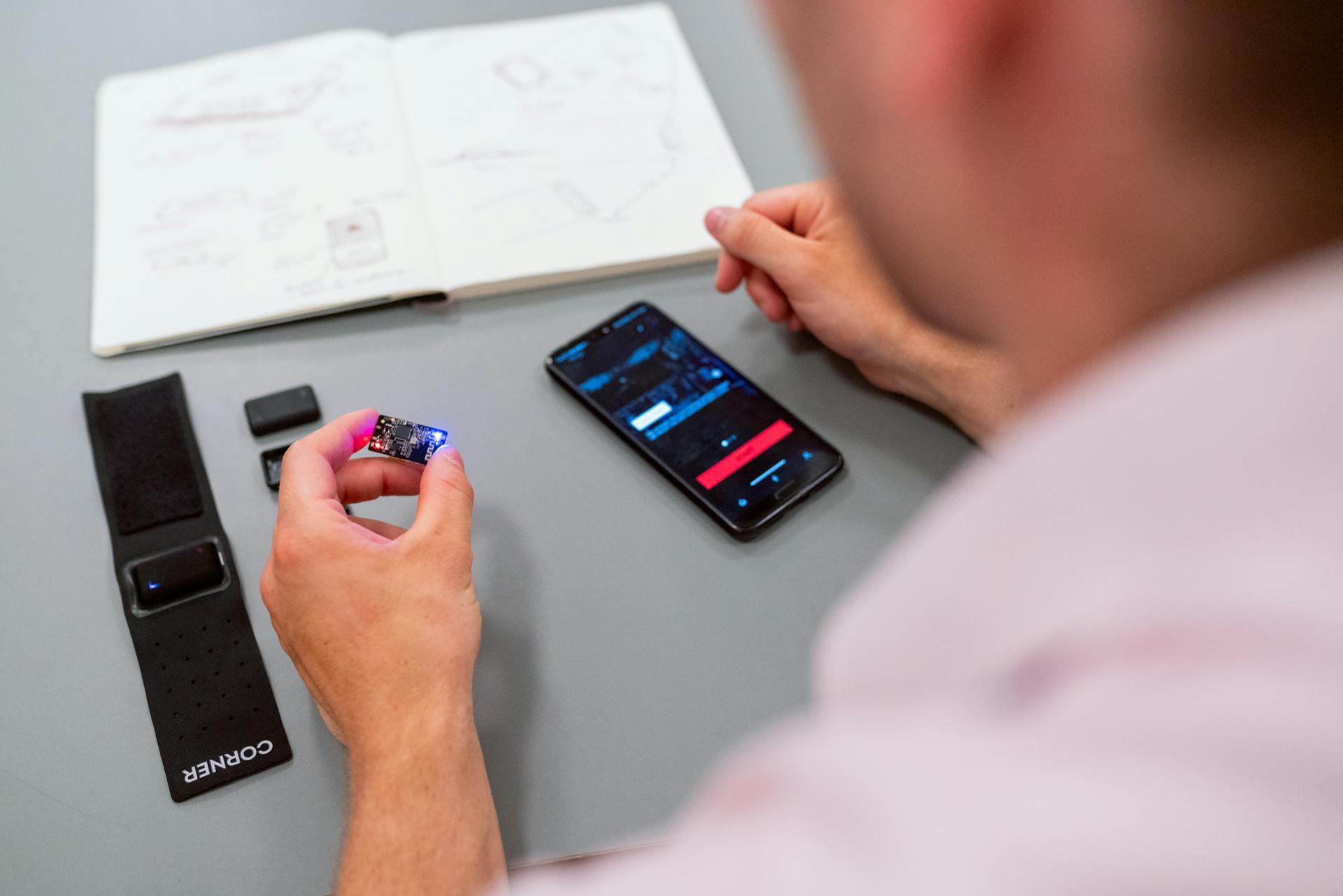
Zustand is a popular state management library for Next.js applications, designed to simplify the process of managing global state.
