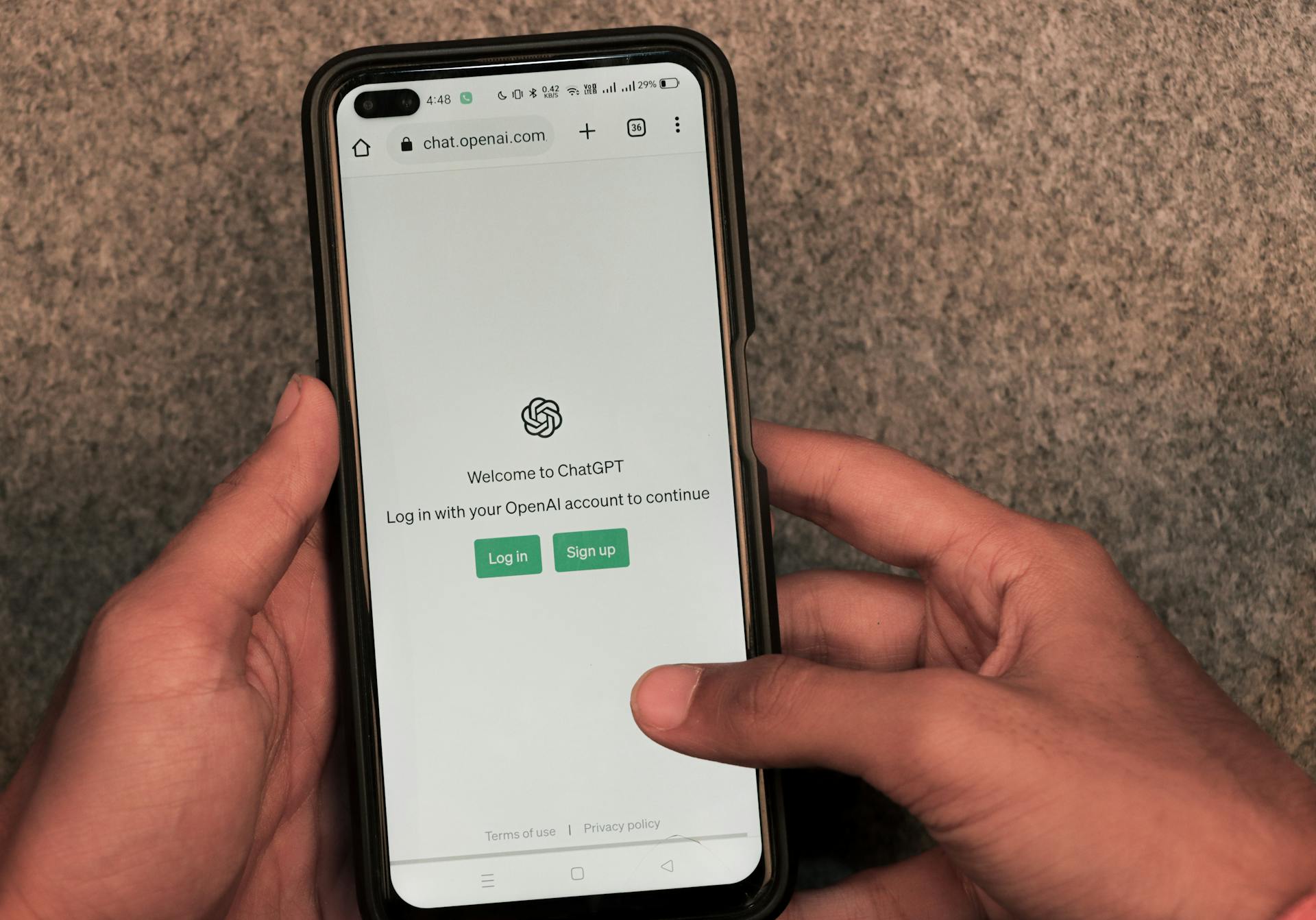
Building a responsive Next.js navbar is crucial for a seamless user experience. A well-designed navbar should adapt to different screen sizes and devices.
To start, you'll need to create a new file for your navbar component, such as Navbar.js. This will help keep your code organized and reusable.
A responsive navbar typically uses a combination of flexbox and media queries to adjust its layout. For example, you can use the `display` property in your CSS to change the navbar's layout from horizontal to vertical on smaller screens.
See what others are reading: Nextjs Nested Layout
Setting Up the Navbar
To set up the Navbar in Next.js, you'll need to create a new file in the components directory called Navbar.js.
In this file, you'll import the Link component from next/link and use it to create a link to the home page.
The Navbar component will contain a list of links, which are created using the Link component.
Each link is wrapped in a element, and the Link component is used to create a link to the corresponding page.
You can customize the appearance of the Navbar by adding a CSS file in the same directory as the Navbar.js file.
For example, you can add a styles.css file and use CSS classes to style the Navbar.
This will allow you to customize the colors, fonts, and layout of the Navbar to fit your needs.
A unique perspective: Can Amazon S3 Take in Nextjs File
Dynamic Navbar Content
To dynamically render the navbar content in Next.js, you can use JavaScript's map() function to render a list of items on the Header component. This approach eliminates the need for hard-coding the user interface of the navbar.
By moving into the data.js file, you can create an array of objects that hold the information or items to be rendered. For example, you can have an array with objects that include a path property, such as "/contact" or "#contact" for sections on the home page.
The map() function allows you to render a list of items on the Header component dynamically, making it easy to manage and update the navbar content.
A fresh viewpoint: Next Js Component Library
Mapping Array Items to Header Component
Mapping Array Items to Header Component is a game-changer for dynamic navbar content.
