need to make to fetch products and manage the cart:
- Fetch all products
- Fetch a single product
- Add items to the cart
- Update the cart
- Retrieve the checkout URL
You can use the following code to create a GraphQL client instance and make requests to the API:
```javascript
import { gql, GraphQLClient } from "graphql-request";
const storefrontAccessToken = process.env.STOREFRONTACCESSTOKEN;
const endpoint = process.env.SHOPURL;
const client = new GraphQLClient(endpoint, {
headers: {
Authorization: `Bearer ${storefrontAccessToken}`,
},
});
```
You can then use the client to make requests to the API using the following queries:
```javascript
const allProductsQuery = gql`
query {
products(first: 10) {
edges {
node {
id
title
price
}
}
}
}
`;
const singleProductQuery = gql`
query ($handle: String!) {
product(handle: $handle) {
id
title
price
}
}
`;
```
Remember to replace the `process.env.STOREFRONTACCESSTOKEN` and `process.env.SHOPURL` variables with your actual Storefront API token and shop URL.
By following these steps, you'll be able to integrate the Shopify Storefront API into your Next.js application and provide a seamless shopping experience for your customers.
Cart and Checkout
In the world of e-commerce, a seamless checkout experience is crucial to driving sales and customer satisfaction. To achieve this, you'll need to create a cart and checkout system that integrates with Shopify.
You'll start by adding a function named addToCart in utils/shopify.js that accepts a product ID and quantity as arguments. This function creates a new cart and adds a product to it.
Related reading: Next Js Strike Checkout
The next step is to create a function named retrieveCart that retrieves the cart, including the product IDs and total estimated cost. You'll add a query for this in utils/shopify.js.
To complete the checkout process, you'll need to create a function named retrieveCheckout that receives a cart ID as an argument. This function will fetch the checkout URL from Shopify, which you can then use to redirect the shopper to the Shopify checkout page.
Here's a summary of the key functions you'll need to create:
- addToCart: adds a product to the cart
- retrieveCart: retrieves the cart, including product IDs and total estimated cost
- retrieveCheckout: fetches the checkout URL from Shopify
With these functions in place, you'll be able to create a seamless checkout experience for your customers.
Adding to Cart
Adding to Cart is a crucial step in the shopping process, and it's essential to get it right.
In our Shopify implementation, a new function named addToCart is added in utils/shopify.js, which accepts a product ID and quantity as arguments.
This function creates a new cart and adds a product to it, setting the stage for managing the cart.
To add a product to an existing cart, we'll build on the foundation established by the addToCart function.
The next step is to create the frontend, starting with the products page, where customers will browse and select products to add to their cart.
Retrieving The Cart
You need to retrieve the cart to display its contents and calculate the total estimated cost. To do this, add a function named retrieveCart in the utils/shopify file, and use the cart ID to query for IDs of the products in the cart and the total estimated cost.
The retrieveCart function uses a query to make the request. This query retrieves the cart ID and fetches the IDs of the products in the cart and the total estimated cost.
Here's a step-by-step guide to creating the retrieveCart function:
1. Add a function named retrieveCart in the utils/shopify file.
2. Use the cart ID to query for IDs of the products in the cart and the total estimated cost.
3. Add a query to make the request.
Now that you have the retrieveCart function, you can use it to retrieve the cart and display its contents to the shopper.
Page Creation
To create pages in a Next.js Shopify app, you need to start with the products page. This involves exporting the getServerSideProps function in pages/index.js and calling the getProducts function from utils/shopify.js.
You'll also need to create the ProductCard component, which will be rendered for each product. To display product details, create a products/[handle].js file in the pages folder, which will fetch the product details by the ID passed in the URL query.
To create a product listing page, you'll need to make sure you can request data from the Storefront API, which includes features like a cart experience, SEO optimization, and collection pages.
For another approach, see: Next Js Pages
Add Domain to Config
To add your Shopify domain to the config, you need to follow a few steps. First, you'll need to access your storefrontAccessToken and domain by logging into your Shopify store admin dashboard, clicking on the Apps link, and then selecting Manage private apps. From there, you can copy the storefront access token and your domain from your Shopify admin URL.
Here are the exact steps to access your storefrontAccessToken and domain:
- Log in to your Shopify store admin dashboard.
- Click on the Apps link on the sidebar.
- Click on Manage private apps at the bottom of the page.
- Select your app, scroll to the bottom of the page and copy the storefront access token.
- Copy your domain from your Shopify admin URL.
Next, you'll need to add your Shopify domain to your next.config.js file to gain access to the image optimization benefits of using the Next.js Image component. To do this, simply add the following code to your next.config.js file:
Add the following to your next.config.js file:
This will allow your app to render images from Shopify.
Broaden your view: Next Config Js
Create Website Pages
To create website pages, you'll need to start by exporting the getServerSideProps function in pages/index.js and calling the getProducts function from utils/shopify.js. This will allow you to fetch data from the Storefront API.
