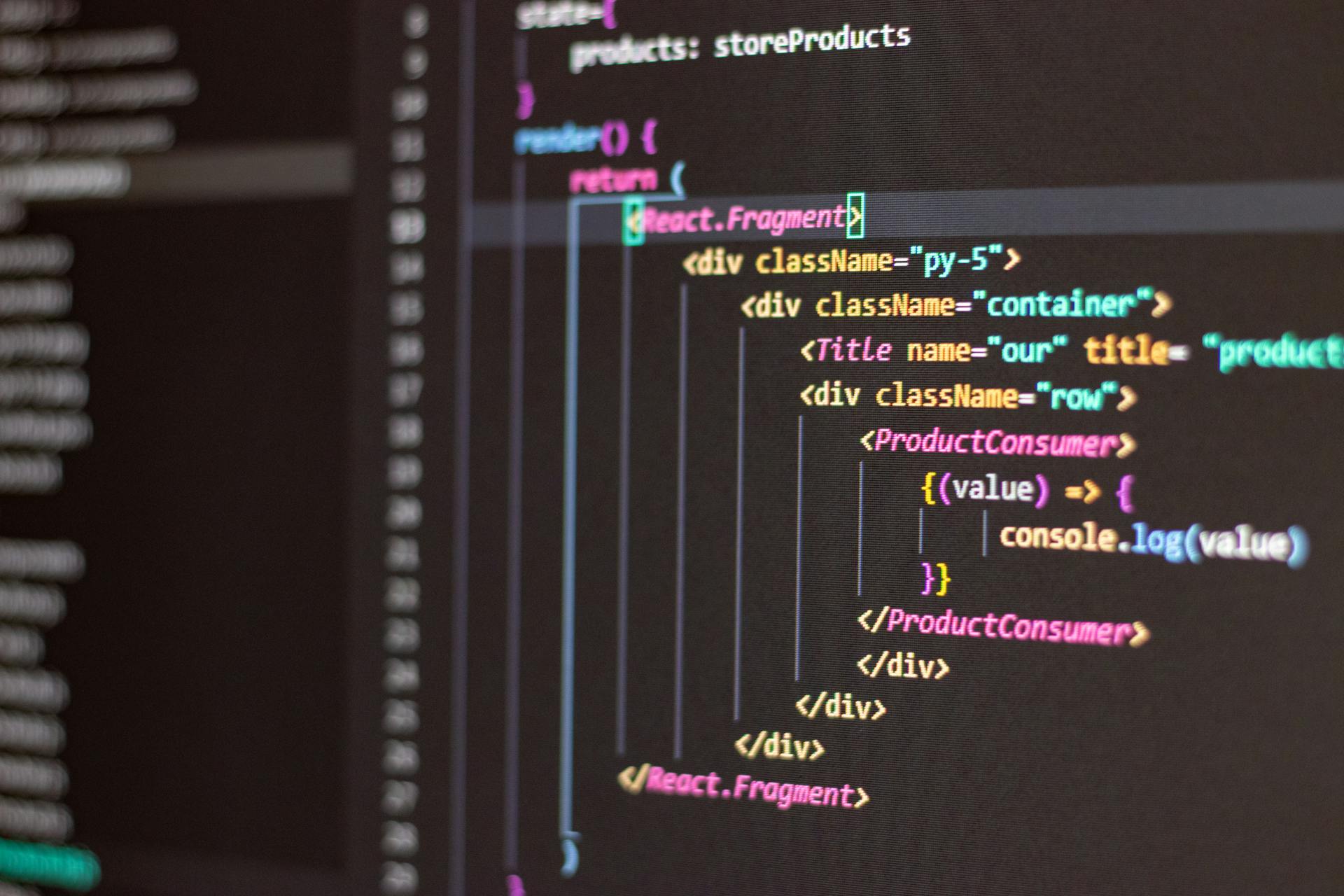
Next.js 14 is a powerful framework for building server-rendered, statically generated, and performance-optimized web applications.
It's built on top of React and provides a lot of built-in features like automatic code splitting, CSS-in-JS support, and API routes.
To get started with Next.js 14, you'll need to have Node.js installed on your machine.