To start, you'll need to move into the data.js file in the src folder and create an array of objects that will hold the information or items you want to render.
This array will contain objects with different properties, such as path. Notably, some objects may have a "#contact" value, indicating that the contact section is on the home page.
You'll then need to import the array from the data.js file so you can access its properties.
By doing this, you can use JavaScript's map() function to render a list of items on the Header component, eliminating the need for hard-coding the user interface of the nav-bar.
With the array in place, you can use map() to create a dynamic list of items that can be easily updated or modified.
A unique perspective: How to Use Reducer Api in Next Js 14
Conditional Nav-Item Rendering
Conditional Nav-Item Rendering is a technique that allows you to dynamically show or hide nav-items based on the current page or route. To do this, you can use Next's useRouter hook, which lets you know when another page/route is currently active or "in-view" in a browser tab.
Fortunately, this hook is quite straightforward to use. We're passing item as props to the NavItem component so that it makes it dynamic to use in any case, not for the contact nav-item only.
Expand your knowledge: Framer Motion Nextjs
If the result is true, it assigns the value as props to the component, which will always be a string because of the prop validation check. This allows you to render the nav-item in the appropriate page.
The snippet above is quite straightforward, it's a simple way to conditionally render the nav-item. If not, it assigns an empty string to the component, which in turn ends up as nothing in the Header component.
If this caught your attention, see: Deploy Nextjs on Render
Adding Dropdown Menus
To add a dropdown menu to your navbar, you'll need to use Bootstrap's dropdown component and the Link component from the next/link module.
First, import the Link component by adding the necessary line at the top of your Navbar.js file.
The JSX code inside the Navbar component needs to be updated to include a dropdown menu, which is created by adding a new list item with the nav-item dropdown classes.
The dropdown toggle link has the nav-link dropdown-toggle classes and includes the necessary data-toggle and data-target attributes to enable the dropdown functionality.
For another approach, see: Adding Stripe to My Nextjs App
Inside the dropdown menu, use the Link component to create navigation links for the dropdown items, each wrapped in a component with the dropdown-item class.
If you follow these steps, you should now see a dropdown menu in the navbar that reveals the dropdown items when the toggle link is clicked.
A fresh viewpoint: Nextjs Pathname Server Component
Return the revised heading
Return the revised heading is a crucial aspect of a dynamic navbar, as it allows users to easily navigate through your website's content. The navbar can be updated in real-time based on user interactions, such as clicking on a dropdown menu or searching for a specific topic.
For instance, if a user clicks on a dropdown menu, the navbar can update to display the new content, such as a list of sub-pages or a search results page. This is achieved by re-rendering the navbar with the new content, which can be done using JavaScript.
A good example of this is the "Responsive Navbar" section, where we see how the navbar adapts to different screen sizes and devices. This is made possible by using a combination of CSS media queries and JavaScript.
By updating the navbar in real-time, you can provide a better user experience and make your website feel more dynamic and engaging.
Additional reading: Next Js Dynamic
Design and Layout
A cluttered navbar can overwhelm users, so it's essential to keep it simple and stick to the essential navigation links. This helps users quickly find what they're looking for.
Use clear and concise labels for your navigation links to make it clear to users what each link represents. This is crucial for users who may not be familiar with technical terms or jargon.
To provide visual feedback, use visual cues such as hover effects or active states to indicate the current page or active link in your navbar. This helps users understand their current location within the website or application.
A responsive design that adapts to different screen sizes is crucial for optimizing your navbar for mobile devices. This ensures a seamless user experience on mobile devices.
You might like: Next Js Useeffect
UI Design Principles
Keep your navbar simple and clutter-free, just like you would want your home to be organized. A cluttered navbar can overwhelm users, making it difficult for them to find what they're looking for.
Use clear and concise labels for your navigation links to make it clear to users what each link represents. This means avoiding jargon or technical terms that may not be familiar to all users.
Providing visual feedback is essential to give users a sense of where they are within the website or application. Use visual cues such as hover effects or active states to provide feedback to users when they interact with the navbar.
Ensure your navbar is accessible to all users, including those with disabilities. Use appropriate HTML tags and attributes, and ensure that the navbar can be navigated using keyboard controls.
Optimize your navbar for mobile devices by using a responsive design that collapses into a mobile menu on smaller screens. This will provide a seamless user experience across different devices and screen sizes.
Mobile
Our mobile navigation design needs work. The current design doesn't scale well to smaller screens.
To fix this, we can create a new navigation menu specifically for mobile. We can store the state of whether the menu is open or closed in our application's state.
Curious to learn more? Check out: Next Js Navigation
We can then use a button element to toggle the menu open and closed. This is a simple but effective way to control the menu's visibility.
Conditional rendering comes in handy here, allowing us to hide or show menu items based on whether the menu is open or closed. We can also change the icon on the button to indicate its current state.
Adding animations to the menu can help make it feel more polished and user-friendly. We can use Framer Motion to create animations that make the menu options slide in and out by scaling them.
By setting up these animations to run whenever our component is mounted or unmounted, we can create a smooth and seamless user experience.
Broaden your view: Nextjs Button
Sources
- https://nextui.org/docs/components/navbar
- https://www.freecodecamp.org/news/dynamic-navigation-in-nextjs/
- https://www.squash.io/creating-a-navbar-in-next-js-using-javascript/
- https://upmostly.com/next-js/how-to-enhance-your-next-js-app-with-a-dynamic-navigation-bar
- https://flowbite.com/docs/getting-started/next-js/
Featured Images: pexels.com