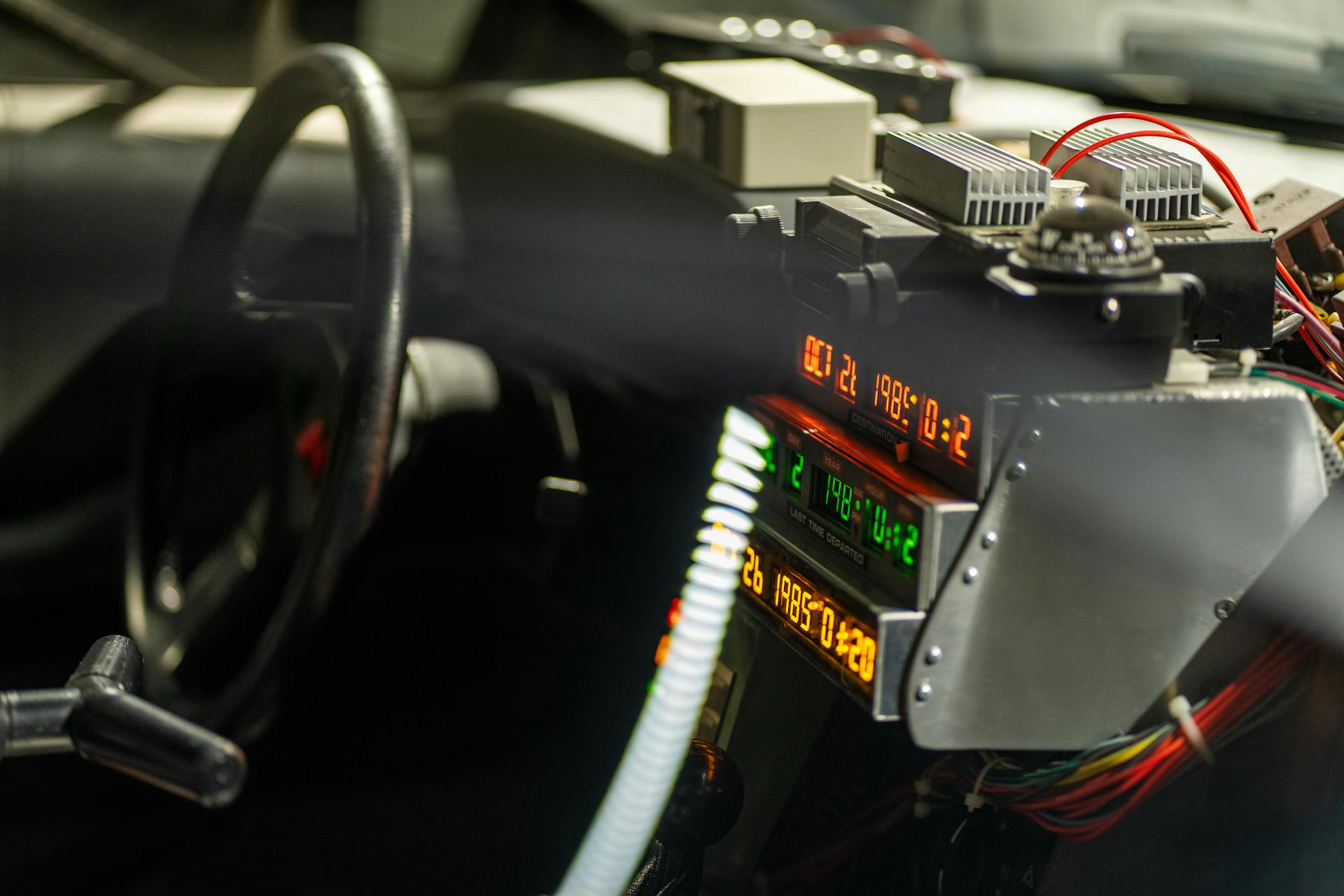
Setting up a Next.js App Router with tRPC can be a bit overwhelming, but don't worry, we'll break it down step by step.
