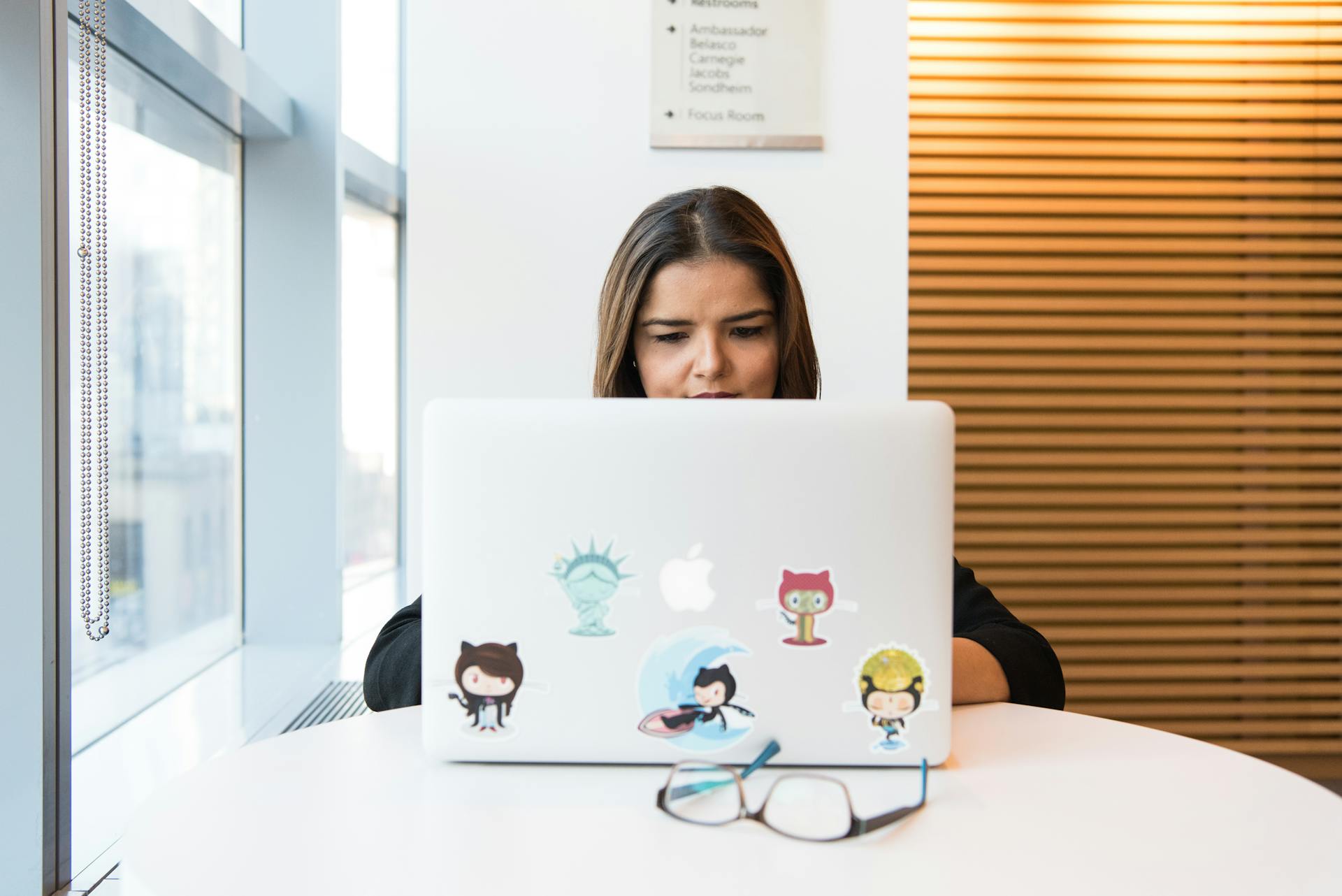
React-infinite-scroll-component is a popular library for implementing infinite scrolling in Next.js applications. It allows users to load more content as they scroll down the page, creating a seamless experience.
This approach is particularly useful for large datasets or content-heavy applications, where loading all data at once can be slow and inefficient. By using React-infinite-scroll-component, you can improve user engagement and reduce the load on your server.
