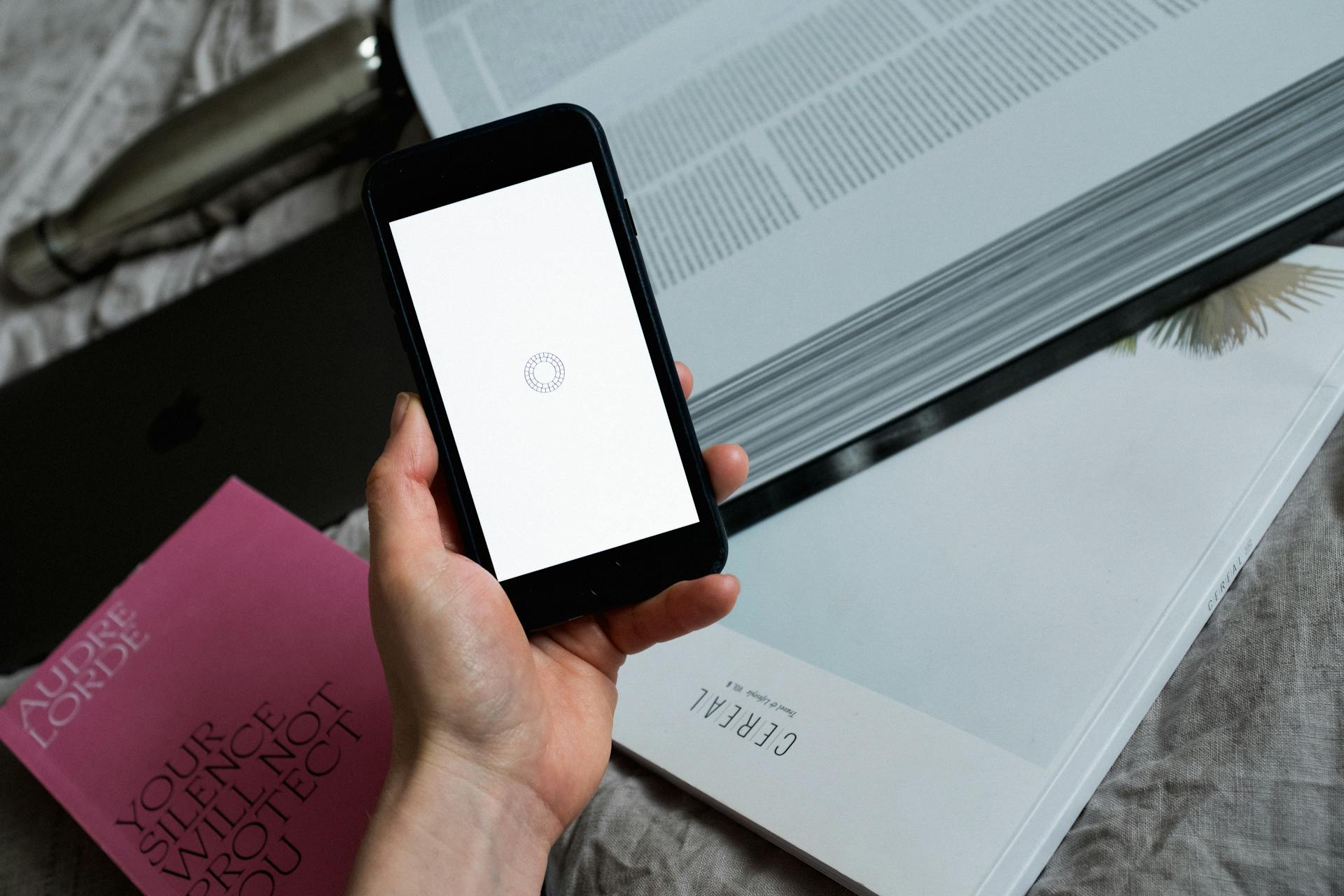
Next.js Suspense is a game-changer for optimizing performance in your React applications. By using Suspense, you can easily handle dynamic imports and loading states, making your app feel more responsive and engaging to users.
With Suspense, you can load data and components on demand, without blocking the main thread of your app. This is achieved through the use of a special component called Suspense Boundary, which wraps your app's components and handles the loading state for you.
The key to mastering Next.js Suspense is to understand how to use it in conjunction with dynamic imports. By importing components and data only when they're needed, you can significantly reduce the amount of code that needs to be loaded upfront, making your app feel faster and more efficient.
