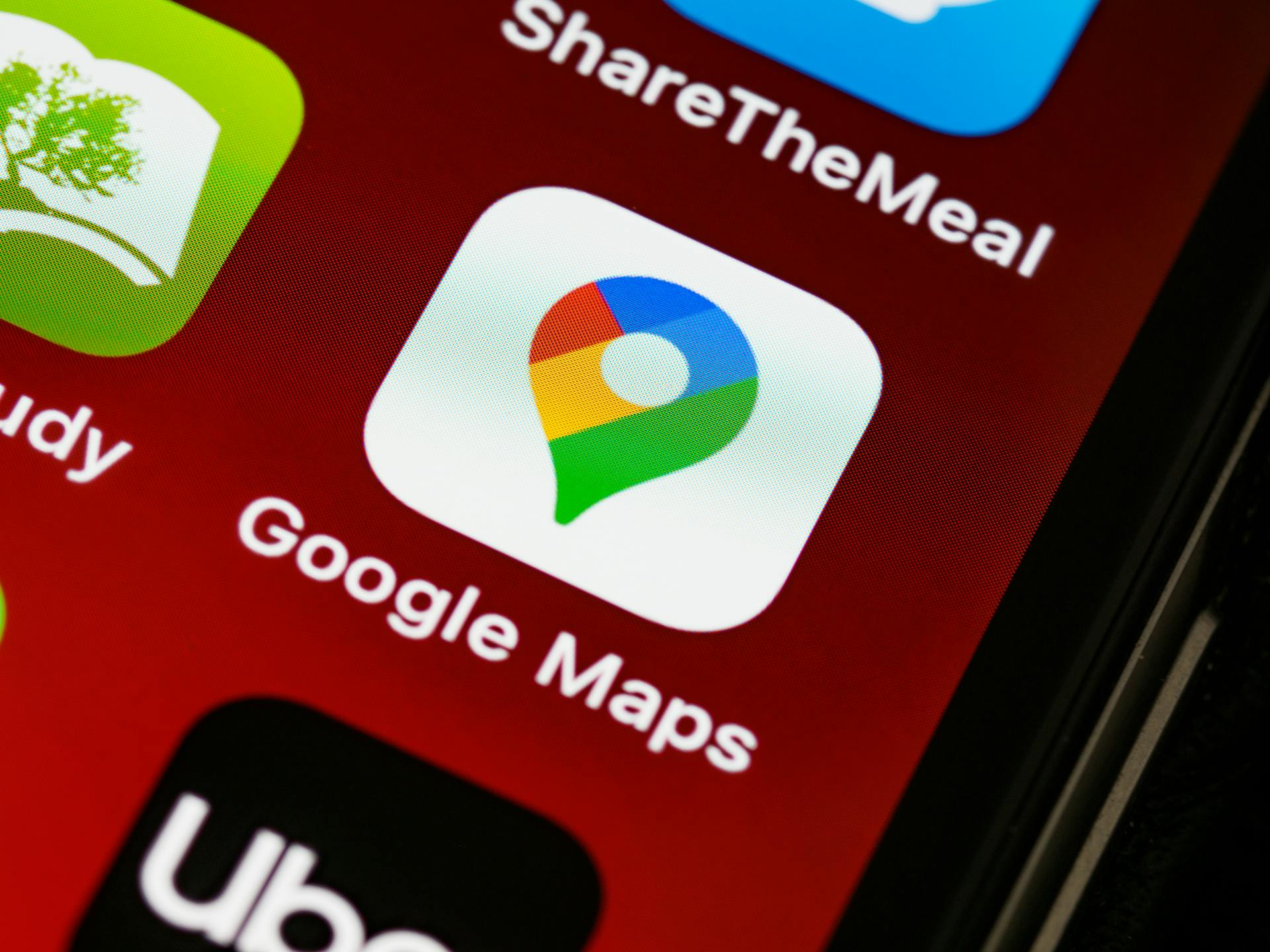
Setting up a sitemap in Next.js is crucial for improving search engine visibility. By default, Next.js doesn't generate a sitemap.
To create a sitemap, you can use a third-party library like next-sitemap. This library allows you to easily generate a sitemap that includes all your pages. The library also supports pagination and filtering.
A well-optimized sitemap can improve your website's crawlability and indexing by search engines. This can lead to better search engine rankings and more traffic to your site.