One of the key benefits of React-infinite-scroll-component is its ease of use. It provides a simple and intuitive API that makes it easy to integrate into your Next.js application. With just a few lines of code, you can get started with infinite scrolling.
The library also supports pagination, which is essential for handling large datasets. By dividing the data into manageable chunks, you can ensure that your application remains responsive and efficient.
A different take: Next Js Build Collecting Page Data
Implementing Infinite Scroll
Implementing infinite scroll involves replacing a button with a scroll-trigger element, such as a spinner or text indicating the loading, which triggers the loading of the next batch of data when it comes into view within the viewport.
Discover more: Next Js App Router Tailwind Spinner Loading Page
This is basically how the infinite scroll feature works, and we can detect the intersection of an element using the JavaScript Intersection Observer API. We'll cover two methods for implementing infinite scroll, one involving a small dependency that simplifies using the Intersection Observer API in React, and the other directly using the Intersection Observer API, which is slightly more complex to implement in React.
We need to utilize the useEffect Hook because some async tasks will be involved to accomplish the infinite scroll feature, and the useEffect Hook we used here depends only on the hasMoreData state for now.
Suggestion: Next Js Cache
Implementing Infinite
Implementing infinite scroll involves replacing the button in the PostList component with a scroll-trigger element, such as a spinner or text indicating the loading.
We can detect the intersection of an element using the JavaScript Intersection Observer API, which is covered in two methods: using a small dependency that simplifies using the Intersection Observer API in React, or directly using the Intersection Observer API, which is slightly more complex to implement in React.
For more insights, see: Next Js App Router Api
We need to utilize the useEffect Hook because some async tasks will be involved to accomplish the infinite scroll feature.
The useEffect Hook we used here depends only on the hasMoreData state for now, because we don’t have anything like the isInView boolean available, as we did in the previous component.
Every Next.js developer might be interested in providing overriders or delegating the rendering of global loading, error, empty, and hasMore states to another React component.
We can implement infinite scroll by creating a copy of the PostList component and naming it PostListInfiniteRIO or any other suitable name, and then easily implement this new component in the page.tsx file, replacing the PostList component.
To implement infinite scroll, we can use the React.lazy function from React, which takes a function that calls a dynamic import(). This function must return a Promise which resolves to a module with a default export containing a React component.
We can use the useState Hook to manage existing posts and page numbers received from the server, and establish the necessary logic to load the next cargo of posts.
Suggestion: Nextjs Loading Page Ssr
The loadMorePosts function has three state variables working together to deliver different responsibilities: offset, posts, and hasMorePosts.
The posts variable holds the initial posts we expect to receive from the server in the PostList component whenever the page loads, and we append new data to this array based on the hasMoreData boolean.
We can use the getPosts action and receive an empty response, in which case we set the hasMorePosts boolean to false, which will stop making requests to load more content.
The visibility of the trigger button is controlled by the hasMoreData boolean, and we can integrate the PostList component into the page.tsx file at the app directory’s root.
We can fetch the initial data using the getPosts Server Action and load the posts from 0 up to the value we specified for our POST_PER_PAGE constant.
The final step is to extend our current implementation to include infinite scrolling for loading data instead of the load more button.
Broaden your view: Next Js Loading Initial Props
Horizontal vs Vertical
Implementing Infinite Scroll requires careful consideration of scroll direction. It's essential to match the scroll direction of your renderer with the InfiniteScroll component.
To ensure a seamless scroll experience, make sure your renderer and InfiniteScroll are both either horizontal or vertical. This means setting the scrollDirection property in the InfiniteScroll component to either "horizontal" or "vertical".
When setting up your layout, wrap the Renderer and the sentinel in a flex container. This will allow you to easily adjust the scroll direction by conditionally setting the flex-direction property to column or row.
When the scrollDirection is "horizontal", don't forget to set the overflow-x property to true. This will prevent the content from being cut off and ensure a smooth scrolling experience.
Recommended read: Next Js Component Rendering Analyzer
Preparing Data and State
The data you're rendering with InfiniteScroll is an array of type T, which is passed to the props.Renderer as a React.ReactNode. This is where the rendering of your data begins.
Here's an interesting read: Nextjs Rendering
You have the option to provide overriders or delegate the rendering of global states like loading, error, empty, and hasMore to another React component. This is recommended because InfiniteScroll takes care of rendering these states by design.
