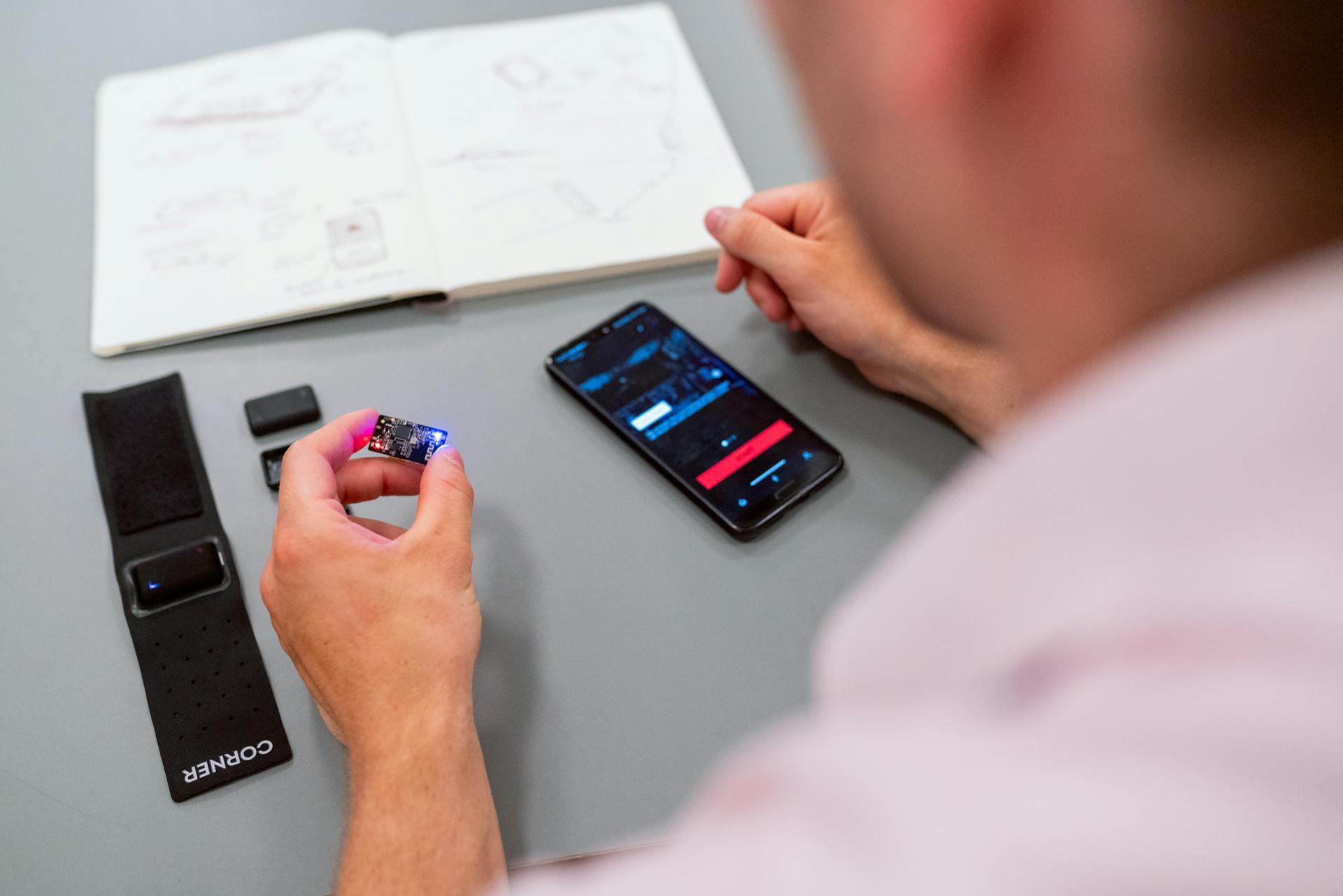
SSR in Next.js is a server-side rendering technique that allows you to render your React application on the server, so that the first HTML is sent to the client, improving SEO and user experience.
This technique is especially useful for complex applications that require a lot of data loading, as it allows you to pre-render the initial HTML, reducing the amount of data that needs to be transferred to the client.
By using SSR in Next.js, you can improve your application's performance and make it more scalable.
Next.js provides a built-in support for SSR, making it easy to implement and use.
What is SSR in Next.js
SSR in Next.js is a powerful feature that allows you to render your application on the server-side. This means that the server generates the HTML for each page, rather than the browser.
The power of Next.js lies in its Server Side Rendering (SSR) capabilities. This is what sets it apart from other frameworks. It improves SEO by having all the code of the application in one page, which is a big plus.
