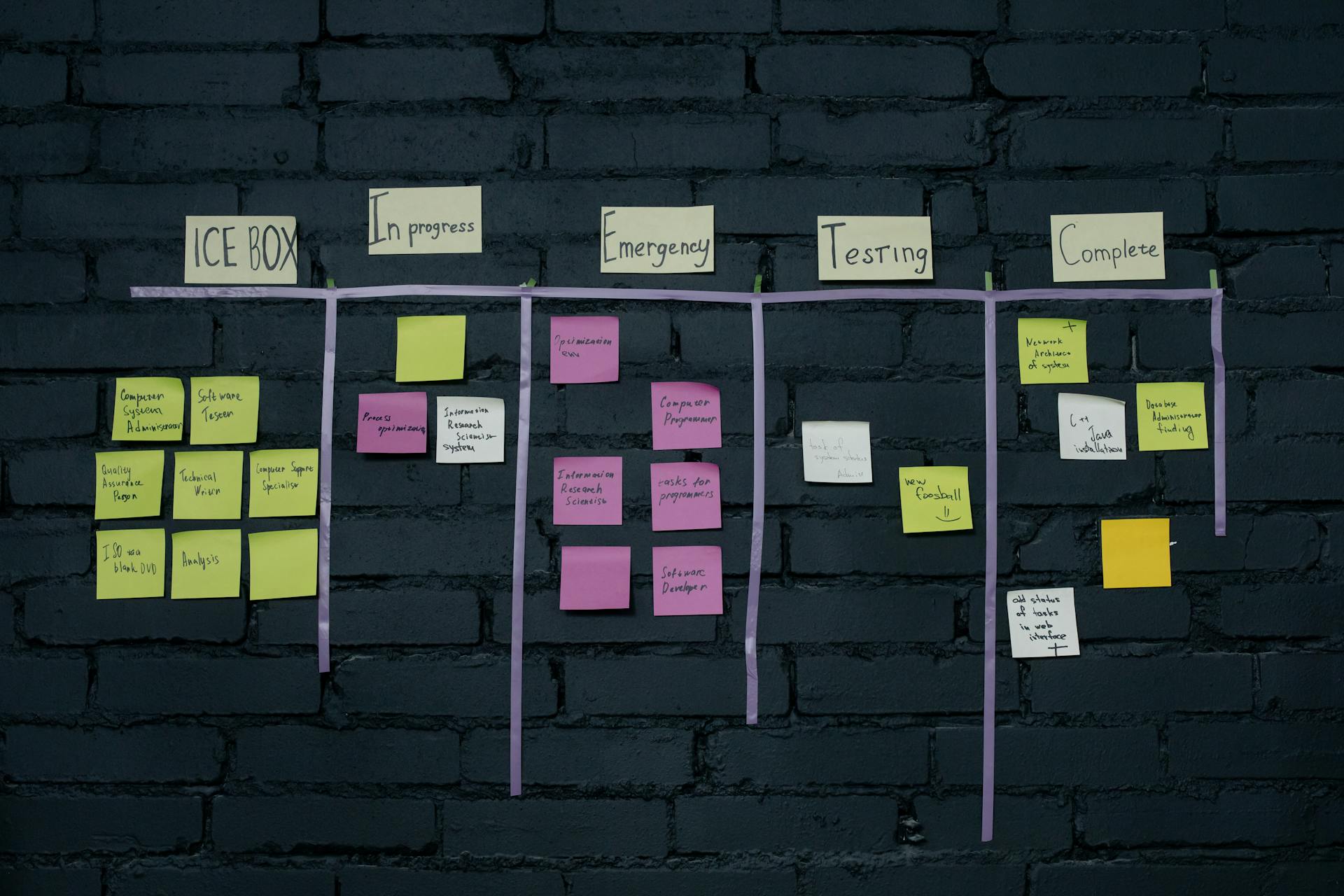
A Kanban board is a visual representation of your workflow, and Nextjs is a React-based framework for building server-rendered and statically generated applications.
To create a Kanban board with Nextjs, you'll need to set up a project with the Nextjs CLI, which can be done by running `npx create-next-app my-kanban-board`.
Nextjs provides a built-in API route feature that allows you to create RESTful APIs with ease. This is particularly useful for creating a Kanban board, as it enables you to interact with your board's data in a flexible and scalable way.
The Nextjs API route feature is enabled by default in new Nextjs projects, so you won't need to do any additional configuration to get started.
