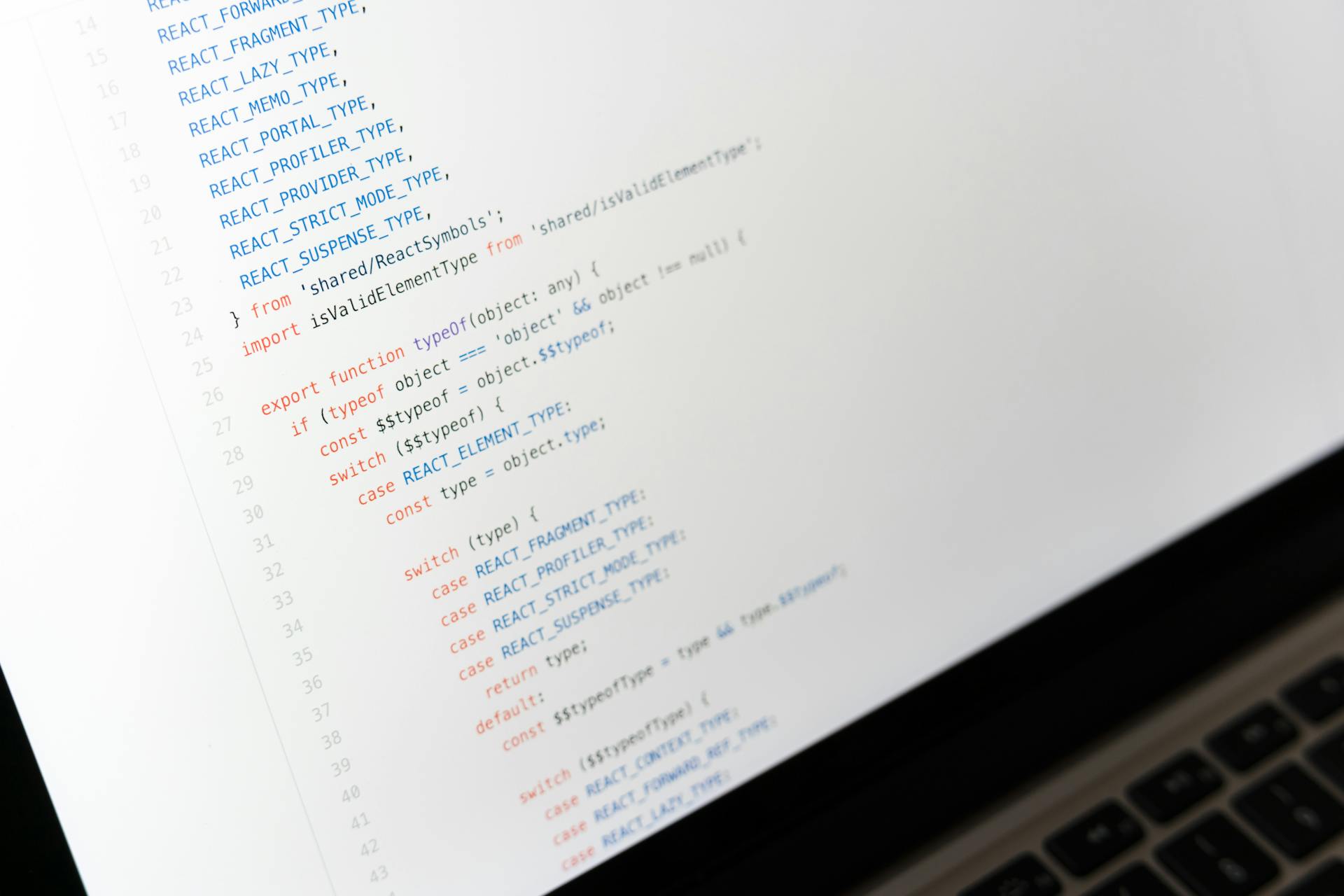
Nextjs Standalone is a server-rendered, statically generated React application that allows developers to build fast, scalable, and SEO-friendly websites and applications.
It's built on top of the popular React framework, making it easy for developers to learn and adapt to.
Nextjs Standalone comes with built-in support for internationalization, which means developers can easily handle multiple languages and regions.
This feature is especially useful for global companies that need to cater to diverse audiences.
With Nextjs Standalone, developers can also use the popular CSS-in-JS solution, styled-components, to write reusable and maintainable CSS code.
This approach eliminates the need for CSS preprocessors and reduces the complexity of CSS maintenance.
Nextjs Standalone also provides a built-in development server, which allows developers to quickly test and iterate on their applications.
This feature saves developers a significant amount of time and effort, making it easier to build and deploy modern web applications.