Here are the roles of Next.js that make SSR possible: Static generationServer Side Rendering (SSR)
SSR in Next.js also provides a fallback option, which is set to true by default. This means that when you navigate to a new page, you'll see a "Loading..." message before the page loads. You can test this by entering a new ID over 3 in the address bar of your browser, like http://localhost:3000/posts/4.
Nextjs Ssr
Nextjs SSR is a powerful feature that allows you to render pages on the server-side. This means that when a user requests a page, the server generates the HTML for that page instead of the client's web browser.
Nextjs SSR can be achieved using various methods, including the use of react-query in hooks for data management. For example, the refine framework uses react-query in its hooks to fetch data on the server-side.
You can use the getList method from your dataProvider to fetch users data and pass it through props as conventionally done in Next.js. Then, users data is available in the props of your /users page, and you can use it to load data on the server-side.
Nextjs SSR also has roles such as static generation and Server Side Rendering (SSR) itself. The power of Nextjs is SSR, which improves SEO by having all the code of the application in one page.
Here are the key benefits of Nextjs SSR:
- Improved SEO by having all the code in one page
- Reduced loading times and improved user experience
- Significant boost to search engine rankings
To take advantage of Nextjs SSR, you can use the "use client" directive to opt-out from Server Components, as refine and its dependencies are not yet compatible with Server Components. This is especially useful when working with the refine framework.
Nextjs SSR also allows you to write direct database queries without them being sent to browsers, making it a powerful tool for optimizing performance.
Development to Production
In Next.js, development and production environments have some key differences. For every change, you need to delete .next or and re-run the cmd displayed above to build again your application.
You have to do that in static generation case, not in ISR. When the application is built, we can read into the console page | size | first load js. It means that the routes have been built by generating static files into .next/server/pages/index.html
At first load, JS shares all the routes and heading into the browser client. Example of static generation includes _app.tsx => grap every page of the application => 0KB.users => users.tsx & _app.tsx the sum is equal to 64KB.Static Side Generation (SSG) + automatically genereted as static HTML + JSON
The size of the generated files is notable, with _app.tsx taking 0KB and users.tsx & _app.tsx summing up to 64KB. Static Side Generation (SSG) also automatically generates static HTML and JSON.
How SSR Works
SSR stands for Server Side Rendering, and it's a key feature of NextJS. NextJS uses SSR to improve SEO by rendering all the code of the application on one page.
The power of NextJS lies in its ability to render pages on the server, making it possible to test the application with a fallback setting enabled. If you enter a new id over 3 in the address bar of your browser (for example, http://localhost:3000/posts/4), a page will display "Loading..." before reaching the expected page.
Here are the roles of NextJS that make SSR possible:
- Static generation
- Server Side Rendering (SSR)
Server Side Rendering is what makes NextJS so powerful, and it's the core of its functionality. By using SSR, NextJS can render pages on the server, making it possible to test the application with a fallback setting enabled.
Next.js SSR Features
Next.js offers two main roles: static generation and Server Side Rendering (SSR). The power of Next.js lies in SSR, which improves SEO by having all the code of the application in one page.
Static generation and SSR are two different approaches to rendering pages in Next.js. Static generation is not related to the user session, so you can specify the nextServerContext parameter as null.
SSR is a powerful tool for improving performance and SEO, but it can become a bottleneck without proper optimization. To enhance SSR performance, you can use caching rendered pages, utilize Static Generation (SSG), or employ Incremental Static Regeneration (ISR).
Here are the key benefits of SSR in Next.js:
- Improves SEO by having all the code of the application in one page
- Allows for caching rendered pages
- Utilizes Static Generation (SSG)
- Employs Incremental Static Regeneration (ISR)
One of the benefits of SSR is that it allows for direct database queries without them being sent to browsers. This is because getStaticProps runs only on the server-side and is not included in the JS bundle for the browser.
Optimizing NextJS for Faster Performance
NextJS is well-known for its server-side rendering (SSR) capabilities, which can drastically improve the performance of web applications.
Optimizing NextJS not only enhances the user experience by reducing loading times but also significantly boosts search engine optimization (SEO).
Server-side rendering shifts much of the workload to the server, where the HTML is generated for each request, delivering fully-rendered pages to the client's browser.
Immediate page rendering makes content visible to users much faster since the browser receives already rendered pages.
SEO benefits are also a plus, as search engines can crawl and index fully rendered HTML more effectively than content rendered client-side.
Caching is a crucial strategy for enhancing the performance of server-rendered pages, and storing the output of rendered pages can dramatically reduce the time taken to serve a page to the user.
