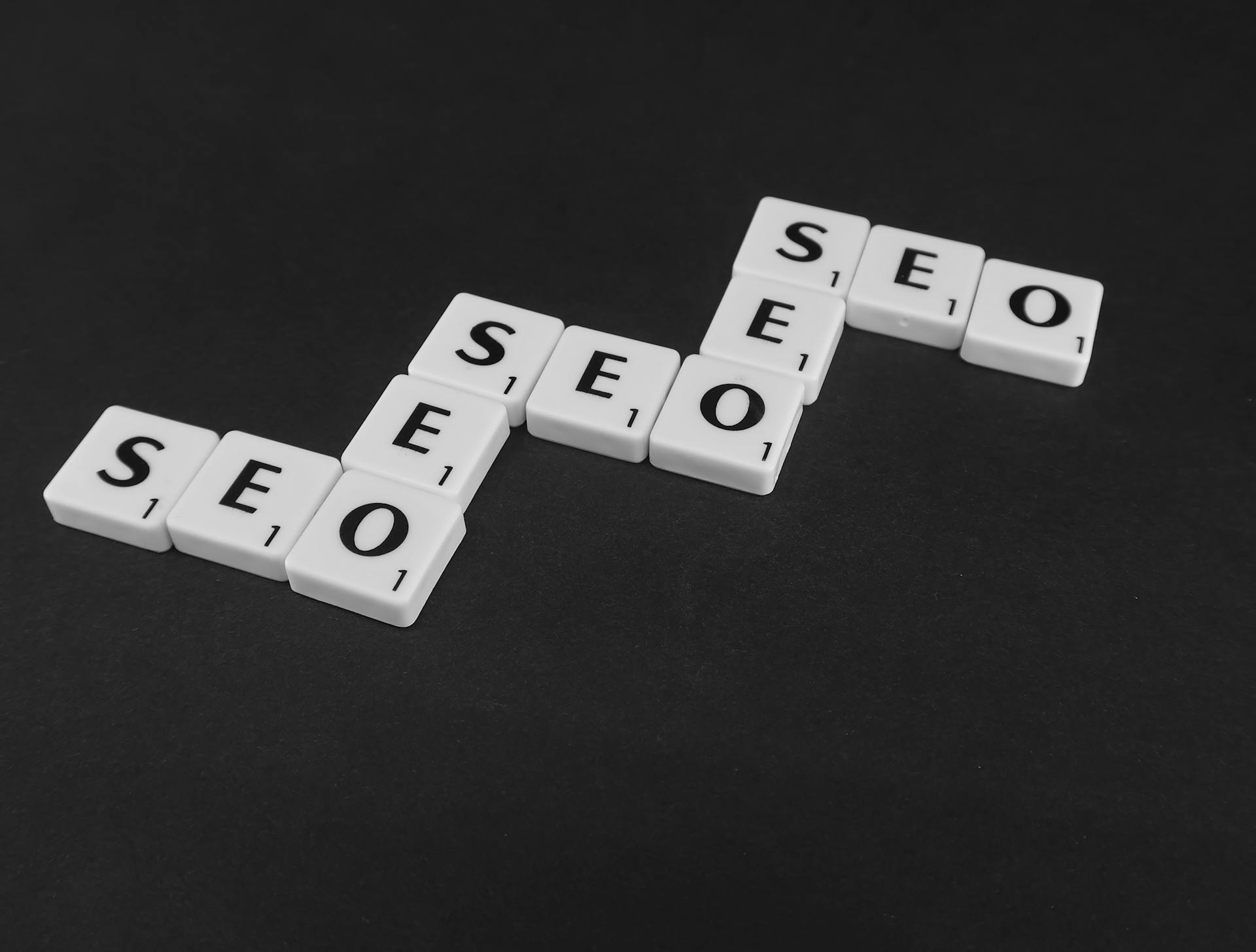
Building Algolia Next.js search with Next.js and Contentful is a powerful combination for creating fast and scalable search experiences. This approach leverages the strengths of each technology to deliver a seamless user experience.
Next.js provides a solid foundation for building server-rendered React applications, while Contentful offers a headless CMS for managing content. By integrating Algolia's search API, you can unlock the full potential of your search functionality.