It's a lightweight library that doesn't require any boilerplate code, making it easy to integrate into your Next.js project.
By using Zustand, you can manage global state in a predictable and easy-to-debug way, reducing the complexity of your application.
This library is particularly useful for managing application-wide state, such as theme settings or authentication data.
Additional reading: Next Js Using State Context
What Is Zustand?
Zustand is a state management library for React applications. It provides a simple and efficient way to manage global state by storing it in a single object.
Zustand is designed to be easy to use and understand, making it a great choice for developers of all levels. By using Zustand, you can easily manage state across your entire application.
Zustand uses a single object to store all of your application's state, making it easy to access and update state from anywhere in your code. This object is called the "store".
Discover more: Using State in Next Js
The store is the central hub of your application's state, and it's where you'll spend most of your time working with Zustand. By using the store, you can easily access and update state from anywhere in your code.
One of the key benefits of using Zustand is that it helps to avoid the "prop drilling" problem that can occur when working with complex state management systems. Prop drilling occurs when you have to pass state down through multiple levels of your component tree, which can be cumbersome and error-prone.
See what others are reading: Nextjs State Management
Store and State Management
Store and State Management is a crucial aspect of building scalable and maintainable applications with Zustand and Next.js.
You can create a store to manage the state of your shopping cart, for example, with the createfunction from Zustand. The store has three properties in its state: cart, totalItems, and removeFromCart. You can use this store in your components to access and update the cart status easily.
In order to persist the state, you can use the persist middleware to store the state in the browser's local storage. This way, the state will be maintained even after the page is reloaded or the browser is closed. However, you might encounter an error if the state sent by the server does not match the state rendered on the client.
To fix this error, you can create a local state with useState and use useEffect to set the local state with the store data. This will help avoid inconsistencies and ensure that the local state is updated when mounting the component.
For your interest: Use Client Nextjs
Store for Cart
Creating a store for the cart is a crucial step in managing the state of your shopping cart. You can use the create function to create a store that manages the state of the cart.
The store has three properties in its state: cart, which is an array of products added to the cart, totalItems, which is the total number of products in the cart, and removeFromCart, which removes a product from the cart and updates the status accordingly.
See what others are reading: Create Cookies Nextjs
The store uses the set function provided by Zustand to update the shopping cart state immutably, maintaining the integrity of the state at all times. This allows you to access and update the shopping cart status easily and efficiently in your components.
To create the store, you can use the following code snippet:
```markdown
useCartStore:
types.d:
In this code, we use createfunction to create a store to manage the state of the shopping cart.
```
Here's a summary of the store properties:
This store provides a centralized way to manage the state of your shopping cart, making it easier to access and update the cart status in your components.
Configure the Store
To create a store in Zustand, you need to define the shape of your application state. This is done by creating separate slices for different parts of your state, such as products and cart.
The product slice is a good place to start. Create a file called `createProductSlice.ts` inside the `lib/slices` directory and define an interface `Product` that represents the shape of the data returned by your fake products API endpoint.
Take a look at this: Create Next Js App
Here's an example of what the `Product` interface might look like:
```html
- interface Prod
id: number;
name: string;
price: number;
}
```
The product slice should also include a `fetchProducts` method that fetches the list of products from the API.
Next, create a file called `createCartSlice.ts` inside the `lib/slices` directory and define an interface `Cart` that represents the shape of the cart state. This should include properties for the cart items, such as `cart`, `addToCart`, `removeFromCart`, `updateQuantity`, and `showCart`.
Here's an example of what the `Cart` interface might look like:
```html
- interface C
cart: Product[];
addToCart: (product: Product) => void;
removeFromCart: (productId: number) => void;
updateQuantity: (productId: number, quantity: number) => void;
showCart: boolean;
}
```
Finally, create a file called `store.ts` in the `lib` directory and use the `create` function from `zustand` to create the store. This will allow you to access the product and cart slices as part of the store.