Next.js Suspense
Next.js Suspense is a powerful tool that allows you to handle loading states and errors in a more efficient and user-friendly way. With Suspense, you can create a seamless user experience by displaying a fallback UI while data is being loaded.
You can use Suspense in Next.js by adding a loading.js file to the route directory, where you can define a functional component that will be used as the fallback UI. This component can be exported as the default export of the loading.js file.
To integrate Suspense with data fetching libraries like SWR, you can use the fetch response as the data variable and ensure that it's always available on render. However, keep in mind that Suspense doesn't handle errors that may occur during data fetching, so it's essential to nest your Suspense component within an ErrorBoundary to catch any errors effectively.
Here are some best practices to keep in mind when using Suspense:
- Avoid suspending the whole app by applying Suspense only to parts of the UI that depend on the data being loaded.
- Nest Suspense and error boundaries carefully to ensure predictable behavior and easy error management.
- Provide a fallback for server errors and client-only content by throwing an error in the server environment and wrapping the component in a Suspense boundary.
By following these tips and using Suspense in Next.js, you can create a more efficient and user-friendly application that handles loading states and errors with ease.
Integrating SWR with Next.js
Integrating SWR with Next.js is a powerful combination for efficient data fetching and smooth user experiences. By leveraging Suspense's blocking, SWR's caching, and error boundary, you can build resilient and responsive UIs that efficiently handle loading states and errors.
To integrate SWR with Next.js, you can use a single line of code to simplify the logic of data fetching. This is made possible by SWR's fetch request states, such as success, loading, and error, which can be used to gracefully handle boundaries in a traditional way.
However, if you want to use React Suspense with SWR, you can do so with additional benefits. In Suspense mode, the fetch response is always available in the data variable and is guaranteed to be ready on render without needing to explicitly check if the data is undefined.
But, it's essential to note that Suspense itself doesn’t handle errors that may occur during data fetching. If an error occurs during the fetch request, the promise returned by the data fetching function will be rejected. To handle errors effectively, always nest your Suspense component within an ErrorBoundary.
Here's a summary of the key benefits of integrating SWR with Next.js:
- Use of React Server Components with Suspense
- Partial pre-rendering
- Refactored streaming logic
By following these best practices and using the right tools, you can create efficient and responsive data fetching experiences in your Next.js applications.
Props
In Next.js Suspense, the `children` prop is where you put the actual UI you intend to render. This is where the magic happens, and it's where you'll render your app's components.
The `fallback` prop is an alternate UI to render in place of the actual UI if it hasn't finished loading. It's a lightweight placeholder view, like a loading spinner or skeleton.
You can pass any valid React node as a fallback, but in practice, it's best to keep it simple and lightweight. This is because if the fallback suspends while rendering, it will activate the closest parent Suspense boundary.
Here are the key props you need to know:
- children: The actual UI you intend to render.
- fallback: An alternate UI to render if the actual UI has not finished loading.
As you can see, these props work together to create a seamless user experience. By using `children` and `fallback` in tandem, you can ensure that your app remains responsive even when data is loading.
Optimizing Performance
Concurrent rendering is a key feature in React 18 that allows React to work on multiple tasks simultaneously and prioritize important updates, improving the performance and responsiveness of React applications.
This means React can pause or interrupt low-priority rendering processes to handle urgent tasks, like responding to user input. Memoization can also be used to cache expensive computations, preventing unnecessary re-renders and improving the performance of components during suspenseful loading.
Lazy loading is a technique that allows you to load components only when they are needed, which can significantly improve the initial load time of your application by reducing the size of the initial JavaScript bundle.
Here are some benefits of using Next.js with React Suspense:
- Use of React Server Components with Suspense
- Partial pre-rendering
- Refactored streaming logic
Streaming with Suspense can also improve performance by allowing independent chunks to be sent over and rendered in the browser as soon as they are ready, independent of each other.
Concurrent Rendering
Concurrent rendering is a game-changer for React applications, introduced in React 18 to improve performance and responsiveness. It allows React to work on multiple tasks simultaneously, prioritizing important updates.
By enabling concurrent rendering, React can pause or interrupt low-priority rendering processes to handle urgent tasks, like responding to user input. This means you can keep your UI interactive even when fetching data or performing other time-consuming tasks.
Concurrent rendering is particularly useful with Suspense, which can now selectively suspend parts of the component tree, rather than blocking the entire UI. This is a big improvement over the old way, where Suspense would block the entire UI until data was ready.