Algolia's InstantSearch library is designed to work seamlessly with Next.js, allowing you to easily integrate search into your application. With Algolia's API, you can index your content in real-time, ensuring that your search results are always up-to-date and accurate.
By combining these technologies, you can create a search experience that is both fast and relevant, providing a better user experience for your customers.
Get Started
To get started with Algolia and Next.js, you can start by checking out the GitHub repository that supports the article. Clone the demo app and launch it locally using the provided commands.
You can use DatoCMS's Next.js Blog starter project for simplicity, but keep in mind that any other Next.js project will work. Follow the instructions in the GitHub repository of the Next.js Blog starter project.
Choose Vercel as your hosting and deployment platform, and then follow the initialization process until you reach the confirmation window. You'll be able to access your Vercel project at this point.
The demo application's public URL provided by Vercel is available, and you can launch the project by running the git clone command shown in the confirmation window. This will download the project created automatically by the process.
You're now ready to integrate Algolia InstantSearch, and the first step is to define a SearchBox component.
Contentful Setup
To set up Contentful for Algolia integration, start by going to your Contentful Environment settings and finding the Algolia template. You may need to expand all templates to find it.
You'll need to get your Algolia App ID, Index Name, and API key to configure the Algolia webhook in Contentful. The App ID and name can be found on your main Algolia dashboard by clicking the index name up top.
The API key is located in the Algolia Settings Menu > API Keys. Contentful provides a handy webhook template for Algolia integration that you can start with and customize as needed.
You can customize what is being sent to Algolia via filters and payload. For example, if you want only your blog contents to be indexed, you can add a filter. Then a custom payload can be created to index only specific fields if you choose.
A custom payload is sent as a JSON value. Contentful also allows you to create your own logic in your code that can take care of indexing if a webhook isn't enough.
Installing and Configuring
To get started with Algolia in your Next.js project, you'll need to install the required packages. You can do this by running the command `algoliasearch` to connect to the Algolia API.
The two main packages you'll need are `algoliasearch` and `dotenv`, which will allow you to access environment variables outside of the Next.js application.
Here are the specific packages you'll need to install:
- algoliasearch — to connect to the Algolia API
- dotenv — to access environment variables outside of the Next.js application
Once you've installed the packages, you can update your code to import the necessary components. This will involve importing the Search component in your `my-nextjs-project/src/index.tsx` file.
What We'll Do
To install and configure Algolia, we'll break it down into manageable steps.
We'll start by setting up Algolia to receive data that powers search results on a web application. This is a crucial step, as it enables the search functionality on our site.
The process involves three main steps. Here's a quick rundown of what we'll cover:
- Setting up Algolia to receive data
- Creating a custom script to transform and send the data to Algolia
- Building out the search UI in a Next.js application using the Algolia React InstantSearch UI
This setup will take us just three steps to complete.
Installing Client
To get started with installing the Algolia search client in your Next.js project, you'll need to install the algoliasearch package. This package is a JavaScript client library for the Algolia search engine, providing methods to interact with the Algolia search API.
You can install it using the command `algoliasearch` in your terminal. Additionally, you'll need to install the dotenv package to access environment variables outside of the Next.js application. This is necessary for storing sensitive information like API keys.
