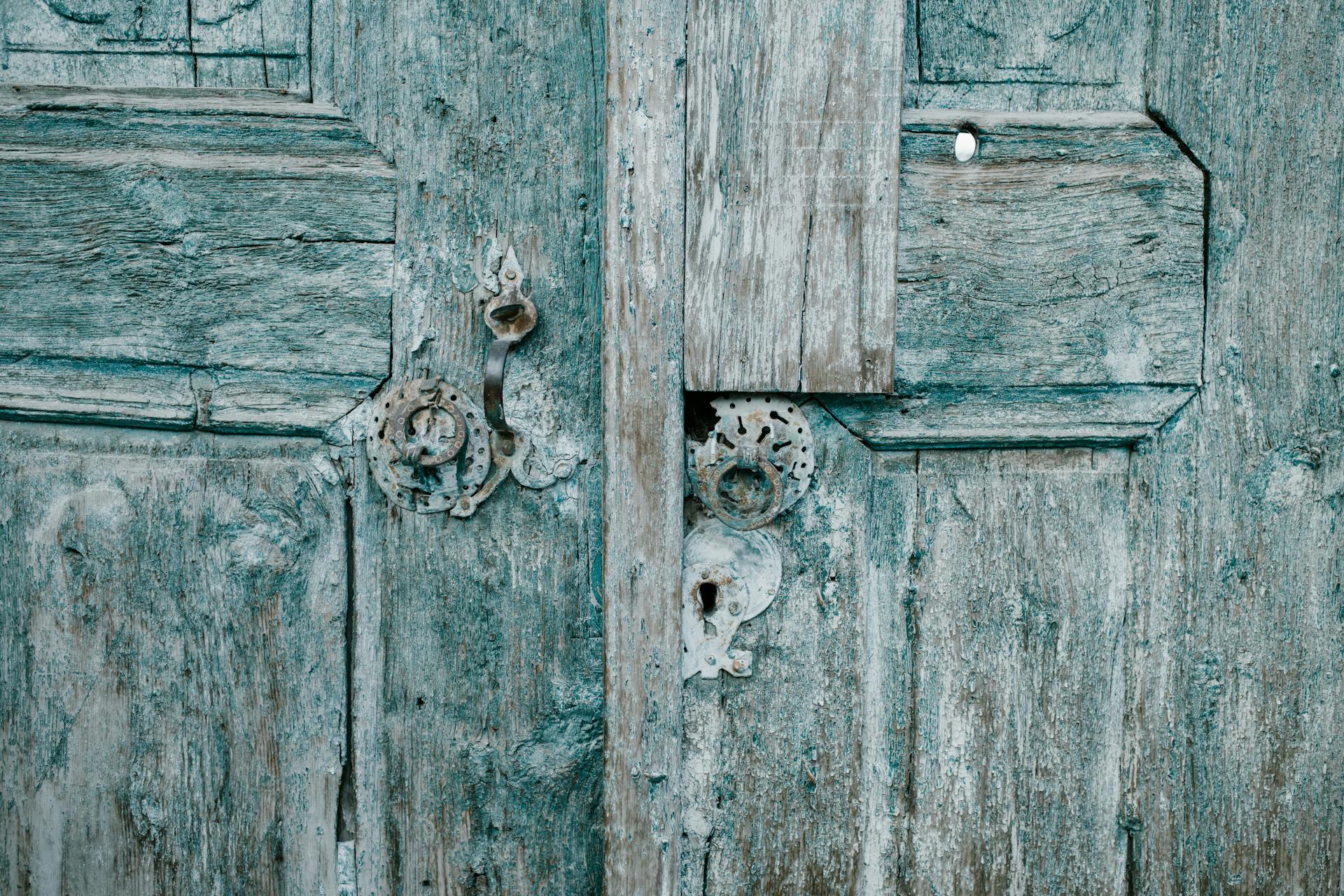
Rust web programming is a game-changer for developers seeking high-performance and secure web applications.
Actix-web is a popular framework for building web applications in Rust, offering a simple and intuitive API for creating web servers.
Rust's ownership system helps prevent common errors like null pointer exceptions, making it a safer choice for web development.
Actix-web provides built-in support for async/await, allowing for efficient handling of concurrent requests.