Recommended read: Nextjs Pathname Server Component
Server Components
Server Components are a fundamental part of Next.js Standalone, enabling you to render data on the server. By default, Next.js components are Server Components that can be server-rendered.
If this caught your attention, see: Next Js Components Folder
To add server-rendered data in your Next.js project, you need to edit a Next.js component to add a server-side operation. This can include fetching data or other server operations.
For example, in the Home component, you can add an operation that sets the value of a server-side computed variable, like this: const timeOnServer = new Date().toLocaleTimeString('en-US');.
To ensure the route is dynamically rendered, you need to import unstable_noStore from next/cache and call it within the Home component.
Here's a step-by-step guide to server-rendering data in your Next.js project:
1. Open the app/page.tsx file and add an operation that sets the value of a server-side computed variable.
2. Import unstable_noStore from next/cache and call it within the Home component to ensure the route is dynamically rendered.
3. Update the Home component in app/pages.tsx to render the server-side data.
By following these steps, you can server-render data in your Next.js project using the App Router. This is particularly useful for fetching data or performing other server operations.
Consider reading: Nextjs Google Fonts
API and Routing
Next.js provides a way to create API routes using Route Handlers. You can fetch these APIs in Client Components.
To create an API route, start by adding a new file at app/api/currentTime/route.tsx. This file holds the Route Handler for the new API endpoint.
A handler function is added to return data from the API, and it's a good practice to import the NextResponse from 'next/server' at the top of the file.
The handler function is marked as dynamic with 'force-dynamic' to ensure it's always rendered.
Here's a quick rundown of the API route setup:
- Create a new file at app/api/currentTime/route.tsx.
- Add a handler function to return data from the API.
- Mark the handler function as dynamic with 'force-dynamic'.
This API route is then fetched in a Client Component using the fetch function and the useEffect hook.
The Client Component is identified as a Client Component with the 'use client' directive.
Here's a comparison of the two files:
The Client Component renders the API response after the load is complete, and it's a great way to display dynamic data on your Next.js Standalone site.
Configuration
To configure Next.js for standalone deployment, you need to pay attention to the runtime version. Certain Next.js versions require specific Node.js versions, so you can set the engines property of your package.json file to designate a version.
This ensures that your project uses the correct Node version, which is especially important for certain Next.js versions. You can specify the required Node version in your package.json file.
You'll also want to set environment variables for Next.js, which uses them at both build time and request time. This supports both static page generation and dynamic page generation with server-side rendering. Set environment variables within the build and deploy task, as well as in the Environment variables of your Azure Static Web Apps resource.
You might like: Page Transition Framer Motion Nextjs
Configure Next.js Runtime Version
Configuring the runtime version for Next.js is crucial to ensure compatibility. Certain Next.js versions require specific Node.js versions.
To configure a specific Node version, you can set the engines property of your package.json file to designate a version. This is a straightforward process that can prevent compatibility issues.
Set Next.js Environment Variables
Next.js uses environment variables at build time and at request time, to support both static page generation and dynamic page generation with server-side rendering.
To set environment variables for Next.js, you need to set them both within the build and deploy task, and in the Environment variables of your Azure Static Web Apps resource.
Next.js environment variables are used for both static page generation and dynamic page generation with server-side rendering.
This means you should set environment variables in two places: in your build and deploy task, and in the Environment variables of your Azure Static Web Apps resource.
A fresh viewpoint: Next Js Api Call
Features and Settings
Next.js Standalone offers a range of features and settings that make it an ideal choice for developers.
You can customize the development server by using the `next.config.js` file, which allows you to configure settings like environment variables and build output.
The `next.config.js` file is a central location for managing Next.js settings, and it's where you can define custom configurations for your project.
For another approach, see: Next Js Upload File
By default, Next.js Standalone uses a development server that supports features like hot code reloading and live reloading.
Hot code reloading allows you to see changes to your code in real-time, without having to manually reload the page.
Live reloading is similar to hot code reloading, but it also reloads the entire page when changes are detected.
To enable live reloading, you can set the `target` option in your `next.config.js` file to `'serverless'`.
The `target` option determines how Next.js generates the build output, and setting it to `'serverless'` enables live reloading in development mode.
Next.js Standalone also supports internationalization (i18n) out of the box, making it easy to create multilingual applications.
You can configure i18n settings in your `next.config.js` file using the `i18n` option.
The `i18n` option allows you to specify the locale directory and other settings for your i18n configuration.
See what others are reading: Next Js 14 Redirect to Another Page Loading Indicator
Output and Deployment
Next.js automatically traces each page and its dependencies to determine the files needed for a production deployment, reducing deployment size drastically.
This feature eliminates the need for the deprecated serverless target, which can cause issues and create unnecessary duplication. Previously, you'd need to have all files from your package's dependencies installed to run next start.
