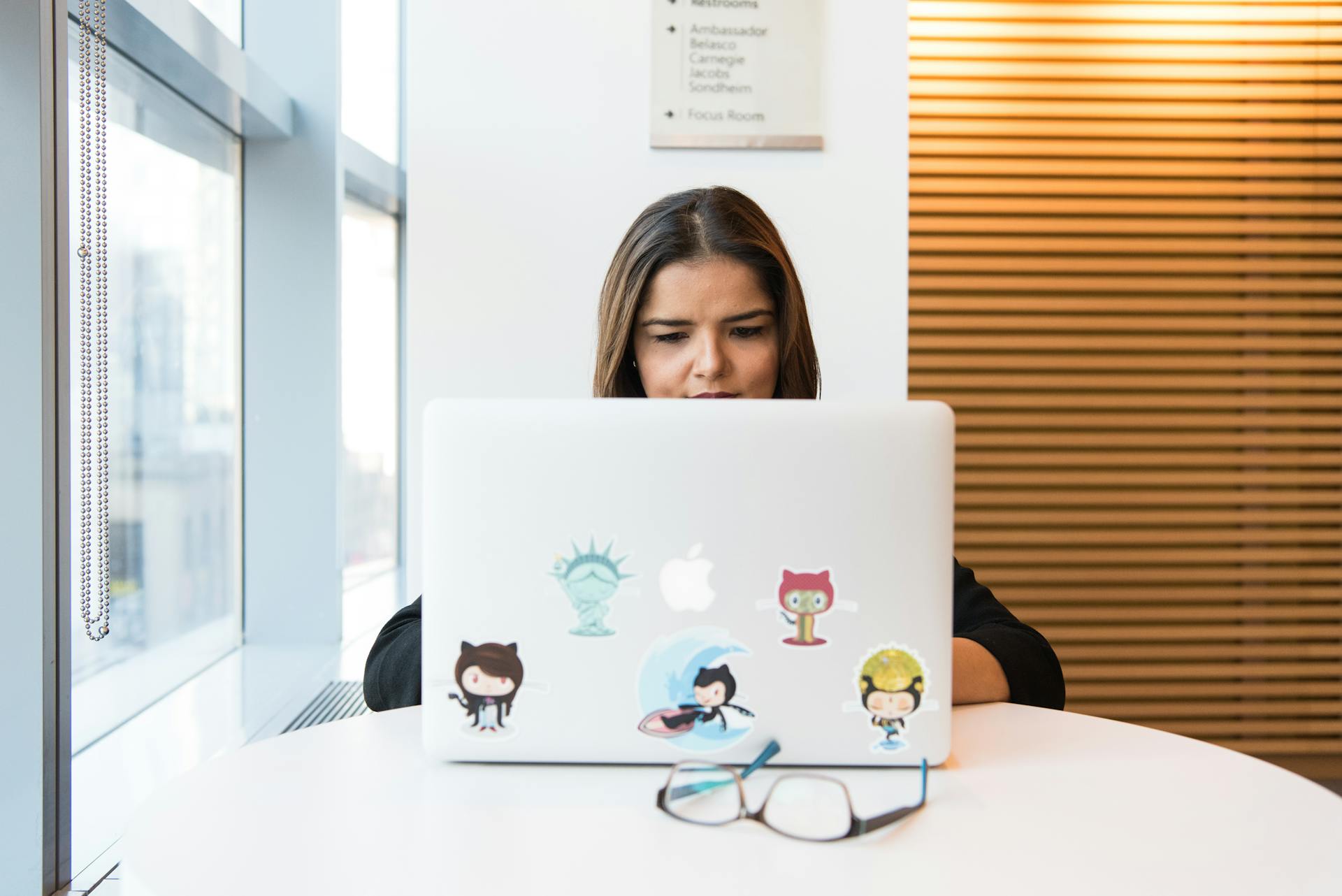
Nextjs is an excellent choice for building server-rendered, statically generated, and performance-driven web applications. You can use Nextjs to create a fullstack application with MongoDB as the database.
To get started with Nextjs and MongoDB, you'll need to install the necessary packages, including @next/heads and mongoose. This will allow you to connect to MongoDB and interact with your data.