Every Next.js developer might want to consider this approach to keep their code organized and maintainable.
Create Custom Hook
Creating a custom hook is a great way to manage complex logic in your application. A custom hook is a reusable function that uses the React Hook API to provide stateful behavior to function components.
The vast majority of the logic governing infinite scroll behavior can be found within a custom hook called useInfiniteScroll. This hook is what we'll use to determine if a user has intersected a given element.
The loadMoreCallback method is what we'll use to determine if a user has intersected a given element. This method is what makes an API call to retrieve more data when the user is intersecting our target element.
Expand your knowledge: Nextjs React Query
We pass a handler method handleObserver and initialization options to IntersectionObserver, which will handle emitted events. If the user is intersecting our target element, then target.isIntersecting is true and an API call is made to retrieve more data.
Intersection Observer may fire multiple intersecting events when the user reaches the target, so we want to debounce those events to avoid making unnecessary calls to our API. We do that by only executing the API call if no other intersecting events have occurred within the last 500 milliseconds.
The magic browser API helping us do that is called “Intersection Observer”. We pass a handler method handleObserver and initialization options to IntersectionObserver, which will handle emitted events.
Direction and Infinity
Infinite scroll can be implemented in two ways, using a small dependency that simplifies the Intersection Observer API in React or directly using the Intersection Observer API.
The Intersection Observer API is useful for detecting when an element comes into view within the viewport, which is how infinite scroll works.
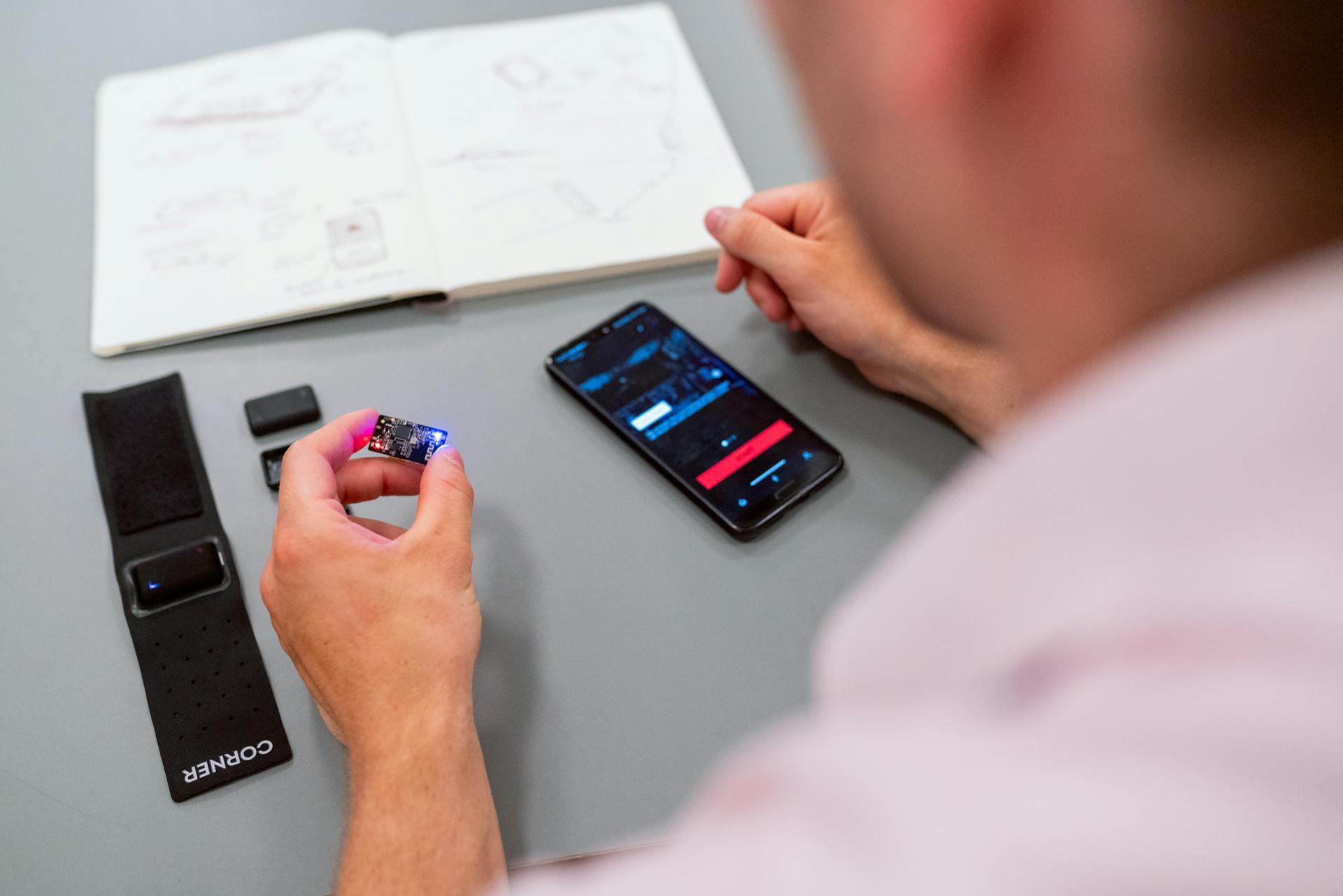
To implement infinite scroll, you need to create a copy of the PostList component and name it PostListInfiniteRIO, and use the useEffect Hook because some async tasks will be involved.
