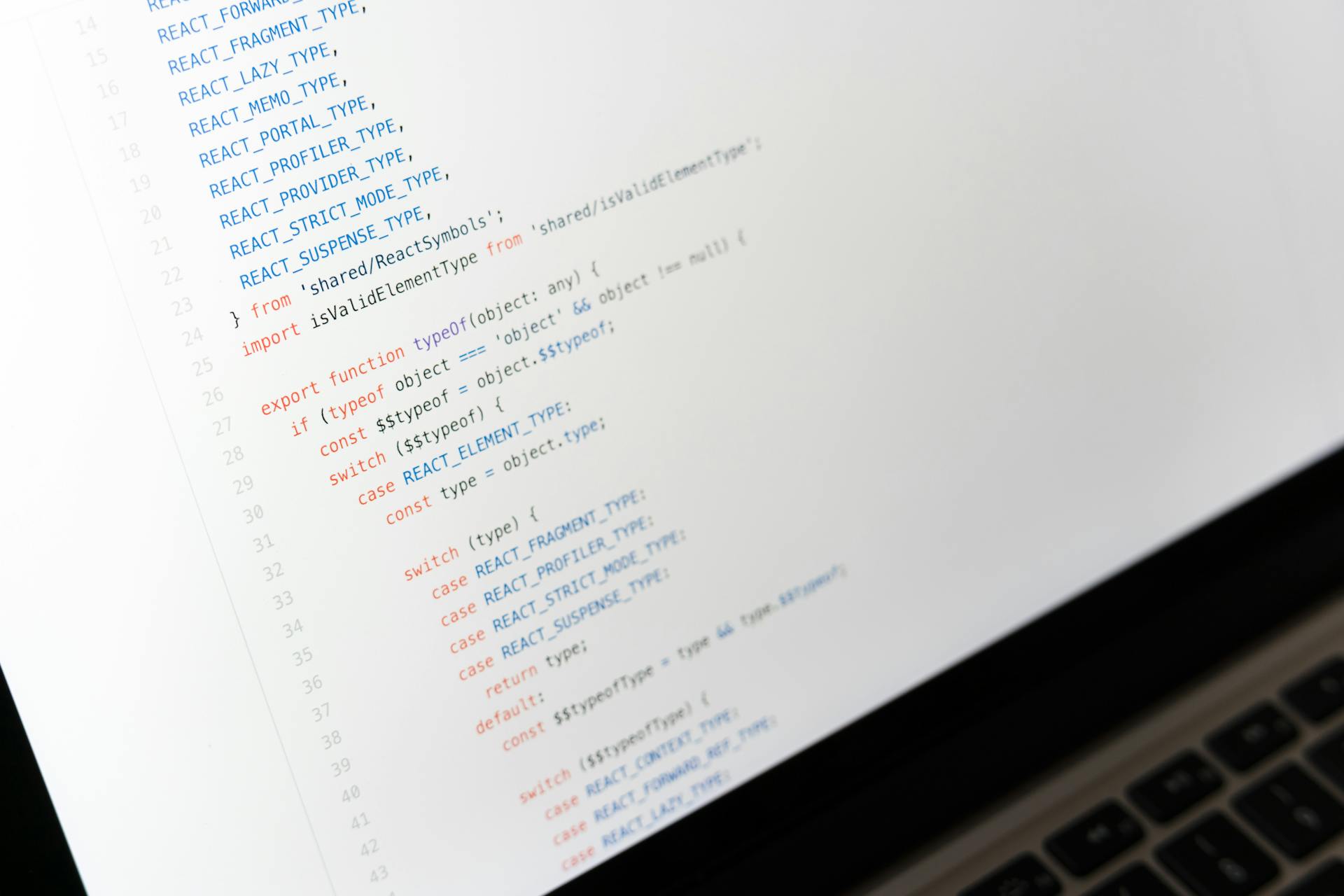
To get started with Electron Nextjs development, you'll need to create a new project using the Electron CLI, which can be done by running `npx create-electron-app my-app` in your terminal.
Nextjs is a popular React framework that allows you to build server-side rendered (SSR) and statically generated websites. This framework is particularly useful for building Electron desktop applications.
The `electron-builder` tool is used to package and distribute your Electron application. This tool allows you to create installers for Windows, macOS, and Linux.
Electron applications can be deployed to various platforms, including desktop, web, and mobile.
Related reading: Create Cookies Nextjs
Next.js Concepts
Next.js is an open-source React framework for production web applications that can supercharge Electron apps by introducing key innovations like server-side rendering and static site generation.