Here's an example of what the `store.ts` file might look like:
```html
import { create } from 'zustand';
import { ProductSlice, CartSlice } from './slices';
const store = create(() => ({
productSlice: ProductSlice(),
cartSlice: CartSlice(),
}));
export default store;
```
Additional reading: Nextjs File Structure
Creating and Managing Components
To create components in Zustand Next.js, you simply import the hook of the corresponding store and extract the states or actions you want to use in your component.
You can see this in action by looking at the Header.tsx and Cart.tsx files, where the state of Zustand is used to display and manage the cart.
The key is to import the necessary hook and extract the relevant states or actions, making it easy to reuse and manage your components across the application.
This approach allows you to keep your components organized and focused on their specific tasks, without worrying about the underlying state management.
By following this pattern, you can create a basic shopping cart that works, as shown in the example code.
However, to further extend the functionality of your cart, you can supplement it with more features according to your specific needs and requirements.
To do this, you can use middleware to persist the state, ensuring that items already in the cart are not lost, even if the page is reloaded or closed.
The useProductsStore hook receives two arguments, allowing you to customize its behavior and fit it to your application's needs.
If you want to change the name of totalItems to totalProducts, you can simply modify the hook to reflect this change.
A unique perspective: 'use Server' Nextjs
State Operations
In a Zustand store, the State interface represents the state structure, which includes a list of products, the loading status, and any errors that may occur during data loading.
The State interface is crucial in managing the application's state, ensuring that the data is accurately reflected in the UI.
To manage the state, you need to define the State interface, which will serve as the foundation for the store's data structure.
On a similar theme: Next Js App Router Project Structure
Fetching
Fetching is a crucial part of state operations, and it's great that we're using Zustand to handle it.
We'll define two important interfaces: State and Actions. The State interface will represent the state structure of the store, which includes a list of products, the loading status, and any errors that may occur during data loading.