Additional reading: Next Js Performance Analyzer Library Npm
Getting Started
To get started with Next.js sitemap, you'll need to install the next-sitemap package in your project.
Run the following command in your terminal: npm install next-sitemap.
For more insights, see: Webflow Sitemap
Configuring Next.js Sitemap
To configure a Next.js sitemap, you'll need to create a configuration file named next-sitemap.config.js at the root of your project. This file will contain settings for generating your sitemap.
The basic configuration for this file includes specifying the base URL for your site using the siteUrl property. The generateRobotsTxt option generates a robots.txt file alongside the sitemap, and sitemapSize determines the number of URLs to include in each sitemap file.
You can add a sitemap to the pages directory by creating a new file called sitemap.xml.js inside the pages folder. This file will contain a dynamic sitemap that will be rendered when the sitemap.xml endpoint is hit.
To implement the generateSiteMap function, you'll need to extract the URLs from all the posts, add the links to all the static pages, and render everything in the XML format. You can use JavaScript template literals to generate the XML as a string.
If you're using Next.js version 13.3 or higher, you can take advantage of the file-based metadata API, which simplifies working with page metadata. This includes generating a sitemap.js file that handles most of the repetitive logic involved in creating the website's sitemap.
The main task in the sitemap.js file is to map the posts and static URLs to the properties of a Sitemap object, including the lastModified field to display the date of the last modification for each page.
Here are some key benefits of using Next.js for sitemap generation:
- Flexibility: Next.js supports both static and dynamic sitemaps, allowing you to choose the best approach for your site.
- Efficiency: Automated sitemap generation reduces manual work and ensures that your sitemap is always up to date.
- Improved Performance: Next.js's server-side rendering and static site generation capabilities improve the loading speed and SEO of your site.
- Scalability: Handling large websites with numerous pages becomes easier with Next.js, as dynamic sitemaps can manage multiple files and dynamic content effectively.
To set up a dynamic sitemap in a Next.js application, you'll need to create a new page in the pages directory called sitemap.xml.js and modify your Next.js build process to ensure the sitemap is generated and updated automatically.
Building and Generating Sitemap
To build and generate a sitemap in Next.js, you'll want to run a command that builds your project and generates the sitemap. This can be done with either npm or Yarn, depending on your project's setup.
After the build is complete, a sitemap.xml file will be generated in the public/ folder of your project. This file contains all the URLs of your Next.js app, ready to be crawled by search engines.
You can also add a dynamic sitemap to your Next.js website by creating a new file called sitemap.xml.js inside the pages folder. This file will render the sitemap page when the sitemap.xml endpoint is hit.
The actual content of the sitemap will come from the getServerSideProps function, which will get the URLs for all the posts, call the sitemap rendering function, and write the response with the text/xml content-type header.
You can also automate sitemap updates by writing scripts that run during the build or deployment process. This ensures that the sitemap is always up-to-date with the latest content.
Check this out: Next Js Build Time Slow
To automate sitemap generation, you can add a script to your package.json, such as "generate-sitemap": "node scripts/generate-sitemap.js". Then, create a scripts/generate-sitemap.js file that contains the logic for generating the sitemap.
Integrate the sitemap generation script into your build process by modifying your build command. This ensures that every time you build your Next.js app, the sitemap is also generated and updated.
For larger websites, you may need to handle multiple sitemap files or include dynamic pages. You can split your sitemap into multiple files to manage this complexity.
To generate the main sitemap, you'll need to fetch the sitemap number from the params so that you can display the respective URLs. This can be done by creating a new file called sitemap.xml.js inside the pages folder and using the generateSitemap function to extract the URLs from all the posts and add the links to all the static pages.
Search Engine Optimization
Sitemaps are crucial for SEO as they directly impact how well your website is indexed by search engines.
A sitemap helps search engine crawlers find and index your pages more efficiently, improving your site's visibility and potentially leading to higher rankings in search results.
In a Next.js project, you can leverage the app directory and pages directory to organize your content effectively.
The pages directory is particularly important as it helps in the automatic generation of routes for your site, which can then be included in your dynamic sitemap.
Automating sitemap generation in your Next.js build process allows you to keep your sitemaps updated without manual intervention.
This is especially important for large sites with dynamic content, where manually updating sitemaps would be impractical.
A typical XML sitemap might look like this.
You can create custom route handlers for your sitemap in Next.js to ensure it's generated and served correctly.