First, you'll need to create a new Next.js project using the `npx create-next-app` command. This will set up a basic Next.js project with the App Router enabled.
Next, you'll need to install tRPC using npm or yarn. This will add the necessary dependencies to your project, including the `@trpc/client` and `@trpc/server` packages.
With tRPC installed, you can create a new `trpc` instance in your project by running the `npx trpc init` command. This will generate a new `trpc` configuration file in your project.
Explore further: New Nextjs Project Typescript
Setup
To set up a NextJS app router with tRPC, you'll need to create a monorepo using PNPM, a fast and efficient package manager. This will allow you to have a type-safe frontend and backend, reusing code and interfaces between the two.
Create a directory for your project and initialize an PNPM project using `pnpm init`. Then, create a `pnpm-workspace.yaml` file to define the packages in your project, including the frontend and backend.
If this caught your attention, see: Cookies Nextjs
To set up the backend project first, install the necessary packages and configure the tRPC router. For tRPC v11, you'll need to explicitly set the "version" option to "v11" and add options to let ZenStack know where to import the tRPC factories.
Here are the packages you'll need to install:
- PNPM
- tRPC package
- @zenstackhq/trpc plugin
Once you have your backend project set up, you can move on to setting up the NextJS frontend with the app router.
Monorepo Setup
To set up a monorepo, we need to define the tRPC router from the backend and export the app router type, so that the frontend project can import that type and re-use it in the frontend.
Using a monorepo will help us have a type-safe NextJS frontend, completely eliminating a class of errors and providing autocomplete features that make front-end development a breeze.
We'll use PNPM to set up the workspace, a fast and efficient package manager that I love for its speed and efficiency.
You might enjoy: Nextjs Monorepo
We'll create a directory for the project, including the backend and frontend code, and then initialize a PNPM project to create a package.json in the root directory.
A pnpm-workspace.yaml file is also necessary to define the packages we'll have in the project, which in this case will be the front-end and back-end projects.