Next.js 14 introduces a new feature called "Dynamic Imports" which allows you to import components and modules dynamically at runtime.
Create Project and Add Dependencies
To create a Next.js project, you can launch the setup wizard with `npx create-next-app`. This will guide you through the process of setting up a new project. You'll need to hit 'y' or 'n' a few times to complete the wizard.
You can also create a new Next.js project using the command `npx create-next-app` or by following the instructions in the Vercel documentation. Next.js provides several features to help you organize your project while following routing conventions.
To add dependencies to your project, you can use one of the following commands: `npm install @neondatabase/serverless` or `neon serverless driver postgres.js node-postgres`. This will install the necessary dependencies for your project.
Here are the steps to create a new Next.js project and add dependencies:
- Create a new Next.js project using `npx create-next-app` or by following the instructions in the Vercel documentation.
- Add project dependencies using one of the following commands: `npm install @neondatabase/serverless` or `neon serverless driver postgres.js node-postgres`.
You can also get started with Next.js Edge Functions and Neon by following the instructions in the Vercel documentation. This will guide you through the process of setting up a new project and adding dependencies.
File Structure
In a Next.js 14 project, it's essential to organize your files in a structured way. The top-down approach is a great way to think about structuring your App Router applications, starting with the general path structure and working your way down.
A typical page will look like this: app/page.tsx, which will contain its own content. You can also create a shared layout file, app/layout.tsx, to apply to all pages.
Recommended read: Next Js 14 Layout Async
The layout will contain common elements that should be present on all pages, such as a header or navigation menu. However, you may need to create a Route Group with its own nested layout to apply to specific pages that require different elements, like the Home icon in this case.
For your interest: Nextjs Pages
Project Organization
You can think of structuring your App Router application from a top-down approach, starting with your general path structure and working your way down.
A page will typically have a layout, which can be shared across multiple pages, just like how the three pages in the example all looked the same minus their content. The layout will apply to all pages, and each page file will contain its own content.
You can create a Layout file, like app/layout.tsx, to define the shared layout. This approach makes it easy to reuse code and keep your project organized.
For example, the author of the article chose to create four files: app/layout.tsx, app/page.tsx, app/projects/page.tsx, and app/about/page.tsx, to implement this approach.
Check this out: Next Js 14 Redirect to Another Page Loading Indicator
To set up the project, you'll need to create a new Next.js project using the command npx create-next-app. This will launch the setup wizard, which you can complete by hitting y or n a few times.
Next.js provides several features to help you organize your project while following routing conventions, such as the ability to create a new directory for your components and create files and directories as needed.
You can create an app/(subpages)/components directory and a quick header only for the subpages, as shown in the example. This will help you keep your components organized and make it easier to reuse them across your project.
Suggestion: Next Js Components
What Are?
NextJS API routes are serverless functions that handle incoming HTTP requests and return responses. They live in the pages/api directory of your NextJS project.
You can create these routes easily, and they deploy with your NextJS app altogether, making it a more straightforward way to build your API endpoints.
Traditionally, we would need to set up a separate server on a new port, but now you can achieve the same server functionality without doing all that.
See what others are reading: How to Use Reducer Api in Next Js 14
Routing and Pages
In Next.js 14, routing is file-system-based, where URL paths are determined by files and folders in the codebase. This is crucial for proper routing functionality.
To create a route, you need to create a folder and a page file inside it. For example, creating a src/app folder and a page.tsx file inside it will represent the route. The page.tsx file should define a simple React component, such as a "Home" page.
There are two methods for fetching data using server-side requests in Next.js Pages Router: getServerSideProps and getStaticProps. getServerSideProps fetches data at runtime, while getStaticProps pre-renders pages at build time.
Next.js API Routes follow a simple structure:
- File location: API routes are placed in the pages/api directory.
- File naming: The file name becomes the route path (e.g., pages/api/users.js becomes /api/users).
- Default export: Each file exports a default function that handles the API requests.
The handler function receives two parameters: req and res. req is an instance of http.IncomingMessage, plus some pre-built middlewares, while res is an instance of http.ServerResponse, plus some helper functions.
