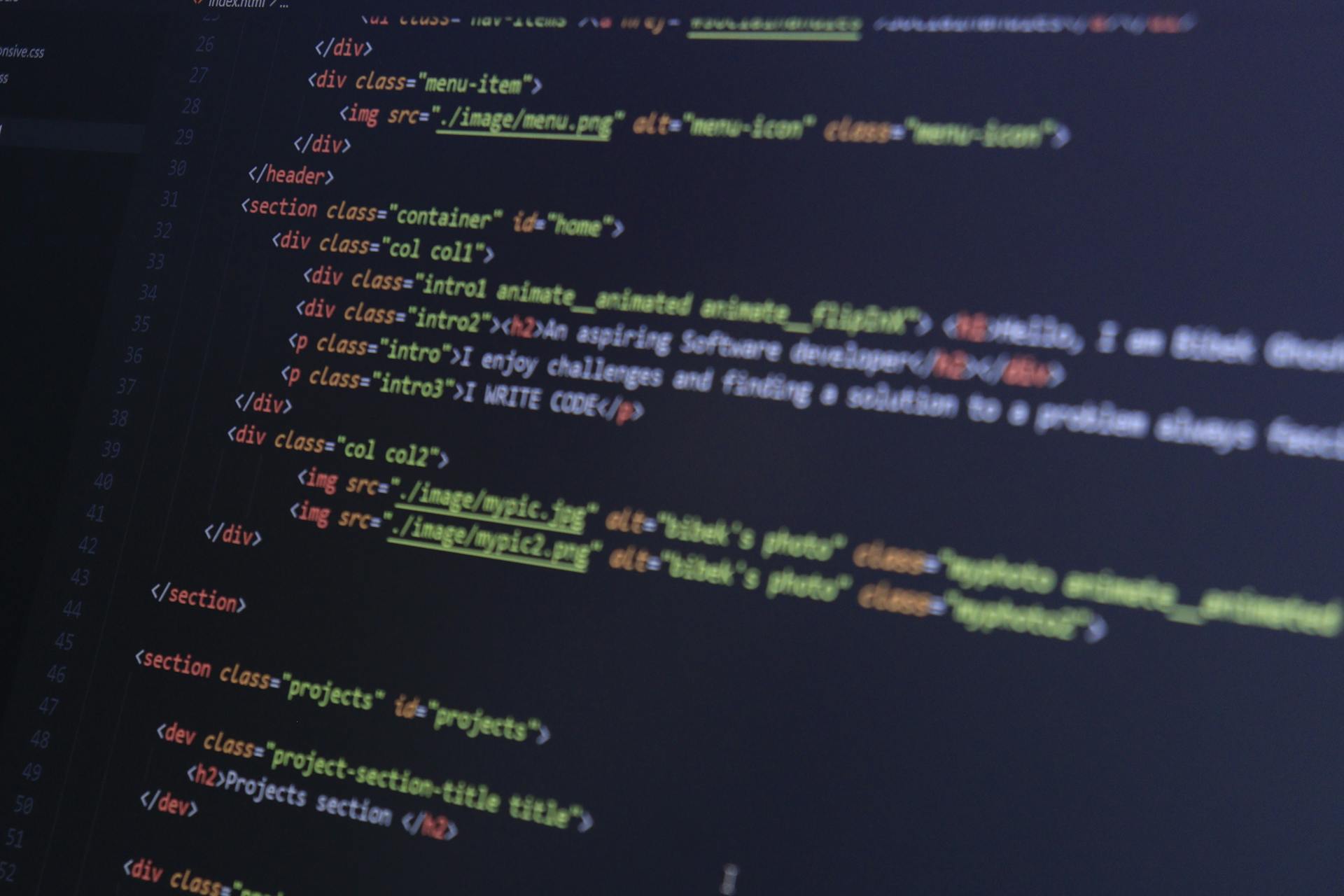
The MERN stack is a popular full-stack web development framework that combines MongoDB, Express.js, React.js, and Node.js to create robust and scalable applications.