This setup will help us reduce code duplication and make maintenance easier, as we'll be able to reuse code and types across both the frontend and backend.
Additional reading: How to Add Backend to Nextjs
Setting Up
Setting up a tRPC server in NodeJS involves several key components. The appRouter is the main router of the tRPC server, responsible for handling all routings for it. Context is also crucial, as the createContext function runs every time a request is sent to the tRPC server, allowing you to run code before handling a route, like handling authentication and protecting routes.
To integrate the tRPC server with an Express server, you can use the createExpressMiddleware function exposed by the @trpc/server library. This middleware is built to handle internal routing for the tRPC server.
For more insights, see: Trpc Nextjs App Directory
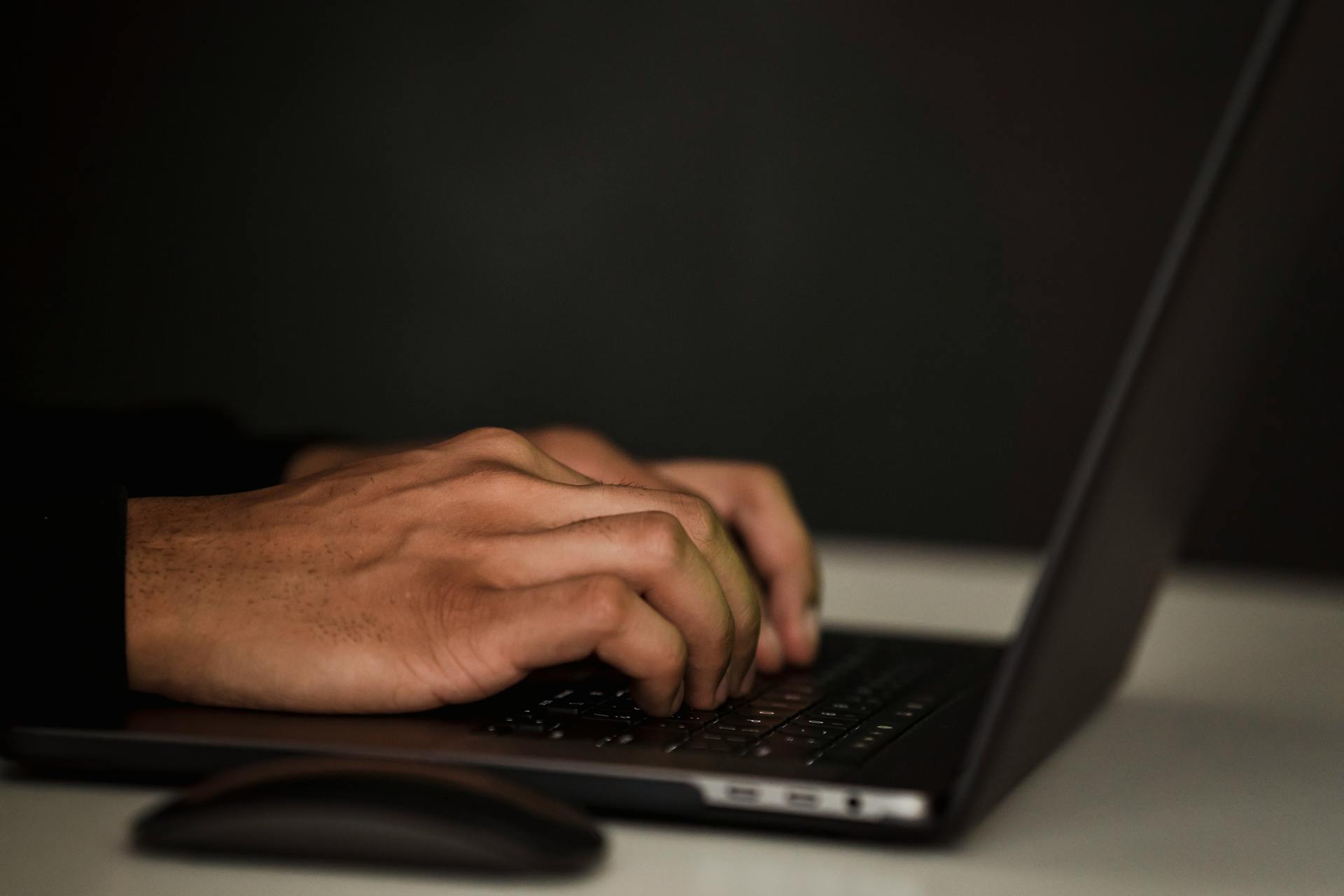
To send requests from the NextJS client to the tRPC server, you need to set up the tRPC client. This involves initializing the tRPC client, creating a file for the tRPC client, and exporting the AppRouter type from the backend.
The tRPC client requires a type-safe interface, which is provided by the AppRouter type. This interface is exported from the backend and gives the tRPC client a robust and reliable way to communicate with the server.
Here are the steps to set up the tRPC client:
- Install the tRPC client dependencies for NextJS
- Install the backend as a package so that you can use the tRPC router interface in the frontend too
- Initialize the tRPC client
- Create a file for the tRPC client and export the AppRouter type from the backend
By following these steps, you can set up a tRPC server and client in your NextJS project, enabling you to send requests from the client to the server and receive robust and reliable responses.
On a similar theme: Next Js Debug
Nextjs Configuration
To set up NextJS 14 with App Router, start by naming your project, in this case, "frontend", and selecting the default options. Make sure to update the project name in the pnpm-lock.yaml file if you choose a different name.
In the app directory, open the layout.tsx file and wrap your application with the providers you created earlier. This will integrate your tRPC client with React query for caching and other features.
Next, create a tRPC client for your NextJS frontend, which will allow it to send requests to your tRPC server.