You'll also need to create a products/[handle].js file in the pages folder, which will fetch the product details by the ID passed in the URL query. This page uses a ProductDetails component, so create components/ProductDetails/ProductDetails.js file and add the necessary code.
To create a product listing page, you'll need to request data from the Storefront API, which requires A cart experience, SEO optimization, collection pages and product filtering, and integration with a headless CMS. You can create a dynamic page type by putting the file in a folder in pages/ called product/.
You'll also need to create a components/ folder in the root directory and add files like Header.js, Layout.js, and ProductCard.js to it. Each file will be a component that you'll later import into pages/.
Check this out: Next Js Fetch Cache
Here's a list of the three types of "page" components you'll need in your site:
- index.js - The homepage!
- cart.js - The cart page!
- [product].js - The template page that defines each product!
To statically generate critical pages, you'll need to define the path to the first product's data in your getStaticPaths() function and provide paths as well as true or blocking as the fallback value. This will allow Next.js to generate the path for that product, serve the page to the user, and cache it automatically on Netlify's CDN.
Components and Functions
To get started with building your Next.js Shopify app, you'll want to create a components folder in the root directory.
In this folder, you'll add files for individual components, such as Header.js, Layout.js, and ProductCard.js. Each component will serve a specific purpose, like displaying your app's header or wrapping your entire app with a layout.
The ProductCard component is particularly useful, as it will receive product data as a prop and display it to your shoppers.
Take a look at this: Next Js Component
State Management Setup
State Management Setup is crucial for any project, and it's surprisingly easy to set up. Create a context folder at the root of your project.
To get started, create a shopContext.js file inside the context folder. This file will hold the state management code for your project.
In this file, you'll create a state to store all your initial values. This is where you'll define the starting point for your application's state.
The createCheckout function is a key part of this setup. It creates an empty checkout instance that will be updated later when you call addItemsToCheckout. This function is called whenever the page loads, so it's essential to get it right.
For more insights, see: What Is .next Folder in Next Js
Create Shared Components
To create shared components in a Next.js project, start by creating an src folder at the root of your project. Inside this folder, create a components folder where you'll add various files for your components.
In the components folder, you'll need to create four files: Header.js, Footer.js, Hero.js, and Cart.js. These files will serve as the foundation for your shared components.
The Header.js file is a crucial component that contains a simple sidebar showing the list of products in the cart. It loops over the data received and displays the product details, including quantity.
A conditional statement in the Header.js file depends on the value of the isCartOpen function to open and close the cart. This is a clever way to make the cart interactive.
To make context data available throughout the project, wrap your entire application with the ShopProvider component. This ensures that all components have access to the necessary data.
By following these steps, you'll have a solid foundation for creating shared components in your Next.js project.
A fresh viewpoint: Next Js Fetch Data save in Context and Next Route
Add Components
To add components to your project, you'll need to create a components folder in the root directory. This is where you'll store all your reusable UI components.
First, add a components folder to your project. Inside this folder, you'll create individual files for each component, such as Header.js, Layout.js, and ProductCard.js.
You can start by adding Header.js to your new folder. This component will contain the code for your website's header, including the navigation menu.
Next, add a Layout.js file that will wrap your entire app and add your Header and Footer component to every page. This will give your website a consistent layout throughout.
As you add more components, you can import them into your pages using the import statement. For example, you can import the ProductCard component into your product listing page.
Here are some common components you'll need for a basic e-commerce website:
- Header.js: contains the code for your website's header, including the navigation menu
- Layout.js: wraps your entire app and adds your Header and Footer component to every page
- ProductCard.js: receives product data as a prop and displays it to your shoppers
Unified Headless Functionality
To achieve unified headless functionality, our team started by creating serverless functions that could be shared across projects to query the Shopify API. These functions had essential functionality such as getting a product, getting a list of products, adding a product to cart, getting a cart, and removing a product from the cart.
We also shared the same styles across projects, allowing us to show a near replica of the same site to everyone, just built in a different way. This approach helped us maintain consistency and efficiency in our development process.
Here's a list of the essential functions we created for the Shopify API:
- Get a product
- Get a list of products
- Add product to cart (and make a new cart object if one doesn’t exist)
- Get a cart (that holds products)
- Removes product from the cart
Make sure to deploy your site first with these functions before calling them, so you don't run into any concurrency issues later in querying them.
Sources
- https://bejamas.io/hub/guides/how-to-build-an-e-commerce-storefront-with-next-js-and-shopify
- https://www.telerik.com/blogs/build-fast-ecommerce-site-nextjs-shopify
- https://www.commerceworm.com/articles/nextjs-shopify-storefront-tutorial
- https://www.learnwithjason.dev/ecommerce-nextjs-shopify/
- https://www.netlify.com/blog/2021/09/13/build-your-own-headless-commerce-site-with-next.js-and-shopify/
Featured Images: pexels.com