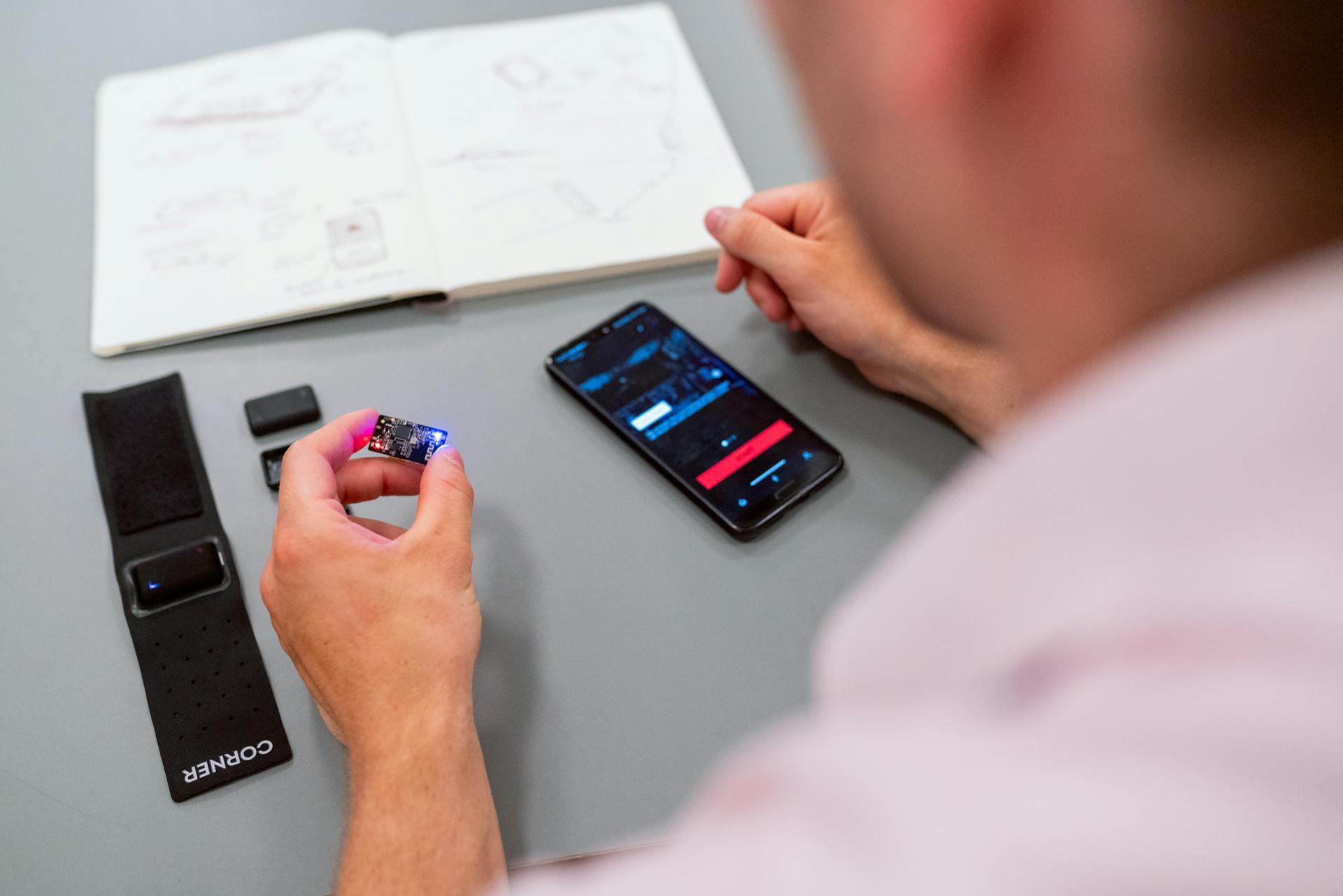
Next.js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications. It was created by Vercel, a company that specializes in web development and deployment.