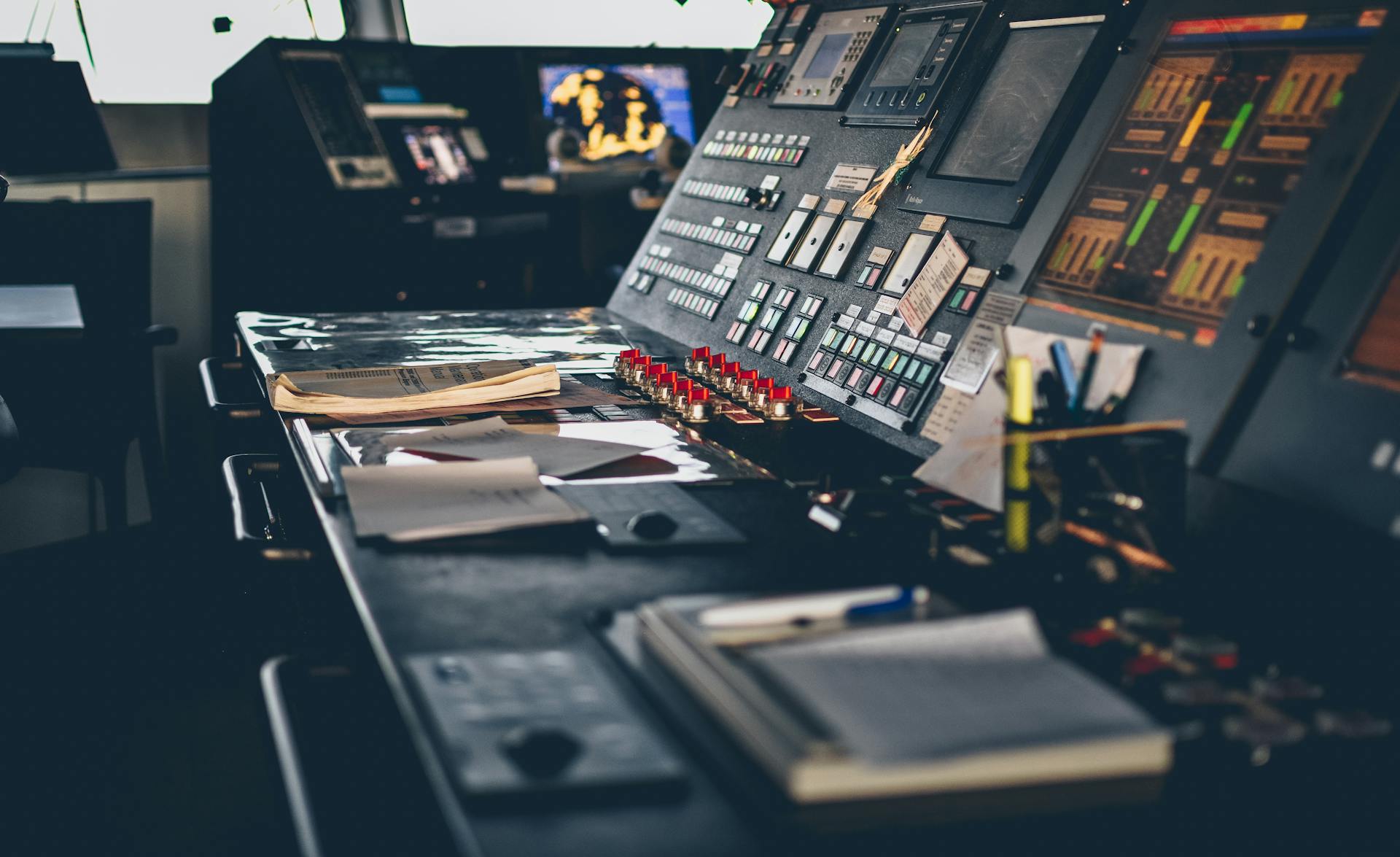
Here are the dependencies you'll need to install:
- algoliasearch — to connect to the Algolia API
- dotenv — to access environment variables outside of the Next.js application
Once you've installed the packages, you can update your code to import the Search component. This is a pre-built component from the react-instantsearch-dom package that can be seamlessly integrated into your React application to create a dynamic search experience.
Frontend Integration
You can integrate Algolia InstantSearch in your Next.js frontend by building custom search components that fit your application's style and interaction. This is especially useful when the basic components provided by Algolia don't meet your needs.
To get started, create a components folder inside your Next.js project directory and create a new file named Search.tsx. Initialize the Algolia client with your Application ID and Search-Only API Key, which you can find in your Algolia Account Settings.
You can then use the default InstantSearch components, such as InstantSearch, SearchBox, and Hits UI, to render the search UI in your Next.js application. These components work nicely with server-side rendering, so you can use them on Next.js page files out of the box.
Here are the steps to use the default InstantSearch components:
1. Create a new component file for the InstantSearch code and import the algoliasearch dependency.
2. Initialise a new algoliasearch client with the public environment variables you set up earlier.
3. Import the InstantSearch, SearchBox, and Hits UI components and render them in the component as follows:
- Pass the searchClient and the indexName you set up with Algolia as props into the InstantSearch component.