One key benefit of concurrent rendering is that it lets you avoid showing Suspense fallbacks in favor of inline indicators. This is especially useful in application code where you want to mark a part of the UI as non-urgent and let it "lag behind" the rest of the UI.
Here are some ways to use concurrent rendering effectively:
- Use the useDeferredValue Hook to pass a deferred version of the query down the component tree.
- Use Transitions to mark the whole update as non-urgent, typically used by frameworks and router libraries for navigation.
- Wrap components in Suspense boundaries to selectively suspend parts of the component tree.
By leveraging concurrent rendering and Suspense, you can create a smoother, more responsive user experience that's better for both users and search engines.
Memoization
Memoization is a powerful technique that can significantly improve the performance of your application. By caching the results of expensive computations, you can prevent unnecessary re-renders and improve the performance of components during suspenseful loading.
This technique is particularly useful when dealing with complex computations or data that doesn't change often. Memoization can help reduce the computational overhead and make your application feel more responsive to users.
I've seen firsthand how memoization can make a big difference in the performance of a React application. By applying memoization to a component that renders a list of items, we were able to reduce the number of unnecessary re-renders and improve the overall user experience.
Memoization can also be used to optimize the performance of suspenseful loading scenarios. By caching the results of expensive computations, you can ensure that components render quickly and efficiently, even when data is still loading.
Lazy Loading in Next.js
Lazy loading is a technique that allows you to load components only when they are needed, significantly improving the initial load time of your application by reducing the size of the initial JavaScript bundle.
In Next.js, you can use the React.lazy function to create a lazy-loaded component. The React.lazy function takes a function that returns a dynamic import, which means you can use dynamic imports to load the component's module only when it's required.
Lazy loading is especially useful in Next.js because it allows you to defer the loading of components until they are actually needed, rather than loading them all at once. This can be a big win for performance, especially on slower networks or for larger applications.
To use lazy loading in Next.js, you can follow the same approach as in regular React applications. Simply use the React.lazy function to create lazy-loaded components and wrap them with the React.Suspense component for data fetching.
Here are some key benefits of lazy loading in Next.js:
- Significant improvement in initial load time
- Reduces the size of the initial JavaScript bundle
- Improves performance on slower networks
- Allows for more efficient loading of components
By using lazy loading in Next.js, you can create a faster, more efficient, and more scalable application that provides a better user experience.
Preventing Revealed Content Hiding
