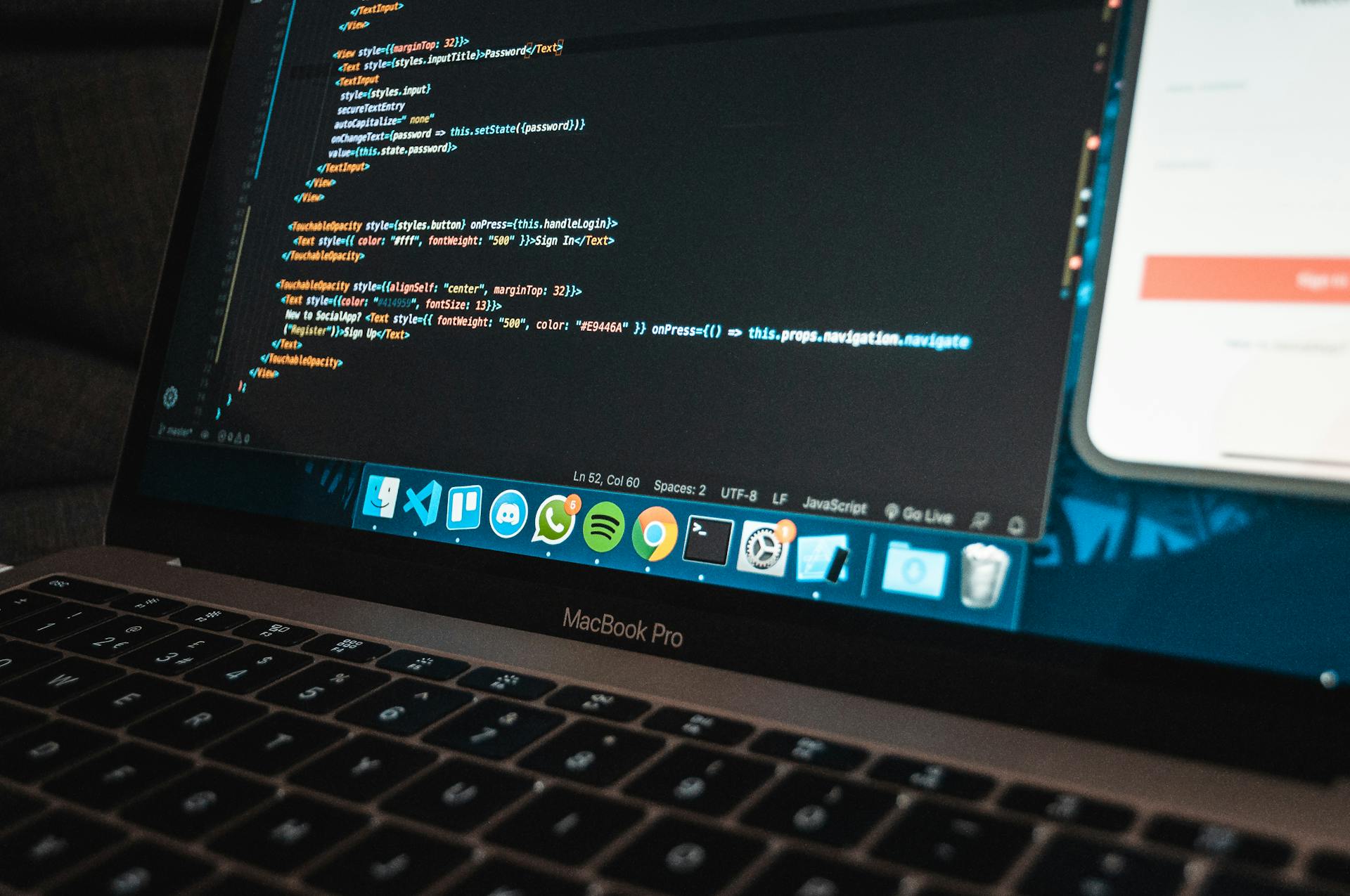
CS50's Web Programming with Python and JavaScript is a course that covers the basics of web development using these two popular programming languages.
The course starts with the basics of HTML and CSS, teaching you how to build simple web pages. You'll learn how to use CSS to style your web pages and make them visually appealing.