Next.js is built on top of Node.js and uses modern web technologies like ES6 and Webpack to enable fast and efficient development. This allows developers to create complex web applications quickly and easily.
One of the key features of Next.js is its ability to pre-render pages at build time, which improves performance and reduces the load on servers.
What is Next.js?
Next.js is a React framework that enables several extra features, including server-side rendering and static rendering. It's traditionally used to build web applications rendered in the client's browser with JavaScript, but that has its problems.
Next.js sidesteps these issues by allowing some or all of the website to be rendered on the server-side before being sent to the client. This is one of the reasons it's become so popular.
Next.js requires Node.js and can be initialized using npm.
Background
Next.js is a React framework that enables several extra features, including server-side rendering and static rendering. This is a game-changer for building web applications, as it allows some or all of the website to be rendered on the server-side before being sent to the client.
Developers recognize several problems with traditional client-side rendering, such as not catering to users who don't have access to JavaScript or have disabled it. This can lead to potential security issues, significantly extended page loading times, and harm to the site's overall search engine optimization.
Next.js sidesteps these problems by allowing server-side rendering, making it a popular choice among developers. As of October 2024, the framework is used by many large websites, including Walmart, Apple, Nike, Netflix, TikTok, Uber, Lyft, Starbucks, and Spotify.
The framework's original author, Guillermo Rauch, is currently the CEO of Vercel, and the project's lead maintainer is Tim Neutkens. Google has contributed to the Next.js project, including 43 pull requests in 2019.
Development History
Next.js was first released as an open-source project on GitHub on October 25, 2016.
It was developed based on six principles, including out-of-the-box functionality requiring no setup, JavaScript everywhere, and automatic code-splitting and server-rendering.
Next.js 2.0 was announced in March 2017 and included several improvements that made it easier to work with small websites.
Version 7.0 was released in September 2018 with improved error handling and support for React's context API.
Version 8.0, released in February 2019, was the first version to offer serverless deployment of applications.
Next.js 9.3, announced in March 2020, included various optimizations and global Sass and CSS module support.
Version 9.5, released on July 27, 2020, added new capabilities including incremental static regeneration, rewrites, and redirect support.
Next.js 11 was released on June 15, 2021, introducing Webpack 5 support and a preview of real-time collaborative coding functionality called "Next.js Live".
Version 12, released on October 26, 2021, added a Rust compiler, making the compilation faster, AVIF support, and Edge Functions & Middleware.

Next.js 13, released on October 26, 2022, brought about a new routing pattern in beta, with the addition of the App Router.
The stable version of App Router was released in May 2023, allowing developers to use it in production.
In October 2023, Vercel released Next.js 14, which comes with improved memory management using edge runtime.
Key Features
Next.js is an incredibly powerful tool for building fast and secure web applications.
It supports styling with CSS, as well as precompiled Scss and Sass, CSS-in-JS, and styled JSX.
One of the main features of Next.js is its use of server-side rendering to reduce the burden on web browsers and provide enhanced security.
This can be done for any part of the application or the entire system, allowing for content-rich pages to be singled out for server-side rendering.
Next.js also includes support for dynamic routing, making it a convenient choice for developers.
Hot-module replacement is another feature that allows modules to be replaced live, without requiring the server to be restarted.
Automatic code splitting is also available, which only includes code necessary to load the page, reducing the overall load time.
Incremental Static Regeneration and static site generation are also supported, allowing for a pre-built version of the website to be cached and sent to users.
Routing and Optimization
Routing in Next.js is incredibly easy, you just need to create a file within the pages folder and it becomes a route. For example, creating a file called about.js will give you a /about page.
You can even create folders to create routes like /blog/my-post-title. And if you want to dynamically create pages, you can use a file name like [color].js, which will allow you to create a page for each color in your array.