A jarring user experience can occur when a component suspends and the closest parent Suspense boundary switches to showing the fallback, hiding already revealed content. This can happen when a navigation state update is urgent, and React doesn't have time to wait for the content to load.
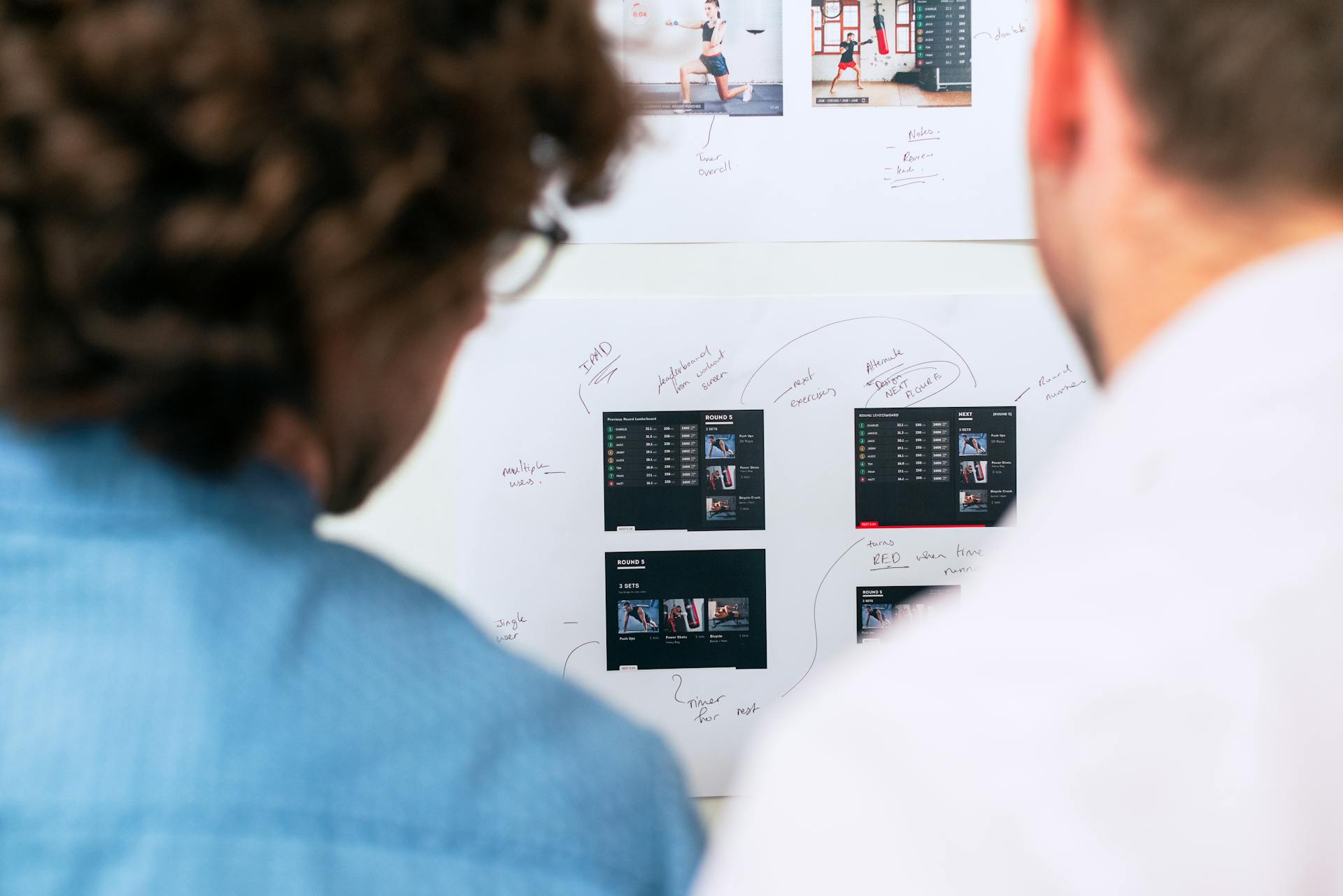
To prevent this, you can mark the navigation state update as a Transition with startTransition. This tells React that the state transition is not urgent, and it's better to keep showing the previous page instead of hiding any already revealed content.
A Transition doesn't wait for all content to load, just long enough to avoid hiding already revealed content. For example, if the website Layout is already revealed, it would be bad to hide it behind a loading spinner.
You can use Transitions in Suspense-enabled routers, which are expected to wrap navigation updates into Transitions by default.
Resetting Navigation Boundaries
React will avoid hiding already revealed content during a Transition, but you can express that it is different content with a key.
Navigating within a user's profile page doesn't trigger the fallback for already visible content, as expected.
However, navigating between two different user profiles makes sense to show the fallback, treating different users' profiles as different components.
By specifying a key, you ensure React resets the Suspense boundaries during navigation, which is what Suspense-integrated routers should do automatically.
Data Fetching and Loading
You can use React Suspense to display a fallback while content is loading, by wrapping a component with a Suspense boundary. This will display the fallback until all the code and data needed by the children has been loaded.
To reveal nested content as it loads, you can nest multiple Suspense components to create a loading sequence. Each Suspense boundary's fallback will be filled in as the next level of content becomes available.
In Next.js, you can use the React.lazy function to create lazy-loaded components and wrap them with the React.Suspense component for data fetching. However, you need to make sure that dynamic imports are used only on the client-side to avoid server-side conflicts.
To show stale content while fresh content is loading, you can use the useDeferredValue Hook to pass a deferred version of the query down. This will keep showing the previous results until the new results are ready.
Here are some common use cases for data fetching and loading:
- Displaying a fallback while content is loading: use React Suspense to display a fallback until all the code and data needed by the children has been loaded.
- Revealing nested content as it loads: use multiple Suspense components to create a loading sequence.
- Showing stale content while fresh content is loading: use the useDeferredValue Hook to pass a deferred version of the query down.
- Avoiding suspending the whole app: implement Suspense only to parts of the UI that depend on the data being loaded.
By using these techniques, you can create a smooth and responsive user experience in your Next.js application.
Sources
- https://blog.logrocket.com/using-next-js-react-suspense-create-loading-component/
- https://dev.to/peterlidee/using-loadingjs-and-suspense-in-next-13-1n7g
- https://react.dev/reference/react/Suspense
- https://pasquale-favella.github.io/blog/14
- https://www.nico.fyi/blog/simplify-data-fetching-with-rsc-suspense-and-use-api-in-next-js
Featured Images: pexels.com