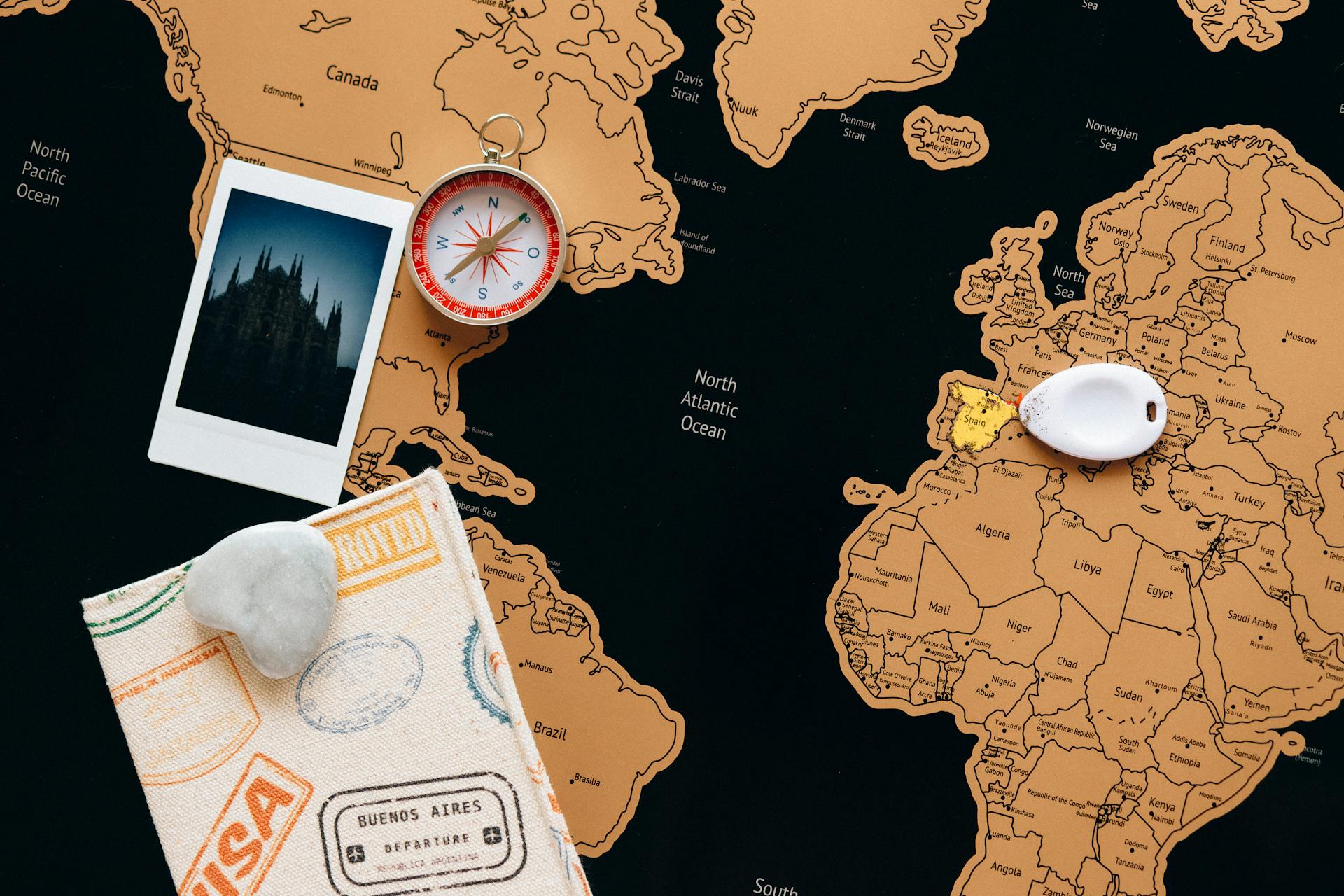
Building interactive maps with Mapbox and Next.js is a powerful combination. Mapbox provides a robust set of tools for creating custom maps, while Next.js streamlines the development process with its server-side rendering and static site generation capabilities.
With Mapbox, you can create custom maps that are tailored to your specific needs, from adding markers and pop-ups to integrating with third-party services. This flexibility is especially useful for applications that require a high degree of customization.
Mapbox's API provides a wide range of features, including support for multiple map styles, 3D rendering, and real-time updates. This means you can create maps that are not only visually appealing but also highly functional.
By integrating Mapbox with Next.js, you can leverage the strengths of both technologies to create highly interactive and engaging maps that are also fast and efficient.
Setting Up
To set up a MapBox map in your Next.js project, you'll first need to create a new project with a TypeScript template. This involves opening the project folder and installing MapBox GL with TypeScript types.
Next, you'll need to create a .env.local file to store your MapBox access token securely. This will prevent you from hardcoding it directly into your application code.
The components/mapbox-map.tsx file is where you'll initialize the MapBox map. You can find the parameters for initialization in the MapBox documentation.
To view the map, import it into your main application page, specifically pages/index.tsx. After running the project, you can access the map by visiting http://localhost:3000, where you'll see a full-screen web map.
To render a MapBox map in your Next.js project, you'll need to add the react-map-gl library, which is a suite of React components for MapBox. However, this library requires the global window object to work correctly, which can be a challenge when using server-side rendering in Next.js.
