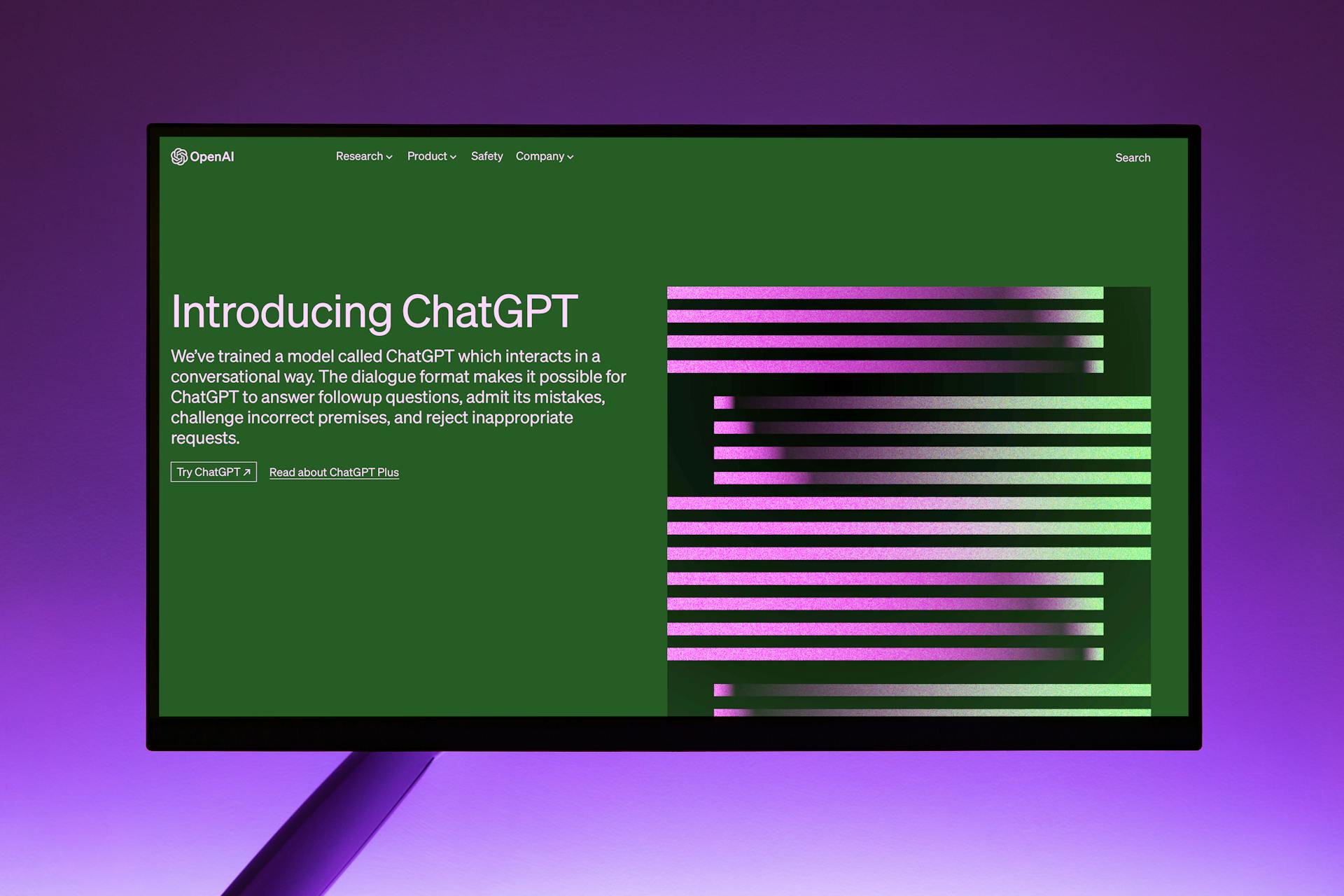
Configuring breadcrumbs in Next.js is a straightforward process that involves creating a Breadcrumbs component and using it to display the navigation trail.