Server-side rendering and static site generation improve performance by generating first page HTML at build time rather than client-side, resulting in a faster initial load time.
Next.js capabilities like simplified routing, API endpoints, TypeScript support, and Sass compilation reduce boilerplate code in Electron apps, allowing developers to focus on building features rather than wiring up configurations.
Here are the key components of a Next.js Electron app:
Developers can leverage this boilerplate structure to build complex, engaging cross-platform Electron apps using the latest web technologies.
Pages
Pages in Next.js work exactly the same way as in a standard Next.js application, with no exception.
To create a new page, you can create a new file inside the renderer/pages directory and write the content you want. For example, you can create a new sample.js file with the following content.
You can also update the default home.jsx file in the renderer/pages directory to link to your new page instead. This is done by updating the file with the content below.
Pages are an essential part of any web application, and Next.js makes it easy to create and manage them.
Here are some key points to keep in mind when working with pages in Next.js:
- Create a new file inside the renderer/pages directory to create a new page.
- Update the default home.jsx file to link to your new page instead.
IPC
Inter-process communication (IPC) is a crucial aspect of Next.js development, especially when working with Electron applications.
IPC is used for communication between the main and renderer processes in Electron, and it's essential to sanitize and validate data carefully before sending it through IPC channels to prevent unauthorized access or code execution.
To expose a minimal API to the renderer process, you can't simply import the ipcRenderer object directly into your Next.js code. Instead, you need to create a bridge between the processes.
Here are the key things to keep in mind when working with IPC in Next.js:
- Sanitize and validate data carefully before sending it through IPC channels.
- Expose a minimal API to the renderer process, and avoid exposing the ipcRenderer object directly.
- Use a preload script to expose an ipc object to the renderer process, and define a concrete API to interact with the main process.
By following these best practices, you can ensure secure and efficient communication between your Next.js application and the main process.
React Framework Supercharging Apps
Next.js is an open-source React framework for production web applications that supercharges Electron apps. It introduced key innovations like server-side rendering and static site generation to improve performance.
NextJS boosts initial load time since first page HTML is generated at build time rather than client-side. This feature is especially useful for Electron apps.
One of the key benefits of using NextJS in Electron apps is that it simplifies routing, API endpoints, TypeScript support, Sass compilation, and more. This reduces boilerplate code in Electron apps, allowing developers to focus on building features rather than wiring up configurations.
The NextJS framework enhances developer experience by elegantly managing common complexity points in web projects. This enables engineers to build JavaScript desktop apps faster alongside Electron.
Here are some key NextJS features that supercharge Electron apps:
- Server-side rendering
- Static site generation
- Simplified routing
- API endpoints
- TypeScript support
- Sass compilation
Security and Best Practices
Security is a top priority when building Electron and Next.js applications. Enable Node.js integration selectively, as it's disabled by default in Electron for enhanced security.
To prevent malicious code injection, implement a robust Content Security Policy (CSP) to restrict what scripts, styles, and resources can be loaded within your application's renderer processes.
Context isolation is a security feature in Electron that creates a separate context for each renderer process, preventing cross-site scripting (XSS) attacks and other potential security vulnerabilities.
To prevent unauthorized access or code execution, sanitize and validate data carefully before sending it through IPC channels.
Secure HTTP headers like X-XSS-Protection, X-Frame-Options, and Content-Security-Policy can be implemented using Next.js middleware or custom server middleware.
Enforce HTTPS for all communication between your Next.js application and the server to encrypt data transmission and protect it from eavesdropping and tampering.
Server-side rendering (SSR) offers SEO benefits, but be mindful of potential XSS vulnerabilities if user-controlled data is directly rendered on the server. Sanitize all user input before including it in the SSR output.
Here are the security best practices summarized:
- Enable Node.js integration selectively
- Implement a robust Content Security Policy (CSP)
- Use context isolation in Electron
- Sanitize and validate data before sending it through IPC channels
- Implement secure HTTP headers
- Enforce HTTPS for all communication
- Sanitize user input in Server-Side Rendering (SSR)
Integrating Nextron
To integrate Nextron into an existing project, you need to add essential dependencies like electron, nextron and next.
The Dolt Workbench, a Next.js application, already runs in web browsers, so we can use Electron to develop a desktop version compatible across different operating systems.
In the root package.json, specify the main script location for Electron as app/background.js. This is necessary for Electron to run the app.
After installing dependencies, add commands to the scripts section of your package.json to enable development and build processes for the Electron app.
