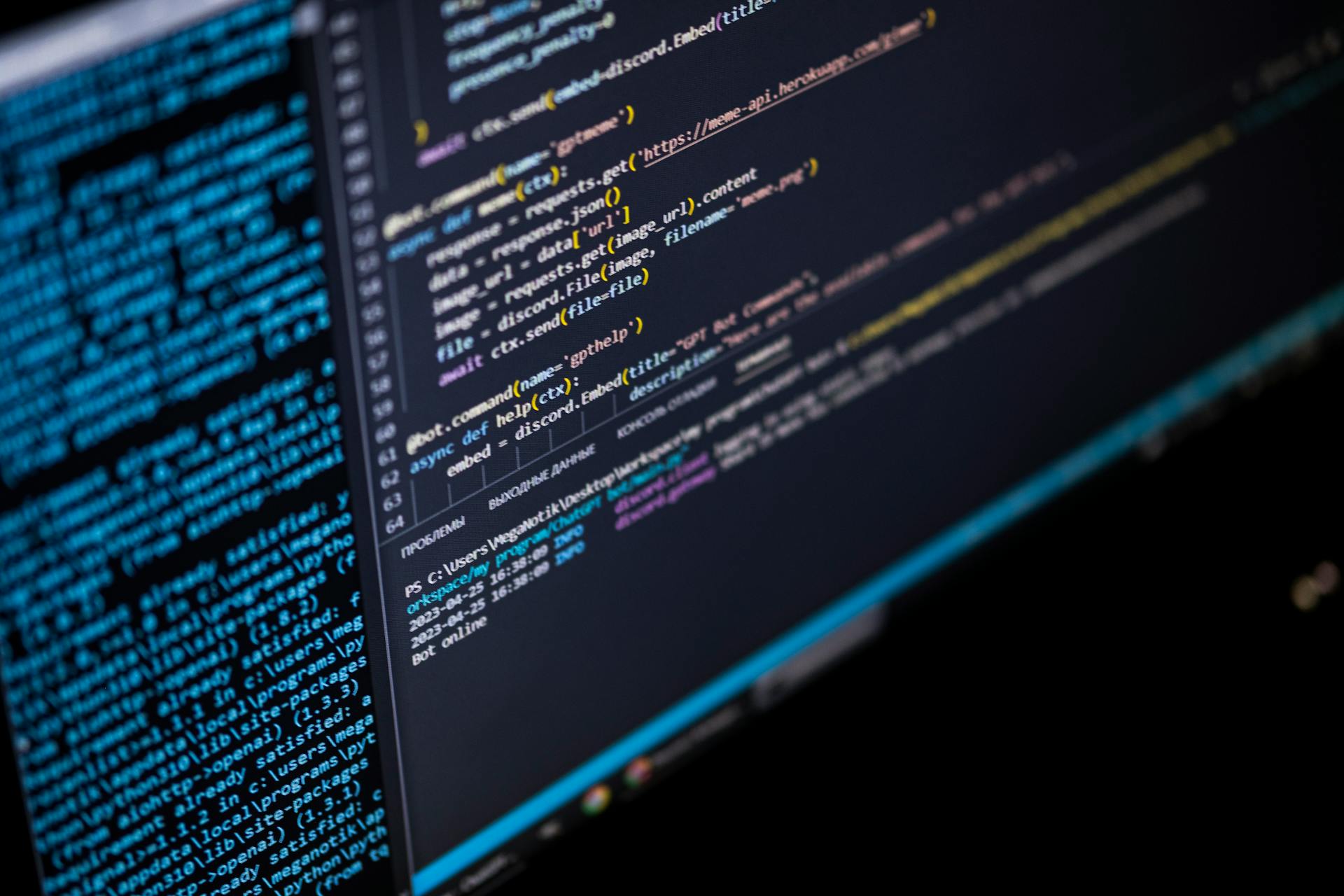
Next.js is a popular framework for building server-rendered, statically generated, and performance-optimized web applications. It's particularly well-suited for Progressive Web Apps (PWAs), which provide a seamless user experience across devices.