Worth a look: Building a Web Application
Rust Web Frameworks
Rust web frameworks offer a range of options for building web applications, each with its strengths and suited for different types of projects. Actix-web is known for its performance and flexibility, while Rocket focuses on ease of use and developer experience.
Rust offers several web frameworks that make it easier to build web applications. Each framework has its strengths and is suited for different types of projects. Some of the popular web frameworks in Rust include Actix-web, Rocket, and Warp.
Actix-web is known for its performance and flexibility, making it a popular choice for building high-performance web applications. Rocket focuses on ease of use and developer experience, making it a great choice for developers who want to build web applications quickly.
On a similar theme: Making Analytics Website in Django
Warp is a lightweight framework that emphasizes composability, making it a great choice for building web applications that require a high degree of customization.
Here's a brief comparison of some of the popular Rust web frameworks:
Ultimately, the choice of web framework depends on the specific needs of your project. By considering factors such as performance, ease of use, and community support, you can choose the best framework for your needs.
If you're looking for a lightweight framework that emphasizes composability, Warp may be the best choice. If you need a framework that prioritizes ease of use and developer experience, Rocket may be the way to go. And if you're looking for a high-performance framework with flexibility, Actix-web is definitely worth considering.
Suggestion: Best Ide for Web Programming
Rocket Framework
Rocket Framework is a web framework for Rust that emphasizes safety, speed, and ease of use. It provides a robust set of features that make it suitable for building web applications and APIs.
Consider reading: Go Programming Language Web Framework
Rocket leverages Rust's type system to ensure that data is validated at compile time, reducing runtime errors. This feature is known as Type Safety.
The framework includes tools for testing applications, making it easier to ensure code quality. Rocket also supports asynchronous programming, allowing for efficient handling of concurrent requests.
Here are some key features of Rocket:
- Type Safety: Ensures data validation at compile time.
- Asynchronous Support: Enables efficient handling of concurrent requests.
- Built-in Testing: Provides tools for testing applications.
Creating a basic Rocket application involves setting up a Rust environment and writing a simple web server. To get started, you'll need to install Rust and create a new project using Cargo.
Here are the steps to create a basic Rocket application:
- Install Rust.
- Create a new project using Cargo.
- Add Rocket as a dependency in Cargo.toml.
- Write a simple "Hello, World!" application in src/main.rs.
- Access the application by navigating to http://localhost:8000 in your web browser.
Warp Framework
Warp is a lightweight and flexible web framework for Rust that emphasizes composability.
It provides a powerful warp filter system for clean and maintainable code.
Warp simplifies the process of handling WebSocket connections, allowing developers to define routes that can upgrade HTTP connections to WebSocket connections seamlessly.
Warp's filter system is based on reusable components that can be combined to create intricate request handling logic.
Filters can be composed using operators like and, or, and map, facilitating a clean and modular approach to constructing web applications.
Warp's filter system ensures type safety, significantly reducing the likelihood of runtime errors and enhancing application stability.
Filters can return errors, which can be managed gracefully, leading to a better user experience and simplifying debugging processes.
Here are some key concepts of Warp's filter system:
- Filter Composition: Filters can be combined using operators like and, or, and map.
- Type Safety: Warp leverages Rust's robust type system to ensure that filters are type-safe.
- Error Handling: Filters can return errors, which can be managed gracefully.
Warp's performance and flexibility make it a popular choice for building web applications in Rust.
Comparison of Frameworks
Choosing the right framework for your Rust web project can be overwhelming, especially with the numerous options available. Actix-web is known for its performance and flexibility, making it a popular choice.
Rust offers several web frameworks, each with its strengths and weaknesses. Rocket focuses on ease of use and developer experience, while Warp emphasizes composability and is a lightweight framework.
To help you make an informed decision, let's break down the key factors to consider when choosing a framework. Performance, ease of use, and community support are crucial considerations.
Check this out: Django (web Framework)
Here's a comparison of some popular Rust web frameworks:
Ultimately, the right framework for your project depends on your specific needs and goals. By considering the strengths and weaknesses of each framework, you can make an informed decision and choose the best tool for the job.
Server-Side Rendering
Server-Side Rendering is a technique where web pages are generated on the server and sent to the client as fully rendered HTML. This approach is increasingly being used in Rust web programming due to its efficiency and concurrency capabilities.