Nextjs has a built-in API route feature that makes it easy to create RESTful APIs. This feature can be used to interact with your MongoDB database and return data to your Nextjs application.
Check this out: How to Start a Nextjs Project
Connecting to MongoDB
Connecting to MongoDB is a crucial step in building a Next.js app that interacts with MongoDB. You can connect to MongoDB using the MongoDB Node.js driver, which is a popular choice among developers.
To connect to MongoDB, you'll need to import the `MongoClient` class from the `mongodb` package. This can be done by running the command `import { MongoClient } from 'mongodb';` in your code.
Take a look at this: Mongodb on Azure
Once you've imported the `MongoClient` class, you can create a new instance of it by passing your MongoDB connection string to the constructor. The connection string should be in the format `mongodb://localhost:27017/mydb`, where `localhost` is the hostname, `27017` is the port number, and `mydb` is the name of your database.
Here's an example of how to create a new instance of the `MongoClient` class:
```html
const client = new MongoClient('mongodb://localhost:27017/mydb', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
```
You can then use the `client` instance to connect to your MongoDB database and perform various operations, such as creating, reading, updating, and deleting data.
Here's a list of the basic CRUD operations you can perform using the `MongoClient` class:
Note that this is not an exhaustive list, and there are many other methods available in the `MongoClient` class that you can use to interact with your MongoDB database.
By following these steps and using the `MongoClient` class, you can establish a connection to your MongoDB database and start building your Next.js app. Remember to handle errors and exceptions properly to ensure that your app remains stable and secure.
Suggestion: Documentdb Mongodb
Setting Up Next.js
To set up Next.js, you'll need to install it using npm or yarn by running the command `npx create-next-app my-app`. This command will create a new Next.js project in a directory named `my-app`.
The project structure will include a `pages` directory where you'll create your pages, a `public` directory for static assets, and a `styles` directory for global CSS styles.
Next.js comes with a built-in development server that you can run using `npm run dev` or `yarn dev`, which will start the server and make your app available at `http://localhost:3000`.
Additional reading: Nextjs Dev Rel
Package.json
The package.json file is a crucial part of your Next.js project, containing project configuration information and scripts for running and building your app.
This file also includes dependencies that get installed when you run npm install or npm i, making it easy to set up your project with all the necessary tools.
For more info on the Next.js CLI commands used in the package.json scripts, check out the official Next.js documentation at https://nextjs.org/docs/app/api-reference/next-cli.
Curious to learn more? Check out: Nextjs Server Actions File Upload
Creating the Project
To create a new Next.js project, you'll need to run the command `npx create-next-app@latest mongodb-auth`. This will start the process of setting up your project.
The command will prompt you to confirm if you want to proceed, and you'll need to type `y` to continue.
Next, you'll be asked a series of questions to customize your project. You'll be asked if you want to use TypeScript, ESLint, Tailwind CSS, and App Router. You can choose to use these features or opt out of them.
Here's a summary of the options:
Once you've answered these questions, the command will create your new Next.js project, which you can then open with a code editor like Visual Studio Code.
On a similar theme: Nextjs Code Block
Data Management
You can integrate MongoDB data into Next.js pages using the getServerSideProps() method, which enables server-side rendering and fetches data from MongoDB on the server-side.
This approach is ideal for implementing MongoDB queries, as the code within getServerSideProps() never reaches the client-side.
A unique perspective: Getserversideprops Nextjs
To connect to MongoDB, you can use the MongoDB data context, which provides a single point of access to the database and exports an object containing all database model objects.
The MongoDB data context uses Mongoose to connect to the database, and the database connection string (MONGODB_URI) is defined in the .env file.
To save and update data in MongoDB, you can use the updateOne() method in combination with the $set operator, as shown in the example code snippet.
Here's a step-by-step guide to saving and updating data in MongoDB:
The MongoDB data context also provides an easy way to read/write to any part of the database from a single point, making it a convenient tool for managing data in your Next.js MongoDB application.
Intriguing read: Is Nextjs and Mongodb Fullstack
User Management
User management is a crucial aspect of any Next.js application, and MongoDB provides a robust solution for storing and retrieving user data.
The users repo encapsulates all access to user data stored in MongoDB, exposing methods for authentication and standard CRUD operations.