By following these steps, you can easily integrate Algolia InstantSearch into your Next.js frontend and provide a better search experience for your users.
Rendering the UI
Rendering the UI is a crucial part of any search functionality, and Algolia's InstantSearch makes it incredibly easy. Algolia provides a great UI interface they call Instant Search, which is available for JavaScript and other frameworks.
You can use the default InstantSearch components to get started quickly, and they work nicely with server-side rendering, making them a great fit for Next.js applications. In fact, InstantSearch works out-of-the-box with Next.js page files, so you can import the new component to your existing blog index page without any issues.
To render the search UI, you'll need to create a new component file for the InstantSearch code and import the algoliasearch dependency. Then, initialize a new algoliasearch client with the public environment variables you set up earlier.
Here's a brief overview of the key components you'll need to render the search UI:
- InstantSearch: the core component that handles the search functionality
- SearchBox: the component that allows users to input their search query
- Hits: the component that displays the search results
You can customize these components to fit your needs, and even add your own CSS styles or custom React Hooks to integrate with InstantSearch.
By using the default InstantSearch components, you can get up and running quickly, and then customize them to fit your specific use case. This approach allows you to take advantage of the power of InstantSearch while still having full control over the UI and functionality of your search bar.
With InstantSearch, you can also create custom search components to give you more control over the UI and CSS, and to only render the search results when there's a search query present in the input field. This is a great way to optimize the performance of your search bar and provide a better user experience.
By following these steps and using the InstantSearch UI components, you can create a seamless and efficient search experience for your users.
Listen for Changes
To integrate your frontend with Algolia, you'll want to listen for changes in your database. This can be done by creating a searchClient with your Algolia keys.
Creating a searchClient is the first step, which involves connecting to MongoDB and creating a dummy User model. A changeStream can then be created on the User model to listen for changes in the User collection.
To listen for changes, use the on method and pass the change string along with a callback function. This function will be called whenever there is a change in the User collection.
The data passed to the callback function will contain the change information, which includes operationType, fullDocument, and documentKey. These are the key pieces of information you'll need to update your Algolia index.
To get these details, you'll need to pass a callback function to the on method, which will be called whenever there's a change in the User collection.
Syncing and Updating
To keep your Algolia search results up-to-date, you need to sync your data regularly. This can be done through a Cron Job that periodically syncs the data between your Database and Algolia.
Manual syncing is another option, where you can call a function that applies changes to Algolia whenever you modify data in your Database. This approach requires you to do the same for every function that modifies the data.
Listening for changes in your Database is a more event-driven approach. This can be done using ChangeStream in MongoDB, but for Next.js, you'll need a separate node server to handle this.
Syncing with Strapi
To keep content in sync with Algolia, we need to get data directly from our Algolia index. This is done in the components/Search.tsx file.
We specify the index name in the Strapi Algolia plugin configuration, which automatically appends the environment name to the index name. For example, our index name becomes development_strapi-store.
We use connectSearchBox to create a custom search input, as the default input field is not styled. This allows us to customize the search experience.
We also use connectHits to create CustomHits, which enables us to configure how products are rendered. This is useful for creating a user-friendly search experience.
Sync
Sync is a crucial step in ensuring that your data is always up-to-date and accurate. You can sync data with Algolia using a Cron Job, which periodically syncs data between your database and Algolia.
To keep data in sync, you need to update the corresponding data in Algolia whenever you add, update, or delete data in your database. Otherwise, you'll have outdated or wrong search results.
A Cron Job can be run periodically to sync data, but you can also manually sync data whenever you make changes to your database. This involves calling another function that applies the same changes to Algolia.