For development, set output to "standalone" to allow dynamic server-side rendering, which creates a minimal production build that includes only necessary files required to run the application.
In production, set output to export in the Next.js configuration to ensure files are static and optimally bundled for enhanced performance and stability.
Expand your knowledge: How to Run Nextjs to Build
Development and Deployment
Development and deployment is where the magic happens. Once your Electron Next.js app is ready, you can generate distributables for macOS, Windows, and Linux using Electron Builder.
Electron Builder packages the app into an installer for production, and you can configure builder options in electron-builder.yml like icons and metadata. This ensures a seamless user experience.
To package your application for distribution, Nextron provides a lightweight wrapper around other tools. It creates a static export of the frontend application, builds the main backend process, and then invokes electron-builder to package everything up into a set of distributable artifacts.
After running the build process, you'll end up with a bunch of assets in the dist directory, including a binary that you can run to test your application. This is a crucial step in ensuring everything works as expected.
To keep your app up-to-date, you can configure auto-updating to ship updates directly to users. This removes the need to manually distribute new versions. Here are some best practices for auto-updating:
- Use semantic versioning for smooth upgrades
- Notify users of changes in update logs
- Test autoupdating early in development
Developing Your App
You can start building your app's features by editing the source code in the boilerplate structure. The main process code is executed in the main Electron process, located at ./src/main/main.dev.ts, while the entry point for the React renderer process is at ./src/renderer/index.tsx.
Curious to learn more? Check out: Src Directory Nextjs
To add a new page, create a new React component in ./src/renderer/screens, add a route for it in ./src/renderer/router.tsx, and import and render the page component. This leverages the boilerplate structure to build complex, engaging cross-platform Electron apps using latest web technologies.
You can access Node.js API in the Main process through ./src/main, and shared code can be placed in ./src/shared. This allows for a clean separation of concerns and makes it easier to maintain and update your app.
Here's a step-by-step guide to adding a new page:
- Create a new React component in ./src/renderer/screens
- Add a route for it in ./src/renderer/router.tsx
- Import and render the page component
This will help you build a robust and scalable app that meets your users' needs.
APIs
APIs are essential for building robust and scalable applications, and in the context of Electron development, they play a crucial role in facilitating communication between the main and renderer processes.
To expose a minimal API to the renderer process, you can't simply import the ipcRenderer object directly, as it relies on privileged filesystem access which the renderer is not granted.
Setting up this bridge between processes can be one of the less intuitive aspects of Electron development, but fortunately, Nextron comes with a basic preload script that exposes an ipc object to the renderer process.
However, it's recommended to replace this script with your own, defining a more concrete API that doesn't expose the ipcRenderer object directly or run business logic.
In Nextron, you'll notice that the preload script is a development dependency, denoted by --save-dev, which allows you to keep track of code and assets needed for the renderer process.
When building your application for distribution, keep in mind that anything you need in the main process must be installed as a regular dependency.
To implement the API, you can keep all business logic in one file, such as main/handlers.ts, which is a good practice for maintainable applications.
In this file, you can define API calls like select-repositories, which returns a subset of each repository's properties, and consider what data to return from each API call to avoid unnecessary data transfer.
Take a look at this: Nextjs Development Company
Deploying the Final App
You can generate distributables for macOS, Windows, and Linux using Electron Builder.
Electron Builder will package your app into an installer for production, making it ready for users to download and install.
Configure builder options in electron-builder.yml to customize the look and feel of your app, including icons and metadata.
Publish updates using auto-update for seamless delivery, ensuring users always have the latest version of your app.
Configure auto-update in the Main process code to automate the update process and keep your users up-to-date.
If this caught your attention, see: Next Js Using State Context
Project Structure and Tools
The project structure of an Electron Next.js app is straightforward, with a clear separation of Electron and React code into distinct folders. The Electron code is organized in the `src-electron` folder, while the React code is in `src-react`.
The React code is further organized by feature, following a domain-driven design, with UI components related to the application sidebar located under `src-react/ui/Sidebar`. This modular organization makes it easier to customize one layer without impacting the other.
The project uses Webpack for bundling, along with Babel, PostCSS, and other compilers, with shared webpack config in `webpack.common.js`. Additional dependencies can be installed through npm or yarn, which get saved to `package.json`.
Curious to learn more? Check out: Shadcn Ui Nextjs
Layouts
Layouts in Nextron work just like in a regular Next.js application. No special rules apply here.