MERN stack development is ideal for building complex web applications with a scalable database and efficient server-side rendering.
This tutorial will guide you through the process of setting up a MERN stack project, covering the basics of each technology and providing practical examples to help you get started.
You'll learn how to create a RESTful API with Express.js, connect it to a MongoDB database, and use React.js for client-side rendering.
On a similar theme: Mern Stack Development Company
Getting Started
To get started with the MERN stack, you'll need to set up both the back end and front end. The back end will be implemented with MongoDB, Node, and Express, while the front end will be built with React. You'll need to create a MERN stack project, which will have a client (front end) and a server (back end).
To start, you'll need to set up the back end by installing MongoDB, Node, and Express. Once you have the back end set up, you can start working on the front end using React.
Here's a brief overview of the technologies you'll be working with:
- MongoDB: A NoSQL database that stores data in JSON-like documents.
- Express: A web application framework for Node.js that simplifies the process of constructing internet programs and APIs.
- React: A JavaScript library for building consumer interfaces that allows developers to create reusable UI components.
- Node.js: A JavaScript runtime environment that authorizes you to run JavaScript code outside of an internet browser.
Before diving into the MERN stack, it's essential to have a strong understanding of JavaScript fundamentals. Many developers struggle with the MERN stack because they lack a solid grasp of JavaScript.
To become a proficient MERN stack developer, you'll need to invest time in learning the fundamentals of JavaScript, as well as the specific technologies used in the MERN stack. With practice and dedication, you can become a full-stack developer in high demand.
Additional reading: Mean Stack Developer
Setup
Setting up a MERN stack project involves several steps. First, ensure you have Node.js and MongoDB installed on your machine. You can download them from their official websites.
To start, create a new project directory and initialize a new Node.js project by running `npm init -y`. This will create a `package.json` file.
Additional reading: Next Js Drizzle Supabase
Install the necessary dependencies for your project, including Express.js and Mongoose. You can do this by running the command `$ npm i express mongoose body-parser` in your terminal.
Here's a list of the dependencies you'll need to install:
- body-parser: Allows us to get the data throughout the request
- express: Is our main framework
- mongoose: Is used to connect and interact with MongoDB
Next, you'll need to set up a database. You can use MongoDB Atlas to create a new database cluster. Create a new user and enable access to your cluster from your local development environment.
To optimize database performance, consider implementing indexes on frequently queried fields, sharding data across multiple servers, and utilizing caching mechanisms like Redis or in-memory caching.
Once you have your dependencies installed and your database set up, you can start building your MERN stack project.
A unique perspective: Create Responsive Website Tutorial
Backend Development
Backend development is where the magic happens. It's the kitchen in a restaurant, where all the behind-the-scenes work takes place, including the server, applications, and databases that process information for the frontend.
The MERN stack's backend is composed of three main bits: Express, a Node.js server, and a database. Express.js is a lightweight, flexible framework for Node.js that makes building web applications and REST APIs a breeze.
Take a look at this: Next Js 14 Tutorial
To get started with backend development, you'll need to set up a server with Express.js and Node.js. This involves installing dependencies like Express, Mongoose, and Body-Parser, and configuring them to work together.
Here are some key components of a backend setup:
- Express: A helper tool for Node.js that streamlines REST API development
- Mongoose: A library used to connect and interact with MongoDB
- Body-Parser: A middleware that allows you to get data throughout the request
As you build your backend, you'll use API endpoints to communicate between the frontend and backend. API endpoints are like the waiters in a restaurant, taking requests from the frontend and fetching or updating data in the backend.
Install Node.js
Installing Node.js is a straightforward process. Click on the Node.js website to download the installer for your operating system. Follow the installation instructions to install it on your device.
You can download Node.js from its official website. Ensure you download the correct version for your operating system.
