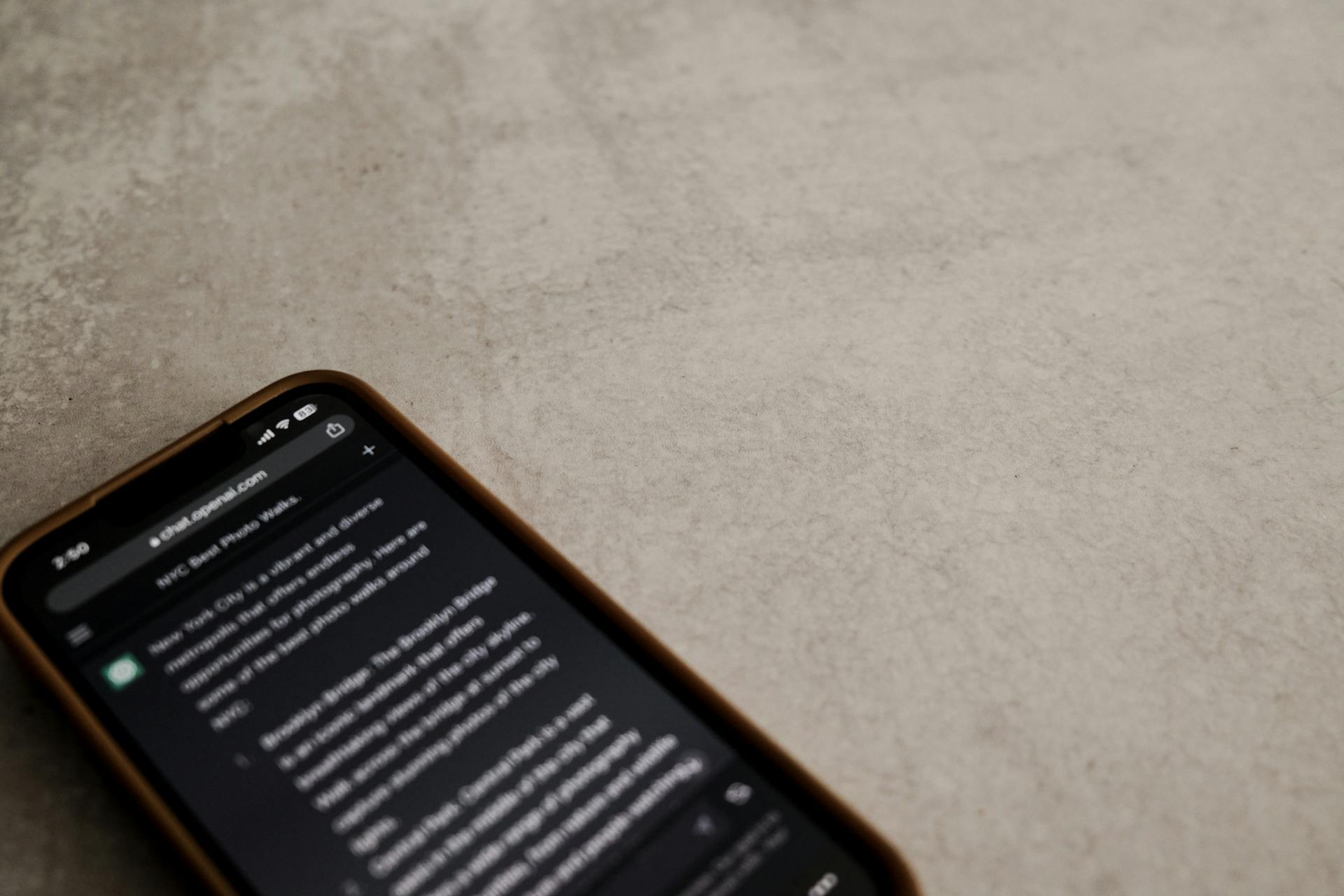
Next.js is an open-source React-based framework for building server-rendered, statically generated, and performance-optimized websites and applications. It's a great choice for building a chatbot.
To get started with Next.js, you'll need to install it using npm or yarn. This can be done by running `npx create-next-app my-chatbot` in your terminal.
Next.js provides a lot of features out of the box, including internationalized routing and API routes. This means you can easily create a chatbot that can understand and respond to multiple languages.
In the next section, we'll dive deeper into setting up your chatbot's API routes.
Readers also liked: Next Js App
Getting Started
To get started with building a Next.js chatbot, you'll need to meet the prerequisites, which include having Node.js 18 or later installed on your computer. This will ensure that your project is set up with the latest features and tools.
To create a Clerk account, an Upstash account, an OpenAI account, and a Vercel account, simply sign up for each service on their respective websites. These accounts will provide you with the necessary tools and resources to build and deploy your chatbot.