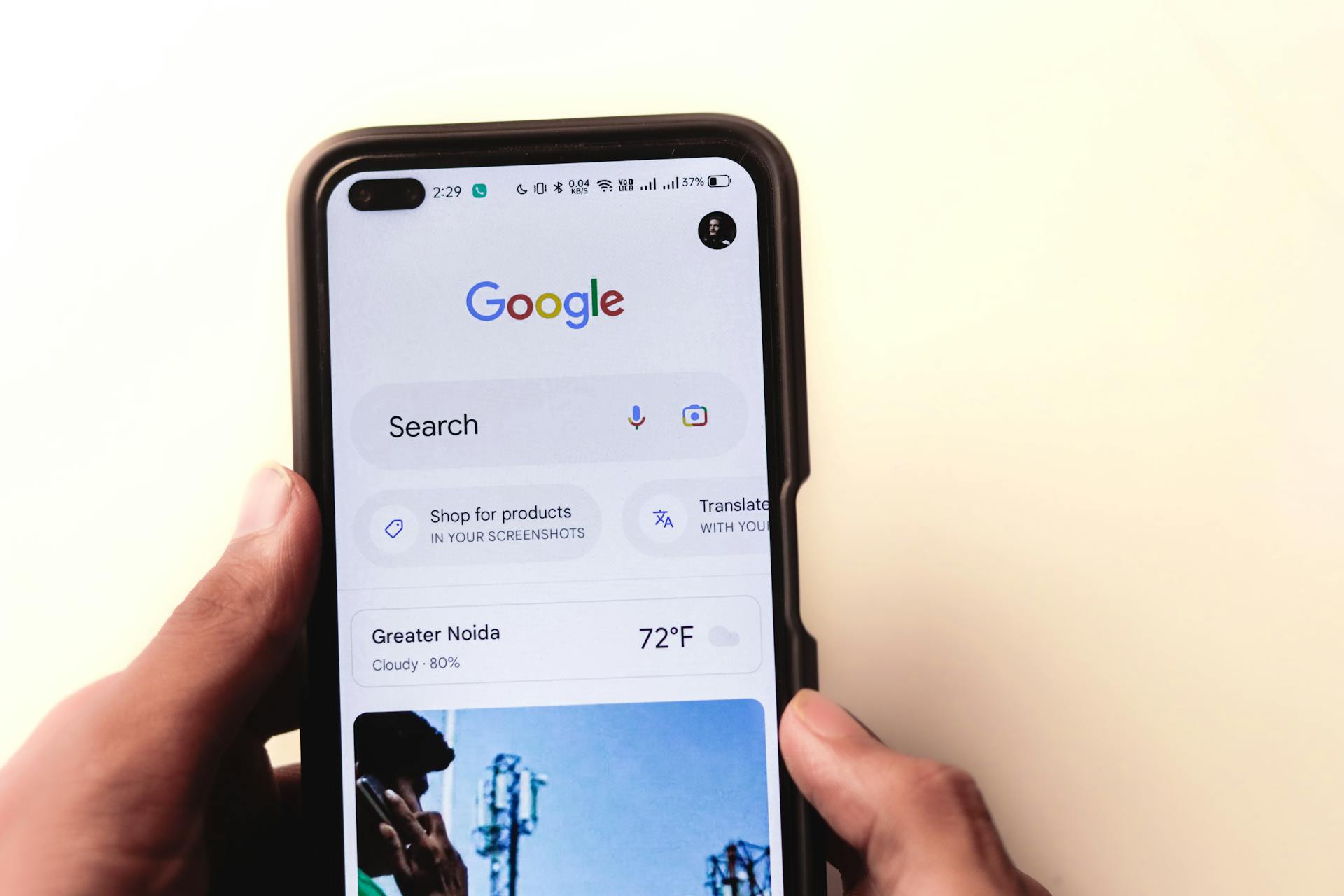
Event-Driven Sync is another approach, where you listen for changes in your database and apply the same changes to Algolia. This can be done using ChangeStream in MongoDB, but it's not possible on Nextjs, so a separate node server is needed.
You can verify that the data synchronization process works by clicking "Save" and "Publish" in your CMS, which will perform an HTTP POST request towards the specified endpoint. The new data should then appear in your Algolia index data page.
Sources
- https://www.xtivia.com/blog/integrating-contentful-algolia-nextjs/
- https://strapi.io/blog/adding-advanced-search-to-a-strapi-and-next-js-project-with-algolia
- https://dev.to/thatanjan/full-stack-search-with-algolia-nextjs-and-mongodb-part-1-1n14
- https://www.contentful.com/blog/add-algolia-instantsearch-to-nextjs-app/
- https://www.datocms.com/blog/algolia-nextjs-how-to-add-algolia-instantsearch
Featured Images: pexels.com