To get started with Node.js, you'll need to create a new project directory. Open your terminal or command prompt and create a new directory for your project. This will serve as the foundation for your backend development.
Here's an interesting read: Next Js Stack
Here are the basic steps to get started with Node.js:
- Download the Node.js installer from the official website.
- Follow the installation instructions to install Node.js on your device.
Once you've installed Node.js, you can initialize a new project by running npm init -y in your project directory. This will create a package.json file.
Node.js Backend Development
Node.js Backend Development is a crucial part of the MERN stack, and it's where all the behind-the-scenes work happens, like the kitchen in a restaurant. It includes the server, applications, and databases that work together to process the information you see on the frontend.
Node.js is a powerful JavaScript runtime that allows for the development of scalable server-side applications, making it a popular choice for backend development. Express.js is a lightweight, flexible framework for Node.js, designed to build web applications and REST APIs with ease and efficiency.
To set up a Node.js backend, you'll need to create a server with Express.js and Node.js. This can be done by initializing the server and creating routes for your application. You'll also need to install dependencies such as body-parser and mongoose to handle different types of data.
Check this out: Mean Stack Development Company
The terms POST, PUT, DELETE, and GET are types of requests used in REST APIs, similar to how you would place an order in a restaurant. These requests are used to create, update, delete, and retrieve data, respectively.
Here are some key components of a Node.js backend:
- Server: Handles incoming requests and sends responses
- Applications: Run on the server and perform tasks
- Databases: Store and retrieve data
- Express.js: A framework for building web applications and REST APIs
- Node.js: A JavaScript runtime for server-side development
By understanding these components and how they work together, you'll be well on your way to building a robust Node.js backend for your MERN stack application.
Frontend Development
Frontend Development is a crucial part of building a MERN stack application. To start, you'll need to create a new React.js app using the Create React App command in your terminal. This will set up a basic project structure for you to work with.
You'll also need to set up a proxy to forward API requests to your Express.js server. This involves opening the package.json file in your client directory and adding a proxy setting.
To fetch data from your backend, you can use the fetch API or libraries like Axios in your React components. This will allow you to send requests to your Express.js server and retrieve data to display on the frontend.
For another approach, see: How to Deploy Mern App on Netlify
Here are the basic steps to get started with frontend development in a MERN stack application:
Learn HTML, JavaScript, and CSS Basics
Learning HTML, JavaScript, and CSS basics is like having the right ingredients for a recipe. You don't need to master everything at once, just the essential ingredients that make your web project come to life.
Understanding JavaScript is key, as it's used heavily in the MERN stack. You'll use JavaScript day-to-day when creating full-stack MERN apps.
Learning the basics of JavaScript is like having a food processor in your kitchen that makes coding easier and cleaner. It's a natural first step to learn, and it's not about mastering everything at once, but rather focusing on the essentials.
To get started, focus on understanding the main things you'll use day-to-day when creating full-stack MERN apps. This will give you a solid foundation to build on.
Recommended read: Mern Full Form
Building the Frontend
To start building your frontend, you'll need to create a new React.js app using Create React App. Run the command `create-react-app` in your terminal to get started.
Check this out: How to Deploy Mern App for Free
The next step is to create a proxy setting in your package.json file to forward API requests to your Express.js server. This will allow your frontend to communicate with your backend.
Inside your React components, you'll use the fetch API or libraries like Axios to fetch data from your Express.js backend.
Here are some key components you'll need to create:
- Header: A navigation bar at the top of the screen
- Index: Lists all of the available blog posts
- New: Form which allows user to create new blog post
- Single: Displays a single blog post based on the id
- Edit: Form which allows user to update blog post based on id
These components will be used to display data fetched from your Express.js backend.
To create these components, you'll need to create a components folder inside your src directory. Each component will have its own .js file inside the components folder.