As you progress through the course, you'll learn how to use Python to create dynamic web pages. You'll see how to use Flask, a popular Python web framework, to build web applications.
With CS50's Web Programming, you'll gain a solid understanding of how to build web applications using Python and JavaScript, from basic to advanced topics.
On a similar theme: Learning Web Programming
Introduction
CS50's Web Programming with Python and JavaScript is a comprehensive training course that covers a wide range of topics. You'll learn how to build web applications from scratch.
HTML and CSS are the building blocks of the web, and you'll learn about these fundamental technologies. Git is also covered, which is essential for managing code and collaborating with others.
Discover more: Web Programming Tutorial
The course dives into Python programming, which is a popular language for web development. You'll also learn about Django, a high-level Python framework for building web applications.
Database management is another crucial aspect of web development, and you'll learn about SQL, Models, and Migrations. JavaScript is also covered, which is used for creating interactive web pages.
User Interfaces are a critical part of web development, and you'll learn how to create intuitive and user-friendly interfaces. Testing, CI/CD, and Scalability and Security are also covered, which are essential for building robust and maintainable web applications.
Here's a summary of what you'll learn in the course:
- HTML and CSS
- Git
- Python and Django
- SQL, Models, and Migrations
- JavaScript
- User Interfaces
- Testing, CI/CD, and Scalability and Security
Core Concepts
In CS50's Web Programming with Python and JavaScript, you'll learn the core concepts that power the web.
You'll start by learning about HTML, the standard markup language used to create web pages.
A website is made up of multiple files, each with its own unique role, such as index.html, which is the main entry point for users.
The browser renders HTML as a web page, using a process called parsing, which involves breaking down the code into its constituent parts.
CSS, or Cascading Style Sheets, is used to add visual styling to a web page, including layout, color, and font.
A CSS selector is used to target specific elements on a web page, allowing you to apply styles to just the right parts.
JavaScript is a programming language used to add interactivity to a web page, making it more dynamic and engaging.
You'll learn about the Document Object Model, or DOM, which is a tree-like structure that represents the web page, allowing you to access and manipulate its elements.
Variables are a fundamental concept in programming, used to store and reuse values, and you'll learn how to declare and use variables in Python and JavaScript.
Functions are reusable blocks of code that perform a specific task, and you'll learn how to define and call functions in Python and JavaScript.
Server-side and client-side are two important concepts in web development, with server-side referring to the code that runs on the server and client-side referring to the code that runs on the user's browser.
Suggestion: Open Browser Console Chrome
JavaScript Fundamentals
JavaScript is a powerful scripting language that allows you to declare variables to store and manipulate data, including strings, numbers, and objects. This enables you to create dynamic web pages.
Functions are a fundamental concept in JavaScript, allowing you to define and call upon blocks of reusable code to perform specific tasks. This makes your code more efficient and easier to maintain.
JavaScript also provides various data types, including strings, numbers, and objects, which can be used to store and manipulate data. These data types are essential for creating interactive web applications.
Here are some key concepts in JavaScript:
- Variables and Data Types: JavaScript allows you to declare variables to store and manipulate data.
- Functions: Functions are blocks of reusable code that can be defined and called upon to perform specific tasks.
- Conditional Statements: JavaScript provides if statements, switch statements, and other conditional constructs to control the flow of your code.
- Loops: Loops like for and while enable you to repeat actions or processes, making it easier to work with arrays, lists, and other data structures.
- DOM Manipulation: The Document Object Model (DOM) represents the structure of an HTML document as a tree of objects, and JavaScript can be used to interact with and manipulate this tree.
Arrow Functions
Arrow functions are a cleaner way to define functions in JavaScript, allowing them to be defined in just a single line of code.
This shorthand syntax is achieved by using an arrow (=>) between the input to a function and the block of code to be executed.
JavaScript's syntax for functions can be a little less intuitive, but arrow notation makes it more readable.
Intriguing read: Webflow Code Embed with Css
Start With a Strong Foundation
Before diving into the world of JavaScript, it's essential to have a solid grasp of HTML and CSS basics. Review any prerequisite materials or courses recommended by experts to ensure you're well-prepared.
HTML, CSS, and JavaScript are the building blocks of the web, and understanding their basics is crucial for success. Start with the fundamentals of HTML, such as structuring content with tags and attributes.