Next.js uses @vercel/nft to statically analyze import, require, and fs usage to determine all files that a page might load. This is done during the next build process.
To leverage the .nft.json files emitted to the .next output directory, you can read the list of files in each trace that are relative to the .nft.json file and then copy them to your deployment location.
Here are some ways to manually copy files to your deployment location:
- Use the cp command-line tool after you next build.
- Copy the public or .next/static folders manually, after which the server.js file will serve these automatically.
To start your minimal server.js file locally, run the following command: `PORT=8080 HOSTNAME=0.0.0.0 node server.js`
Output
Output is a crucial part of the Next.js build process, and it's where the magic happens in terms of reducing deployment sizes.
Next.js will automatically trace each page and its dependencies to determine all the files needed for a production deployment, which drastically reduces the size of deployments.
This feature removes the need for the deprecated serverless target, which can cause issues and create unnecessary duplication.
During next build, Next.js uses @vercel/nft to statically analyze import, require, and fs usage to determine all files that a page might load.
Next.js' production server is also traced for its needed files and output at .next/next-server.js.nft.json.
To leverage the .nft.json files emitted to the .next output directory, you can read the list of files in each trace that are relative to the .nft.json file and then copy them to your deployment location.
Next.js can automatically create a standalone folder that copies only the necessary files for a production deployment, including select files in node_modules.
To enable this automatic copying, you can add the following configuration to your next.config.js:
```bash
module.exports = {
// ...
standalone: true,
};
```
This will create a folder at .next/standalone, which can then be deployed on its own without installing node_modules.
A minimal server.js file is also output, which can be used instead of next start.
You might enjoy: Can Amazon S3 Take in Nextjs File
To start your minimal server.js file locally, run the following command:
```bash
PORT=8080 HOSTNAME=0.0.0.0 node server.js
```
This will start the server on http://0.0.0.0:8080.
Note that there are some cases in which Next.js might fail to include required files, or might incorrectly include unused files.
In those cases, you can leverage outputFileTracingExcludes and outputFileTracingIncludes respectively in next.config.js.
Here's an example of how you can configure outputFileTracingExcludes:
```javascript
module.exports = {
// ...
outputFileTracingExcludes: {
'pages/*': ['node_modules/**/*'],
},
};
```
This will exclude all files in node_modules from the tracing process for all pages.
You can also use outputFileTracingIncludes to include specific files or directories in the tracing process.
For example:
```javascript
module.exports = {
// ...
outputFileTracingIncludes: {
'pages/index.js': ['public/image.jpg'],
},
};
```
This will include the public/image.jpg file in the tracing process for the pages/index.js page.
Note that the key of outputFileTracingIncludes/outputFileTracingExcludes is a glob, so special characters need to be escaped.
Finally, if you're experiencing performance issues with tracing dependencies, you can try using turbotrace, a faster and smarter alternative to the JavaScript implementation.
To enable turbotrace, you can add the following configuration to your next.config.js:
```javascript
module.exports = {
// ...
outputFileTracingEngine: 'turbotrace',
};
```
This will use turbotrace to perform the tracing process, which can significantly improve performance.
For another approach, see: How to Use Reducer Api in Next Js 14
Production Checklist
Before you start deploying your product, make sure you have a solid production checklist in place. This will ensure a smooth transition from development to deployment.
Verify that all necessary dependencies are installed and up-to-date, as mentioned in the "Setting Up Your Environment" section.
Test your product on different browsers and devices to ensure compatibility, just like the "Cross-Browser Testing" example.
Run automated tests to catch any bugs or issues, as described in the "Automated Testing" section.
Create a backup of your production database to prevent data loss in case of an emergency, following the "Database Backup" best practices.
Use a continuous integration and deployment tool to automate the build, test, and deployment process, as outlined in the "CI/CD Pipeline" example.
Document your deployment process and make it easily accessible to the team, just like the "Deployment Documentation" section.
Related reading: Nextjs Hero Section Template
Frequently Asked Questions
Can you use Next.js without a server?
Yes, you can use Next.js without a server, but it's limited to serving static HTML/CSS/JS assets. Static export allows hosting on any web server that supports static assets, but some Next.js features require a server.
Does Next.js need a backend?
Next.js includes its own server, but you can still use an existing backend if needed. In most cases, Next.js can run independently without a custom backend setup.
Sources
- https://www.prisma.io/nextjs
- https://learn.microsoft.com/en-us/azure/static-web-apps/deploy-nextjs-hybrid
- https://nextjs.org/docs/pages/api-reference/next-config-js/output
- https://docs.sentry.io/platforms/javascript/guides/nextjs/manual-setup/
- https://nextjs.org/docs/pages/building-your-application/deploying/production-checklist
Featured Images: pexels.com