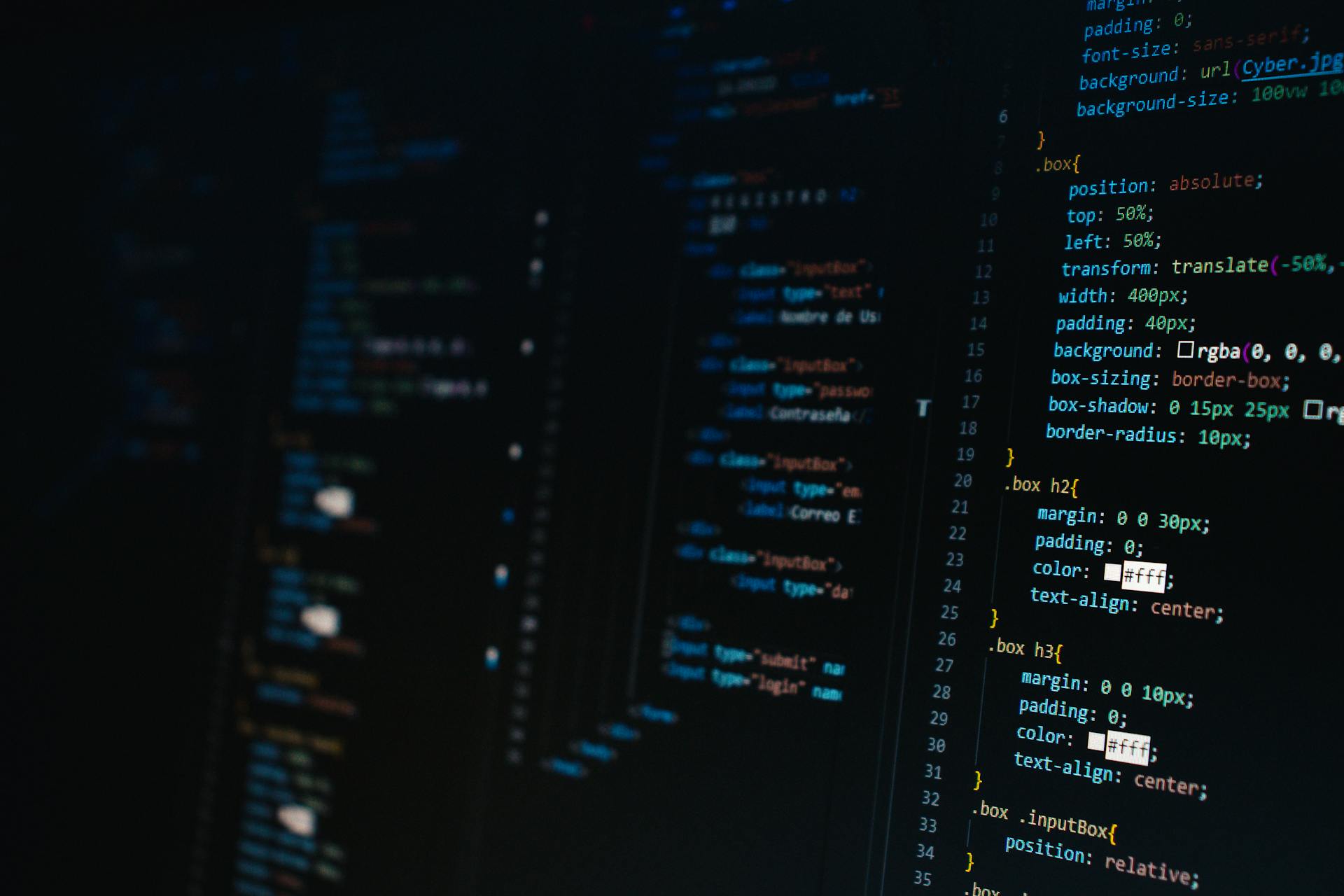
Nextjs is an open-source React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
It's ideal for complex applications and websites that require fast page loads and SEO benefits.
To start with Nextjs, you'll need to install the Nextjs CLI tool, which can be done by running the command npm install next@latest or yarn add next@latest.
This will install the Nextjs CLI tool, allowing you to create a new Nextjs project quickly and easily.
A fresh viewpoint: Tutorial Building Useful Nextjs Tool
Get Started
To get started with Next.js, you can use the create-next-app command to create a new project. This command will ask you to choose a name for your project and some options, then creates a project directory for you and installs the necessary dependencies.
You can also create a Next.js project manually by installing the next, react, and react-dom packages, and adding scripts to your package.json file. This will allow you to start your app in development mode with `npm run dev`, in production mode with `npm run start`, and build your app for production with `npm run build`.
Discover more: Nextjs Dark Mode
One of the easiest ways to get started with Next.js is to use the create-next-app command, which will create a project directory and install the necessary dependencies. This command is simple and straightforward, and you can also choose from a list of starters to get going with a specific setup, such as Next.js with Redux or Next.js with Tailwind CSS.