To create a shared layout across multiple pages, start by creating a new layout file in the /renderer directory. For example, let's call it layout.js.
Layouts need to be created manually, unlike in a regular Next.js application where the _app.js file is automatically included. You'll need to create this file just outside the pages folder.
With a little CSS, you can style your navbar and pages to get the desired results.
Worth a look: Nextjs Server Actions File Upload
Configuration and Dependency Management
electron-react-boilerplate uses Webpack for bundling, along with Babel, PostCSS, and other compilers.
The webpack.common.js file contains shared webpack config used across development and production. This allows for consistent bundling across different environments.
Additional dependencies can be installed normally through npm or yarn, which get saved to package.json. This makes it easy to add new libraries or tools to your project.
For customizing the bundler output, tweaking Babel transforms, or adding more PostCSS plugins, modify the respective config files in config/. This flexibility allows you to adapt the build setup to your specific needs.
Related reading: Next Js Webpack Config
Project Layout
The project layout is organized into separate folders for Electron and React code. This is done to keep the codebase clean and maintainable.
The Electron code is located in the `src-electron` folder, which serves as the entry point for the main process. The `src-electron/main.js` file launches the app window.
In contrast, the React UI code is organized by feature following a domain-driven design. This means that all UI components related to a specific feature, such as the application sidebar, are located in a dedicated folder.
For example, all UI components related to the sidebar are located under `src-react/ui/Sidebar`. This promotes a clear separation of concerns and makes it easier to customize one layer of the codebase without impacting the others.
You might enjoy: What Is .next Folder in Next Js
Dev Tools
Dev Tools are essential for debugging and identifying issues in your project. You can view client-side logs in the main window by opening the developer tools once the page has loaded.
To debug the GraphQL server process, you can send stdout and stderr logs to the main browser window. This helped one developer identify why the GraphQL server was exiting after the app started.
The issue was that the schema.gql file was being modified during the GraphQL server's runtime, but the Electron app packs all resources in a read-only .asar file. This prevents the file from being written to.
To fix this, you need to write the schema.gql file to the Application Support folder instead. This can be done by conditionally setting the schema file path in the GraphQL server configuration.
For another approach, see: File Upload Next Js Supabase
Exploring Github
GitHub hosts thousands of open-source Electron and NextJS boilerplates to choose from.
Developers can browse these boilerplates by various filters such as stars, forks, keywords, and topics to find well-tested templates aligned to their needs.
Some top Electron boilerplate GitHub repos include:
- electron-react-boilerplate: A popular boilerplate with React and Redux configured out the box. Over 18k stars.
- electron-next-boilerplate: Combines Electron with NextJS for SSR React desktop apps. 100+ stars.
- electron-forge-react-boilerplate: Scaffolded using Electron Forge and TypeScript. 600+ stars.
The open-source nature of these boilerplates allows developers to browse source code before using, check coding styles, fork as a starting point, submit PRs, and get involved.
On a similar theme: Nextjs Open Source Personal Projects
Boilerplates and Ecosystem
Boilerplates provide developers with pre-configured project templates to accelerate building desktop applications using web technologies.
By using boilerplates, developers can skip repetitive project initialization tasks and directly focus on writing application logic. This is especially true for Electron and NextJS boilerplates, which simplify project kickoffs.
These pre-configured project templates come with folder structures, workflows, configs, dependencies, and code samples already set up according to best practices. This allows developers to build high-performance desktop apps leveraging the power of React and Node.js.
Electron and NextJS boilerplates make it easier to develop cross-platform desktop apps using JavaScript, HTML, and CSS. By combining these two technologies, developers can quickly build rich applications using web technologies.
Pairing Electron and NextJS provides a solid foundation for developing desktop apps with React's component model.
See what others are reading: Next Js React Fundamentals
Frequently Asked Questions
What is the difference between Electron and NextJS?
Electron is a framework for building desktop applications with system-level access, whereas Next.js is a framework for building server-rendered web applications with optimized performance. While Electron has a larger footprint, Next.js is lightweight and ideal for web development.
Sources
- https://blog.logrocket.com/building-app-next-js-electron/
- https://stronglytyped.uk/articles/building-publishing-desktop-applications-electron-nextjs
- https://nextjsstarter.com/blog/electron-nextjs-boilerplate-a-developers-guide-to-efficiency/
- https://tuliocalil.com/desktop-apps-with-electron-react-and-sqlite/
- https://dolthub.com/blog/2024-09-11-building-an-electron-app-with-nextjs/
Featured Images: pexels.com