Related reading: Nextjs App Route Get Ssr Data
All users are fetched in a useEffect() hook with the user service, and accessed via the userService.users property.
The users list page displays a list of all users in the Next.js tutorial app and contains buttons for adding, editing, and deleting users.
The delete button calls the userService.deleteUser() method, which sets the user isDeleting property so the UI displays a spinner on the delete button while the user is deleted from the API.
To handle user deletion, the userService.deleteUser() method is used, which automatically logs out the current user if they delete their own record.
The users [id] API route handler supports HTTP GET, PUT, and DELETE requests by passing an object with those method names to the apiHandler() function.
The request schema for the update() function in the users [id] API route is defined with the joi data validation library and assigned to the update.schema property.
The users API route supports HTTP GET and POST requests by passing an object with those method names to the apiHandler() function.
Discover more: How to Update Nextjs
The getAll() function in the users API route returns all users from MongoDB by calling usersRepo.getAll().
The create() function in the users API route creates a new user in MongoDB by calling usersRepo.create().
The request schema for the create() function in the users API route is defined with the joi data validation library and assigned to the create.schema property.
To authenticate users, the usersRepo.authenticate() function is used in the Next.js API login route.
The login route handler sets a JWT auth token in an HTTP only 'authorization' cookie on successful authentication.
The client-side user service sends a request to the login route to logout the user and then redirects to the login page.
The register handler receives HTTP POST requests containing user details, which are registered in the Next.js tutorial app by the usersRepo.create() method.
For your interest: Next Js Cookies
Querying
Querying MongoDB with Next.js is a crucial step in building a robust and scalable application. You can achieve this by creating a new file inside the lib directory and giving it the name mongodb.js.
To establish a Next.js MongoDB connection, add the following code to the file. This will enable you to make API calls to your MongoDB database. The code should be placed in a file named mongodb.js inside the lib directory.
Create a new file inside the pages/api directory and give it the name posts.js. This is where you will define the POST and GET methods to insert and fetch posts. The Next.js MongoDB connection is made possible by the code in the mongodb.js file.
The getServerSideProps() method in Next.js allows you to fetch data from your MongoDB database on the server-side. This means that every time a page is loaded, the getServerSideProps() method runs on the server-side, fetches the necessary data, and passes it to the React component as props. This approach is ideal for implementing MongoDB queries.
You can use the getServerSideProps() method to fetch data from your MongoDB database by creating a new file called movies.tsx within the pages directory. Inside this file, you can add the necessary code to fetch the data and pass it to the React component as props.
The MongoDB data context is used to connect to MongoDB using Mongoose and exports an object containing all of the database model objects in the application. This provides an easy way to read/write to any part of the database from a single point. The database connection string (MONGODB_URI) environment variable is defined in the .env file.
Expand your knowledge: Next Js Db
Creating Endpoints
Creating Endpoints is a crucial step in building a Next.js application with MongoDB integration. You can create API endpoints within your Next.js application to interact with MongoDB by establishing a dedicated directory called api inside your pages directory.
To create a new API endpoint, you need to create a new file within the api directory. For example, you can create a file named movies.tsx to retrieve and return a list of 20 movies from your MongoDB database.
You can also create dynamic API routes by using the [id] parameter. For instance, you can create a route handler for the /api/users/* route that handles HTTP requests with any value as the [id] parameter. The user id parameter is attached by Next.js to the params object which is passed to the route handler.
Here's a brief overview of the types of API routes you can create:
These are just a few examples of the types of API routes you can create. By following these steps and examples, you can create your own API endpoints to interact with your MongoDB database.
Login Route
The login route is a crucial endpoint in your Next.js API, and it's handled by a route handler that receives HTTP POST requests sent to /api/account/login. This route is designed to support authentication by validating the username and password sent with the request.
The route handler uses the joi data validation library to define a request schema, which is assigned to the login.schema property. This schema is then validated by the validate middleware, ensuring that the incoming request meets the expected format.
On successful authentication, the route handler sets a JWT auth token in an HTTP only 'authorization' cookie, which is a secure way to store user authentication information. The client-side user service can then use this token to authenticate future requests.
To logout, the client-side user service sends a request to this API route and is then redirected to the login page, effectively ending the user's session. This logout functionality is an essential part of any authentication system, allowing users to securely end their sessions.
