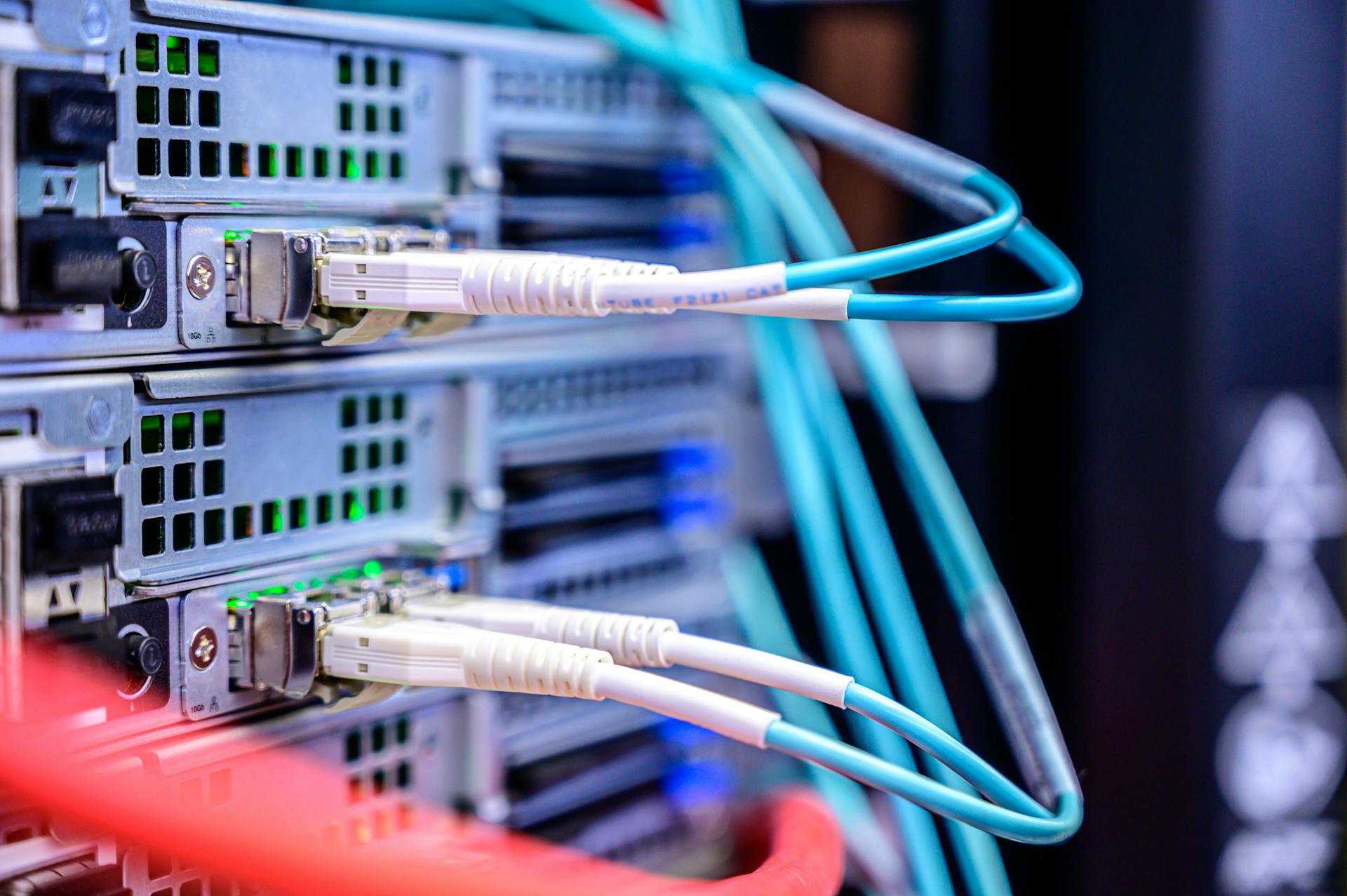
To set up a Next.js Discord app, you'll need to create a new Next.js project using the `npx create-next-app` command. This will give you a basic project structure to work with.
First, install the Discord API library using npm or yarn, `npm install discord.js` or `yarn add discord.js`. This library provides a simple way to interact with the Discord API.
Next, create a new file in your project directory, `main.js`, and add the necessary code to initialize the Discord bot and set up the event listeners. For example, you can use the `client.on('ready', () => { ... });` method to listen for the bot's ready event.
Before you can use the Discord API, you need to register your bot on the Discord Developer Portal and obtain an API token. This token will be used to authenticate your bot and make API requests.
You might like: Nextjs Stripe
Setup and Configuration
To set up Discord in your Next.js app, you'll need to create a server list by filtering channels with their extra data and getting all distinct server names and images from that.
Suggestion: Next Js Call Api on the Server Example
Discord uses servers, channels, and direct messages to organize communication. In Next.js, you can replicate this structure by adding a server key to the extraData object when creating a channel. This allows you to filter channels within a specific server.
You can also add a category field to the extraData object to group channels inside a server.
Discover more: Nextjs Pathname Server Component
Setup Data
In Discord, we can join servers and organize channels into categories. We can also have direct messages with each user, independent of servers and channels.