The State interface is essential for managing the data in our store, and it's great that we're thinking about the loading status and potential errors from the start.
In this case, we'll have one action called fetchData, which will be used to load the product data from the API. This action will be a key part of our state operations, and it's great that we're keeping it simple and focused on a single task.
Related reading: Next Js Tailwind Spinner Loading Page
Seven Answers
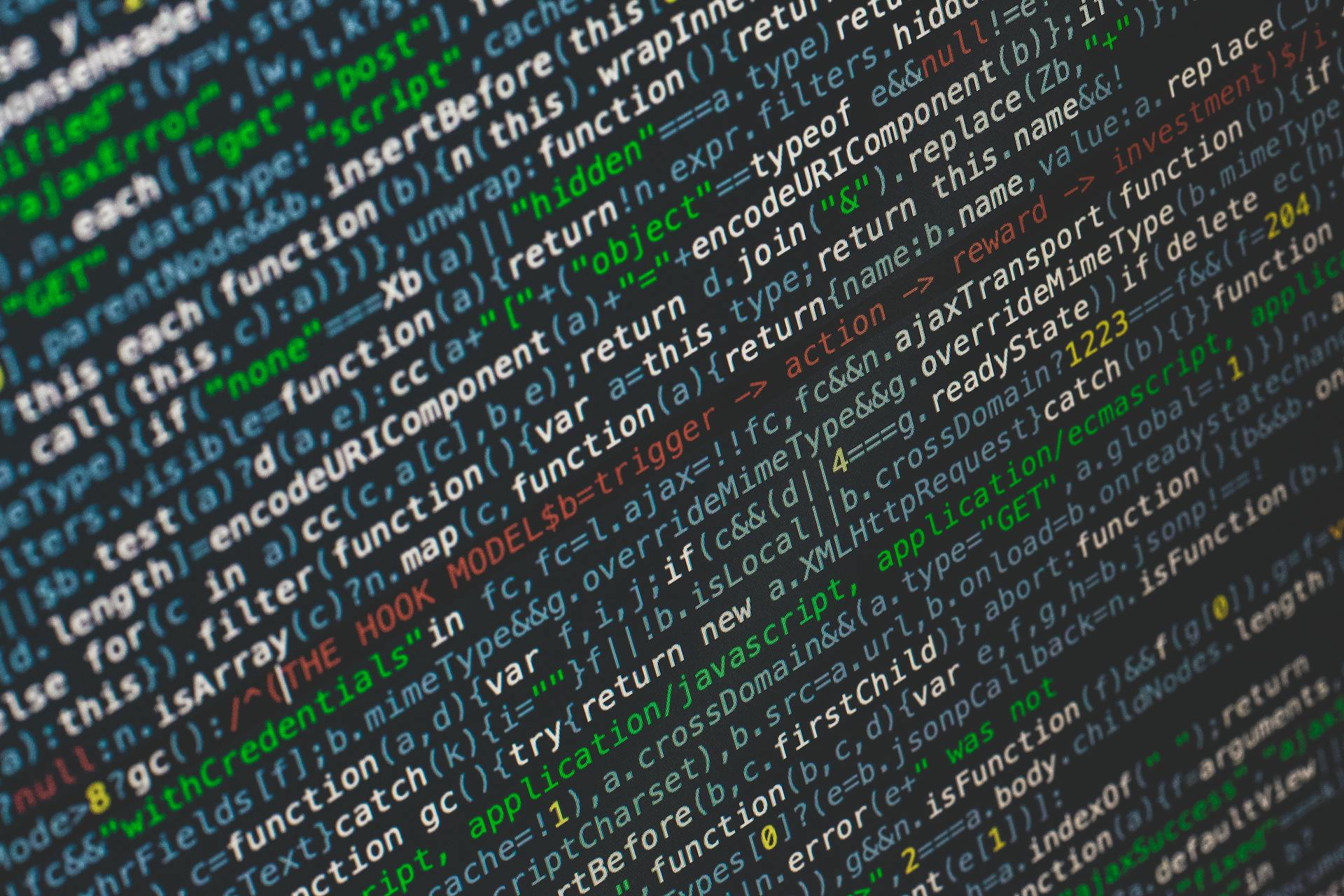
State operations can be tricky, especially when it comes to persisting state between pages while using server-side rendering (SSR) with Next.js. You want to show a user's profile picture as they move between pages, but you're getting an error saying that the client-side content doesn't match the server-rendered HTML.
You're saving data into the user's browser's local storage using Zustand, but it's not working as expected. The error message from Next.js points to a mismatch between the server-side and client-side variables.
To solve this, load your data from local storage only in the `useEffect` hook, equivalent to `onMounted` in Nuxt/Vue. This ensures that the client-side HTML differs, and the variable is loaded after the component/page has first rendered the HTML.
Here are some solutions to this problem:
- Use Zustand's `skipHydration` property to control when hydration occurs.
- Create a Next.js Layout and wait until the first `useEffect` hook fires to render child elements.
- Update your Zustand state data inside `useEffect()` to a new `useState()`.
You can also try setting `isSSR` to `true` in your Next.js page, assuming it's being server-side rendered, and then use `useEffect` to set it to `false` on client-side immediately the page gets mounted.
Remember, these solutions are specific to Next.js and Zustand, so make sure to adapt them to your project's needs.
Additional reading: Next Js Client Side Rendering
Sources
- https://hackernoon.com/how-to-build-a-shopping-cart-with-nextjs-and-zustand-state-management-with-typescript
- https://greenonsoftware.com/articles/integrations/how-to-integrate-state-management-in-zustand-with-nextjs/
- https://articles.wesionary.team/a-guide-to-managing-state-in-next-js-with-zustand-22a2899e5d0f
- https://stackoverflow.com/questions/72649255/nextjs-zustand-persist-state
- https://reactdev.ru/libs/zustand/guides/nextjs/
Featured Images: pexels.com