A strong foundation in CSS is also vital, as it allows you to control the layout, appearance, and behavior of web pages. Practice writing CSS code to style HTML elements.
Reviewing prerequisite materials or courses can save you time and frustration in the long run. The CS50 team recommends reviewing these materials before diving into more advanced topics, such as JavaScript.
Web Development
Web development involves a lot of behind-the-scenes work to create a secure and functional website.
Regular code reviews and security testing are crucial to identify and address potential weaknesses in your application. This includes vulnerability scanning and penetration testing to ensure your website is secure.
Keeping all software components, including libraries and frameworks, up to date is essential to patch known security vulnerabilities.
Recommended read: Create Responsive Website Tutorial
HTML and CSS Basics
HTML is the backbone of every web page, used to create the structure and content of a website.
HTML stands for Hypertext Markup Language, a technology that's been around since the early days of the internet.
Understanding the basics of HTML is crucial for building a website, as it allows you to create the fundamental elements of a web page, such as headings, paragraphs, and links.
HTML is not used for styling, but rather for defining the content and structure of a web page.
CSS, on the other hand, is used for styling and layout, making it easier to create visually appealing and user-friendly websites.
CSS stands for Cascading Style Sheets, a technology that's been around since the mid-1990s.
CSS allows you to separate the presentation of a web page from its content, making it easier to update and maintain a website.
These two technologies, HTML and CSS, are the fundamental building blocks of web development, and are used together to create the vast majority of websites on the internet.
You might enjoy: Building a Web Scraper in Python
Web Apps & Databases
Web applications and databases go hand in hand. Web applications often rely on databases to store user data, content, and other information.
To create feature-rich and data-driven web applications, understanding how to work with databases and SQL is fundamental. This is because databases are organized collections of data that are designed to be easily accessed, managed, and updated.
Relational databases like MySQL, PostgreSQL, and SQLite are among the most commonly used in web development. These databases are the repositories for information used by web applications, from user profiles and product listings to messages and transactions.
Databases store user data, content, and other information as users interact with web applications, such as when they register on a website, post a comment, or make an online purchase.
Approach
The CS50W course is a great example of an approachable way to learn web development. It involves about 8 hours of study per week over 12 weeks.
The course is taught by Brian Yu, who brings a wealth of knowledge to the table. He covers languages such as Python and JavaScript, frameworks such as Flask and Django, and services like GitHub and Heroku.
You can expect to learn a lot in a relatively short amount of time, which is perfect for those who want to get started quickly. The course is part of edX’s Professional Certificate in Computer Science for Web Programming.
Discover more: Webflow Crash Course
Security
Web security is a top concern in the digital age, as the internet is a prime target for malicious actors seeking to exploit vulnerabilities in web applications.
Authentication and Authorization are key security concepts that verify the identity of users and determine what actions they can perform within the application. This includes using mechanisms like username and password, OAuth, and authorization methods to control user access.
Input Validation is crucial to prevent common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). By validating and sanitizing user inputs, you can safeguard your application and its users from a range of threats.
Regular code reviews can help identify and address security vulnerabilities in your codebase. This involves following coding best practices that minimize security risks and keeping all software components, including libraries and frameworks, up to date to patch known security vulnerabilities.
Implementing robust error handling is essential to prevent exposing sensitive information in error messages. This includes keeping all software components up to date and regularly reviewing your codebase for security vulnerabilities.
Worth a look: What Is Web Authoring Software
Working with Databases
Working with databases is a crucial aspect of web development, and it's essential to understand how to work with databases and SQL to create feature-rich and data-driven web applications.
Databases are organized collections of data that are designed to be easily accessed, managed, and updated. They are the repositories for information used by web applications, from user profiles and product listings to messages and transactions.
Relational databases like MySQL, PostgreSQL, and SQLite are among the most commonly used in web development.
Here's an interesting read: Outline of Web Design and Web Development
To ensure the security of your web application, it's vital to understand how to work with databases and SQL. This includes designing the database schema and implementing database interactions to store and manage data.
Here are some key considerations when working with databases and SQL:
- Relational databases like MySQL, PostgreSQL, and SQLite are commonly used in web development.
- Databases come in various types, with relational databases being among the most commonly used.