The Link component is another powerful tool in Next.js, allowing you to do client-side route transitions and making page transitions smoother for users. It's used in place of an a tag and is especially useful for linking to other pages within your app.
Routing
With Next.js, you can create routes by simply creating a file within the pages folder. This will automatically turn that file into a route. For example, creating an "about.js" file will give you a "/about" page.
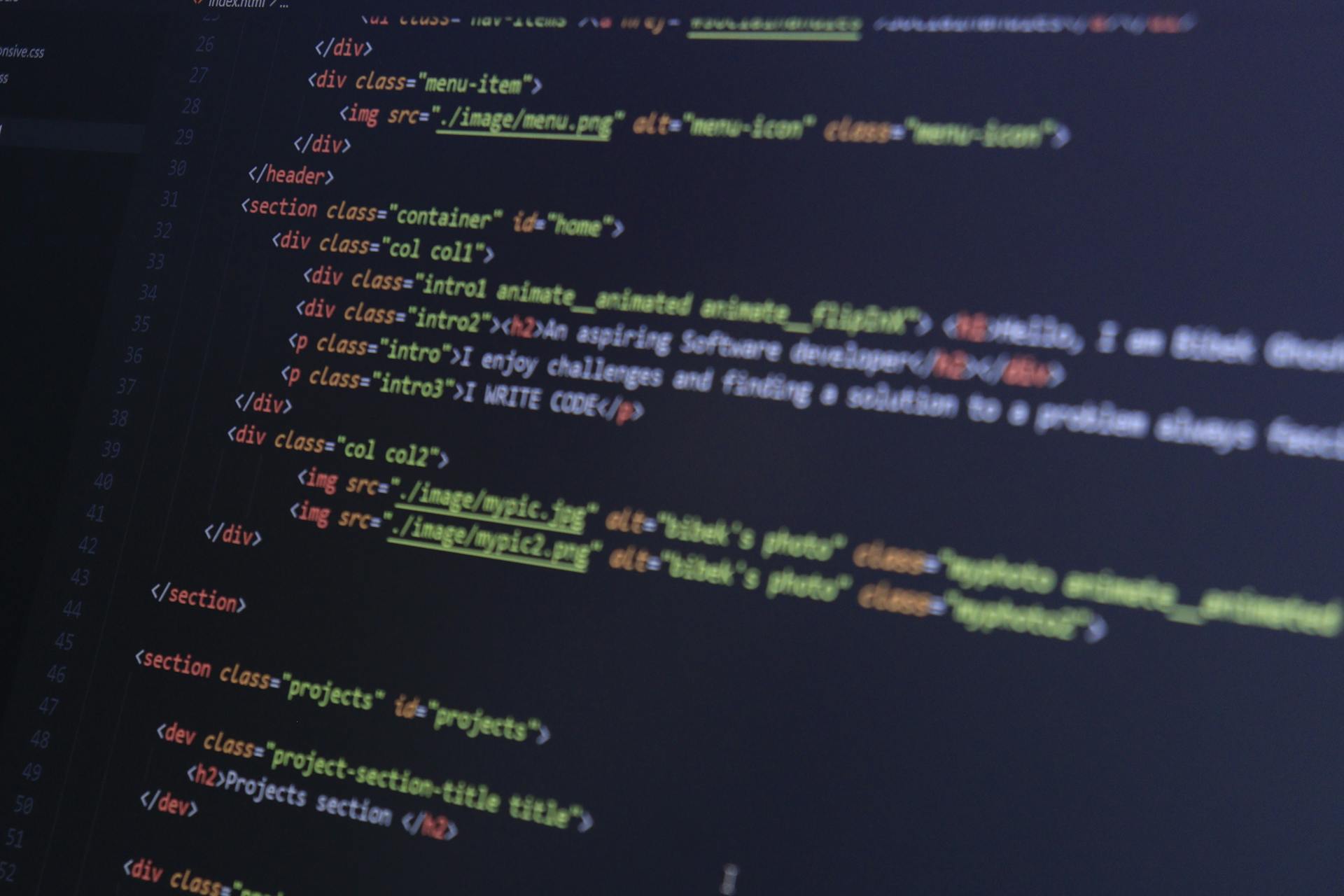
You can also create nested routes by making folders, allowing you to create routes like "/blog/my-post-title". This is useful for organizing your routes in a logical way.