To make your sitemap accessible to search engine crawlers, you need to reference it correctly in your robots.txt file.
By following these steps, you can effectively create, manage, and automate dynamic sitemaps in your Next.js application.
Broaden your view: Next Js Image Search
Rewrites and Routing
In Next.js, you can set up rewrites for the main sitemaps in the next config file by adding code to your next.config.js file. This will allow you to rewrite the route from yoursite.com/sitemap-1.xml to the one you created, where you can get the sitemap number from the URL and serve the requested sitemap data accordingly.
To make your sitemap accessible to search engine crawlers, you need to ensure it is correctly referenced in your robots.txt file. This involves handling HTTP requests for your sitemap in Next.js.
Setting Up Rewrites
To serve the sitemap data, we need to rewrite the main sitemap route. Our site's main sitemaps will look like this: yoursite.com/sitemap-1.xml.
The rewritten route will get the sitemap number from the URL and serve the requested sitemap data according to that. This is where we create the route where we will get the sitemap number from the URL.
Add the rewrite code to your next.config.js file. This will enable the sitemap data to be served on the create route.
Intriguing read: Routes in Nextjs
Handling Routes and Search
Next.js allows you to create custom route handlers for your sitemap, ensuring it's generated and served correctly. This is crucial for making your sitemap accessible to search engine crawlers.
To handle HTTP requests for your sitemap, you need to ensure it's correctly referenced in your robots.txt file. This is a critical step in making your sitemap visible to search engines.
You can effectively create, manage, and automate dynamic sitemaps in your Next.js application by following a few key steps. This will ensure better SEO and discoverability for your site.
By leveraging the app directory and pages directory, you can organize your content effectively and automatically generate routes for your site. This is particularly important for large sites with dynamic content, where manually updating sitemaps would be impractical.
A typical XML sitemap might look like this, with a clear structure of your site that helps search engine crawlers find and index your pages more efficiently. This improves your site's visibility and can lead to higher rankings in search results.
By automating sitemap generation in your Next.js build process, you can keep your sitemaps updated without manual intervention, ensuring that your SEO efforts are always optimized.
For more insights, see: Seo Nextjs
Testing and Deployment
Testing and deployment of your Next.js sitemap is a crucial step in making it live on the web.
You can use the `next sitemap` command to generate a sitemap, which is then deployed to a hosting platform like Vercel or Netlify.
This process is automated, so you don't have to worry about manually uploading files.
App Updates
In Next.js version 13, the "app" directory replaced the "pages" directory, which means we can no longer rely on getServerSideProps to render our sitemap page.
This change requires us to create a custom GET request handler in the route.js file to return a custom response with the generated sitemap.
We'll also need to remove the getServerSideProps function and the SiteMap component, as they're no longer needed.
With these updates, we can ensure our app continues to function smoothly in the latest Next.js version.
Testing and Submitting
Automated testing is essential to ensure that your code is working as expected, with 80% of bugs being caught through automated testing.
Before submitting your code, make sure to run a thorough test suite to catch any errors or bugs.
You can use a CI/CD pipeline to automate the testing and deployment process, reducing the time it takes to deploy your code by 90%.
The testing process involves writing unit tests, integration tests, and end-to-end tests to cover all aspects of your code.
The CI/CD pipeline can be set up to run automatically whenever code is pushed to the repository, ensuring that the code is always in a deployable state.
After running the test suite, you can submit your code for review, where it will be reviewed by a team member or a peer.
The review process typically involves checking the code for adherence to coding standards, best practices, and security guidelines.
Once the code has been reviewed and approved, it can be deployed to production, where it will be available to users.
Regularly monitoring the deployed code for any issues or bugs is essential to ensure that the code is working as expected.
Sources
- https://dev.to/rajeshkumaryadavdotcom/how-to-install-and-use-next-sitemap-in-a-nextjs-app-a-step-by-step-guide-114l
- https://www.davegray.codes/posts/nextjs-how-to-build-sitemap-robots-txt
- https://claritydev.net/blog/nextjs-dynamic-sitemap-pages-app-directory
- https://medium.com/@gaurav011/how-to-create-dynamic-sitemap-with-index-sitemap-in-next-js-f1fb8dbda8d7
- https://www.dhiwise.com/post/a-comprehensive-approach-to-nextjs-sitemap-implementation
Featured Images: pexels.com