- Understanding how to work with databases and SQL is fundamental to creating feature-rich and data-driven web applications.
By following best practices and understanding how to work with databases and SQL, you can create a secure and reliable web application that meets the needs of your users.
Web Security
Web security is a top concern in the digital age, as the internet is a prime target for malicious actors seeking to exploit vulnerabilities in web applications.
Implementing best security practices can safeguard your application and its users from a range of threats, including data breaches, unauthorized access, and malicious attacks.
Authentication and authorization mechanisms, such as username and password, OAuth, and proper user permissions, are essential for verifying the identity of users and determining what actions they can perform within the application.
Input validation and sanitization of user inputs can prevent common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Encryption protocols like HTTPS protect data transmitted between the user's browser and the server, ensuring that sensitive information remains confidential.
Regularly reviewing your codebase for security vulnerabilities and following coding best practices can minimize security risks.
Performing security testing, including vulnerability scanning and penetration testing, can identify and address potential weaknesses in your application.
Keeping all software components, including libraries and frameworks, up to date can patch known security vulnerabilities.
Developing an incident response plan can address security breaches swiftly and effectively.
Web security should be a top priority, and implementing security measures from the beginning can prevent vulnerabilities and keep your application and users safe.
Project Development
Project development is a crucial step in building a web application. Start by defining the scope and purpose of your project, including the problem it solves and its target audience.
Create a project plan that outlines features, functionalities, and design considerations to guide your development process. This will help you stay organized and focused throughout the project.
To build a visually appealing and interactive user interface, utilize HTML, CSS, and JavaScript for front-end development.
Explore further: Best Programming Language for Web Development
Is CS50W Worth It?
CS50W is a comprehensive course that covers all the essential tools and concepts for building a strong foundation in web development.
The course lasts until December and can be completed in 2 to 3 months, depending on your pace and commitment.
You'll gain hands-on experience with front-end and back-end technologies, including HTML, CSS, JavaScript, and Python, Django framework.
The projects and assignments are designed to challenge and reinforce your learning, so you can apply what you've learned in real-world scenarios.
Taking CS50W can give you a solid foundation in web development, making it a worthwhile investment of your time.
A different take: Webflow Master Course
Challenges and Learnings
Developing a project can be a challenging but rewarding experience. One of the primary reasons for this is the introduction of new techniques and concepts, such as APIs, JSON, and JavaScript, which can be overwhelming, especially for those new to programming.