To start, you'll need to create a Breadcrumbs component that will render the breadcrumb items. This component should be placed in a separate file, such as `components/Breadcrumbs.js`.
In this component, you'll use the `useRouter` hook from Next.js to access the current route and its ancestors. This will allow you to generate the breadcrumb items dynamically.
The `useRouter` hook returns an object with information about the current route, including its path and its ancestors. You can use this information to render the breadcrumb items.
If this caught your attention, see: Nextjs Userouter
Configuring Breadcrumbs
In Next.js, you can configure breadcrumbs using the `useBreadcrumbs` hook from the `@nextui/breadcrumbs` package. This hook allows you to define a breadcrumb structure for your application.
To create a breadcrumb, you need to define an array of breadcrumb items, where each item is an object with a `label` and a `href` property. The `label` property is the text that will be displayed in the breadcrumb, and the `href` property is the URL that will be linked to.
You can also use the `active` property to mark a breadcrumb item as active, which will be highlighted in the breadcrumb navigation. For example, if you have a breadcrumb item with `label` set to "Dashboard" and `href` set to "/dashboard", and the current URL is "/dashboard", the breadcrumb item will be marked as active.
To configure the breadcrumb, you can use the `useBreadcrumbs` hook and pass it an array of breadcrumb items. For example, you can use the following code to define a breadcrumb for a dashboard page: `[ { label: 'Dashboard', href: '/dashboard' }, { label: 'Users', href: '/users' } ]`.
You might enjoy: How to Use Reducer Api in Next Js 14
Defining the Component
To define the component, we start with the basic structure of the NextBreadcrumbs React component, importing the correct dependencies and rendering an empty Breadcrumbs MUI component.
The NextBreadcrumbs component uses the MUI Breadcrumbs component as a baseline, creating the basic structure of the component.
We can then add in the next/router hooks, which will allow us to build the breadcrumbs from the current route.
Broaden your view: Nextjs Pathname Server Component
The Crumb component is a pretty dumb component for now, except that it will render normal text instead of a link for the last breadcrumb, making it perfect for rendering the last item of the breadcrumbs.
We can render the Crumb component as normal text instead of a link for the last breadcrumb, which is useful when the user is already on the last breadcrumb's page.
The Crumb component will render normal text instead of a link for the last breadcrumb, and will render links to be clicked for all the other crumbs.
Recommended read: Nextjs Components Library
Properties and Interface
The properties and interface of Next.js breadcrumbs are quite straightforward. They're defined in a table that shows the prop name, description, data type, example, and default value.
One of the most important properties is `useDefaultStyle`, which determines whether to use the default styles for the breadcrumbs. If set to `true`, make sure to import the CSS in `_app.js`.
The `rootLabel` property sets the title for the first breadcrumb pointing to the root directory, defaulting to `'HOME'`. If you want to omit the root label, set `omitRootLabel` to `true`.
When it comes to customizing the labels, you can use the `labelsToUppercase` property to display them as uppercase. Alternatively, you can use the `replaceCharacterList` property to replace specific characters in the label.
The `transformLabel` property allows you to customize the label strings by providing a transformation function that receives the label string and returns a string or React component.
The `omitIndexList` property is used to omit specific indexes in the breadcrumb path, such as the index `1` in the path `'/home/category/1'`.
Here's a summary of the properties:
Example and Usage
To use NextJS breadcrumbs, you can utilize the `useBreadCrumbs` hook from any page. This hook works with asynchronous data fetching, making it straightforward to add a loading state while the data is being fetched.
The component needs to be used within Next.js and won't work in plain React. It will always display a dynamic breadcrumb navigation, based on the current path of the Next router.
You can implement Breadcrumbs on Next JS with Antd Design as below, where you can see how to align its style with antd. This involves creating a simple component that uses Next JS router and some chunk of JS.
Here's an example of how to access the breadcrumb path: `path[0] = " " , path[1] = " Array_value_one", path[2] = "Array value two"`. This can be useful for understanding how to structure your breadcrumb navigation.