To create a Next.js app, you can use either the create-next-app command or create the project manually. If you choose to create the project manually, you'll need to install the next, react, and react-dom packages, and add scripts to your package.json file.
You can start a new Next.js app using the create-next-app command, which will create a project directory and install the necessary dependencies. This will give you a good starting point for managing your project's source code history.
Here are the basic scripts to get you started:
- dev starts Next.js in development mode.
- start starts Next.js in production mode.
- build builds your Next.js app for production.
Regardless of which method you choose, this will generate the basic Next.js application template.
Creating a Next.js Project
To create a Next.js project, you'll need Node.js and npm (or npx) installed. Ideally, npm comes with your Node.js installation.
You can check if you have Node.js installed by running a command in your terminal. If npm (and npx) comes with your Node.js installation, you can confirm it with another command.
If you prefer the yarn package manager, you can install it with a command and then confirm the installation with another command.
Creating a Next.js app can be done in two ways: with create-next-app or manually. The simplest way is to use create-next-app, which will ask you to choose a name for your project and some options.
Using create-next-app is simple and straightforward. You can also get going with a starter like Next.js with Redux, Next.js with Tailwind CSS, or Next.js with Sanity CMS.
Alternatively, you can create a Next.js app manually by installing three packages: next, react, and react-dom. Then, add the following scripts to package.json: dev starts Next.js in development mode, start starts Next.js in production mode, and build builds your Next.js app for production.
Here are the basic steps to create a Next.js app manually:
- Install Node.js if you haven't already.
- Install the three packages: next, react, and react-dom.
- Add the following scripts to package.json: dev, start, and build.
Project Structure
================
A Next.js project starts with three folders: pages, public, and styles. Each Next.js project starts with 3 folders: pages, public, and styles.
The pages folder contains your page files, each with a unique route automatically created from the file name. For example, the Next.js page hello.js would be found at pages/hello.js.
Some pages, like _app.js, include an underscore prefix in their name to mark them as custom components. These components are used by Next.js to work with other components.
The public folder is for static file serving, meaning these files don't change and can only be referenced. This folder often contains images or icons the site uses as well as internal information like Google Site Verifications.
The styles folder contains our CSS style sheets, which determine the appearance of all our page elements. The globals.css file sets the general standard that all pages in the project will use.
Here's a breakdown of the main folders and their purposes:
Understanding the folder structure is key to manipulating it to fit your own projects.
Pages and Routing
In a Next.js app, the pages folder is one of the Next-specific folders you get. It's where you'll find all your page components.
Each file in the pages folder is a page and each page is a React component. This is a fundamental concept to grasp when working with Next.js.
Custom pages are special pages prefixed with the underscore, like _app.js. These pages are used for specific use cases, such as setting up global layouts.
Next.js has a file-based routing system where each page automatically becomes a route based on its file name. For example, a page at pages/profile will be located at /profile.
Here's a list of the types of pages you can have in Next.js:
- Pages with a file name, like profile.js, which will be located at /profile.
- Custom pages, like _app.js, which are used for specific use cases.
You can also have index routes in your pages folder, which are automatically routed to the starting point of your application. For example, pages/index.js will be routed to /.
Styling and Formatting
Next.js comes with three styling methods out of the box: global CSS, CSS Modules, and styled-jsx.
To add basic styles to your Next.js app, start by removing all the content in app/globals.css and replace it with the following basic styles. This will give you a clean slate for your styles.
You can also use ESLint and Prettier for linting and formatting your codebase. This is a popular combination that many developers enjoy using. To include it in your Next.js project, create a .eslintrc file at the root of your app with the content specified in the example.
You can either lint and format your code manually or let your editor take control. If you're using VSCode, you'll need to install the ESLint VSCode plugin and add the following commands to your VSCode settings.
Styling
Next.js offers three styling methods out of the box: global CSS, CSS Modules, and styled-jsx. These methods can be used to add visual flair to your application.
The Next.js starter app includes some basic CSS styles, but they're not intended for reuse in a real app. To create a clean slate for your styles, remove all the content in app/globals.css and replace it with basic styles.
Next.js has an extensive article about Styling that provides a more in-depth look at these methods. This article is also referenced in Comparing Styling Methods in Next.js.
Linting and Formatting
Linting and formatting is a crucial aspect of coding, and it's great that there are tools like ESLint and Prettier that can help with it. These tools are highly opinionated, but empirical metrics show that most people enjoy using them.
ESLint is a linter that checks your codebase for errors, while Prettier formats your code to make it more readable. One popular setup is Wes Bos's ESLint and Prettier Setup, which extends eslint-config-airbnb and includes sensible defaults.
To include this setup in your Next.js project, you can install it locally by running a command in your terminal. You'll also need to create a .eslintrc file at the root of your app with specific content.
