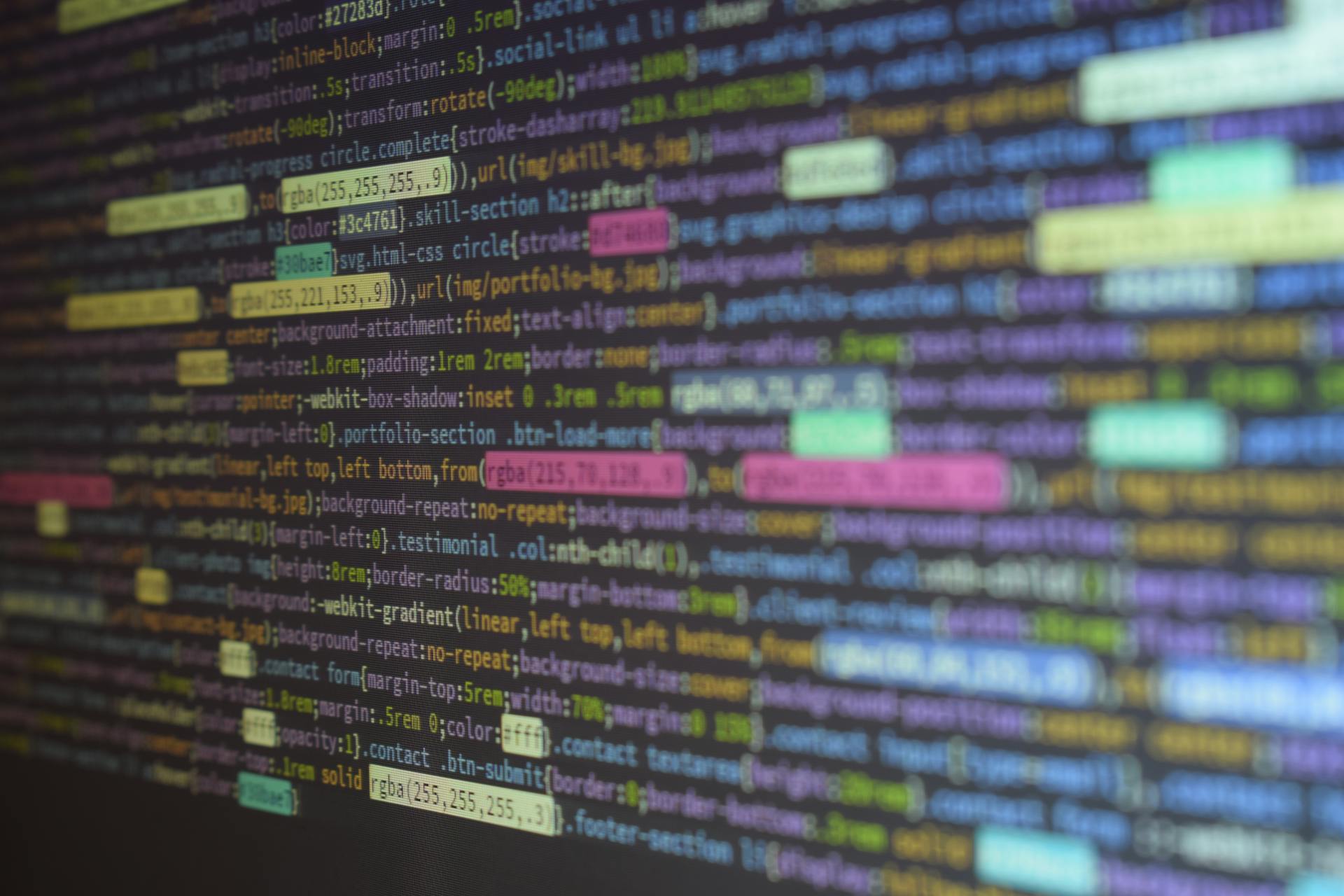
In Next.js, the `pathname` and `path` properties are used to manage client-side routing, but they have distinct differences that can impact your development workflow.
The `pathname` property is a string that represents the URL path of the page, including any query parameters. For example, if you're on a page with the URL `/about/team?name=John`, the `pathname` would be `/about/team`.
Using `pathname` can simplify your development workflow by allowing you to easily access and manipulate the current URL path. This can be particularly useful when working with client-side routing.
The `path` property, on the other hand, is an object that contains the parsed URL components, including the pathname, query parameters, and hash. For example, if you're on a page with the URL `/about/team?name=John#top`, the `path` object would contain the pathname `/about/team`, query parameters `{ name: 'John' }`, and hash `#top`.
For more insights, see: Next Js Routing
Using Pathname in Next.js
Pathname in Next.js is a powerful tool for handling URLs and routing. It's used to create a unique identifier for each page.
You can access the pathname through the use of the `pathname` property, which is available in the `router` object. This property returns the current pathname as a string.
In Next.js, pathname is used to determine the current page and its location in the URL hierarchy. It's essential for creating dynamic routes and handling URL changes.
Suggestion: Next Js Pathname
What Is a Dynamic Path Segment
In Next.js, you can define dynamic routes by creating pages with filenames that include dynamic segments enclosed in square brackets [].
Dynamic path segments are a key feature of dynamic routing in Next.js, allowing you to create pages with URLs that contain dynamic parameters.
You can create a dynamic route for blog posts with a dynamic data by creating a file named [data].js inside the pages directory.
The filename [data] is a dynamic path segment, which means that URLs can change.
Dynamic path segments are essential for building pages that interact with dynamic data, such as a blog post with a unique identifier.
A unique perspective: Pages Router Next Js
Use Pathname
Using Pathname in Next.js can be a bit tricky, but don't worry, I've got you covered.
Pathname is a built-in function in Next.js that helps you navigate your application's routes. It's like having a map to guide you through your app.
To use Pathname, you need to import it from next/router. This is a crucial step, as it allows you to access the current URL and route information.
You can use Pathname to get the current URL, like this: `import { useRouter } from 'next/router'; const { pathname } = useRouter(); console.log(pathname);`. This will log the current URL to your console.
Pathname is also useful for checking if you're on a specific page. For example, you can use `if (pathname === '/') { console.log('You are on the homepage!'); }` to check if the user is on the homepage.
A fresh viewpoint: Nextjs Get Pathname
Linking in Next.js
Linking in Next.js can be a bit tricky, especially when using the pathnames setting. This setting affects how dynamic params are passed to the Link component.
The Link component wraps next/link and localizes the pathname as necessary. It's a crucial part of creating active links in Next.js.
Depending on your pathnames setting, dynamic params can be passed in different ways. If you're using the pathnames setting, you can either pass params as an internal pathname or as an href prop.
The useSelectedLayoutSegment hook allows you to detect if a given child segment is active from within the parent layout. This returns an internal pathname that can be matched against an href.
In case you need to create a component that receives an href prop, you can compose the props from Link with the ComponentProps type. This will give you strict typing based on your routing configuration.
If you need to link to unknown routes in certain places, you can disable type checking on a case-by-case basis. This will allow unknown routes to pass through as-is, but they'll receive relevant locale prefixes in case of absolute pathnames.
If this caught your attention, see: Nextjs Pathname Server Component
Sources
- https://next-intl-docs.vercel.app/docs/routing/navigation
- https://nikolasbarwicki.com/articles/highlight-currently-active-link-in-nextjs-13-with-app-router/
- https://blog.stackademic.com/extracting-dynamic-path-segment-data-2-886ebca0f642
- https://paul.mineev.me/blog/nextjs-router-tips-and-tricks
- https://web.dev/articles/route-prefetching-in-nextjs
Featured Images: pexels.com