Discord's setup is quite different from Stream Chat's, which doesn't come built-in with servers. However, we can add custom fields to a channel in the extraData object.
One custom field we can add is a server key, which we set to one of our servers when creating a channel. This allows us to filter channels within a specific server.
We can also add a category field to the extraData object to group channels inside a server easily.
By filtering channels with their extra data, we can get a list of distinct server names and images.
Recommended read: Nextjs Google Fonts
App Redirect Configuration
To configure your app to redirect to the authentication endpoint, you need to set up a redirect URL in the Discord "My Applications" page. This is where you'll specify the URL for the Discord auth callback endpoint generated by next-auth.
The redirect URL should be the same as the URL for the Discord auth callback endpoint, which is http://localhost:3000/api/auth/callback/discord. Make sure to enter this URL in the "Redirects" input under the "OAuth2" page.
You also need to create a catch all API route for auth, which will allow next-auth to handle all requests to /api/auth/*. This is done by creating a file called pages/api/auth/[…nextauth].ts and adding the necessary code.
Take a look at this: Next Js 14 Redirect to Another Page Loading Indicator
Authentication
Authentication is a crucial part of any app, and in our case, we're using next-auth to handle it.
We've configured next-auth to use our Discord app and set up our Discord app to send users back to our app after authentication.
Our app is wrapped in SessionProvider, giving us access to the current user's session with signIn, signOut, and useSession.
Passing the 'discord' string to signIn skips the page with "Sign in" buttons for each service, redirecting us directly to the Discord auth page instead.
For your interest: Discord Clone Nextjs
Context and Routing
Context and Routing is a crucial aspect of building a Next.js Discord application. Next.js allows for server-side rendering, which enables faster page loads and improved SEO.
In a Next.js Discord application, routing is handled by the Next.js Router. This allows for client-side routing, which enables users to navigate through the application without a full page reload.
The Next.js Router also supports internationalized routing, which allows for routing to be defined for multiple languages and locales. This is particularly useful for Discord applications that need to support users from different regions.
A fresh viewpoint: Framer Motion Page Transitions Nextjs Examples
Using Next.js in This Guide
Next.js is a great choice for building a Discord authentication page, thanks to its easy setup. It allows you to get started with building your application right away, and even provides a built-in development server.
One of the key benefits of using Next.js is its scalability. Next.js applications can handle large amounts of traffic, making it well-suited for building high-performance applications.
Next.js also allows for server-side rendering, which is especially useful when building an authentication page. This enables you to securely handle user credentials on the server, rather than exposing them to the client.
Here are some additional benefits of using Next.js for building a Discord authentication page:
- Easy setup: Next.js makes it easy to set up a new project.
- Scalability: Next.js applications can handle large amounts of traffic.
- Server-side rendering: Next.js allows for secure handling of user credentials on the server.
- Easy deployment: Next.js can be easily deployed to various hosting providers.
Creating a Context
We'll use a Context to handle servers and channels in a single place, giving us convenient access and central points to manage our state. This is similar to what we did in Example 4, where we created a DiscordContext to handle servers and channels.
A Context is a way to share data between different parts of our application, and it's especially useful when we have complex state that needs to be managed. We'll use a type for our state that includes functions to create a server, create a channel, and change the current server.
We can define a type for our state, as shown in Example 4, with properties for creating a server, creating a channel, and changing the current server.
You might like: Next Js Fetch Data save in Context and Next Route
Our state object will have functions to create a server, create a channel, and change the current server, which will make it easier to manage our application's state.
To create a server, we'll need to create a channel with the name and image in the extraData, as shown in Example 4. This will make sense, as servers without channels wouldn't make sense anyway.
We can create a table to summarize the functions that our state object will have:
We'll also need to create a convenience hook to access our DiscordContext, as shown in Example 4, to make it easier to use our Context in our application.
Table of Contents
Here's the "Table of Contents" section of the blog article:
We'll be covering the essential steps to build an authentication system using next-auth and Discord.
First, we'll explore what next-auth is and its role in our authentication system.
Next, we'll dive into adding authentication routes with next-auth, which will enable users to log in to our app.
A unique perspective: Nextjs Auth Providers
Configuring our Discord app to redirect to our app is also crucial, and we'll go over how to do that.
We'll also discuss using signIn to authenticate with our Discord app, which is a key feature of next-auth.
Finally, we'll cover accessing auth session data in our app, which is essential for a seamless user experience.
Here's a quick overview of the topics we'll be covering:
- What is next-auth?
- Adding authentication routes with next-auth
- Configuring our Discord app to redirect to our app
- Using signIn to authenticate with our Discord app
- Accessing auth session data in our app
Sources
- https://n3tc0rd.digitalpress.blog/nextjs-discord-login-page/
- https://bun.sh/guides/ecosystem/nextjs
- https://getstream.io/blog/discord-clone-project-setup/
- https://www.linkedin.com/posts/getstream_building-a-discord-clone-using-nextjs-tailwindcss-activity-7202354895864147968-UCgM
- https://blog.with-heart.xyz/authenticating-users-with-discord-in-a-nextjs-app
Featured Images: pexels.com