The `useCallback` hook is used to create only one reference to each helper function, preventing unnecessary re-renders of the breadcrumbs when the page layout component re-renders.
You can use the `rootLabel` option to set a custom label for the root breadcrumb, or set it to `null` if you don't want to display a label at all.
Note that the `rootLabel` option is optional and can be set to a string or `null`. This is useful for customizing the appearance of your breadcrumb navigation.
Advanced Topics
Next.js breadcrumbs can be customized to meet specific needs.
Using the `getStaticPaths` function, you can generate dynamic paths for your pages. This is particularly useful for e-commerce sites where product categories and subcategories are numerous.
Customizing the breadcrumb component is also possible. For instance, you can use the `useParams` hook to access the current URL parameters.
Custom Styling (Css)
Custom styling is a powerful tool in Next.js components, allowing you to tailor the look and feel of your breadcrumbs to suit your needs.
You can style each HTML element of the breadcrumb component separately, either by using inline styles or by assigning your own classes.
Assigning custom classes is a great way to reuse styles across multiple components, and it's a more organized approach than using inline styles.
An inline style object for the breadcrumb list is available for those who prefer a more direct approach to styling.
By using custom styling, you can create a unique and visually appealing breadcrumb component that matches your application's design.
A unique perspective: Next Js Components Folder
Memoizing Generated
Memoizing Generated Breadcrumbs can be a game-changer for performance.
The breadcrumb list is recreated every time the component re-renders, which can be inefficient.
We can memoize the crumb list for a given route to save some performance.
This is achieved by wrapping the generateBreadcrumbs function call in the useMemo React hook.
This simple improvement can make a noticeable difference in your application's speed and responsiveness.
A unique perspective: Nextjs Performance
Optimizing Breadcrumb Text
Optimizing breadcrumb text can greatly enhance the user experience, making it easier for users to navigate complex routes.
Improving the display of breadcrumb text can be a good first step in optimizing it.