For another approach, see: Next Js Client Side Rendering
Creating Endpoint
To create an endpoint in Next.js, you need to establish a dedicated directory called `api` inside your `pages` directory. Every file you create within this `api` directory will be treated as an individual API endpoint.
You can create a new file named `movies.tsx` within the `api` directory to create an endpoint that retrieves and returns a list of 20 movies from your MongoDB database.
To create a server action for registering users, you need to use the `server` keyword and import the necessary libraries, such as `connectDB` and `User`.
Here are the key steps to follow:
- Create a new file in the `api` directory
- Import the necessary libraries, such as `connectDB` and `User`
- Use the `server` keyword to define the server action
- Define the endpoint handler function, which will handle the request and response
Here is an example of a server action for registering users:
```markdown
export const register = async (values: any) => {
const { email, password, name } = values;
try {
await connectDB();
const userFound = await User.findOne({ email });
if (userFound) {
return { error: 'Email already exists!' };
}
const hashedPassword = await bcrypt.hash(password, 10);
const user = newUser({
name,
email,
password: hashedPassword,
});
const savedUser = await user.save();
} catch (e) {
console.log(e);
}
}
```
You can also create API routes for handling fetching, updating, and deleting a contact by creating a new file in the `phonebooks` folder and defining the API route handlers.
Here's an example of how you can define API routes for handling fetching, updating, and deleting a contact:
```markdown
export default async function handler(req, res) {
const { method } = req;
switch (method) {
case 'GET':
return getPhonebooks(req, res);
case 'POST':
return createPhonebook(req, res);
case 'PATCH':
return updatePhonebook(req, res);
case 'DELETE':
return deletePhonebook(req, res);
Related reading: Nextjs Script Async
}
}
```
This is a basic example of how you can create an endpoint in Next.js. By following these steps, you can create a new endpoint that interacts with your MongoDB database.
Here is a summary of the key steps:
By following these steps, you can create a new endpoint in Next.js that interacts with your MongoDB database.
Users Id Route
The Users Id Route is a dynamic API route handler that handles HTTP requests with any value as the id parameter. This parameter is attached to the params object passed to the route handler.
The route handler supports HTTP GET, PUT, and DELETE requests. These methods are mapped to the functions getById(), update(), and _delete() respectively.
The update() function has a request schema defined with the joi data validation library. This schema is assigned to the update.schema property and is validated by the validate middleware called by the api handler.
This route handler is a key part of the Next.js tutorial app, allowing for dynamic user management.
If this caught your attention, see: Next Js Dynamic
Users Route
The users route is a crucial part of any application that deals with user data. It's used to create, read, update, and delete (CRUD) user information.
The Next.js users route handler supports HTTP GET, PUT, and DELETE requests, which are mapped to the functions getById(), update(), and _delete() using the apiHandler() function.
To create a new user, you can use the POST request method, which is supported by the users route handler. The request schema for the create() function is defined with the joi data validation library and assigned to the create.schema property.
Here are the supported methods for the users route handler:
The users route handler also uses the validate middleware to handle schema validation for the update() function. This ensures that the request data conforms to the expected schema before it's processed.
To register a new user, you can use the server action, which is imported from the @/lib/mongodb module. The register function takes in user values and uses the bcrypt library to hash the password.
The users repo encapsulates all access to user data stored in MongoDB and exposes methods for authentication and standard CRUD operations. The repo is used on the server-side by the Next.js users and account API route handlers.
The users list page displays a list of all users and contains buttons for adding, editing, and deleting users. The delete button calls the userService.deleteUser() method, which sets the user isDeleting property to display a spinner on the delete button while the user is deleted from the API.
Additional reading: Turbo Repo Nextjs
Sources
- https://hevodata.com/learn/next-js-mongodb-connection/
- https://jasonwatmore.com/next-js-13-app-router-mongodb-user-rego-and-login-tutorial-with-example
- https://www.mongodb.com/developer/languages/typescript/nextauthjs-authentication-mongodb/
- https://pieces.app/blog/building-a-fullstack-application-with-next-js-and-mongodb
- https://www.mongodb.com/developer/languages/javascript/nextjs-building-modern-applications/
Featured Images: pexels.com