Rust's compiled nature allows for faster execution times compared to interpreted languages, ensuring that your web applications load quickly and efficiently. This is a huge plus for users who hate waiting for web pages to load.
Rust's ownership model prevents common bugs such as null pointer dereferences and data races, leading to more stable applications that enhance user experience and reduce maintenance costs. This means you can focus on building great features instead of debugging issues.
Consider reading: C Programming Web Server
To implement Server-Side Rendering with Rust, you can use frameworks like Actix-web or Rocket. These frameworks provide a solid foundation for building scalable and efficient web applications.
Here are the benefits of using Rust for Server-Side Rendering:
- Performance: Rust's compiled nature allows for faster execution times.
- Memory Safety: Rust's ownership model prevents common bugs.
- Concurrency: Rust's async features enable handling multiple requests simultaneously.
To get started with Server-Side Rendering in Rust, you'll need to install Rust and set up your environment. Then, create a new Rust project using Cargo and add dependencies for your chosen web framework.
Worth a look: Dropbox Rust
Assembly and WASM
Assembly and WASM is a crucial aspect of Rust web programming, allowing developers to run code written in languages like Rust in the browser, providing near-native performance.
Rust compiles to WebAssembly, enabling high-performance applications in the browser, which can significantly enhance user engagement and satisfaction.
WebAssembly is a low-level bytecode format that runs in modern web browsers, designed to be a compilation target for high-level languages like Rust, C, or C++.
Key features of WebAssembly include portability, performance, and security, making it highly portable across different devices and browsers, and running in a safe, sandboxed environment, minimizing the risk of security vulnerabilities.
Curious to learn more? Check out: Browser without Tracking
Rust's strong type system and memory safety features carry over to WebAssembly, reducing runtime errors and improving the reliability of your applications.
To get started with Rust and WebAssembly, you need to install the Rust toolchain and the wasm-pack tool, create a new Rust project, write your Rust code and compile it to WebAssembly, use wasm-pack to build and package your project for use in web applications, and integrate the generated WebAssembly module into your JavaScript code.
Here are the steps to integrate WebAssembly with JavaScript frameworks like React, Vue, or Angular:
- Choose Your Framework
- Decide on a JavaScript framework (e.g., React, Vue, Angular).
- Set Up Your Framework Project
- For React, create a new project using Create React App:
- Install WASM Package
- Copy the generated WASM package from your Rust project into your JavaScript project.
- Load WASM in Your Framework
- In your React component, load the WASM module:
By following these steps, you can create efficient and safe web applications that take full advantage of WebAssembly's performance benefits, and enhance user engagement and satisfaction.
Database Connectivity
Database connectivity is a crucial aspect of Rust web programming, and there are several libraries that make it easy to interact with various databases. Diesel is a popular ORM (Object-Relational Mapping) library that supports PostgreSQL, SQLite, and MySQL databases.
To use Diesel, you need to add it as a dependency in your Cargo.toml file, specifying the version and features you need. For example, to use PostgreSQL, you would add `diesel = { version = "1.4", features = ["postgres"] }`.
You can then use Diesel's query builder to interact with your database. For example, you can use the `insert_into` method to create a new record, like this: `insert_into(users::table).values(&new_user).execute(&connection)?`. This is a much safer and more efficient way to interact with your database than writing raw SQL queries.
Some common libraries for database connectivity in Rust include diesel, sqlx, and diesel.
Curious to learn more? Check out: Cloudfront Aws Webflow Example
Rocket Database Integration
Rocket Database Integration is a powerful tool for storing and retrieving data efficiently. To integrate a database with Rocket, you need to add dependencies to your Cargo.toml file, including diesel and the database driver you need.
For example, if you're using PostgreSQL, you would add the following line to your Cargo.toml file:
You might enjoy: Css in Html File
```toml
[diesel]
version = "1.4"
features = ["postgres"]
```
This will allow you to use the diesel ORM to interact with your PostgreSQL database.
Once you have added the dependencies, you can set up the database by creating a new database and running migrations using Diesel CLI.
To create a connection pool, you can use Rocket's built-in support for connection pooling. Here's an example of how to do it:
```rust
#[macro_use]
extern crate rocket;
use rocket_sync_db_pools::{database, diesel};
#[database("my_db")]
struct DbConn(diesel::PgConnection);
#[get("/users")]
async fn get_users(conn: DbConn) -> String {
// Query the database
"List of users"
}
```
This will create a new connection pool called "my_db" that you can use to interact with your database.