To dynamically create routes for each item in an array, you can create a file called "[color].js" and put the file name inside brackets. This will allow you to create a page for each item in the array without having to create a separate file for each one.
For instance, if you have an array of colors, you can create a file called "[color].js" to create a page for each color. This will make it easy to add or remove colors without having to update multiple files.
Ssr vs. Ssg
When you're building a Next.js app, you've got two main options for how to handle data: Static Site Generation (SSG) and Server-Side Rendering (SSR). SSG is great for sites that don't need to update too often with content, like a blog.
With SSG, Next.js generates HTML for each page at build time, which can then be cached by a CDN for super performance. This means if your site is built and deployed, it won't update with any API changes, like if the 2022 color of the year was added.
SSR, on the other hand, allows you to still render HTML on the server-side, but do it for each request made by a user to the page instead of at build time. This is perfect for sites that need to update dynamically.
To use SSR instead of SSG, you'd replace getStaticProps and getStaticPaths with getServerSideProps. However, this example won't work because we didn't actually create an API!
If you want to read more about SSR vs. SSG, there's a full blog post about the difference.
Integration and Tools
Next.js has a rich ecosystem of integrations and tools that make it easy to build and deploy applications. The official Sanity toolkit for Next.js is a great example of this, allowing developers to integrate content from Sanity with features like previews and Studio embedding.
One of the key benefits of using Next.js is its ability to integrate with other services and tools. The Sanity toolkit is a great example of this, providing a simple and straightforward way to integrate Sanity content into a Next.js application.
Create a App
Let's get started with creating an app that integrates with our tools. To do this, we'll use the CLI to initialize a Next.js app, which will generate a bunch of starter files for us.
This process is similar to most app initialization scripts, making it easy to get started. We'll then start the development server, which has hot reloading built-in and links to the docs on the generated home page.
Sanity Toolkit
The Sanity Toolkit is a must-have for Next.js developers. It's the official toolkit for integrating content from Sanity.
With the Sanity Toolkit, you can easily add previews to your Next.js app. This is super helpful for testing and debugging purposes.
The toolkit also includes Studio embedding, which allows you to seamlessly integrate Sanity's Studio into your app. This makes it easier to manage and edit content.
Webhook verification is another key feature of the Sanity Toolkit. This ensures that your app receives and processes webhooks correctly.
The Sanity Client is another important tool for Next.js developers. It provides a Sanity Client for Next.js Apps with App Dir Support.
This means you can easily connect your Next.js app to Sanity and start fetching data.
Frequently Asked Questions
What is next.js vs. React?
Next.js is a framework built on React, used for server-side rendering and web app development, while React is an open-source library for rendering UI components to the DOM. Understanding the key differences between Next.js and React is essential for choosing the right tool for your project.
Is NextJS a frontend or backend framework?
NextJS is a frontend framework that integrates with Node.js for backend capabilities, making it a unique hybrid solution. This combination allows developers to build robust and scalable applications with a single framework.
Is Next.js a JavaScript framework?
Next.js is a React framework, not a JavaScript framework, but it's built on top of JavaScript and leverages its capabilities. It's a powerful tool for building fast and scalable web applications with React.
Is next a library or framework?
Next.js is a framework, not a library, as it provides additional features beyond React's core functionality. It's built on top of React, offering server-side rendering and code splitting, among other benefits.
Is NextJS a fullstack framework?
Yes, Next.js is a full-stack framework, meaning it supports both front-end and back-end development. It's a powerful tool for building robust and scalable web applications.
Featured Images: pexels.com