Here are the essential configurations you'll need to set up for your Next.js application:
- TypeScript: Provides robust type-checking, which helps catch errors early in the development process.
- ESLint: Ensures code quality and consistency across your project.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development.
- src/ directory: Organizes your code better by separating source files from configuration files.
- App Router: Simplifies routing and enhances the overall structure of your application.
Running the Application
To run your chatbot application, you need to start the development server.
This is a crucial step to see your application in action. Open your terminal and execute the command to start the server.
Once the server is running, you can access your chatbot interface by navigating to http://localhost:3000 in your web browser.
Here, you will find the chat page where you can interact with your chatbot. Simply type your messages into the input field, and the chatbot will respond in real-time, providing a seamless conversational experience.
A different take: Start Next Js App
Prerequisites
To get started with this project, you'll need to have a few things in place. Node.js 18 or later is a must-have, as it's the foundation upon which everything else will be built.
You'll also need to create accounts with several services: Clerk, Upstash, OpenAI, and Vercel. These accounts will provide the necessary tools and infrastructure to bring your project to life.
Having these prerequisites covered will ensure a smooth and successful journey as you work on your project.
Project Setup
To set up a Next.js chatbot project, start by ensuring you have Node.js installed on your machine. You can download it from nodejs.org.
First, create a new Next.js project using the command `npx create-next-app my-chatbot` or `npx create-next-app ollama-nextjs-chatbot` or `npx create-next-app chatbot-app`. This will initialize a new Next.js project in a directory with the specified name.
Next, navigate into your project directory and install the necessary dependencies for integrating Llama.cpp and ModelFusion by running the command `npm install` or `yarn install`.
Your project structure should look like this:
- `pages/`
- `public/`
- `styles/`
- `components/`
- `models/`
- `services/`
- `utils/`
You can also use the following command to create a new Next.js project: `npx create-next-app nextjs-chatbot`. This will create a project with a folder structure such as this:
* `nextjs-chatbot/`
+ `components/`
+ `models/`
+ `services/`
+ `utils/`
+ `pages/`
+ `public/`
+ `styles/`
After setting up your project, you can start the development server by running the command `npm run dev` or `yarn dev`. This will create a new NEXT.js project called chatbot-app and start the development server. You can access your application at http://localhost:3000.
In your project directory, create a file called `.env.local` to store your environment variables.
Recommended read: Next Js Development Company
Next.js Chatbot Project
To start building your Next.js chatbot project, you'll need to have Node.js installed on your machine. You can download it from nodejs.org. Once Node.js is set up, you can create a new Next.js application using the command "npx create-next-app my-chatbot" in your terminal.
Next.js will serve as the backbone for both the frontend and the API routes of your chatbot. To create a Next.js app, open your terminal, cd into the directory you’d like to create the app in, and run the command "npx create-next-app my-chatbot". This will configure Tailwind CSS with the project.
After setting up your project, navigate into your project directory and install the necessary dependencies for integrating Llama.cpp and ModelFusion. You can do this by running the command "npm install llama-cpp model-fusion" in your terminal. Your project structure should look like this:
For your interest: Next Js Npm Install
Configure Your Application
Configuring your Next.js chatbot application is a crucial step in setting it up for success. You'll be prompted to configure various aspects of your application during the setup process.
A fresh viewpoint: Next Js Spa
TypeScript is a recommended setting for our chatbot project, providing robust type-checking that helps catch errors early in the development process.
ESLint is another essential configuration, ensuring code quality and consistency across your project. This means you can focus on building a great chatbot without worrying about messy code.
Tailwind CSS is a utility-first CSS framework that allows for rapid UI development. This is a game-changer for building a visually appealing chatbot.
The src/ directory is a great way to organize your code, separating source files from configuration files. This keeps your project tidy and makes it easier to find what you need.
The App Router simplifies routing and enhances the overall structure of your application. This makes it easier to build and maintain a complex chatbot.
Here are the recommended configurations for our chatbot project at a glance:
- TypeScript: Provides robust type-checking
- ESLint: Ensures code quality and consistency
- Tailwind CSS: A utility-first CSS framework
- src/ directory: Organizes your code better
- App Router: Simplifies routing and enhances the overall structure
Prerequisites
To get started with building a Next.js chatbot, you'll need to meet some basic prerequisites.
Node.js 18 or later is the minimum requirement to run your project.
Having a Clerk account is essential for managing authentication and user sessions in your chatbot.
You'll also need to sign up for an Upstash account to handle message queuing and storage.
An OpenAI account is necessary for integrating AI-powered features into your chatbot.
Lastly, a Vercel account will help you deploy and manage your chatbot's frontend.