You can also configure the database in Rocket.toml file by adding the following lines:
```toml
[global.databases]
my_db = { url = "postgres://user:password@localhost/my_db" }
```
This will allow you to access the database configuration from your Rocket application.
By following these steps, you can create a basic Rocket application that integrates with a database using diesel ORM.
Readers also liked: How to Use Inspect Element to Find Answers
MongoDB
MongoDB is a NoSQL database that stores data in flexible, JSON-like documents. This allows for efficient data storage and retrieval.
Check this out: Data Text Html Charset Utf 8 Base64
Rust has a dedicated driver for MongoDB, which enables developers to interact with the database efficiently. You can use this driver to perform various database operations.
To set up MongoDB, you need to add the MongoDB driver to your Cargo.toml file. This is done by specifying the dependency in the following format: "-a1b2c3-mongodb = "2.0"".
Here are the basic steps to get started with MongoDB in Rust:
- Set up MongoDB by adding the MongoDB driver to your Cargo.toml file.
- Create a client instance using the `Client` type from the `mongodb` crate.
- Connect to the database using the `ClientOptions` type.
- Perform CRUD (Create, Read, Update, Delete) operations on the database.
Here's a basic example of how to perform CRUD operations in MongoDB using Rust:
- Create a new user document and insert it into the database.
- Update an existing user document.
- Delete a user document.
This can be achieved using the following code:
```rust
let mut client_options = ClientOptions::parse("mongodb://localhost:27017").await?;
let client = Client::with_options(client_options)?;
let collection = client.database("test").collection("users");
let new_user = doc! { "name": "John Doe", "email": "[email protected]" };
collection.insert_one(new_user, None).await?;
collection.update_one(doc! { "name": "John Doe" }, doc! { "$set": { "name": "Jane Doe" } }, None).await?;
collection.delete_one(doc! { "name": "Jane Doe" }, None).await?;
```
Check this out: Web Traffic Name
44 Packages
As you start working with database connectivity, you'll likely encounter a wide range of packages. A total of 44 packages are available in the standard library for Python's database connectivity.
These packages can be broadly categorized into two types: low-level and high-level. Low-level packages provide direct access to the database, while high-level packages abstract away the complexities of database interactions.
One of the most widely used low-level packages is sqlite3, which is a built-in Python package that allows you to interact with SQLite databases. It's a great choice for small projects or prototyping.
Another popular low-level package is psycopg2, which is a PostgreSQL database adapter for Python. It provides a high degree of control over database interactions and is a good choice for complex projects.
High-level packages, on the other hand, provide a more abstracted interface to the database. They often include features like automatic connection management and query building. One of the most popular high-level packages is SQLAlchemy, which is a SQL toolkit and Object-Relational Mapping (ORM) system.
Recommended read: Web Programming Using Python
Authentication and Security
Authentication and Security is a crucial aspect of Rust web programming. Implementing proper authentication and security measures helps protect user data from unauthorized access and enhances the overall security of your application.
To secure user credentials, Rapid Innovation recommends hashing passwords before storing them in the database. This is a crucial aspect of security, as storing plain text passwords poses a significant risk. Hashing passwords ensures that even if an attacker gains access to the database, they won't be able to obtain the original passwords.
Hashing passwords involves using a hashing algorithm, such as bcrypt, to convert the password into a fixed-length string of characters. This string is then stored in the database. When a user attempts to log in, the password is hashed again and compared to the stored hash to verify the password.
Here's an example of how to hash a password using bcrypt:
```javascript
const bcrypt = require('bcrypt');
const saltRounds = 10;
const password = 'userPassword';
bcrypt.hash(password, saltRounds, (err, hash) => {
console.log(hash);
});
```
To verify a password, you can use the `bcrypt.compare()` function, which compares the entered password to the stored hash.
```javascript
const enteredPassword = 'userEnteredPassword';
const storedHash = 'storedHashFromDB';
bcrypt.compare(enteredPassword, storedHash, (err, result) => {
if (result) {
console.log('Password is valid');
} else {
console.log('Invalid password');
}
});
```
In addition to password hashing, implementing HTTPS and TLS in your Rust web application is also crucial for securing data in transit. This ensures that sensitive information, such as user credentials and personal data, is encrypted and protected from eavesdropping and tampering.