Next.js allows for serverless deployment, which means you can host your app without worrying about server management or scaling. This approach also reduces costs and increases reliability.
With Next.js, you can leverage serverless functions to handle tasks such as authentication, data storage, and API integrations. This enables you to build scalable and maintainable applications with minimal overhead.
Serverless deployment also allows for faster deployment and testing, which is crucial for PWAs that require frequent updates to stay relevant.
Getting Started
To begin with Next.js, create a new project by clicking on MyWeb in the sidebar and opening your Next.js app in your browser.
You can use any stage name here, but it's good to create a new stage for production.
Once your project is set up, you can start building your app using Next.js.
Here's an interesting read: Next Js Cookies
Create a Project
To create a project, you can start by clicking on MyWeb in the sidebar and opening your Next.js app in your browser. This will allow you to access your project and begin working on it.
You can use any stage name you like, but it's a good idea to create a new stage for production to keep your projects organized.
See what others are reading: Next Js Create App
Setting
As you dive into the world of containers, setting up your environment is crucial for a smooth experience.
To access your app, you'll need to map the host port to the container port, which is done using the ports option. This means you'll access your app via localhost:3000.
You'll want to set up your ports carefully to ensure everything runs smoothly. The ports option maps the host port to the container port, forwarding requests made to the container at port 3000.
Remember, the ports option is essential for accessing your app from the host machine.
Serverless and Performance
Serverless development is a great way to build Next.js apps, and it's easy to get started. To begin, you'll need to configure your AWS credentials.
To create a serverless Next.js app, you can use OpenNext and the Nextjs component. This involves initializing SST in your app and selecting the defaults, which will create a sst.config.ts file in your project root.
For production, it's essential to monitor your app's state and performance in real-time. Sentry can help you identify issues by tracking your Next.js application's state and performance in real-time.
Check this out: Nextjs Performance
Initializing with Bun
Initializing with Bun is a straightforward process. You can start by checking out the docs and installing Bun.
To generate a working Next.js base app, use the command mentioned in the example. This will present you with a series of configuration options, but for simplicity, you can stick with the defaults.
After running the command, you can access your app's dev server by visiting localhost:1313.
Readers also liked: Bun vs Next Js
Serverless
To get started, you'll need to configure your AWS credentials. This will allow you to access AWS services from your local machine.
Next, we'll initialize SST in our app by selecting the defaults and picking AWS. This will create a sst.config.ts file in your project root.
To deploy our app using OpenNext and the Nextjs component, we'll add the necessary code above the Nextjs component.
Intriguing read: Next Js Software Staging Environment Aws Amplify Gen 2
Performance Monitoring
Performance Monitoring is essential for serverless applications, and Next.js is no exception. You can use tools like Sentry to track your application's state and performance in real-time.
Sentry can alert your development team when errors occur, allowing them to quickly address the issue and prevent it from impacting the user experience. This is crucial for ensuring the quality and functionality of your application.
Monitoring your application's performance also provides valuable insights, such as response times and resource usage. This information can help you optimize your application and improve its overall reliability.
Identifying performance bottlenecks and errors is a crucial step in ensuring the quality and functionality of your application. With Sentry, you can get actionable insights to resolve these issues and improve your application's performance.
See what others are reading: Next Js Sentry
Defining the Service
Defining the Service is an essential step in setting up a Next.js app with Docker. Create a file called compose.yml in your project's root directory, just like we did earlier.
The service name is crucial, and it's best practice to choose a name relevant to the project. Its name doesn't exactly matter, but a name like "nextjs-app" would be a good choice.
The Docker Compose file will contain a single service, which is defined by a specific configuration. This configuration includes the image name, which is typically the same as the Dockerfile name.
You might enjoy: File Upload Next Js Supabase
API and Routing
Next.js allows you to create REST API endpoints by placing files with desired endpoint names inside the /pages/api directory.