Setup and Configuration
To set up a Kanban board in Next.js, you'll need to add a resetServerContext() function in the getServerSideProps method where you're using drag and drop functionality. This ensures the server-side context is reset before each request, preventing conflicts or inconsistencies related to server-side rendering.
The layout setup is crucial for your Kanban board. You'll want to add a base layout to your pages/index.tsx file, which will display the basic structure of your board.
To keep track of your containers and their items, you'll need to define some states. You'll have the following states: containers, activeId, currentContainerId, itemName, containerName, showAddContainerModal, and showAddItemModal. These states will help you manage the drag and drop functionality and render the modal for adding containers and items.
Here's a list of states you'll need to define:
- containers: stores all the containers with items
- activeId: tracks the current selected or dragging element Id
- currentContainerId: used when adding a new item to a container
- itemName: used in the controlled input for item name
- containerName: used in the controlled input for container name
- showAddContainerModal: renders the modal for adding a container
- showAddItemModal: renders the modal for adding an item
Setup
Let's dive into the setup process for our Kanban board. First, we need to set up our base layout, which will serve as the foundation for our board. This involves adding our base layout to the pages/index.tsx file.
To get started, we need to organize our files in a logical structure. The folder structure should include a useHandleTicketsState folder for managing state, a domain folder for data and types, and separate folders for useDrop and useDrag.
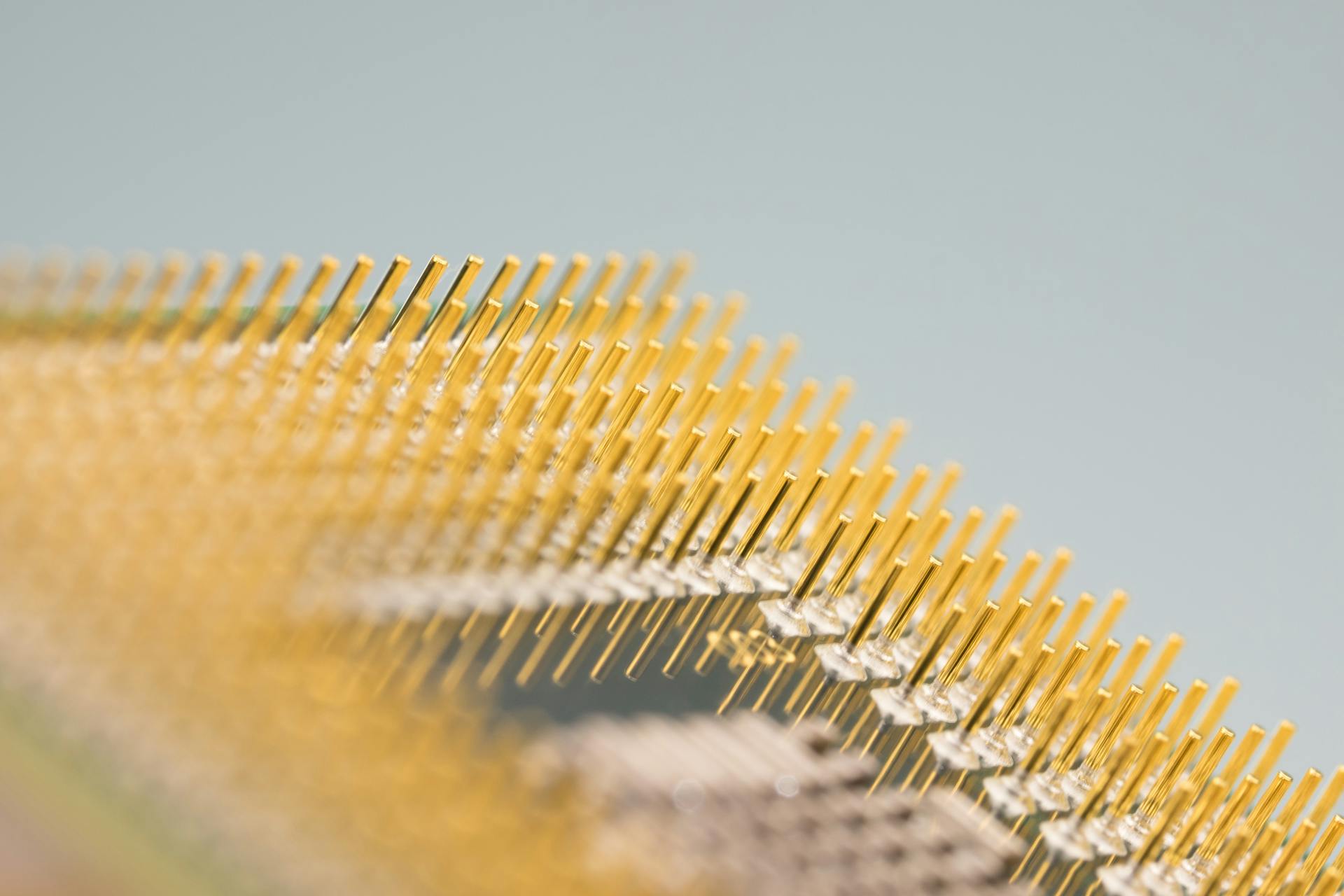
Next, we need to set up our state and types. This involves defining the type for our containers, which will hold all the items on the Kanban board. We also need to define the React states required for the app, including containers, activeId, currentContainerId, itemName, containerName, showAddContainerModal, and showAddItemModal.