On a similar theme: Webflow Password Protect
Here's an example of how to set up HTTPS in Rust using the `rustls` crate:
```rust
use warp::Filter;
use std::sync::Arc;
use rustls::{ServerConfig, NoClientAuth};
use std::fs::File;
use std::io::BufReader;
#[tokio::main]
async fn main() {
let certs = {
let cert_file = &mut BufReader::new(File::open("cert.pem").unwrap());
rustls::internal::pemfile::certs(cert_file).unwrap()
};
let mut config = ServerConfig::new(NoClientAuth::new());
config.set_single_cert(certs, rustls::PrivateKey(
std::fs::read("key.pem").unwrap()
)).unwrap();
let addr = ([127, 0, 0, 1], 3030).into();
let server = warp::serve(warp::path("hello").map(|| "Hello, HTTPS!"));
let tls_acceptor = Arc::new(rustls::ServerSession::new(&config));
warp::serve(server).tls(tls_acceptor).run(addr).await;
}
```
Testing HTTPS is also important to ensure that your server is set up correctly. You can use tools like `curl` to test your HTTPS server.
```bash
curl -k https://localhost:3030/hello
```
By implementing password hashing and HTTPS, you can significantly enhance the security of your Rust web application and protect user data from unauthorized access.
A fresh viewpoint: The Html File
Testing and Debugging
Testing and debugging are essential parts of the development process for Rust web applications. They help ensure that the application behaves as expected and is free from bugs.
There are several types of testing, including unit testing, integration testing, and end-to-end testing. Unit testing tests individual components for correctness, integration testing tests how different components work together, and end-to-end testing tests the entire application flow from start to finish.
A unique perspective: Webflow Ab Testing
For debugging, you can use the Rust compiler's built-in error messages to identify issues, leverage the dbg! macro to print variable values during execution, and utilize logging libraries like log or env_logger for more structured logging.
Here are the different types of testing:
- Unit Testing: Tests individual components for correctness.
- Integration Testing: Tests how different components work together.
- End-to-End Testing: Tests the entire application flow from start to finish.
By following these guidelines, developers can ensure that their Rust web applications are secure, reliable, and maintainable.
Request Guards and Forms
Request Guards and Forms are powerful tools in Rocket that help you validate incoming requests and handle form submissions.
To implement request guards, you need to define a struct that implements the FromRequest trait. This allows you to check for specific headers, validate query parameters, or enforce authentication. For example, you can create a guard that checks for an API key.
Request guards can be used to enforce authentication by checking for a specific header, such as the "x-api-key" header. You can do this by implementing the FromRequest trait and checking if the header exists.
To handle forms, you need to define a struct that represents the form data. This struct should derive the FromForm trait, which allows Rocket to automatically populate the struct with form data.
Here's an example of how you can use request guards and forms in Rocket:
- Request guards can be used to check for specific headers, such as the "x-api-key" header.
- Forms can be handled by defining a struct that represents the form data and deriving the FromForm trait.
- Request guards and forms can be used together to create a secure route that checks for an API key and handles form submissions.
In the example code, the secure route checks for an API key using a request guard and handles form submissions using the FromForm trait. This allows you to create a secure route that validates incoming requests and handles form submissions.
Testing and Debugging
Testing and debugging are essential parts of the development process for Rust web applications. They help ensure that the application behaves as expected and is free from bugs.
Types of testing include unit testing, integration testing, and end-to-end testing. Unit testing tests individual components for correctness, integration testing tests how different components work together, and end-to-end testing tests the entire application flow from start to finish.
Use the Rust compiler's built-in error messages to identify issues, leverage the dbg! macro to print variable values during execution, and utilize logging libraries like log or env_logger for more structured logging.
To run tests, use the command specified in the relevant section.
Best practices for testing include writing tests for all public functions, keeping tests isolated and independent, and using descriptive names for test functions to clarify their purpose.
By following these guidelines, developers can ensure that their Rust web applications are secure, reliable, and maintainable.
Here are some common debugging tools:
- Integrated Development Environments (IDEs)
- Debugging Proxies
- Log Analyzers
These tools can help developers set breakpoints, inspect variables, and step through code.