To fix CORS errors that occur when accessing these endpoints from a different origin, you can leverage the cors package to enable cross-origin sharing. This can be done by installing the package, importing it, and running custom middleware before the API route sends its response.
The cors package allows you to enable specific methods, such as POST, GET, and HEAD, for each endpoint. Once set up correctly, you should stop experiencing CORS errors.
API Routes
API Routes are a crucial part of any application, allowing you to create REST API endpoints by placing files with the desired endpoint names inside the /pages/api directory.
These endpoints are then mapped to the /api/* URL and can be accessed from within the application by making asynchronous requests. Next.js allows users to create these endpoints by placing files with the desired endpoint names inside the /pages/api directory.
However, once your application has been deployed and you try to access the API endpoint from a different origin, you get the cors error. This is because Next.js doesn't enable cross-origin sharing by default.
To fix this error, you can leverage the popular cors package to enable cross-origin sharing before your API route sends its response. You can install the cors package and then import it to run custom middleware before the API route sends its response.
A fresh viewpoint: Nextjs Error Page
Api/Slug
API/Slug errors occur when a dynamic page is being rendered on the server-side using the Static Site Generation (SSG) feature in Next.js, but the getStaticPaths() function is not defined in the page's component.
The getStaticPaths function is a required part of the Next.js API for building server-side rendered (SSR) or statically generated (SSG) pages that use dynamic routes.
This error happens specifically with the getStaticProps API.
To fix this error, you need to add a getStaticPaths() function to the page component for /pageName/[slug].
The getStaticPaths function could fetch a list of all the available blog post slugs from a database and return them as the possible values for the [slug] parameter.
For more insights, see: Nextjs Error Boundary
Middleware and Debugging
Middleware enables the execution of code prior to the completion of a request, and based on the incoming request, it modifies the response sent to the user by rewriting, redirecting, or even adding headers.
This error usually happens when you're using Next.js with a custom server, and you did not explicitly specify the server host URL in your configuration.
You can quickly fix this error by specifying the hostname in your server configuration file. If you are using nx, you can do this by modifying the serve options in your project configuration file (project.js).
Recommended read: Using State in Next Js
Storage and Containers
To deploy a Next.js app in a container, we'll use AWS Fargate with Amazon ECS, replacing the run function in our sst.config.ts file.
This creates a VPC with a bastion host, an ECS Cluster, and adds a Fargate service to it. It's a solid foundation for containerized deployment.
To build our Docker image and deploy, we run a command that builds the image and deploys it to the Fargate service.
Recommended read: Nextjs Image Component
Add Redis
Adding Redis to your storage and container setup can be a game-changer for applications that require high-performance data retrieval.
We need Redis because it allows us to implement a force-dynamic approach, which prevents Next.js from caching the counter.
This is especially important in applications where data needs to be updated frequently, such as a live counter.
By using Redis, you can ensure that your application always has access to the latest data, without relying on caching.
In some cases, this can make a huge difference in the user experience, especially if your application is handling a high volume of requests.
For example, in a Next.js application, adding Redis can help prevent caching issues that might arise from using a counter.