The useEffect Hook depends only on the hasMoreData state for now, as you don't have anything like the isInView boolean available.
You can also use lazy loading with infinite scroll to efficiently manage large sets of data, which is better than using conventional custom loading with hooks.
Lazy loading is implemented by using the React.lazy function from React, which takes a function that calls a dynamic import.
Infinite scroll can be implemented by creating mock data for your cards and using the async loading function to push more values to the dataset.
The async loading function is used to make an API call or add datasets without the need for pagination buttons.
You can also implement scroll direction and two-way infinite scroll, but it's not necessary for some use cases, and the implementation is not covered in this guide.
A unique perspective: Next Js 14 Layout Async
Intersection and Fetching
To make infinite scrolling work, we need to detect when the user has reached the bottom of the page. This is where Intersection Observer comes in, allowing us to observe the visibility of an element on the page.
We define the intersection target by adding a loadMoreCallback method as a ref to our desired component. This method is then re-rendered after new data is loaded, ensuring the intersection target moves along with the bottom of the page.
The Intersection Observer will fire an intersection event at the bottom of the page, which is convenient but can lead to some surprising problems.
To detect when a getter should be called to get the next page, we use a sentinel – an empty div that we can detect its on-screen visibility. We will use react-intersection-observer to detect whether our sentinel is visible or not.
After a successful request, there are only three possibilities: we ran out of pages, the sentinel is no longer in view, or we did not run out of pages and the sentinel is still in view.
A different take: Use Theme in Server Components Nextjs
Lazy Loading and Layout
Lazy loading is a game-changer for frontend developers, especially when dealing with large sets of data. It's better and more friendly than using conventional custom loading with hooks.
To implement lazy loading, you can use the React.lazy function, which takes a function that calls a dynamic import(). This function must return a Promise that resolves to a module with a default export containing a React component.
The lazy component will then be rendered inside the Suspense Component, which allows you to have a fallback function that will be used to render a loader before the lazy components finish loading.
You can start by importing bootstrap CSS so it can be accessed globally in the project. This is essential for a smooth user experience.
The React.lazy function returns a Promise that resolves to a module with a default export containing a React component, which is then rendered inside the Suspense Component. This is how you can implement lazy loading in your React application.
For more insights, see: Nextjs Suspense
To handle lazy loading, you should see a code snippet that looks something like this, where the CardComponent is rendered inside the Suspense Component.
Lazy loading is a great way to improve the user experience, especially when dealing with large datasets. It's more efficient and friendly than conventional custom loading with hooks.
Sources
- https://www.thegnar.com/blog/infinite-scroll-react-example-with-typescript-and-nextjs
- https://blog.logrocket.com/implementing-infinite-scroll-next-js-server-actions/
- https://www.omar-ibrahim.com/blog/articles/5/How-to-implement-infinite-scroll-using-Next.js-Server-Actions
- https://cloudinary.com/blog/lazy-loading-with-infinite-scroll
- https://nextui.org/docs/components/table
Featured Images: pexels.com