You can either lint and format your code manually or let your editor take control. To do it manually, you'll need to add two npm scripts: lint and lint:fix. If you're using VSCode, you can install the ESLint VSCode plugin and add specific commands to your settings to automate the process.
Some rules may need to be overridden, such as the react/jsx-props-no-spreading rule, which errors out when JSX props are spread.
Broaden your view: Next Js Free Code Camp
Absolute Imports
Absolute imports are a game-changer for Next.js developers.
You can import components absolutely starting from Next.js 9.4, eliminating the need for relative imports like import Header from '../components/Header'. Instead, you can do so absolutely like import Header from 'components/Header'.
To get this to work, you'll need a jsconfig.json or tsconfig.json file for JavaScript and TypeScript respectively, with specific content. This assumes that the components folder exists at the root of your app, alongside pages, styles, and public.
SEO
SEO is crucial for making your website visible to search engines. NextJS offers built-in SEO optimizations such as server-side rendering.
This means that your website will load faster and be more easily crawlable by search engine bots. Developers can also use meta tags to further enhance SEO.
Automatic code splitting is another feature that NextJS offers, which can improve page load times and search engine visibility.
API Routes and Server-Side Rendering
API routes in Next JS allow you to create server-side logic separately from your main application logic. They're stored in the pages/API directory and can be accessed via HTTP requests.
On a similar theme: Nextjs Api
You can use API routes to handle server-side logic, such as fetching data from an external API or performing complex calculations. This keeps your main application logic clean and easy to maintain.
API routes can be used to fetch data on the server with fetch, which allows you to configure caching and revalidating behavior for each request. This is a powerful feature that enables you to optimize data fetching for your application.
Next JS also offers special functions for fetching data, including getStaticProps and getServerSideProps. These functions are used for Static Generation and Server-side Rendering, respectively.
Here's a brief overview of when to use each:
API routes can be used in conjunction with Server-side Rendering to fetch data on the server and return it to the client. This approach is useful when you need to fetch data that's sensitive or shouldn't be exposed to the client.
Revalidating data is an important aspect of API routes, and Next JS offers two types of revalidation: time-based and on-demand. Time-based revalidation automatically revalidates data after a certain amount of time has passed, while on-demand revalidation is triggered by an event, such as a form submission.
You might enjoy: Apolloclient Nextjs
Advanced Topics
In Next.js, you can use getStaticProps to pre-render pages at build time, making them static and serverless. This approach is especially useful for blogs and documentation sites.
For example, in the "Getting Started" section, we saw how to create a new Next.js project using the command `npx create-next-app my-app`. This command sets up a basic project structure, including a `pages` directory where you can create individual pages.
Using getStaticProps, you can fetch data from an API or database and pass it to the page component as a prop. This allows you to render dynamic content on the server without having to use client-side JavaScript.
Consider reading: Nextjs Context
Third-Party Libraries on the Server
When working with third-party libraries on the server, you might encounter issues with caching and revalidating data.
You can configure caching and revalidating behavior using the Route Segment Config Option.
This is particularly useful when working with libraries that don't support or expose fetch, such as databases, CMS, or ORM clients.
These libraries can be tricky to work with, but with the right approach, you can still achieve the desired caching and revalidating behavior.
For example, you can use React's cache function to configure caching and revalidating behavior for those requests.
Experimental ES Features
In Next.js, you can use some experimental features to take your app to the next level. The Nullish coalescing operator (??) is one of them, allowing you to provide a default value when the expression on the left is null or undefined.
The Optional chaining (?.) feature is another powerful tool that helps you avoid null pointer exceptions by providing a safe way to access nested objects or arrays.
Deployment and Optimization
To deploy your Next.js app, you'll need to use the Vercel CLI. Install it by running `npx vercel install`, which will guide you through the process of logging in to Vercel, creating the app, and deploying it.
Once deployed, you'll need to add your API token to the remote app's environment variables. This allows your app to make requests to Replicate.
Enter the token you used in Step 3, and then deploy again.
Example and Getting Started
Let's dive into the example and getting started with Next.js.
A full Next.js application is laid out in a specific way, starting with the index.js file, which represents the single page on the project.
The index file is the core of this application, containing only this single page.
Frequently Asked Questions
Is Next.js easier than React?
Yes, Next.js is generally easier to use than React due to its simplified coding requirements and streamlined project management. With Next.js, developers can build applications faster and with less code.
Sources
- https://www.smashingmagazine.com/2020/10/getting-started-with-next-js/
- https://replicate.com/docs/guides/nextjs
- https://www.educative.io/blog/nextjs-tutorial-examples
- https://www.geeksforgeeks.org/getting-started-with-next-js/
- https://hackernoon.com/a-step-by-step-guide-to-building-a-simple-nextjs-13-blog
Featured Images: pexels.com