Next.js supports dynamic routes, which can handle complex applications with hundreds of routes. To create dynamic routes, you can create a folder with a dynamic segment in the name, such as [productId]. This will allow you to handle routes like /products/1 and /products/2.
Dynamic routes can be nested, allowing you to handle routes like /products/1/reviews/1. To create nested dynamic routes, you can create a folder with a dynamic segment in the name, such as [productId]/reviews/[reviewId].
Next.js also supports dynamic API routes, allowing you to create flexible endpoints. For example, you can create a user endpoint that takes in a user's id like this pages/api/users/[id].js.
Here's a summary of the routing structure:
Note that the file location and file naming conventions apply to both Pages and API Routes, while the default export applies to both page components and handler functions.
Advanced Routing
Advanced Routing in Next.js 14 is a game-changer for complex applications. Next.js supports dynamic routes, allowing you to create flexible endpoints.
You can handle different HTTP methods within the same API route, and even create nested dynamic routes by having dynamic segments in the folder names. This is achieved by creating a folder structure that reflects the route you want to create.
To implement nested dynamic routes, follow these steps: create a folder with a dynamic segment, like [productId], and then create another folder within it with another dynamic segment, like [reviewId]. This will create a route like /products/productId/reviews/reviewId. You can also use catch-all segments to handle multiple routes with a single file, by using a folder name like [...slug].
A unique perspective: Nextjs Dynamic
Handling Non-Matching
Handling Non-Matching Routes is a breeze with Next.js. Next.js automatically responds with a 404 Not Found response if you enter a URL that cannot map to a file within the app folder.
For example, if you try to access /dashboard, Next.js will take care of it for you, without requiring you to explicitly handle non-matching routes.
This means you can focus on building your app's routing without worrying about edge cases like non-matching routes.
Advanced Routing with Src/App Directory
You can master Next.js 14 routing with the new src/app directory. This feature allows you to create a hierarchical structure within your application, making it easier to manage complex routes.
To implement nested routes, create a src/app/blog folder and inside it, create a page.tsx file for the main blog page. This will render the My blog page when you navigate to localhost:3000/blog.
By creating a nested folder structure, Next.js automatically routes the files accordingly, simplifying the process of creating nested routes and enhancing the organization and structure of your application.
To create nested dynamic routes, create a src/app/products/[productId]/reviews folder and within it, create a new folder named [reviewId]. This structure takes you to the route "/products/productId/reviews", where you can define a React component to display both the productId and the reviewId.
You can create dynamic routes to handle complex applications with hundreds of routes. To do this, create a products folder and within it, create a new folder named [productId]. This will create a dynamic route segment that can accommodate values like 1, 2, 3, and so on.
You might like: Next Js Fetch Data save in Context and Next Route
Dynamic routes are beneficial when implementing the list-detail pattern in any UI application. By understanding how to create dynamic routes in Next.js, you can build flexible and scalable applications that adapt to varying user interactions.
Here are some key benefits of using the src/app directory for advanced routing:
- Improved organization: The src/app directory allows you to create a hierarchical structure within your application, making it easier to manage complex routes.
- Simplified route creation: Next.js automatically routes the files accordingly, simplifying the process of creating nested routes.
- Flexibility: Dynamic routes can accommodate values like 1, 2, 3, and so on, making it easier to handle complex applications with hundreds of routes.
By leveraging the src/app directory and advanced routing features in Next.js 14, you can build scalable and flexible applications that meet the needs of your users.
Middleware Implementation
Middleware Implementation is a powerful tool in Next.js routing. You can use middleware to process requests before they reach your route handler.
Middleware can be used to authenticate and authorize requests, for example. This is especially useful when creating dynamic API routes, like the user endpoint we discussed earlier.
By using middleware, you can add an extra layer of security to your routes, making sure only authorized requests can reach your handler function.
You can implement middleware by adding a middleware function to your route handler. This function will be executed before the handler function, allowing you to process the request and modify its behavior as needed.
Middleware implementation is a flexible and scalable way to handle requests in Next.js. It allows you to separate concerns and keep your code organized, making it easier to maintain and update your routes.
Expand your knowledge: Middleware Next Js
Featured Images: pexels.com