Related reading: Next Js Client Side Rendering
TRPC Integration
To get started with TRPC integration in Next.js App Router, you'll need to create a tRPC helper that can be used in your client code. This helper is essential for making API calls.
You can use the fetchRequestHandler from tRPC to handle requests and responses in the App Router, unlike in the Pages Router where you would use the Next helper. This is because the App Router uses standard Request and Response objects.
To use tRPC with NestJS and NextJS, you'll need to add the tRPC server in the NestJS app and the tRPC client in the NextJS app. Make sure to import the module into the main AppModule.
For your interest: Use Client Nextjs
In a client component, you can use the trpc helper from the utils/trpc.ts file to call procedures. This is similar to how tRPC is used in the Pages Router. The helper is located in the utils/trpc.ts file.
In a server component, you can use the createAsyncCaller helper from the trpc/router/_app.ts file to call procedures. This helper is specifically designed for server components.
Related reading: Next Js App Folder
Router and Middleware
To set up a router in our Next.js App Router with tRPC, we create a single file called _app.ts to define all our endpoints. This keeps things simple, but you can break it up into multiple files if you prefer.
The router is created by initializing our tRPC context and wrapping our root layout with the TrpcProvider. This is a crucial step to get our tRPC server up and running.
We'll also want to create a tRPC helper that we can use in our client code, which involves creating an API endpoint using the fetchRequestHandler from tRPC. This is because the App Router uses the standard Request and Response objects, unlike the Next.js pages router.
You might like: Next Js App
Generated Routers
Generated routers are a crucial part of the tRPC setup, and you can find them in the output folder, one per each data model.
You'll also find a createRouter helper function generated, which returns a router instance for all models. This can be used as your top-level tRPC router or merged with other routers to form a more complex setup.
The createRouter helper function makes it easy to manage multiple routers and their respective data models.
By using the generated routers, you can create a robust and scalable tRPC setup that meets the needs of your application.
Explore further: Nextjs App Route Get Ssr Data
Creating a Router
You should find a bunch of tRPC routers generated in the output folder, one per each data model. A createRouter helper function is also generated, which returns a router instance for all models.
To keep it simple, we’ll define all our endpoints in a single file called _app.ts, but you can (and probably should) break it up into multiple files if you want. This is because we need to initialize our tRPC context and wrap our root layout with the TrpcProvider.
For our last bit of setup, we need to initialize our tRPC context by calling the createContext function. This function runs every time a request is sent to the tRPC server, making it a great place to handle authentication and protect routes.
Here's a brief overview of the key components involved in creating a router:
Now that we have our router set up, we can start sending requests from the NextJS client to the tRPC server.
Authentication and Testing
To test our authentication, start up your local dev server using npm run dev and head over to http://localhost:3000.
You should be automatically redirected to our custom sign-in page at http://localhost:3000/sign-in if you're not already authenticated.
The custom sign-up page is accessible at http://localhost:3000/sign-up using the link we added.
Once authenticated, you can access the blog home page.
To sign out of the application, use the UserButton component we added, which will redirect you back to the sign-in page.
You might enjoy: Using State in Next Js
Client and API
To make a client-side call using the tRPC client, you'll need to update the url property in the trpc.ts file to point to the respective NestJS deployed server, as this won't work when deployed.
You'll want to update the url property to use environment variables if you're deploying your application.
In the NextJS /app directory, you'll see a file called page.tsx, where you can use the trpc client to call the hello procedure you defined in the tRPC server.
The hello procedure is a great place to start, as it's a simple example of making a server-side call using the tRPC client.
For your interest: Nextjs Server Actions File Upload
Frequently Asked Questions
Is tRPC worth learning?
Yes, tRPC is worth learning for developers who prioritize simplicity and type safety in their projects, especially those with a straightforward API structure. It can provide a seamless development experience and is an excellent choice for Next.js projects that heavily rely on TypeScript.
Featured Images: pexels.com