Learning a new language like JavaScript can be difficult, especially when it comes to sequencing event listeners, which can be nested within other event listeners. This can be frustrating, especially when dealing with opaque promises and the .then syntax.
Consider reading: When Communicating It's Important to
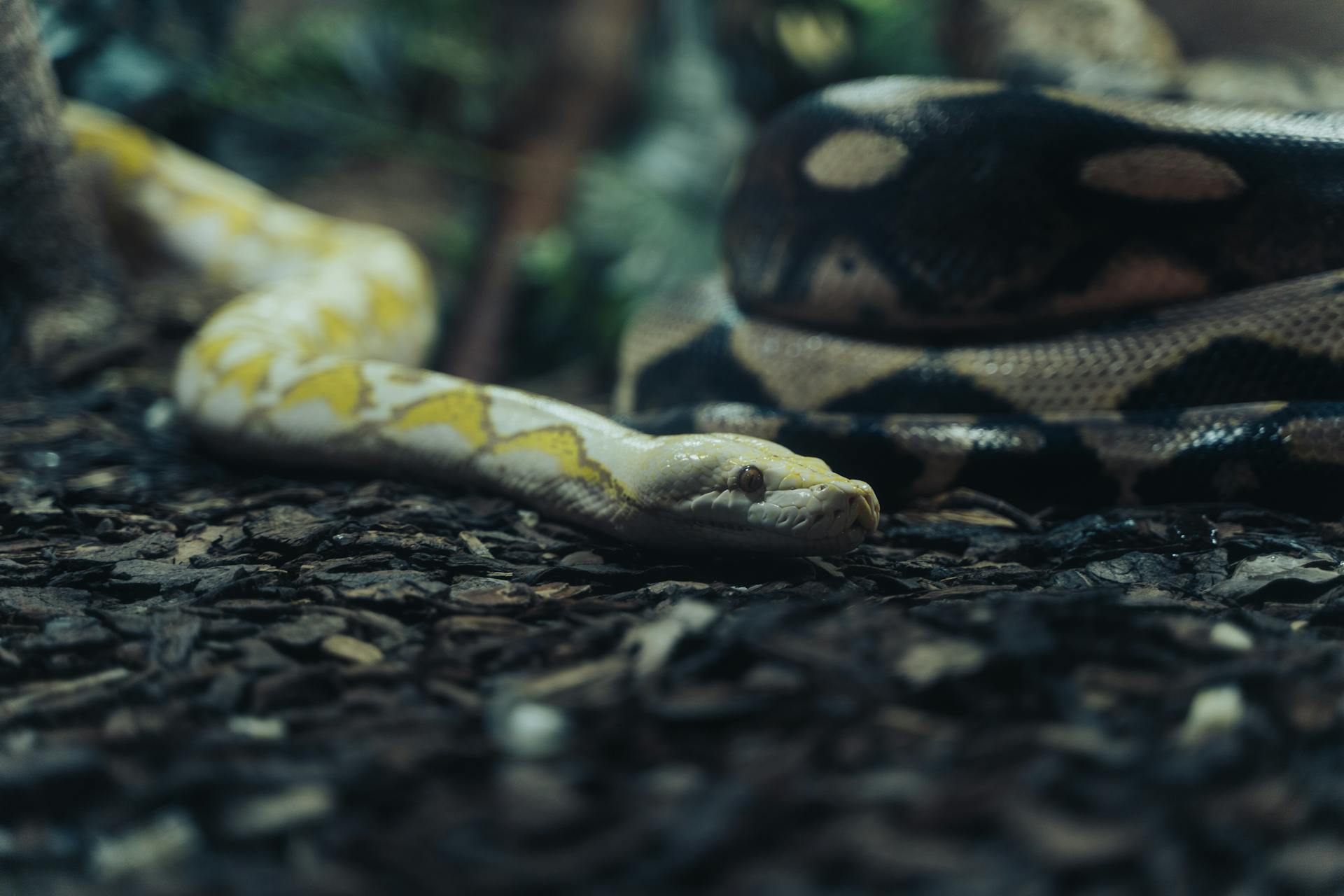
Getting a proper foothold on a project can be tough, especially when 90% of the app's functionality relies on having an email in the database. Creating this first email can present a steep learning curve, requiring many new concepts to be tied together and executed sequentially.
Working with code written by others can be a valuable learning experience, but it can also be frustrating, especially when it's not clear what the code is doing. This is why it's essential to read and understand the documentation, as seen in the example of the archive button issue.
Here are some key challenges and learnings from project development:
In the end, project development is a valuable learning experience that can further our understanding of many core concepts in web programming.
Key Steps in Building the Final Project
Project development involves several key steps to ensure a successful outcome.
Start by defining the scope and purpose of your project, including the problem it solves and the target audience. This will help guide your project plan.
Intriguing read: A Basic Html Project
Create a project plan outlining features, functionalities, and design considerations to keep you on track.
Design the user interface (UI) of your web application using HTML, CSS, and JavaScript for a visually appealing and interactive experience.
Implement the server-side logic of your application, using Python and Flask or another back-end framework as needed.
Design the database schema and implement database interactions to store and manage data securely.
Thoroughly test your application to identify and resolve bugs, ensuring it functions correctly and securely.
Deploy your web application to make it accessible on the internet, configuring web servers, databases, and security settings as needed.
Document your project, including code comments, user guides, and technical documentation, to help users and future developers understand and work with your application.
Present your final project to your peers and instructors, explaining your design choices, challenges faced, and lessons learned.
Tools and Practices
In CS50's web programming with Python and JavaScript, we learned to use a tool like Flask to create a web server in Python. This allowed us to serve HTML files and handle HTTP requests.
To create a simple web server, we used Flask's `flask.run()` method, which serves the HTML file at the specified address. This method is a great way to quickly test our web applications.
We also learned about the importance of using a version control system like Git to track changes to our code. This helps us keep a record of all the changes we make, making it easier to collaborate with others and debug our code.
You might like: Flask Web Dev
Introduction to Git
Git is a powerful tool for managing code and tracking changes in collaborative web development.