Here's a summary of the states we need to define:
- containers - to keep track of all containers with items inside
- activeId - to track the current selected or dragging element Id
- currentContainerId - to use when adding a new item to a container
- itemName & containerName - for controlled Input to get the name of the container and item
- showAddContainerModal & showAddItemModal - to render the modal for container and item
These states will help us manage the data and functionality of our Kanban board. By setting up our base layout, folder structure, and state and types, we're laying the groundwork for a solid and efficient Kanban board setup.
Some Configuration
If you're using Next.js, you'll want to add `resetServerContext()` in the `getServerSideProps` where you're using the drag and drop functionality. This ensures that the server-side context is reset before each request, preventing potential conflicts or inconsistencies related to server-side rendering.
Some configurations are more important than others, but this one is crucial for drag-and-drop functionality. It's like hitting the reset button on your computer - it clears everything out and starts fresh.
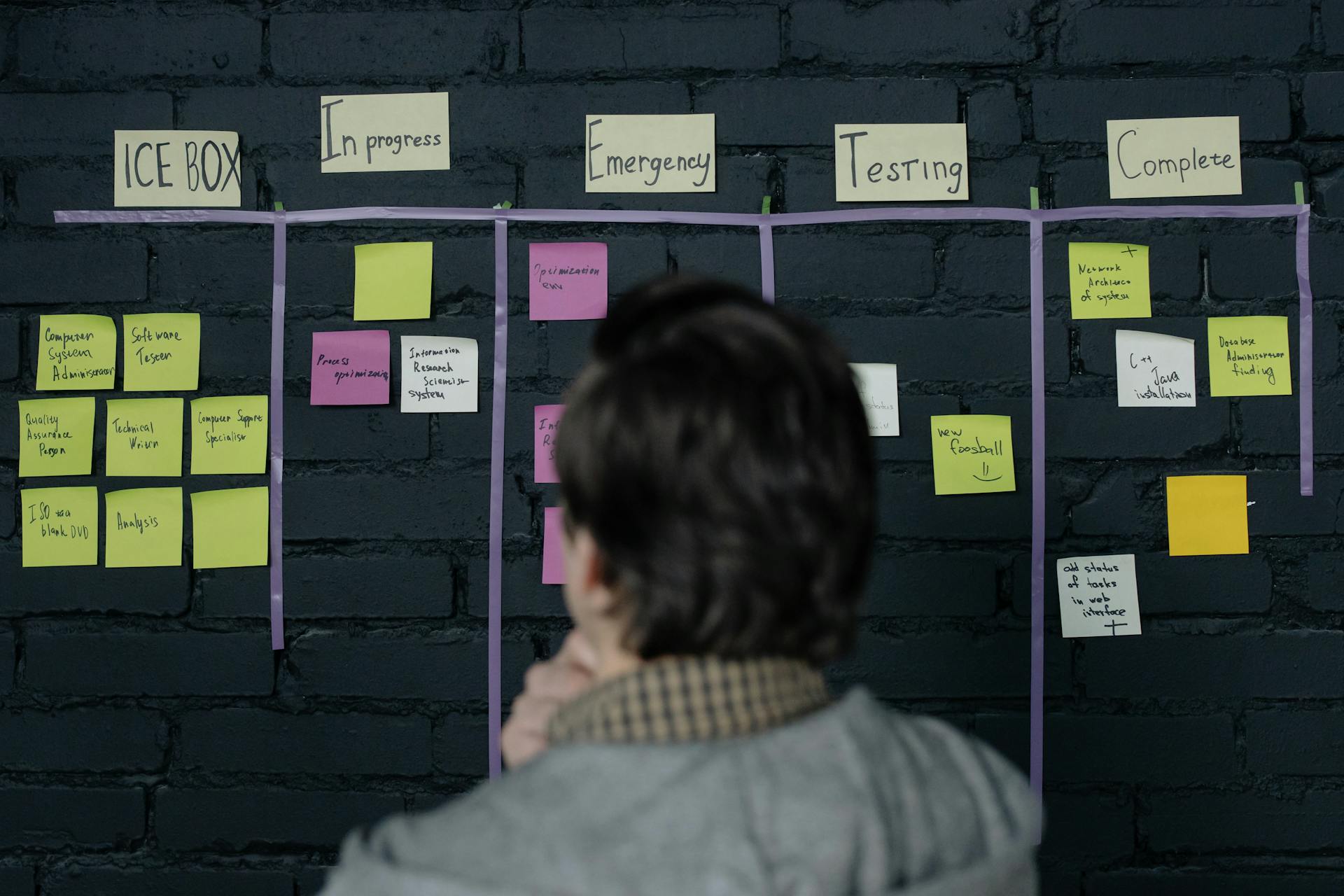
To store the order of columns, you'll need an array that updates whenever the order changes. This array will store the column identifiers, like this: `const columnOrder = ["column-1", "column-2", "column-3"];`.