A caching layer like Redis can be used to implement a simple caching mechanism for NextJS applications.
Here are some key benefits of caching rendered pages:
You can test caching by setting the fallback to true and entering a new ID over 3 in the address bar of your browser, such as http://localhost:3000/posts/4.
Static Site Generation and SSR
Static Site Generation (SSG) can yield significant performance improvements by pre-rendering pages at build time. This allows static pages to be served instantly at request, which is much faster than Server Side Rendering (SSR).
SSR is a powerful feature in Next.js that improves SEO by having all the code of an application in one page. It's achieved through the use of getServerSideProps, which runs only on the server side and generates HTML and JSON files that can be cached by a CDN.
To convert an SSR page to SSG, you would change your page's data-fetching method from getServerSideProps to getStaticProps. This function generates HTML and JSON files at build time, making the page pre-rendered and indexed for SEO.
Here are some key differences between SSG and SSR:
Using Static Generation
Static Generation (SSG) is a powerful technique that can significantly improve performance. Converting SSR pages to SSG can yield significant performance improvements.
SSG pre-renders pages at build time, allowing these static pages to be served instantly at request, which is much faster than SSR. This is ideal for pages with content that does not change frequently or pages where real-time data is not crucial.
To convert an SSR page to SSG in NextJS, you would change your page’s data-fetching method from getServerSideProps to getStaticProps.
Static rendering in NextJS does not require a user session, so you can specify the nextServerContext parameter as null. This is useful for some use cases, such as when you are using the Storage API with guest access.
Here are some key points to keep in mind when using getStaticProps:
- Must be in the pages folder and not in the components folder.
- Run only on server side (code server side).
- The code inside this function will be never includes into the bundle JS or TSX which is sended to the browser.
- APIkey is not required.
- particularity: have an object which one content another object.
getStaticProps generates HTML and JSON files, both of which can be cached by a CDN for performances. The pre-rendered is done at build time.
Incremental Static Regeneration
Incremental Static Regeneration (ISR) is a feature in NextJS that allows you to update static content after building your site.
By setting a revalidate property in getStaticProps, you can determine how often the page should be regenerated, giving you control over when your static content is updated.
ISR works by updating static content incrementally at runtime instead of at build time, which means you can enjoy the benefits of static generation while keeping your pages up-to-date.
This approach can be especially useful for handling more requests efficiently and improving the user experience, making it a valuable addition to your static site generation and server-side rendering strategies.
GetStaticProps
GetStaticProps is a crucial function in Next.js that allows you to pre-render pages at build time. It runs only on the server-side, so you can write direct database queries without them being sent to browsers.
GetStaticProps generates HTML and JSON files, both of which can be cached by a CDN for performance. The pre-rendered HTML is done at build time, and Next.js generates a JSON file holding the result of running getStaticProps(). This JSON file will be used in client-side routing through next/link or next/router.
To use getStaticProps, your page must be in the pages folder and not in the components folder. The function must be run only on the server-side, and the code inside it will never be included in the bundle JS or TSX sent to the browser.
Here are some key facts about getStaticProps:
- Must be in the pages folder and not in the components folder.
- Run only on server side (code server side).
- The code inside this function will be never includes into the bundle JS or TSX which is sended to the browser.
- APIkey is not required.
- particularity: have an object which one content another object.
GetStaticProps generates a JSON file holding the result of running getStaticProps(), which will be used in client-side routing through next/link or next/router. This allows for fast page loading and improved SEO.
Frequently Asked Questions
What is the difference between CSR and SSR in Nextjs?
In Next.js, CSR (Client-Side Rendering) sends a minimal HTML document with JavaScript to the client, which generates HTML dynamically, whereas SSR (Server-Side Rendering) generates the full page's HTML on the server for each request
What does SSR mean in react JS?
Server Side Rendering (SSR) in React JS refers to rendering web pages on the server before sending them to the client, improving page loads and SEO. This technique enables faster and more efficient rendering of React applications.
Is Next.js a server-side language?
No, Next.js is not a server-side language, but rather a framework that utilizes server-side rendering to enhance performance and security. This innovative approach allows for dynamic content rendering on the server, reducing browser load and improving overall user experience.
Sources
- https://connectrpc.com/docs/web/ssr/
- https://refine.dev/docs/3.xx.xx/advanced-tutorials/ssr/nextjs/
- https://github.com/TLRKiliann/nextjs-ssr
- https://loadforge.com/guides/optimizing-server-side-rendering-in-nextjs-for-faster-performance
- https://docs.amplify.aws/react/build-a-backend/server-side-rendering/
Featured Images: pexels.com