Best practices for debugging include reproducing the bug consistently before attempting to fix it, isolating the problematic code to understand the root cause, and keeping a record of bugs and their resolutions for future reference.
Here's an interesting read: Azure Blue Hex Code
Caching Strategies
Caching is a powerful technique used to improve application performance by storing frequently accessed data in memory. This can significantly reduce the load on servers and improve user experience.
In-Memory Caching is a recommended caching strategy that stores data in the application's memory, making it readily available for future requests. This can lead to significant performance improvements.
HTTP Caching is another recommended strategy that stores frequently accessed data in a web browser's cache, reducing the need for repeated requests to the server. This can improve page load times and overall user experience.
Database Query Caching stores frequently executed database queries in memory, reducing the load on the database and improving application performance. This can be especially beneficial for applications with high traffic.
Content Delivery Networks (CDNs) are also a recommended caching strategy that store frequently accessed data at edge locations around the world, reducing latency and improving application performance. This can be especially beneficial for global applications.
Application-Level Caching is a caching strategy that stores data at the application level, making it readily available for future requests. This can lead to significant performance improvements.
Error Handling
Error handling is a crucial aspect of software development, and it's essential to have a solid understanding of how to handle errors effectively.
In Rust, error handling is unique and utilizes the Result and Option types to manage errors effectively. The Result type represents either success (Ok) or failure (Err), while the Option type represents a value that may or may not be present (Some or None).
Using the ? operator simplifies error propagation by returning early if an error occurs. Custom error types can be defined to provide more context about failures, and error logging can be used to log errors for monitoring and debugging.
Graceful degradation is also essential, ensuring that the application can handle errors gracefully and provide user-friendly messages. This can be achieved by returning appropriate HTTP status codes and error messages.
Here are some key strategies for error handling in Rust web applications:
By following these strategies, developers can create robust RESTful APIs that meet the needs of their applications and users.
Deployment and DevOps
Deployment and DevOps is a crucial aspect of Rust web programming. At Rapid Innovation, we specialize in helping clients achieve greater ROI through streamlined processes and reduced time-to-market.
Serverless architecture is a great way to build and run applications without managing servers. With Rust, you can deploy functions in a serverless environment by choosing a serverless platform, setting up your Rust environment, writing your function, creating a Cargo.toml file, building your project, packaging your function, deploying to the serverless platform, and testing your function.
Containerization is another powerful method for deploying applications. Docker is a popular tool for this purpose, and it works exceptionally well with Rust web applications. By using Docker, you can ensure isolation, portability, and scalability for your applications.
To containerize a Rust web application using Docker, you'll need to create a Dockerfile in the root of your Rust project. This file will specify the base image, working directory, and other instructions for building the Docker image. Here's a simple example of a Dockerfile:
Once you've created the Dockerfile, you can build the Docker image by running `docker build -t my-rust-app .`. You can then run the application by executing `docker run -p 8080:8080 my-rust-app` and verifying that it's working by visiting `http://localhost:8080`.
Continuous Integration and Deployment (CI/CD) is another key component of DevOps. By implementing CI/CD, you can streamline the development process and improve code quality. With CI/CD, you can automatically test and merge code changes into a shared repository, and then deploy the application to production after passing tests.
To set up CI/CD for a Rust web application, you'll need to choose a CI/CD platform (e.g., GitHub Actions, GitLab CI, Travis CI) and create a configuration file. For example, using GitHub Actions, you can create a `.github/workflows/ci.yml` file that specifies the build and deployment steps. Here's an example of a CI/CD workflow file:
```yaml
name: Rust CI
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Rust
uses: actions-rs/toolchain@v1
with:
toolchain: stable
- name: Build
run: cargo build --verbose
- name: Run tests
run: cargo test --verbose
```
By implementing CI/CD and containerization, you can enhance the deployment process of Rust web applications and ensure a more efficient and reliable workflow.
For another approach, see: Web Designers Code
Sources
- https://www.rapidinnovation.io/post/rust-in-web-development-frameworks-tools-and-best-practices
- https://www.bandofcoders.com/blog/rust-web-development-build-blazing-fast-secure-apps
- https://rust.libhunt.com/categories/1630-web-programming
- https://www.pixelcrayons.com/blog/software-development/go-vs-rust/
- https://en.wikipedia.org/wiki/Rust_(programming_language)
Featured Images: pexels.com