See what others are reading: Next Js Single Page Application
Containers
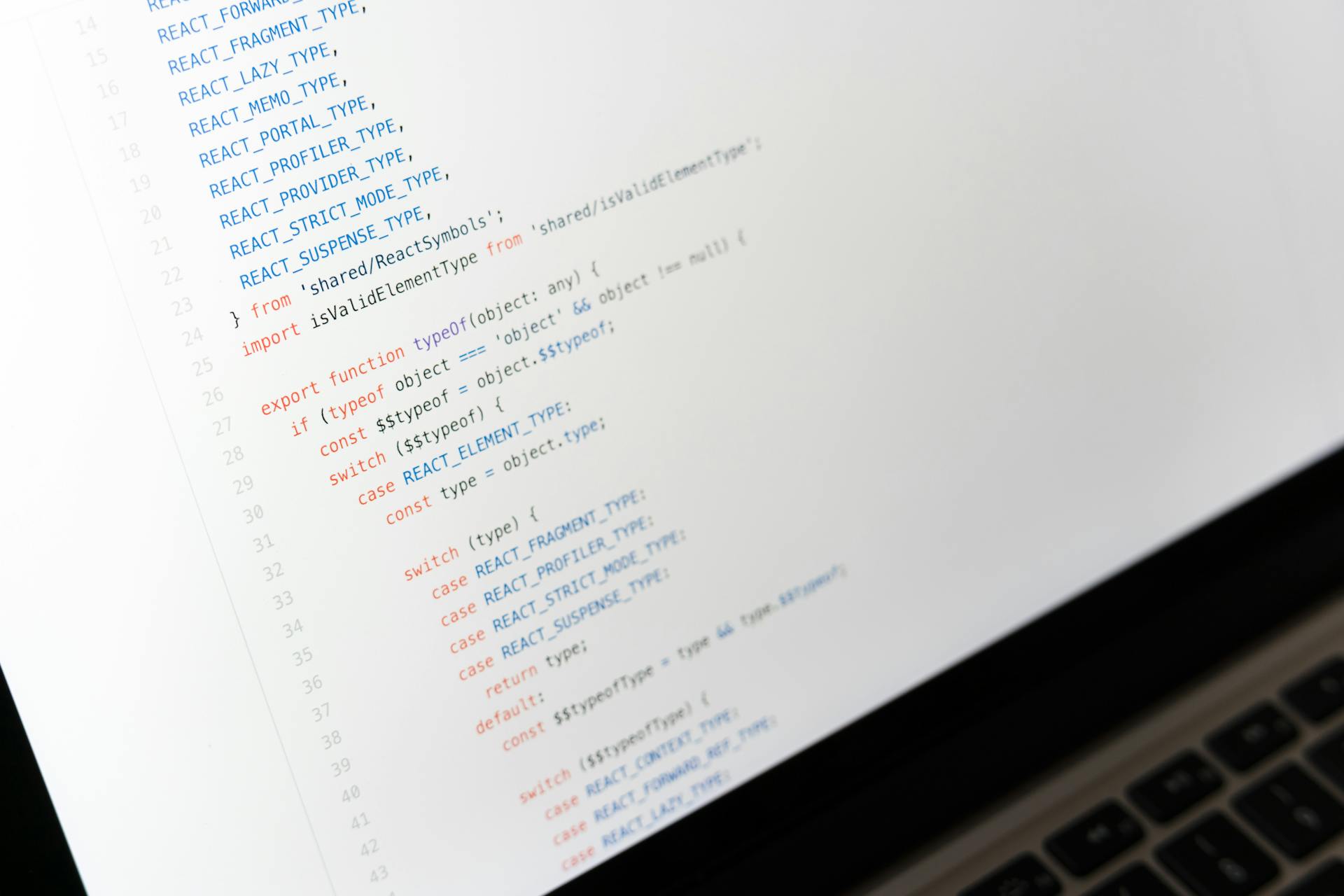
To deploy our Next.js app in a container, we'll use AWS Fargate with Amazon ECS. This involves replacing the run function in your sst.config.ts.
AWS Fargate creates a VPC with a bastion host, an ECS Cluster, and adds a Fargate service to it. This setup is crucial for a smooth container deployment.
We'll add a Dockerfile to create our Docker image. This file is essential for building our container image.
To build our Docker image and deploy, we run a specific command. This command is necessary to get our app up and running in a container.
Curious to learn more? Check out: How to Run Nextjs to Build
Sources
- https://sst.dev/docs/start/aws/nextjs/
- https://nextjs.org/docs/app/building-your-application/configuring/debugging
- https://javascript.plainenglish.io/integrating-rust-into-next-js-how-to-developer-guide-10e533470d71
- https://blog.sentry.io/common-errors-in-next-js-and-how-to-resolve-them/
- https://shipyard.build/blog/nextjs-with-docker/
Featured Images: pexels.com