Here's a list of the components you'll need to create:
- Header
- Index
- New
- Single
- Edit
You'll also need to use a library like Axios to make HTTP calls to your API endpoints.
Remember to set up routes in your React.js application using a library like React Router. This will allow you to render different components based on the URL.
Chat Application
A chat application is a fantastic project to tackle in frontend development. Real-time chat rooms, private messaging, and user profiles are all essential features to include.
To achieve this functionality, you'll want to learn about WebSockets with Node.js for real-time communication. This is a powerful tool for creating instant messaging systems.
You'll also need to store messages, which is where MongoDB comes in handy. This NoSQL database is perfect for storing large amounts of data in a flexible and scalable way.
And of course, you'll want to make sure your chat application has a dynamic and user-friendly interface. That's where React comes in, allowing you to easily update your UI in real-time.
Here are some key technologies to learn for a chat application:
- WebSockets with Node.js for real-time communication
- MongoDB for message storage
- React for dynamic UI updates
Database Setup
To set up your database for a MERN stack application, you'll want to start with MongoDB Atlas, a cloud-based database service that simplifies deploying and managing MongoDB databases. You can initiate a new project on the Atlas dashboard and create a new database cluster using the free plan.
You might like: New Relic Tutorial
To create a user and enable access to your cluster, follow the security quickstart on the Atlas dashboard. This will allow you to create a user and enable access to any network that needs to read and write data to your cluster. You'll also need to add your local development environment and IP address to your database access list.
Some key features of MongoDB Atlas include:
- Multi-cloud database service
- Simplifies deploying and managing MongoDB databases
- Free plan available
You can also use MongoDB's advanced querying techniques, such as aggregation pipelines, to perform complex manipulations and transformations on data sets directly in the database. This includes document grouping, filtering, transformation, and the calculation of aggregate values.
To optimize database performance, consider implementing indexes on frequently queried fields, using sharding to distribute data across multiple servers, and leveraging caching mechanisms like Redis or in-memory caching to reduce database load. You can also craft efficient queries and use MongoDB's query planner to analyze and improve query execution plans. Additionally, designing an optimal document structure by embedding related data and avoiding complex joins can improve performance.
Expand your knowledge: Nextjs Mongodb
API Development
API Development is a crucial part of building a robust MERN stack application. It's like having a messenger between the frontend and backend, facilitating communication and data exchange.
The terms POST, PUT, DELETE, and GET are types of requests used in REST APIs, which are like waiters in a restaurant, taking requests from the frontend, fetching or updating data in the backend, and returning responses.
To create API endpoints, you'll need to use a framework like Express, which is a helper tool for Node.js, making building certain parts of your house (or in this case, your API) easier and faster.
API endpoints are used to communicate between the frontend and backend, and they're created on the server, providing a way for the frontend to interact with it. An API (or Application Program Interface) will be created on our server which will provide "endpoints" where our front-end application can interact with it.
Discover more: Tutorial Building Useful Nextjs Tool
To handle different types of data, such as JSON, URL-encoded, and more, you'll need to install a package like cors and use a module such as body-parser or the express.json() method as a middleware in your backend application.
Here are the four types of requests used in REST APIs:
- POST: Used to create new data.
- PUT: Used to update existing data.
- DELETE: Used to remove data.
- GET: Used to retrieve data.
By using Express and Node.js, you can create a robust backend for your MERN stack application, and by following these steps, you'll be well on your way to building a successful API.
Testing and Deployment
Testing and deployment are crucial steps in bringing your MERN stack application to life. Integration testing checks how different parts of your application work together, ensuring all components interact correctly.
You can use Jest or Mocha, combined with tools like Chai for assertions, to ensure your application's components or services interact correctly. For a more comprehensive test, End-to-End (E2E) testing simulates real user scenarios, ensuring the entire application functions smoothly together. Popular choices for E2E testing in the MERN stack include Cypress or Selenium.