Version control is essential for keeping track of changes and managing code efficiently, as stated in the article. This allows multiple developers to work on a project simultaneously without conflicts.
Collaborative web development relies heavily on version control, which Git provides. By using Git, developers can work on the same project without overwriting each other's changes.
Multiple developers can work on a project simultaneously while keeping track of changes with Git. This makes it an ideal tool for collaborative web development.
Readers also liked: Embed a Pdf on My Websote Hmtl Code
Git enables developers to manage code efficiently by tracking changes and keeping a record of all modifications. This makes it easier to identify and resolve conflicts.
By using Git, developers can work on a project while others are also working on it, without any issues. This is a huge advantage in collaborative web development.
Practice Regularly
Regular practice is essential to improve your web development skills. It's a hands-on skill that requires you to apply what you've learned.
Coding and building web applications regularly helps reinforce your learning. You can do this by completing problem sets and assignments.
The more you practice, the more comfortable you'll become with coding languages and frameworks. This will enable you to tackle complex projects with confidence.
By regularly practicing web development, you'll be able to apply what you've learned and see tangible results. This will keep you motivated and engaged in the learning process.
A different take: Html Coding Practice
Libraries and Frameworks
Building interactive web pages can be a daunting task, but fortunately, there are many tools available to simplify the process.
One such tool is jQuery, a widely used JavaScript library that simplifies DOM manipulation and event handling.
JavaScript libraries like jQuery are incredibly powerful and can save developers a lot of time and effort.
Some popular JavaScript libraries and frameworks include:
- jQuery: A widely used JavaScript library that simplifies DOM manipulation and event handling.
- React: A JavaScript library for building user interfaces, particularly well-suited for single-page applications.
- Angular: A comprehensive JavaScript framework for building dynamic web applications.
- Vue.js: A progressive JavaScript framework for building interactive web interfaces incrementally.
These libraries and frameworks can be used to build a wide range of web applications, from simple websites to complex single-page applications.
Each of these libraries and frameworks has its own strengths and weaknesses, and the choice of which one to use will depend on the specific needs of the project.
Expand your knowledge: Web Programming Frameworks
Event Handling
Event Handling is a fundamental concept in web development, and it's what makes your website interactive. JavaScript is the language that makes it all possible.
JavaScript can listen for and respond to various events, such as clicks, mouse movements, and keyboard input. This allows you to create responsive interfaces that react to user interactions.
To detect events, you use event listeners, which quietly lie in wait for specific events to occur. When an event is triggered, the listener executes a function to respond to it.
You can use the JavaScript query selector function to select specific elements of a webpage from the Document Object Model (DOM). By storing the returned element in a variable, you can manipulate its contents.
Here are some common events that you can detect with JavaScript:
- Clicks
- Mouse movements
- Keyboard input
- Loading a page
By combining event detection with the ability to select and act upon DOM elements, you can create even more interactive websites.
Frequently Asked Questions
Is Python and JavaScript enough for web development?
While Python and JavaScript are essential for web development, they're not the only languages you'll need to master for a comprehensive understanding of web development. To fully develop web applications, you may also want to consider learning other languages like HTML/CSS and backend frameworks like Django or Ruby on Rails.
Is CS50 web enough to get a job?
While CS50 Web provides a solid foundation, it's just the starting point - you'll still need to build projects, network, and gain experience to be job-ready. Take the next step and apply your skills to land your dream tech job.
Does CS50 have JavaScript?
CS50 teaches C, Python, and JavaScript as part of its curriculum, making it a great starting point for self-taught developers. After CS50, you can dive deeper into JavaScript with The Odin Project's web development course.
Sources
- https://www.classcentral.com/course/web-development-harvard-university-cs50-s-web-pro-11506
- https://medium.com/@rpcoron/cs50s-web-programming-with-python-and-javascript-javascript-user-interfaces-and-mail-project-6823aa01fe84
- https://www.appypie.com/cs50-web-development
- https://bakhtiyarsagidanov.com/cs50w/
- https://www.careers360.com/university/harvard-university-cambridge/web-programming-python-and-javascript-certification-course
- https://www.classcentral.com/report/harvard-cs50-guide/
Featured Images: pexels.com