Improving the Component
To improve the map component, we need to add some essential features. We can start by adding props for the MapboxMap component, which will allow us to pass initial map options and a callback for the map load event.
The props we'll add are: initialOptions, onCreated, onLoaded, and onRemoved. The initialOptions prop will exclude the container property of the MapboxOptions interface using the Omit utility type. We'll pass initialOptions to the web map init options using spread syntax and set a callback for the map load event.
Here's a summary of the new props and their purposes:
- initialOptions: parameters for initializing the map, excluding the container property
- onCreated: callback triggered by the creation of the map instance
- onLoaded: callback triggered by the full loading of the map
- onRemoved: callback triggered by the removal of the map instance
By adding these props, we can override the initial map properties and use the onLoaded callback when the map is loaded. This will allow us to store a link to the map instance in the parent component, or use the onRemoved callback to know when the map instance has been removed.
Improving Component
To improve the MapboxMap component, we need to add props for the web map init options using the utility type Omit to exclude the container property of the MapboxOptions interface.
The initialOptions prop should be passed to the web map init options using spread syntax, and we should also set a callback for the map load event.
According to the react-hooks/exhaustive-deps rule, we should specify in the list of dependencies for React.useEffect variables added to the hook, but in this case, it's better to leave the dependency list empty to prevent re-creating the map instance if initialOptions or onMapLoaded changes.
The final component version will look like this, allowing us to override the initial map properties and use the onMapLoaded callback when it's loaded.
We can also use onMapLoaded to store a link to the map instance in the parent component, and onMapRemoved if we need to know that the map instance has been removed.
Here are the used props for the improved component:
- initialOptions - parameters for initializing the map, excluding the container property of the MapboxOptions interface
- onCreated - callback triggered by the creation of the map instance
- onLoaded - callback triggered by the full loading of the map
- onRemoved - callback triggered by the removal of the map instance
By using these props, we can enhance the functionality of the MapboxMap component and provide a better user experience.
Storing Outside of React
You can store the map instance outside of React, but the author of this article will explain how to do it in their next article.

The author has experience with JavaScript and Maps API, and has written about ReactJS in the past.
To use the mapbox-gl instance outside of React, the author suggests looking at their next article, which will cover this topic in more detail.
The author has also mentioned that they will be writing about this topic in their next article, specifically for React and Next.js users who are working with Mapbox.
If you're looking to store the map instance outside of React, you'll need to consider using JavaScript and the Maps API, as the author has suggested in their next article.
Here are some relevant technologies that the author has mentioned in their next article:
- JavaScript
- Maps API
- ReactJS
- Next.js
- Mapbox
- Typescript
Хранение Инстанса Карты
Хранение инстанса карты вне React - это важный вопрос, который затрагивается в одной из следующих статей. Итак, что мы знаем об этом?
Если вы хотите хранить инстанс карты mapbox-gl вне React, вы сможете сделать это, как только будет готова следующая статья.
Чтобы хранить инстанс карты вне React, важно оставить список зависимостей пустым в React.useEffect, чтобы не пересоздавать инстанс карты повторно, если initialOptions или onMapLoaded изменятся.
В этом случае важно оставить список зависимостей пустым, это позволит не пересоздавать инстанс карты повторно, если initialOptions или onMapLoaded изменятся.
Making Pins Interactive
To make pins interactive, we need to add an onClick handler to our pins. This will capture the details of the selected location.
We can use the Mapbox Popup component to display the name of the place when a pin is clicked. The Popup component takes an onClose handler which sets the selectedLocation to {}.
We'll also need to modify the render block to add the onClick handler and the Popup component. This will involve passing the latitude and longitude of the selected location to the Popup component.
By adding these interactive elements, we can make our map fully functioning and interactive.
Getting Started
MapBox is a good alternative to Google Maps with a competitive pricing structure and an easy-to-use API.
With the first 50,000 requests on web being free, it's a great option to consider.
You can set up a Next.js project by following the instructions in the official documentation or by running a simple command in your terminal.
Make sure you have Node.js installed before proceeding.
Once you have your access token, you can start setting up your project.
Data Fetching
To fetch data for the map, you can use a geocoding API like MapBox's search API. This API allows you to fetch a list of locations with their latitudes and longitudes.
MapBox's Geocoding Place Search API takes parameters such as limit and bbox to cap results and specify geographic bounds. For example, to search for Greggs locations in London, you can use the limit query parameter to cap results at 10 and the bbox parameter to specify the latitudinal and longitudinal bounds of London.
The search URL for this query would look something like "https://api.mapbox.com/geocoding/v5/mapbox.places/ London.json?access_token=YOUR_ACCESS_TOKEN&limit=10&bbox=51.3798,-0.1668,51.8081,0.0334". This URL can be used to make a simple fetch call in your page.
Once the data is fetched from the API, you can use it to render the locations as pins on the map.
Sources
- https://dev.to/dqunbp/using-mapbox-gl-in-react-with-next-js-2glg
- https://habr.com/ru/articles/565636/
- https://javascript.plainenglish.io/integrating-mapbox-with-next-js-the-cheaper-alternative-to-google-maps-460e4c2e6efc
- https://medium.com/@pether.maciejewski/next-js-13-mapbox-deck-gl-hexagon-layer-step-by-step-5528e7366750
- https://www.paigeniedringhaus.com/blog/render-multiple-colored-lines-on-a-react-map-with-polylines
Featured Images: pexels.com