The current solution can be cleaned up by including a function to generate a more user-friendly name for each crumb.
This function can be provided to the NextBreadcrumbs component, allowing for more flexibility in customizing the breadcrumb text.
A parent component can then use this function to title-ize subpaths or replace them with a new string.
This implementation can result in more appealing breadcrumbs, as seen in the code example provided.
For another approach, see: Next Js Function Handlers to Filter Data
Seven Answers
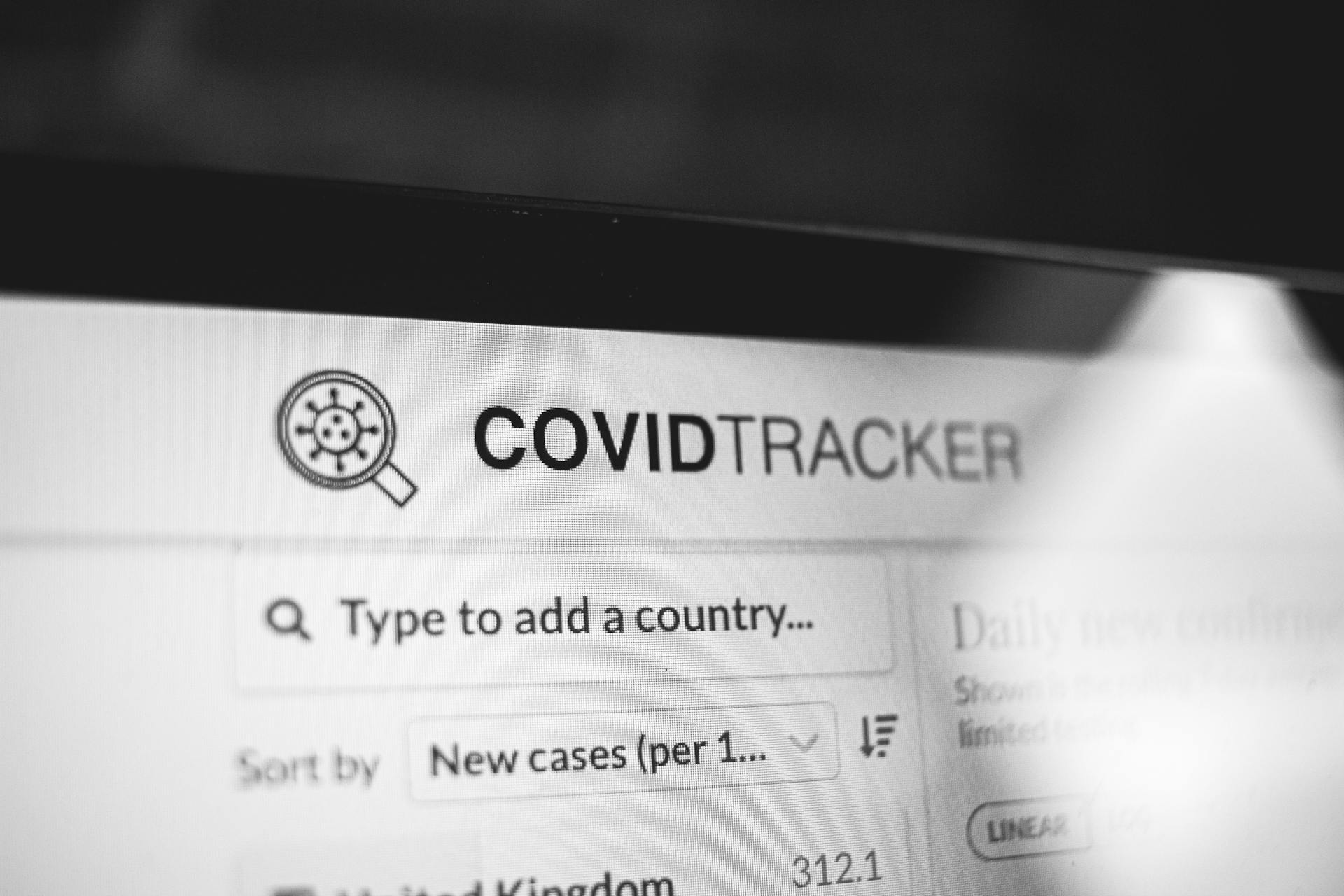
For Next.js routing, there's a simple and effective solution called nextjs-breadcrumbs. You can use it as a reference or write your own component.
If you prefer to write your own code, you can use the router.asPath to get the link to the crumb and the router.route to get the label if it exists in the Route2LabelMap.
The code for custom breadcrumbs in Next.js uses the router.asPath to get the link to the crumb.
Using nextjs-breadcrumbs is a great approach to breadcrumbs in Next.js, it's simple and effective.
The example in the code is in the pages/example.jsx file.
You might enjoy: Useeffect Nextjs
Nested Dynamic Routes
Nested dynamic routes are a powerful feature in Next.js that allow for deeply nested routes with dynamic segments. This feature works in conjunction with Parallel Routes to enable complex routing structures.
You can add nested dynamic routes by creating a route like app/[first]/[middle]/[last]/page.tsx. This route can be visited at a URL like http://localhost:3000/Joseph/Francis/Tribbiani.
The breadcrumbs for this page will be logged from the server, showing the rendering in @breadcrumbs [ 'Joseph', 'Francis', 'Tribbiani' ]. This makes it easy to build breadcrumbs for the page.
This feature is particularly useful for building complex routing structures that require multiple levels of nesting. With nested dynamic routes, you can create routes that are both dynamic and deeply nested.
Additional reading: Page Transition Framer Motion Nextjs
Sources
- https://jeremykreutzbender.com/blog/app-router-dynamic-breadcrumbs
- https://www.gcasc.io/blog/next-dynamic-breadcrumbs
- https://github.com/NiklasMencke/nextjs-breadcrumbs
- https://stackoverflow.com/questions/64541235/breadcrumbs-and-nextjs
- https://hackernoon.com/implement-a-dynamic-breadcrumb-in-reactnextjs
Featured Images: pexels.com