Here's a quick rundown of what this array will do:
- Store the order of columns
- Update whenever the order changes
- Example: `const columnOrder = ["column-1", "column-2", "column-3"];`
Github Repository:
When working with Next.js, it's essential to explore the available repositories to find the right tools for your project.
The Next.js Kanban repository is a great place to start. It's located at Github Repository: nextjs-kanban.
The latest commit to this repository is schema update: Allow cover images for tasksView. This update shows that the developers are actively working on improving the functionality of the Kanban board.
Kanban Board Features
You can create boards with customizable columns and cards to manage tasks efficiently using the Kanban Board feature. This makes it easy to visualize and organize your work.
The Kanban Board allows you to tailor the columns and cards to fit your specific needs, giving you a flexible and effective way to manage your tasks.
Here are some key features of the Kanban Board:
- Customizable columns
- Customizable cards
These features make it easy to create a board that suits your workflow and helps you stay organized and focused.
Features
So you're looking to manage tasks efficiently with a Kanban board? One of the key features is the ability to create boards with customizable columns and cards.
You can also manage user access with user authentication, which allows users to create accounts and log in using Google, GitHub, or email (magic link).
The board and user data is stored securely in a cloud-hosted database on PlanetScale.
Automated testing and deployment are integrated with GitHub Actions for continuous integration.
The platform also includes automated tests, such as unit tests, Playwright tests for API and UI, and Chromatic tests for visual regression.
Documentation is made easy with Storybook, which creates interactive component documentation for easy reference.
Here are the features in more detail:
- Kanban Board: Create boards with customizable columns and cards to manage tasks efficiently.
- User Authentication: Users can create accounts and log in using Google, GitHub, or email (magic link).
- Cloud Database: Utilizes a cloud-hosted database on PlanetScale to store board and user data securely.
- Continuous Integration: Integrates with GitHub Actions for automated testing and deployment.
- Automated Tests: Includes unit tests, Playwright tests for API and UI, and Chromatic tests for visual regression.
- Documentation: Utilizes Storybook to create interactive component documentation for easy reference.
Item
Implementing the DndContext handlers is a crucial step in bringing your kanban board to life. This involves creating a ref for the drag element, which allows ReactDND to track its position and other attributes.
The item property in the useDrag hook is where you specify the data that will be exchanged between the dragged component and where it's dropped. This data will "fly around" with the dragged component until it's dropped.
The type property is a string that links the Draggable component to where it can be dropped. If you put "ticket" in the useDrag hook and also put "ticket" in the useDrop hook in the Column component, it means you can drop the Ticket on the Column.
The drag ref is a reference to the element that will be dragged around the board, allowing ReactDND to track its position and other attributes.
Sources
- https://hedighodhbane.hashnode.dev/react-drag-and-drop-kanban-board
- https://github.com/jonaszb/kanban-next
- https://abhiiishek07.medium.com/kanban-board-multi-list-drag-and-drop-with-react-beautiful-dnd-in-next-js-12b73c32e938
- https://cwaitt.dev/projects/kanban
- https://blog.chetanverma.com/how-to-create-an-awesome-kanban-board-using-dnd-kit
Featured Images: pexels.com