Once you've tested your application, it's time to deploy it. Deployment is the process of taking your code and making it operational on a live environment where users can interact with it. In the context of the MERN stack, deployment involves careful planning, choosing the right hosting solutions, and automating the process as much as possible.
Integration Testing
Integration testing is a crucial step in ensuring your application works seamlessly. It checks how different parts of your application work together.
For the MERN stack, Jest or Mocha can be used in combination with other tools like Chai for assertions. This ensures that different components or services interact correctly.
You might still use Jest or Mocha for integration testing, even if you're using a different testing framework.
End-to-End Testing
End-to-End Testing is crucial for a MERN stack application. For this, Cypress or Selenium are popular choices that simulate real user scenarios.
They ensure the entire application functions smoothly together. This includes the front end in React and the back end with Express and Node.js.
These tools are essential for catching bugs and issues that might not be apparent through other testing methods. They provide a comprehensive view of the application's functionality.
By using Cypress or Selenium, developers can test their application's entire workflow, from user input to server response. This helps ensure a seamless user experience.
For instance, with Cypress, you can automate tasks like adding, deleting, and editing books in a book list application. This saves time and reduces the likelihood of human error.
Deployment Strategies
Deployment strategies for MERN applications can be complex, but they often involve containerization with Docker, utilization of cloud services, and CI/CD pipelines.
Containerization with Docker provides a way to encapsulate application components into containers and separate them from the infrastructure, enabling quicker software delivery and ensuring consistency across different environments. This allows developers to package their MERN applications and their dependencies as containers and enables seamless deployment across various platforms.
You might like: Nextjs Prisma Docker
Cloud services like AWS, Google Cloud, and Azure offer scalable infrastructure and simplified resources for hosting and deploying MERN applications, facilitating the flow of user data from the frontend client to the provider’s system (server), and back.
CI/CD pipelines, such as those offered by Jenkins, CircleCI, GitHub Actions, or GitLab CI/CD, automate the different stages of developing MERN applications, including building, testing, and deploying, which helps maintain code quality, reduce deployment errors, and enable rapid iteration.
A combination of these strategies ensures improved performance, reliability, and ease of maintenance, but the specific strategies will depend on the project requirements.
A unique perspective: How to Use Google Cloud Platform
Final Thoughts
As you wrap up your testing and deployment process, it's essential to remember the foundation of your application is built on a robust stack.
The MERN stack is a powerful combination of MongoDB, Express js, and Node js, which work together to create a server that provides API endpoints for your React js application to fetch data from.
With a solid understanding of how your application is structured, you can now focus on fine-tuning the details, such as ensuring seamless data exchange between your front-end and back-end.
By leveraging the strengths of each technology, you can build a scalable and efficient application that meets the needs of your users.
The key to a successful deployment is to have a clear understanding of how your application works, and the MERN stack provides a great foundation for this.
Learning Resources
The freeCodeCamp curriculum is one of the best places to learn how to code for free. It's super in-depth and covers a lot of the topics you'll need to master the MERN stack.
You can find a ton of free courses on the freeCodeCamp YouTube channel, including the MERN stack book project, which is an excellent one for beginners.
Full Stack open is another good resource, although it doesn't teach MongoDB, it does cover SQL, which is equally as useful.
Here are some free resources to get you started:
- The freeCodeCamp curriculum
- The freeCodeCamp YouTube channel
- Full Stack open
- My MERN stack hotel booking app project on YouTube
Sources
- https://www.geeksforgeeks.org/how-to-use-mern-stack-a-complete-guide/
- https://www.freecodecamp.org/news/mern-stack-roadmap-what-you-need-to-know-to-build-full-stack-apps/
- https://www.iamtimsmith.com/blog/what-is-the-mern-stack-and-how-does-it-work
- https://blog.nextideatech.com/how-to-get-started-with-the-mern-stack-a-comprehensive-guide/
- https://blog.logrocket.com/mern-stack-tutorial/
Featured Images: pexels.com