Here are all the prerequisites summarized in a list:
- Node.js 18 or later
- Clerk account
- Upstash account
- OpenAI account
- Vercel account
API Endpoints
API Endpoints are a crucial part of building a Next.js chatbot, and in this section, we'll explore the different types of endpoints you can create.
You can create an endpoint to retrieve a user's chat history by creating a file named route.ts in the app/api/chat/history directory. This endpoint retrieves up to 100 chat messages for the authenticated user and returns them as a JSON response.
If the user session is not found, the endpoint responds with a 403 status, preventing unauthorized access. This is a key feature to ensure the security of your chatbot.
Take a look at this: Next Js Cookies
To handle user chat interactions with the latest context, you'll need to create an endpoint in the app/api/chat directory. This endpoint fetches the user session and chat messages, verifies the user's session, and adds relevant context dynamically.
By using the aiUseChatAdapter function, the endpoint returns a streaming response, allowing for real-time updates and interactions. This is a powerful feature that enables seamless communication between users and your chatbot.
To upload a PDF file and add its vector embeddings to the vector index, you'll create a POST method in the app/api/upsert directory. This endpoint fetches the user session and uploaded file, validates them, and converts the file to a Blob.
The endpoint then adds the file to the vector index under the user's namespace, allowing for efficient and secure storage of user data. This is a critical feature for building a robust and scalable chatbot.
On a similar theme: Nextjs Usecontext
Building the Interface
Building the interface for your Next.js chatbot is a crucial step in creating an engaging user experience. To start, you'll need to import React hooks for managing state, conversation, and toast elements.
You can do this by replacing the existing code in the app/page.tsx file with the code that begins with importing the necessary hooks. This code also fetches the chat history for the user when the component is mounted and their logged in state changes.
To add an input for the user to submit their prompt and upload a PDF file, you'll need to define an input element that calls the /api/chat endpoint when the user submits their prompt. You'll also need to define a TooltipProvider component that invokes the PDF file uploader input when the user clicks the upload icon.
Remember to import the CSS module in your Chat.js component to make your chat interface visually appealing. You can create a Chat.module.css file in the components folder and define styles for your chat interface.
On a similar theme: Apply Css Nextjs
Implementation
To implement the chat interface, start by installing the pnpm package manager. This will be your primary package manager for the project.
Next, create an API key in the OpenAI Dashboard and copy it. You'll need this key to authenticate your API requests.
Set the environment variable by using the export command in the terminal or by creating a new file called .env.local and pasting the API key there. This will allow you to access the API key in your code.
Install the dependencies by running pnpm install. This will download all the necessary packages for the project.
Run the development server by running pnpm dev. This will start the server and allow you to test the chat interface.
To implement the Vercel AI SDK in a Next.js app, you'll need to add an App route file and a Page file.
Broaden your view: Install Next Js 13
Building the Interface
To start building the chatbot interface, you'll need to open the app/page.tsx file and replace the existing code with the code from the example.
The code begins by importing React hooks for managing the state of the user, conversation, and invoke toast elements. As the component is mounted (and if the user's logged in state changes), the chat history for the user is fetched.
Additional reading: Using State in Next Js
You'll also need to create a signed in and out state of the application for the user, which can be done by adding the code that defines a signed in and out state of the application for the user.
If the user is signed in, it displays their image via UserButton component with additional actions, and a default loading state of their chat history. If the user is signed out, it shows a Sign in to use Nerdcoach button.
To add an input for the user to submit their prompt and upload PDF file, you'll need to add the code that defines an input element which after the user submits their prompt, calls the /api/chat endpoint.
You'll also need to add a TooltipProvider component which invokes the PDF file uploader input if a user clicks the upload icon.
To make the chatbot visually appealing, you can create a Chat.module.css file in the components folder and define styles for your chat interface.
You can create a new file called Chat.js inside the components folder to create a chatbot interface, and use the useState hook to manage the chat messages and user input.
See what others are reading: File Upload Next Js Supabase
When the user types a message and clicks the “Send” button, you'll send the user’s input to Dialogflow for processing and receive a response.
To begin the design of the interface, you would remove all the content of the index.js file which is found inside the pages folder, and replace it with the content below.
The getBotAnswer helper function is then used to get the answer from the bot and update the UI.
Sources
- https://blog.saeloun.com/2023/07/13/building-chatbot-in-next-js-using-vercel-ai-sdk/
- https://www.restack.io/p/using-nextjs-with-ai-applications-answer-building-chatbots-cat-ai
- https://upstash.com/blog/rag-chatbot-upstash-openai-clerk-nextjs
- https://clouddevs.com/next/real-time-chatbot-with-dialogflow/
- https://devpress.csdn.net/cms/62f4b88b7e6682346618882f.html
Featured Images: pexels.com